Thermocouple Type K - Arduino ESP32
by T_rent1998 in Circuits > Arduino
31 Views, 0 Favorites, 0 Comments
Thermocouple Type K - Arduino ESP32
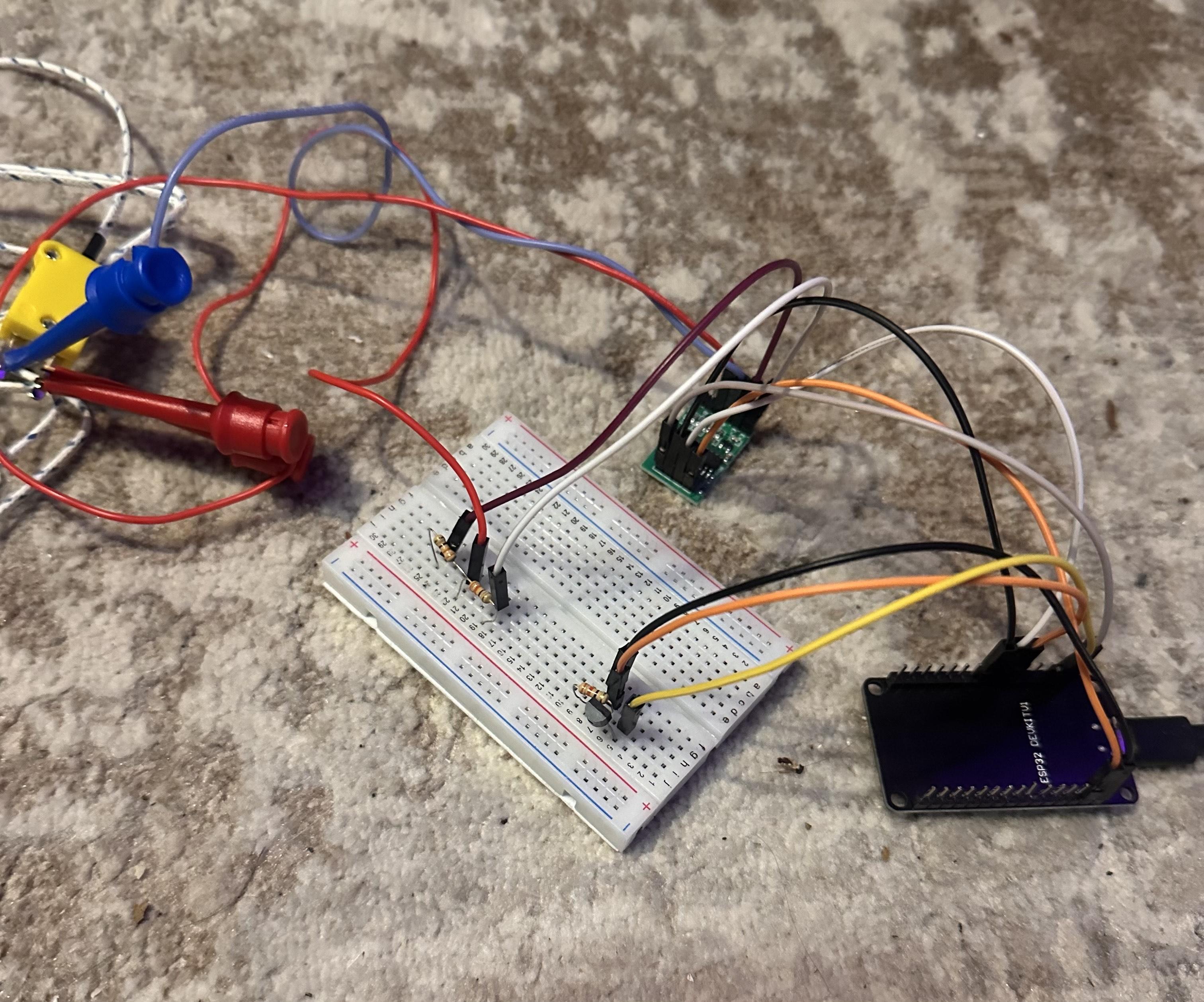
Intro - ESP 32 microcontroller circuit was set up with a HS711 amplifier to read a thermocouple type K for a reference input from digital thermometer.
Supplies
- 1 - Breadboard
- 1 - Thermocouple type K
- 1 - HS711 Amplifier
- 1 - Arduino ESP 32
- 1 - DS18B20 Digital Thermometer
- 3 - resistors
- 5 - male to female jumper wires
- 3 - female to female jumper wires
- 1 - USB to ESP 32 connector
- 2 - hook up clips (female)
- 1 - hook up clip (male)
Connect Electronic Technology
From ESP 32
- D25 -> SCK on HS711 Amplifier
- D26 -> DT on HS711 Amplifier
- VIN -> VCC on HS711 Amplifier
- GND -> GND on HS711 Amplifier
- D27 -> spot A2 on breadboard
- GND -> spot A1 on breadboard
- 3V3 -> spot A3 on breadboard
From HS711 Amplifier
- A+ -> small prong on DS18B20 digital thermometer
- A- -> large prong on DS18B20 digital thermometer
- E+ -> spot F1 on breadboard
- E- -> spot F6 on breadboard
From DS18B20 digital thermometer
- Small prong -> F3 on breadboard
On breadboard
- GND - spot A1 on breadboard
- D27 - spot A2 on breadboard
- 3V3 - spot A3 on breadboard
- Resistor (23k ohms) B2 to B3
- Thermocouple (semi circle outward from rest of connections) in C1, C2, C3
- E+ - spot F1 on breadboard
- E- - spot F6 on breadboard
- Small prong - F3 on breadboard
- Resistor (23k ohms) G1 to G3
- Resistor (23k ohms) G3 to G6
Initialize Arduino ESP 32
Using a USB plugin, plug in the Arduino to your computer.
Initialize the Arduino by downloading Arduino IDE (arduino.cc/en/software)
Under boards manager, search and install Arduino ESP32 Boards by Arduino
Click on ‘select board’, select port, search for and select ‘DOIT ESP32 DEVKIT V1’
Run Code
Under Files, Examples, HX711 Arduino Library, HX711_Basic_Example
#include "HX711.h"
// HX711 circuit wiring
const int LOADCELL_DOUT_PIN = 26;
const int LOADCELL_SCK_PIN = 25;
HX711 scale;
const float Vref = 2.0; // Reference voltage in volts
const int gain = 128; // Gain factor for HX711
const long maxBits = 8388608; // 2^23, the maximum code for 24-bit ADC
void setup() {
Serial.begin(57600);
scale.begin(LOADCELL_DOUT_PIN, LOADCELL_SCK_PIN);
}
void loop() {
if (scale.is_ready()) {
long reading = scale.read();
// Calculate Vtc from the code
float Vtc = (float(reading) * Vref) / (gain * maxBits);
// Print raw reading and calculated voltage
Serial.print("HX711 reading: ");
Serial.print(reading);
Serial.print(" => Vtc: ");
Serial.print(Vtc, 6); // 6 decimal places for precision
Serial.println(" V");
} else {
Serial.println("HX711 not found.");
}
delay(1000);
}
For LOADCELL_DOUT_PIN = 26 and LOADCELL_SCK_PIN = 25
Run code
Downloads
Solve for Voltage of Thermocouple
Update code to find V_tc
V_ref = 2V, gain = 128, 24 bits-sign = 2^23
V_tc = (code x V_ref) / (gain x 2^23)
Add code for solving and press upload
Using Thermocouple Type K reference table, use V_tc to find temperature