What Is the FizzBuzz Algorithm?
by reclaimer94 in Workshop > Science
1107 Views, 0 Favorites, 0 Comments
What Is the FizzBuzz Algorithm?
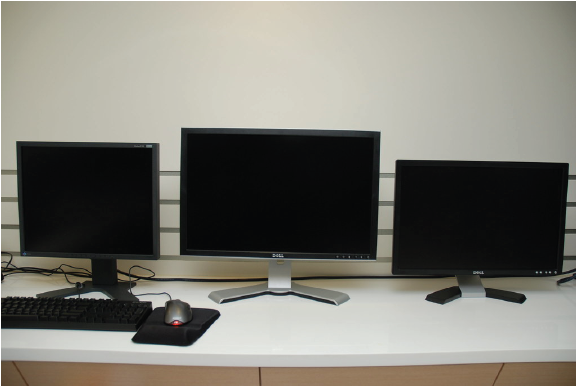
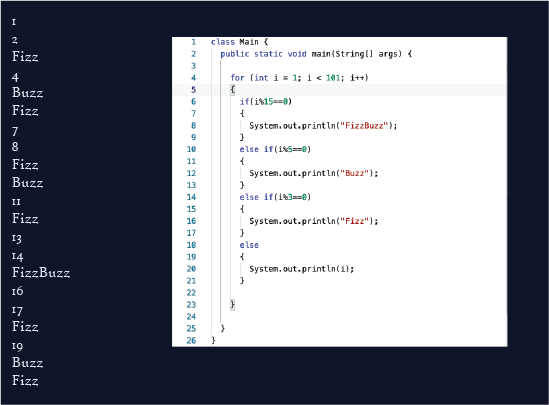
FizzBuzz is a common algorithm asked during an interview in which programming is involved. The prompt typically asks the person to write a program that prints out the numbers 1-100 with every multiple of 3 being placed with "Fizz" and every multiple of 5 being replaced with "Buzz". If a number is a multiple of both 3 and 5, the output should be "FizzBuzz".
The algorithm can be done in many different ways and in any language the author chooses. The goal of this how-to is to demonstrate one way the algorithm can be completed in Java.
Setting It Up
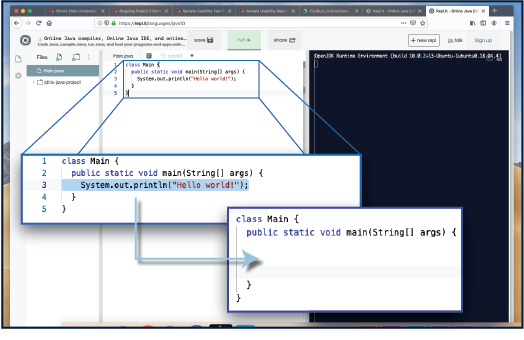
Step 1: Navigate tot he following website https://repl.it/languages/java10
This site is used to demonstrate the algorithm without needing to download anything extra. We need Java to compile in order to ensure our program works.
Step 2: Clear the code that is placed in the middle.
This space is where we are going to write our code to create the algorithm.
The for Loop
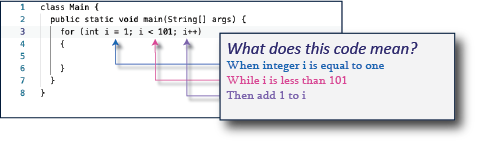
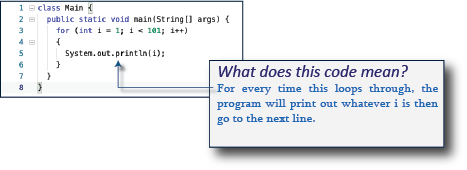
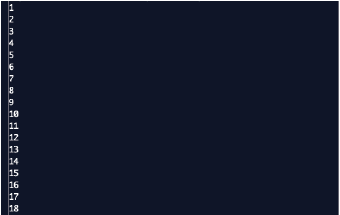
Step 3: Write the for loop
for( int i = 0; i < 101; i++)
What does this code mean?
When integer i is equal to one; while i is less than 101; then add 1 to i
Step 4: Have the output print to 100
System.out.println(i);
What does this code mean?
For every time this loops through, the program will print out whatever i is then go to the next line.
Step 5: Run your code to verify it works.
If/Else Statements
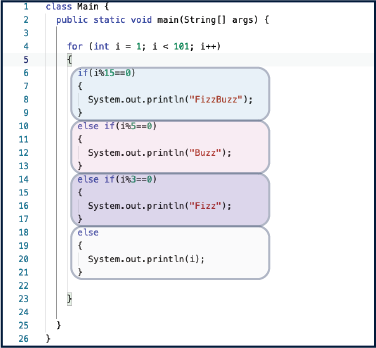
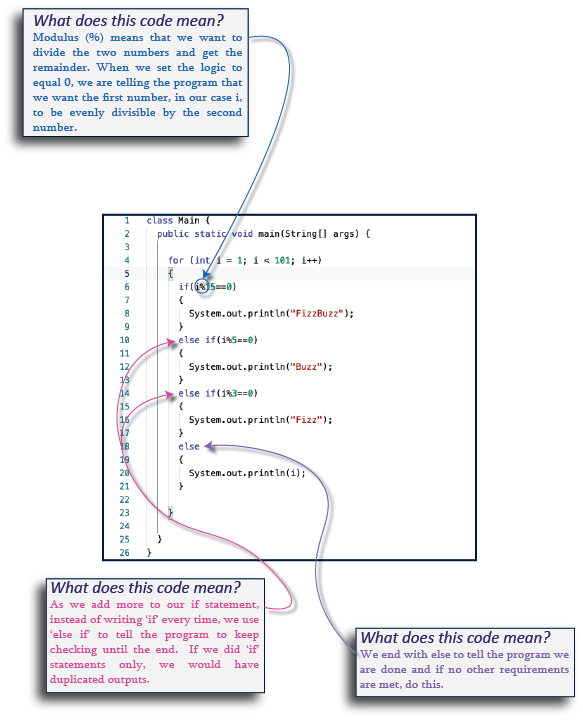
The following steps will all take place within the for loop we created earlier.
Step 6: Start the if statement with a logic statement looking for anything divisible 15
Step 7: Continue the if statement with a logic statement looking for anything divisible 5
Step 8:Continue the if statement with a logic statement looking for anything divisible 3
What does this code mean?
Modulus (%) means that we want to divide the two numbers and get the remainder. When we set the logic to equal 0, we are telling the program that we want the first number, in our case i, to be evenly divisible by the second number.
Step 9: Finish the if statement with printing everything else that isn’t any of the previous requirements What does this code mean? As we add more to our if statement, instead of writing ‘if’ everytime, we use ‘else if’ to tell the program to keep checking until the end. If we did ‘if’ statements only, we would have duplicated outputs. We end with else to tell the program we are done and if no other requirements are met, so this .
Final Product
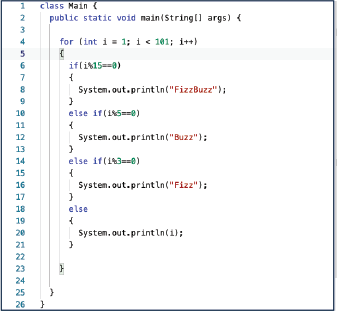
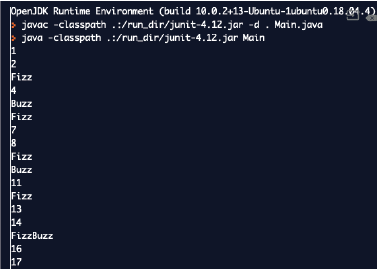
When you’re finished with your code, it should look like the picture above.
Providing you followed everything, you should be able to run your code and receive the following output:
Common Errors
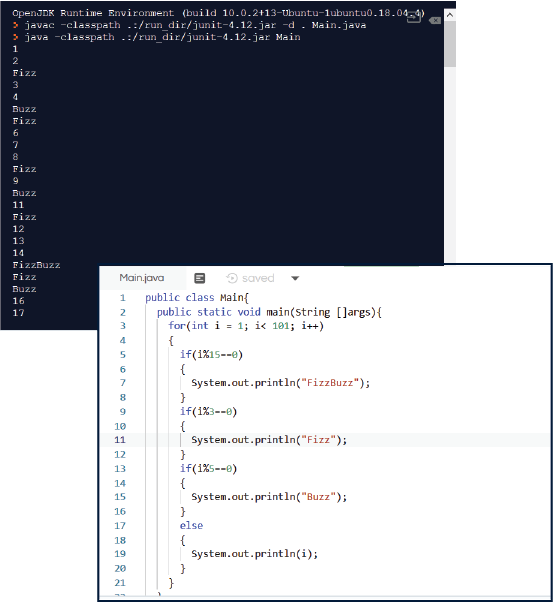
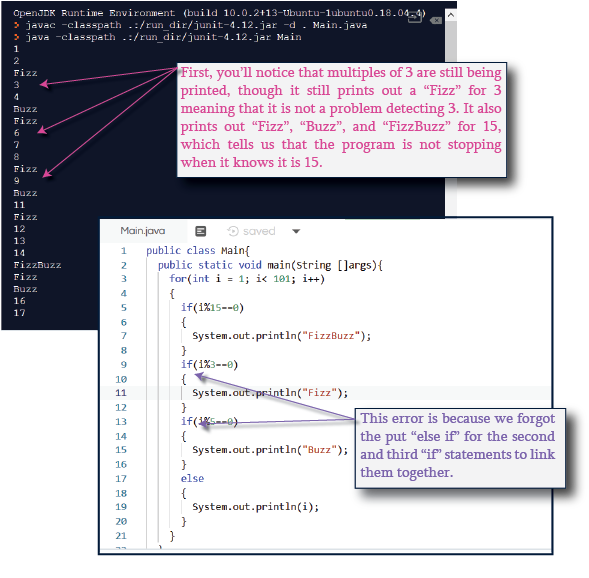
If you inspect the output closely you’ll notice some discrepancies. First, you’ll notice that multiples of three are still being printed, though it still prints out a “Fizz” for three, meaning that it is not a problem detecting three. It also prints out “Fizz”, “Buzz”, and “FizzBuzz” for fifteen, which tells us that the program is not stopping when it knows it is fifteen. This error is because we forgot the put “else if” for the second and third “if” statements to link them together. This code treats each only the final two possibilities, so if the number is not divisible by five it will always print out the number. And when it finds a fifteen it does not immediately stop, so it continues to check both three and five, which are both then also printer. This is exactly what we saw in the terminal, lending further credibility.
Troubleshooting
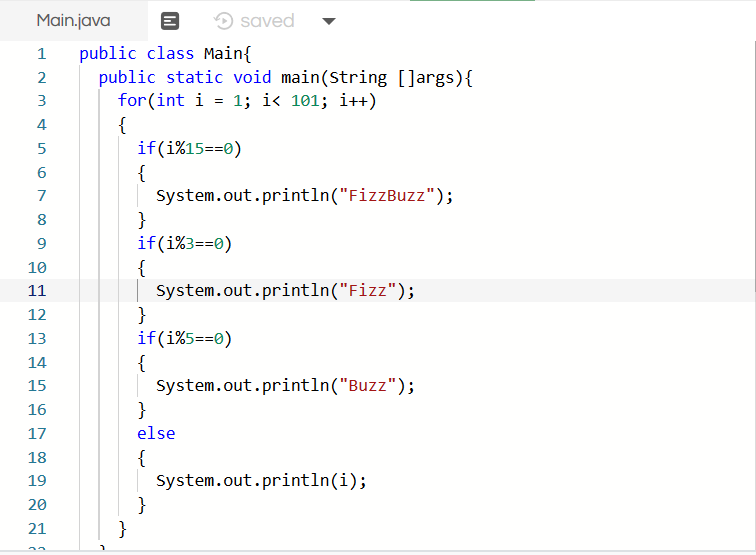
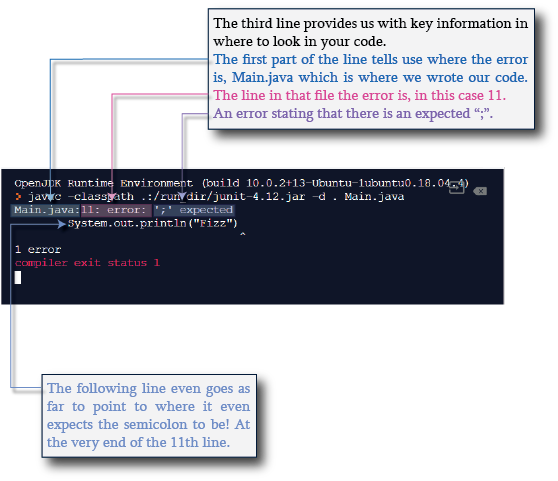
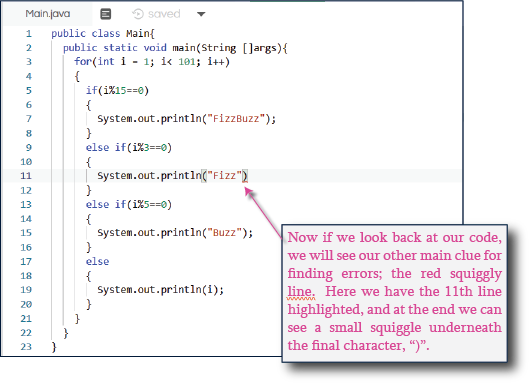
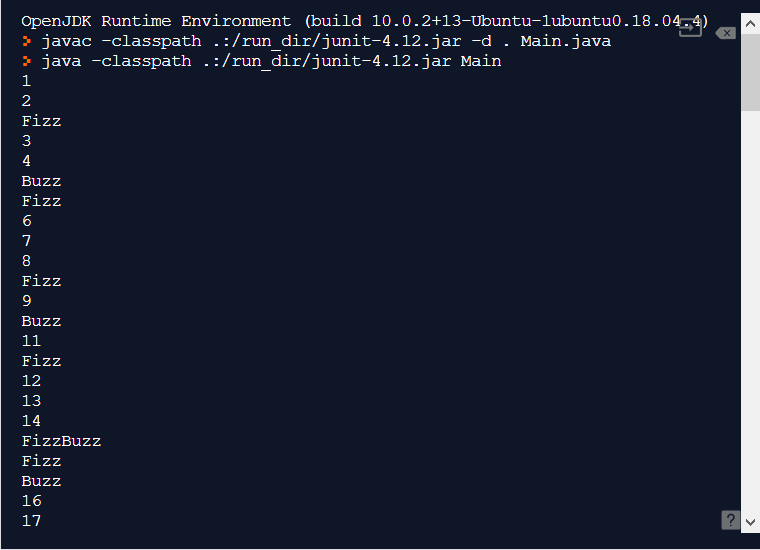
So let’s say you followed the guide well and your code looks just like the pictures, but your output is something we haven’t covered, and does not look it. This is where troubleshooting comes in. Modern IDEs (Integrated Development Environment, applications which help you code) like the one on Repl.it, will provide you with two primary sources.
In the Terminal/Output:
When you write the code in a way that the compiler (translates your code into stuff the computer can use) does not understand, it will throw an “exception” and display some information.
Here we are looking at at the third and fourth lines. The third line provides us with key information in where to look in your code. The first part of the line tells use where the error is, Main.java which is where we wrote our code; the line in that file the error is, in this case 11; and an error stating that there is an expected “;”. The following line even goes as far to point to where it even expects the semicolon to be! At the very end of the 11th line. Now if we look back at our code, we will see our other main clue for finding errors; the red squiggly line.
In the editor:
Here we have the 11th line highlighted, and at the end we can see a small squiggle underneath the final character, “)”. It can be easy to miss when it is so small, but luckily we knew where to look! There are plenty of colors you may see in an IDE, but red usually indicates changes are needed. Here the changes we need to make are just adding the missing semicolon and our code will work like a charm! Even if your error is not exactly the same one discussed above, if you use the tools discussed (red squiggily line and terminal information) then you should be able to troubleshoot your way to working code.