Street Sweep Alert
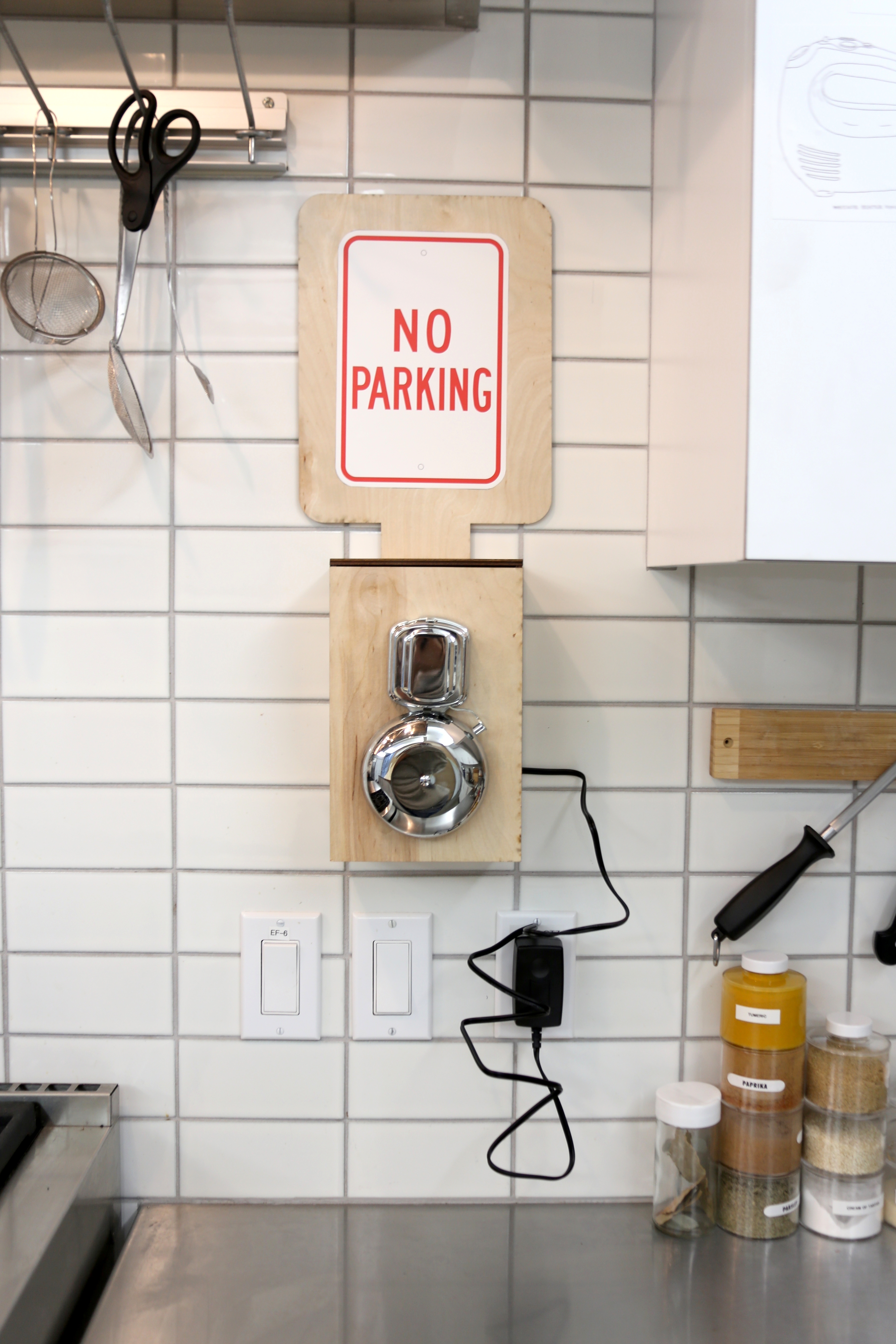
I recently moved up to San Francisco and, being an LA native, have noticed a few cultural differences. The first and most noticeable is that any store, whether it be a 7-Eleven or hardware store, always carries fancy artisan chocolate. The second is that parking is crazy, even crazier than LA. That is why I built the Street Sweep Saver. You are bound to rack up a few parking tickets in this city, so why not avoid a couple with this alarm that reminds you to move your car for street sweeping?
The Street Sweep Saver is an alarm that rings 10 minutes before street sweeping begins on your block. The alarm is made with an electric bell controlled by a beagle bone black board. I designed a wooden casing that hides the electronics. Mount the Street Sweep Saver to your wall or place it on your kitchen table next to your daily cup of joe.
Parts List:
BEAGLE BONE BOARD
- (1x) Getting Started with the BeagleBone Kit RadioShack 277-198
- (1x) Ethernet Cable RadioShack 55008238
- (1x) 5V AC DC power supply RadioShack 55057413
- (1x) Duracell 8G microSD Card with Adapter RadioShack DU-3IN1-08G-R
CIRCUIT
- (1x) Proto Cape Kit for the BeagleBone
- (4x) 'AA' batteries RadioShack 23-2212
- (1x) Enclosed 4 'AA' battery holder RadioShack 270-409
- (1x) NPN transistor RadioShack 276-1617
- (1x) 100 ohm, 1/4 watt resistor RadioShack 271-1311
- (2x) Insulated jumper lead RadioShack 278-1156
- (1x) Electric bell Build.com BCI2105746
BOX
- 3/16" thick plywood
- wood glue
- painters tape
- clamps
- vinyl or sticky paper
- hand drill
Communicating With the Beaglebone Black Board
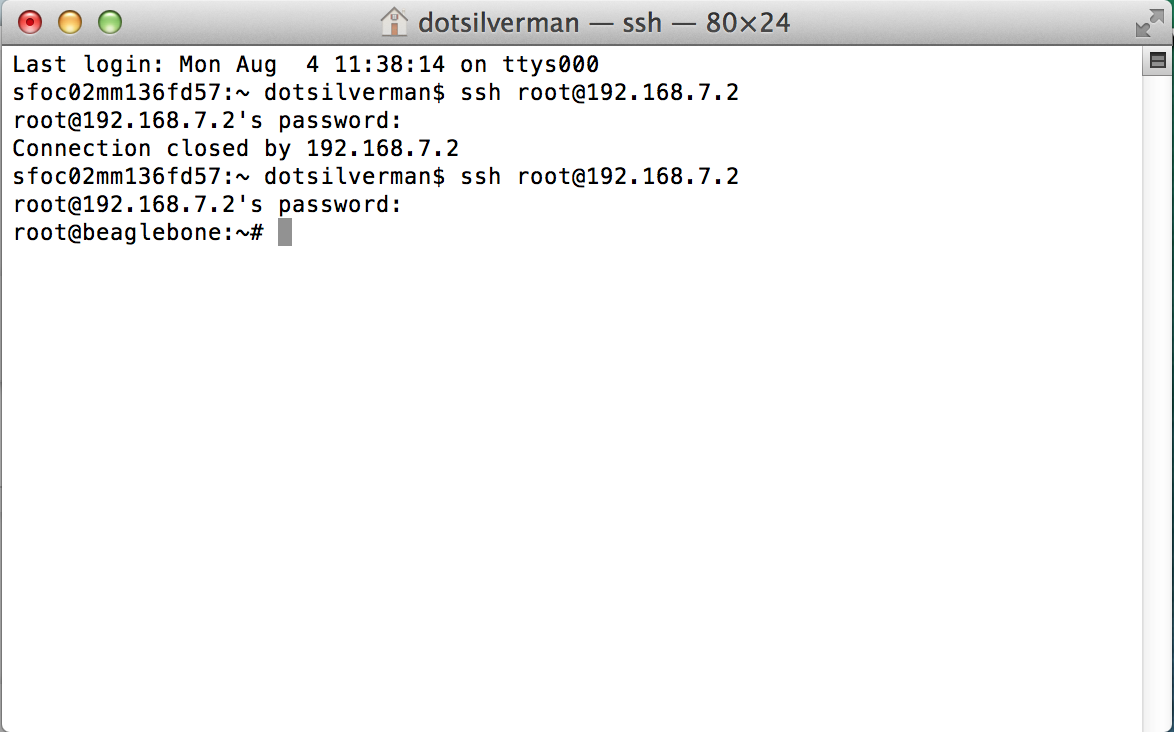
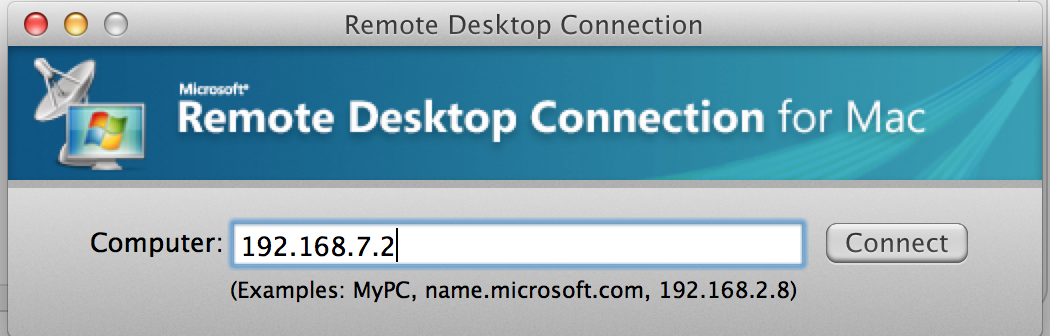
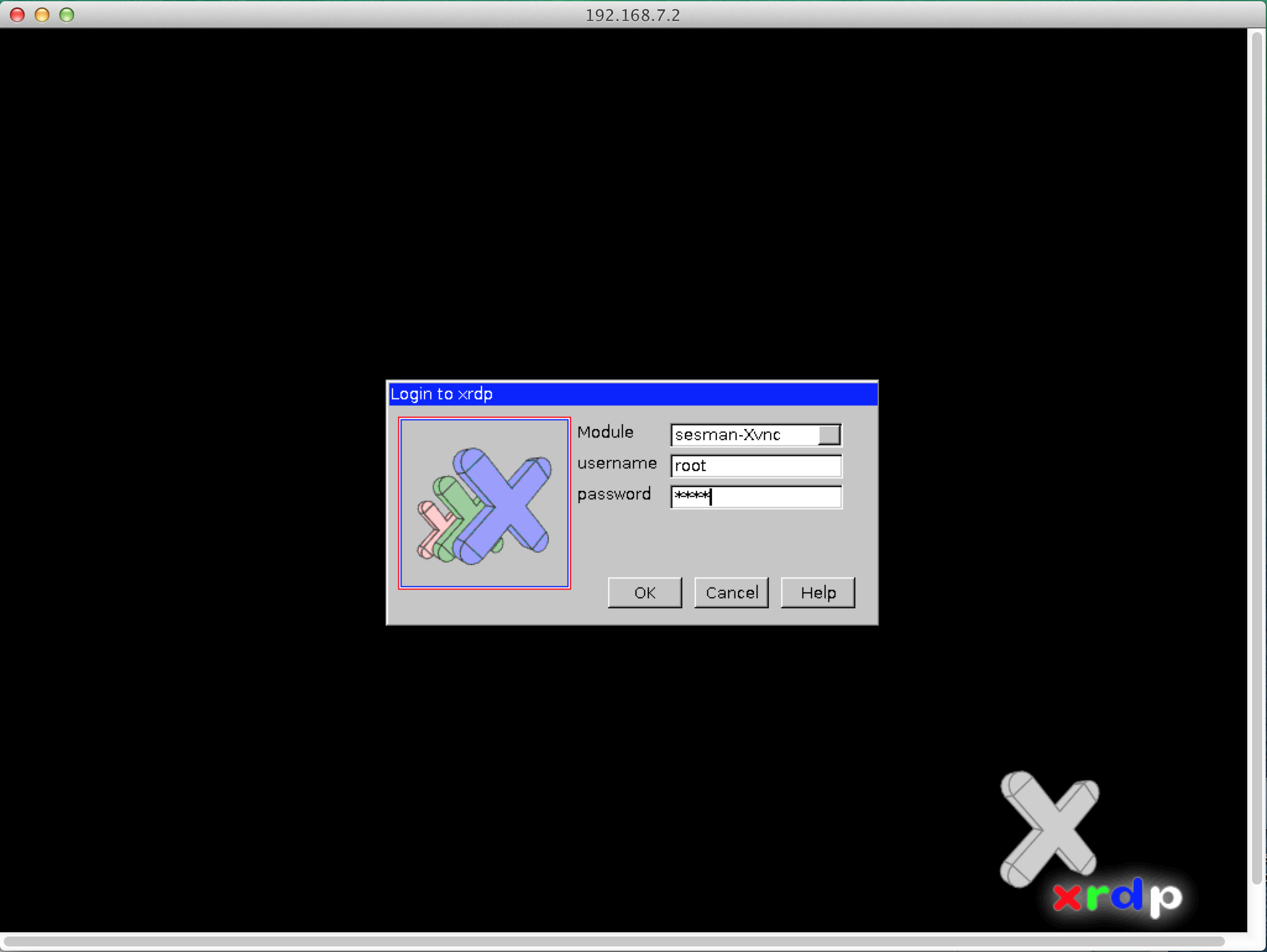
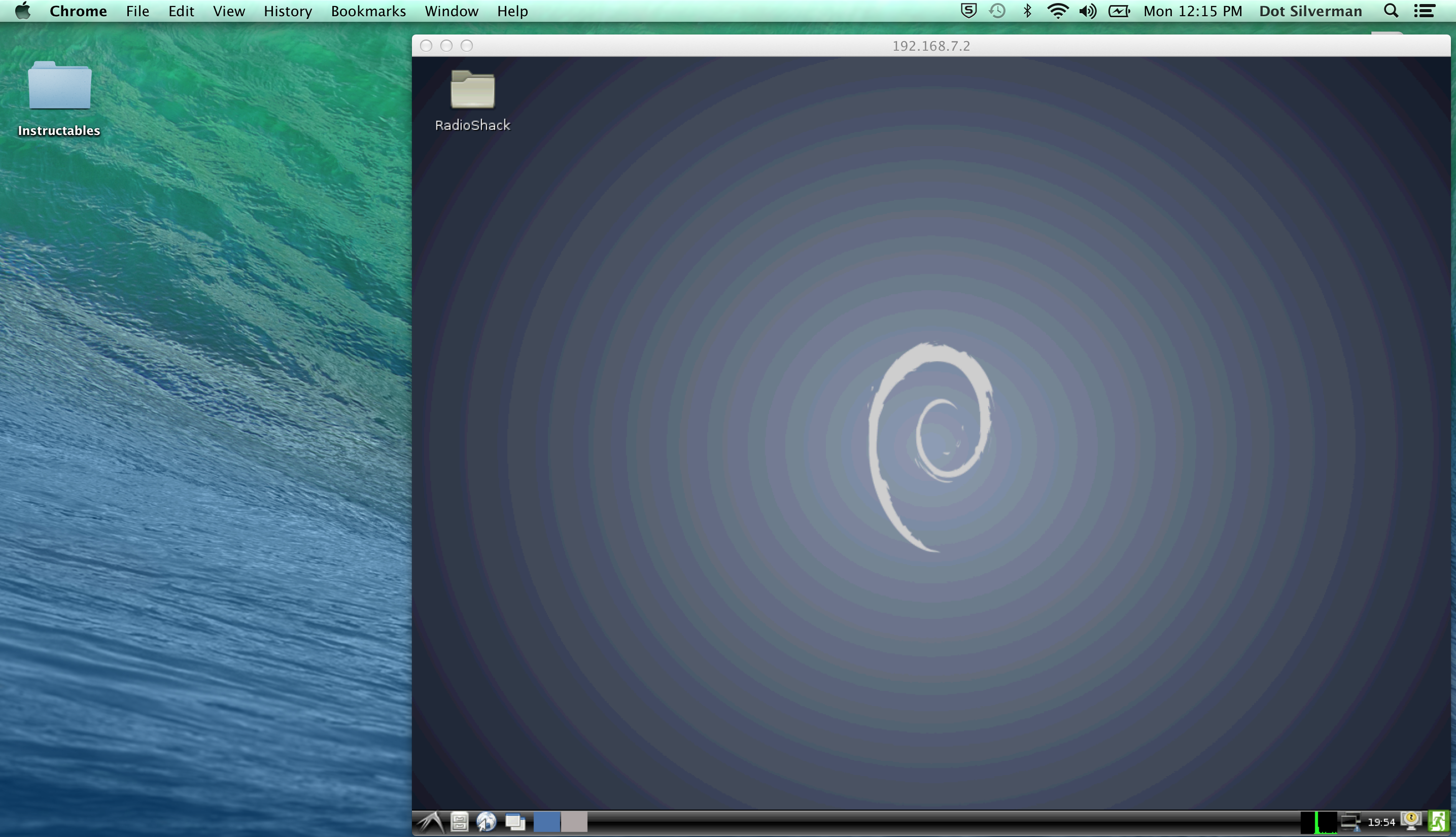
This project is my first experience with the BeagleBone Black Board. In my opinion, the BeagleBoard is quite similar to the RaspberryPi board but with more gpio pins. Read more about the BeagleBoard at its official community site and discover the main differences between the BeagleBoard and Raspberry Pi here.
This project uses a Rev C BeagleBone Black board running off a 16G micro SD card flashed with the Debian environment. You can use any size micro SD card greater than 8G. Although the new BeagleBone Black Board has a 4G onboard processor, Debian's desktop environment requires more storage space. To interact with the board, simply plug it into your computer with the micro HDMI to USB cable that comes with your board. The 'user leds' located next to the ethernet port should flash in a pattern programmed to mimic the human pulse (the beagle makers are cool, right?) and the board's icon should pop onto your Desktop. If you are using a mac, you can program your board two different ways:
METHOD 1: THE TERMINAL
-
Open the terminal and type into the command line
ssh root@192.168.7.2If when you are prompted for a password, type
root
** If this doesn't work because of an "offending ssh key" **
Type into the command line
rm -f .ssh/known_hosts ssh root@192.168.7.2
** If this still doesn't work **
Type into the command line
sudo ssh root@192.168.7.2
METHOD 2: DEBIAN ENVIRONMENT
You can also vnc into the board's desktop environment and program it from there. Follow the steps below to do this.
- Download Remote Desktop Connection for mac from here.
- Open Remote Desktop Connection and in the input box for 'Computer' type '192.168.7.2'
- Inside the remote desktop pop-up, type 'root' for both the username and password.
- You will be presented with the Beagle Board's desktop.
Installing Adafruit's IO Python Library
The Beaglebone Black Board has lots of gpio pins, 65 in total, making it unique amongst the many mini computers and microprocessor boards on the market. Two gpio libraries compatible with the Beagle's gpio pins are BoneScript's built-in library and Adafruit's Python GPIO library. Because I am personally more comfortable with Python, I decided to use the Python GPIO library. The library is fairly simple to download. First, ssh into the Beagle Board and install the following dependencies:
sudo ntpdate pool.ntp.org sudo apt-get update sudo apt-get install build-essential python-dev python-setuptools python-pip python-smbus -y
Then type the following command into install the python gpio library
sudo pip install Adafruit_BBIO
Code
The alarm uses Python's time library to calculate the current time in hours and minutes. If the current time equals the time 10 minutes before street sweeping comes, the bell rings twice. Change cleantime1, cleandate1, cleantime2, and cleandate2 to match your street sweep schedule. The first line of the code may also need to be altered, which will be explained more in the next section.
# **ALTER IF NECESSARY** #!/usr/bin/python import Adafruit_BBIO.GPIO as GPIO import time # pin P8_10 is the bell GPIO.setup("P8_10",GPIO.OUT) # ex format of time.strftime: 'Tue Jul 29 21:44:18 2014' now = time.strftime("%c").split(' ') now_weekday = now[0] now_month = now[1] now_day = now[2] now_time = now[3] now_year = now[4] # hm = hour & minute now hm = now_time[0:5] # Example days and times for street sweeping. Changes these for your own street. # Use a 24-hour time format. Days are the first three letters, beginning with a capital letter ** ALTER IF NECESSARY ** cleantime1= '07:50' cleandate1= 'Mon' cleantime2= '07:50' cleandate2= 'Fri' if cleantime1==hm and cleandate1==now_day or cleantime2==hm and cleandate2==now_day: # bell rings GPIO.output("P8_10", GPIO.HIGH) time.sleep(0.5) GPIO.output("P8_10",GPIO.LOW) time.sleep(0.5) GPIO.output("P8_10", GPIO.HIGH) time.sleep(0.5) GPIO.output("P8_10",GPIO.LOW)
Running Code From Startup
We don't want to manually call the code every time the BBB turns on. Instead, it would be best to have the code run automatically when the board boots up.
Say, for example, the code given in the previous slide is saved under the name 'warning.py' and lives in the directory '/root/Desktop/SideParking'.
First, edit rc.local by typing into the Debian command line
> nano /etc/rc.localA window will appear with the following content
#!/bin/sh -e # # rc.local # # This script is executed at the end of each multiuser runlevel. # Make sure that the script will "exit 0" on success or any other # value on error. # # In order to enable or disable this script just change the execution # bits. # # By default this script does nothing. exit 0Add the path of warning.py before the line 'exit 0', as shown below
/root/Desktop/SideParking/warning.py
Now the BBB will automatically look at this path and execute warning.py upon boot. However, there is one more thing we have to do. We have to make the file warning.py executable. An executable file is a computer file that contains instructions in a form that a computer's operating system can understand and follow.
When a python script is executed in the terminal, it is normally done inside its own folder with the command
> python warning.pyHowever, this script can't be called elsewhere, which is problematic if we want to call it from rc.local. We avoid this problem by adding #! followed by the path to python at the top of the python script
#!/usr/bin/pythonThe example path above may not be everyone's path. To find your BBB's specific path to python, type into the command line
> which python
Next, alter the script's access permissions by typing the command
<p>> chmod +x warning.py</p>This command gives anyone file access permissions. Test that chmod worked by typing
./warning.py
outside the folder where warning.py lives. If chmod worked, warning.py should run.
Finally, test to see that the script runs from startup by rebooting the board. Type in the command> rebootssh back into the BBB with the command
> ssh root@192.168.7.2
and warning.py should run automatically.
Circuit Schematic
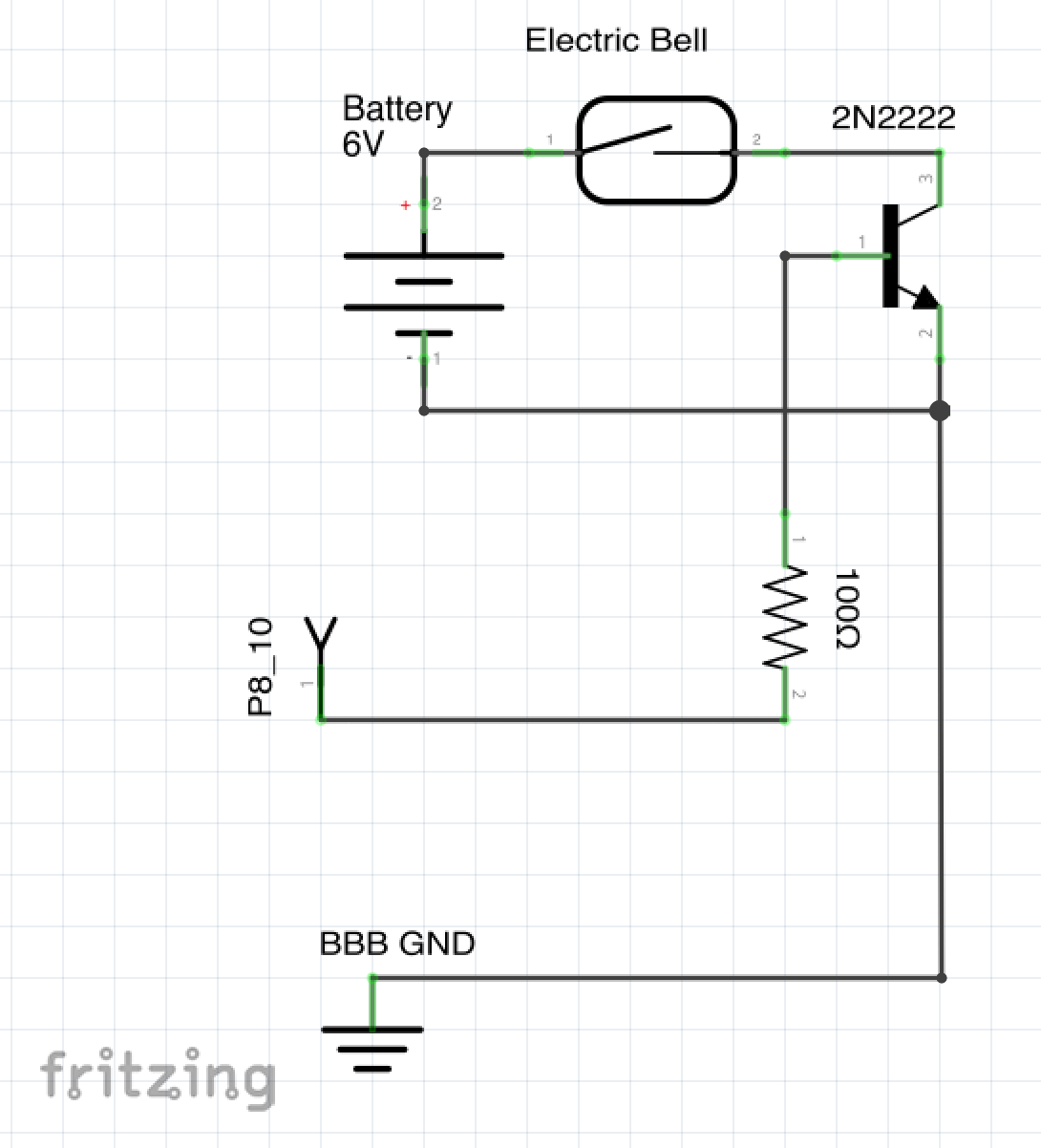
The circuit above is recreated in the next couple steps on a BeagleBone Board protocape. A few notes on the schematic:
- For the 6V power supply I used 4 1.5V AA batteries and a 4 AA Battery Holder from RadioShack. Make sure to connect the ground on the battery pack to the ground on the BBB. The positive end of the battery pack connects to one lead on the electric bell (modeled as a switch in this schematic).
- The second lead on the electric bell connects to the collector on a 2N222 npn transistor. This transistor acts like a relay, where pin 'P8_10' on the BBB controls the collector-to-emitter current flow.
Preparing the BBB Protocape
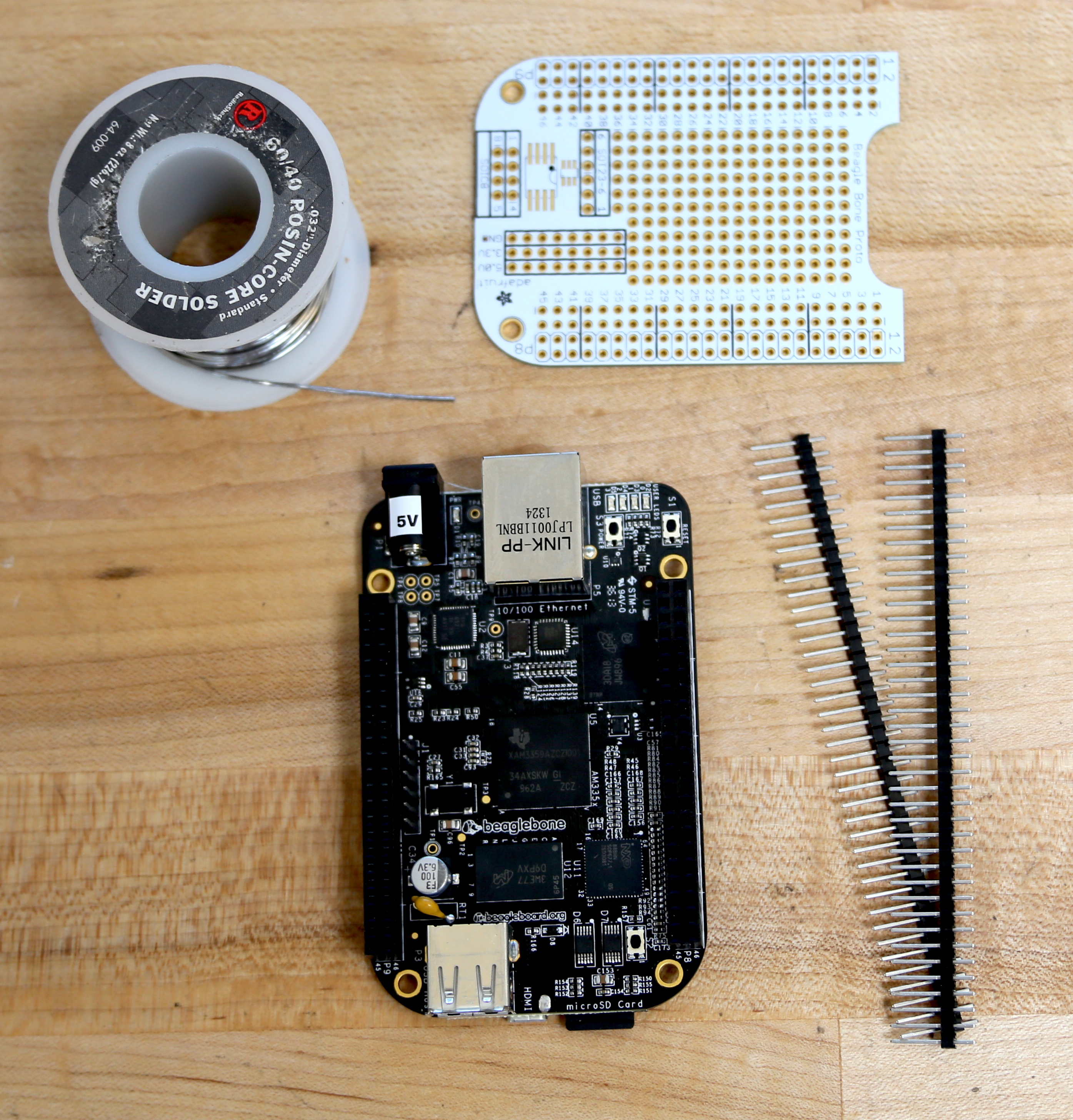
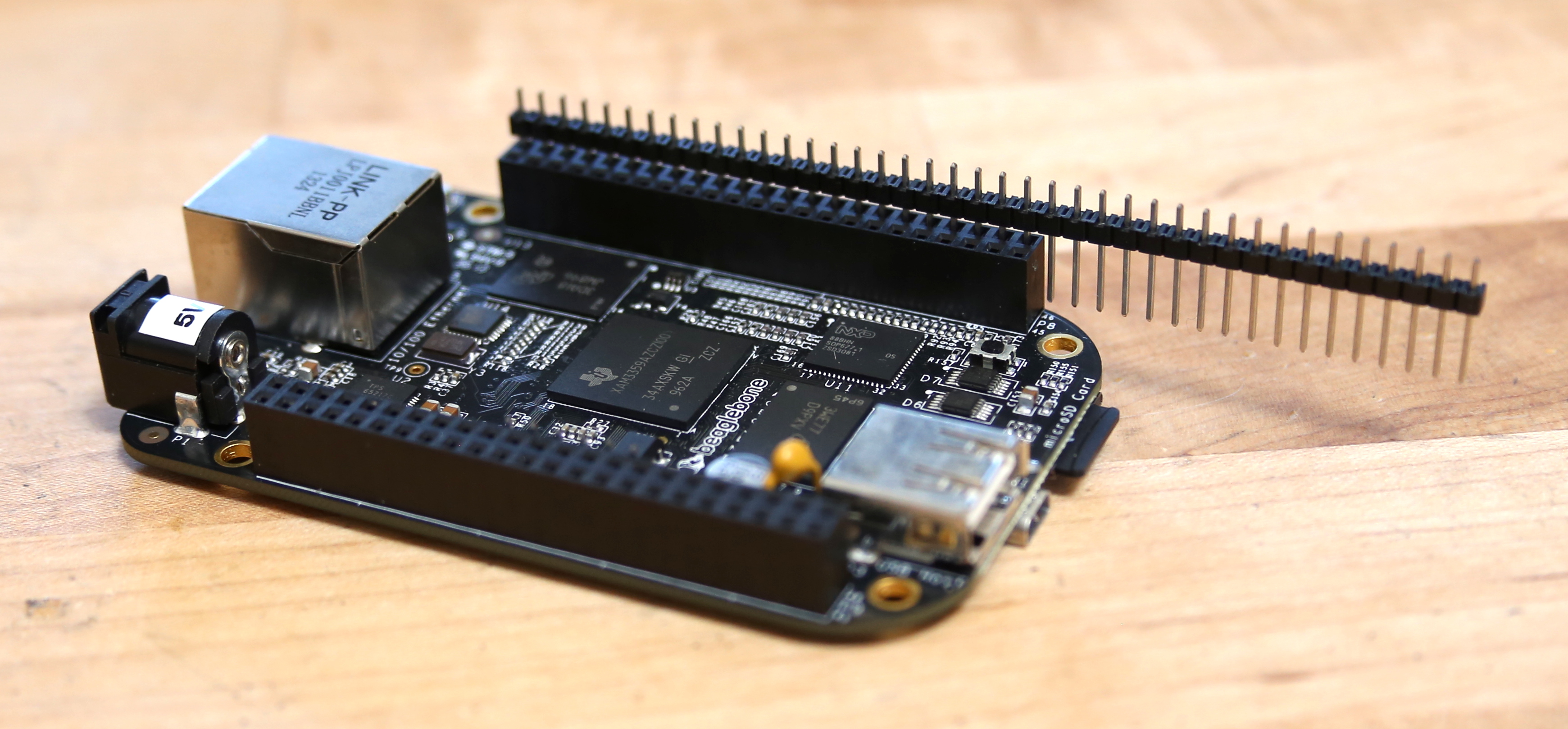
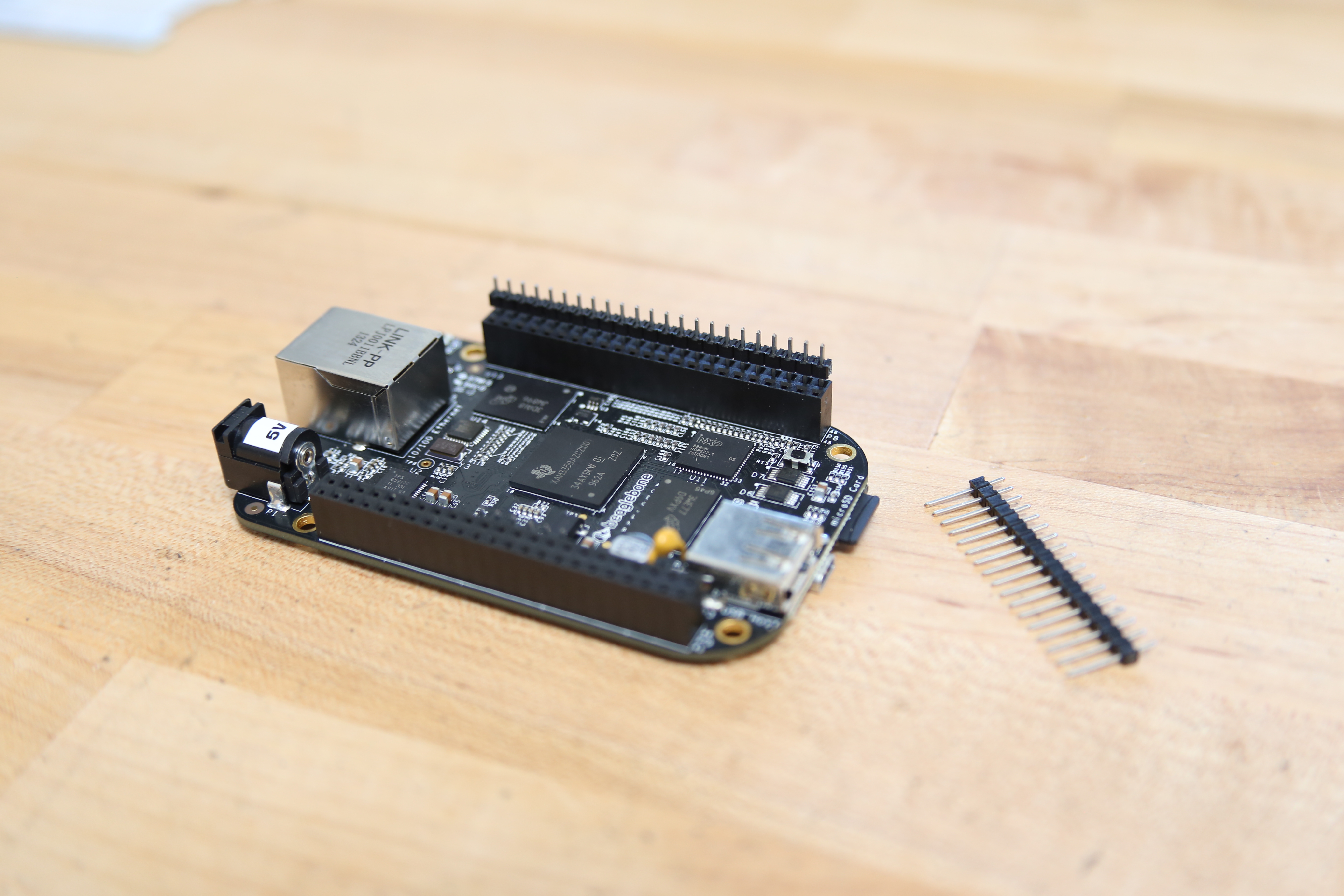
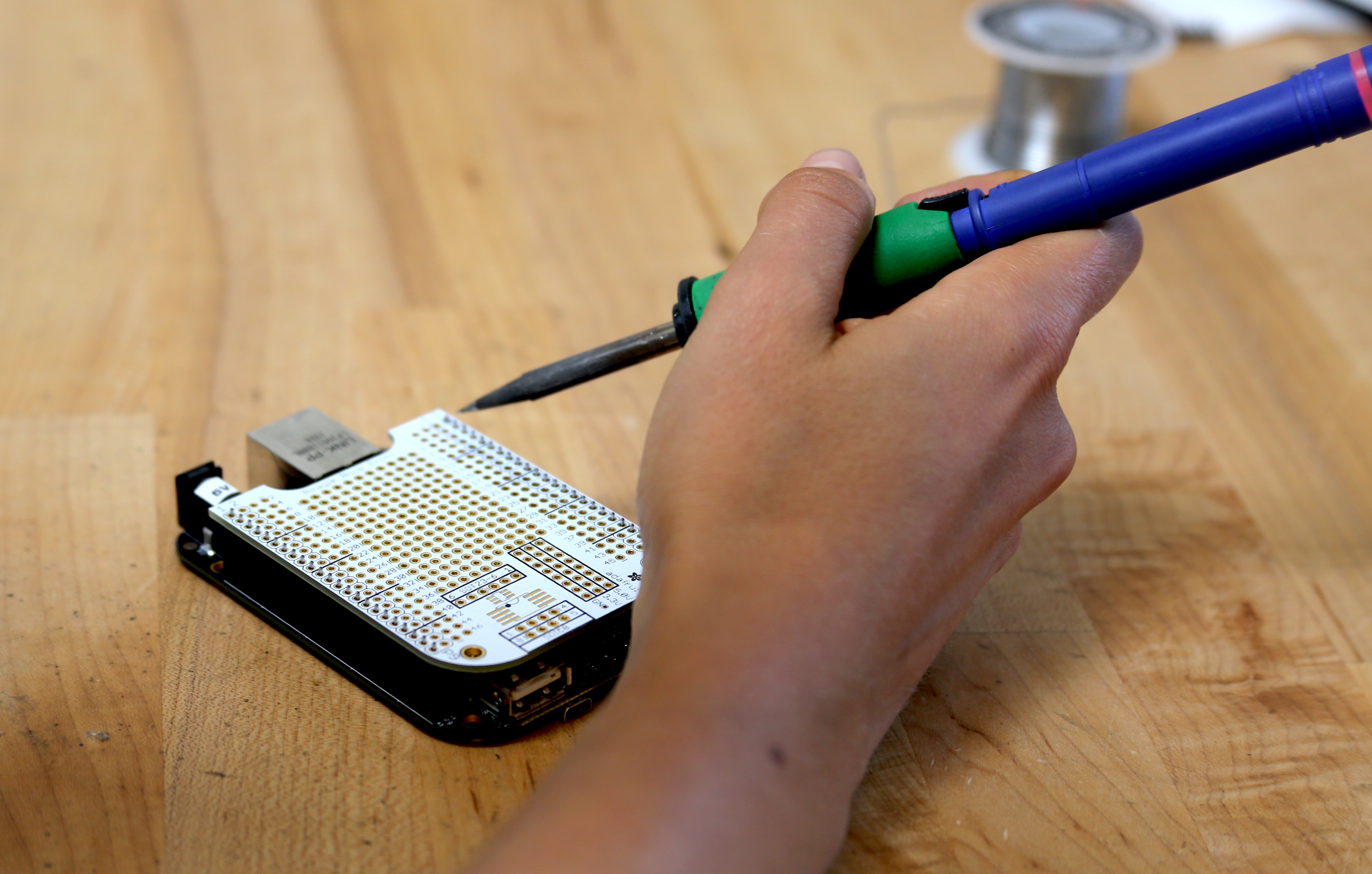
The BBB Protocape kit comes with a pair of header pins. Stick the header pins into the female pins on the BBB and break the remainder off. Rest the Protocape on top of the header pins and solder them together.
Soldering the Circuit
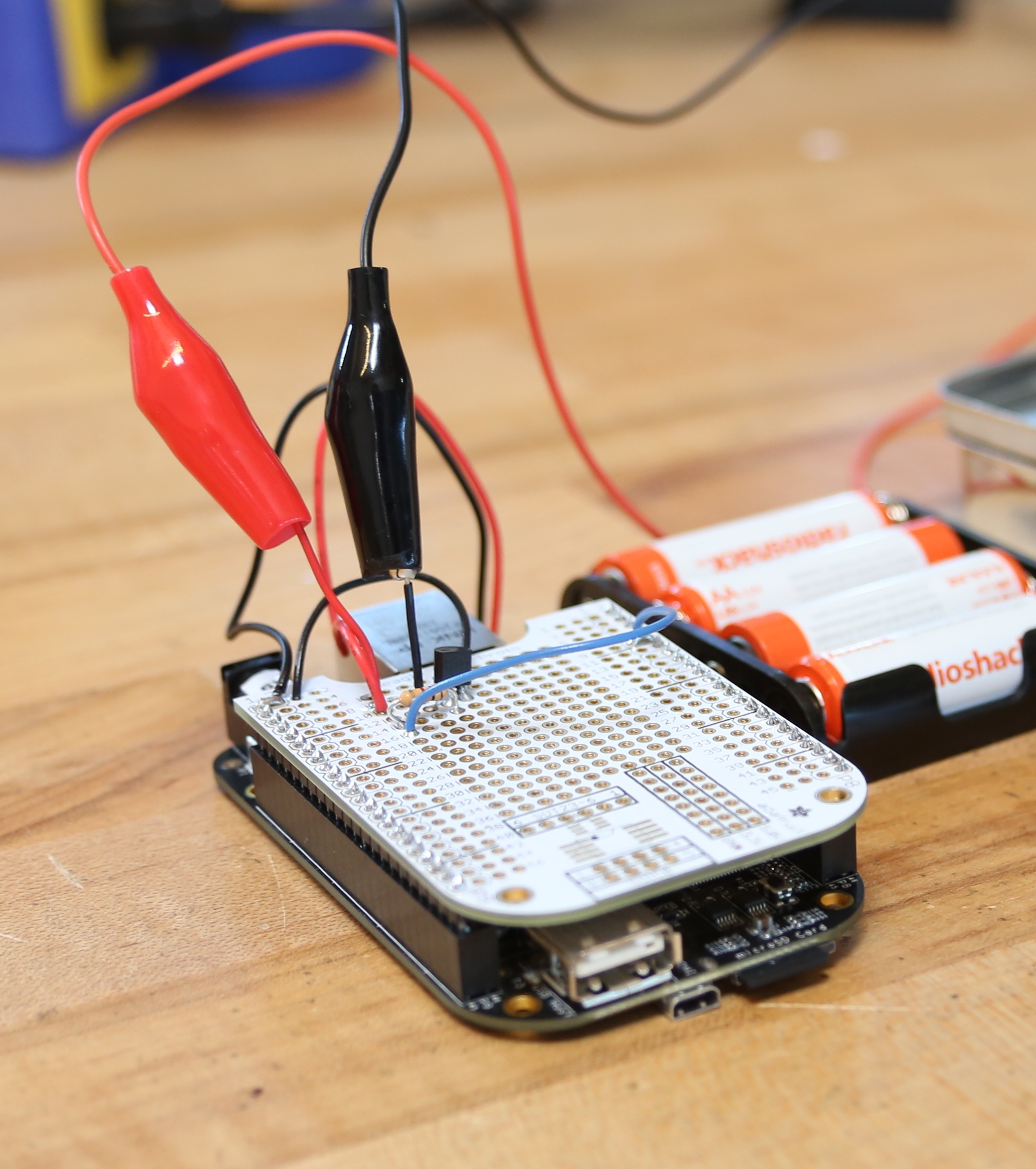
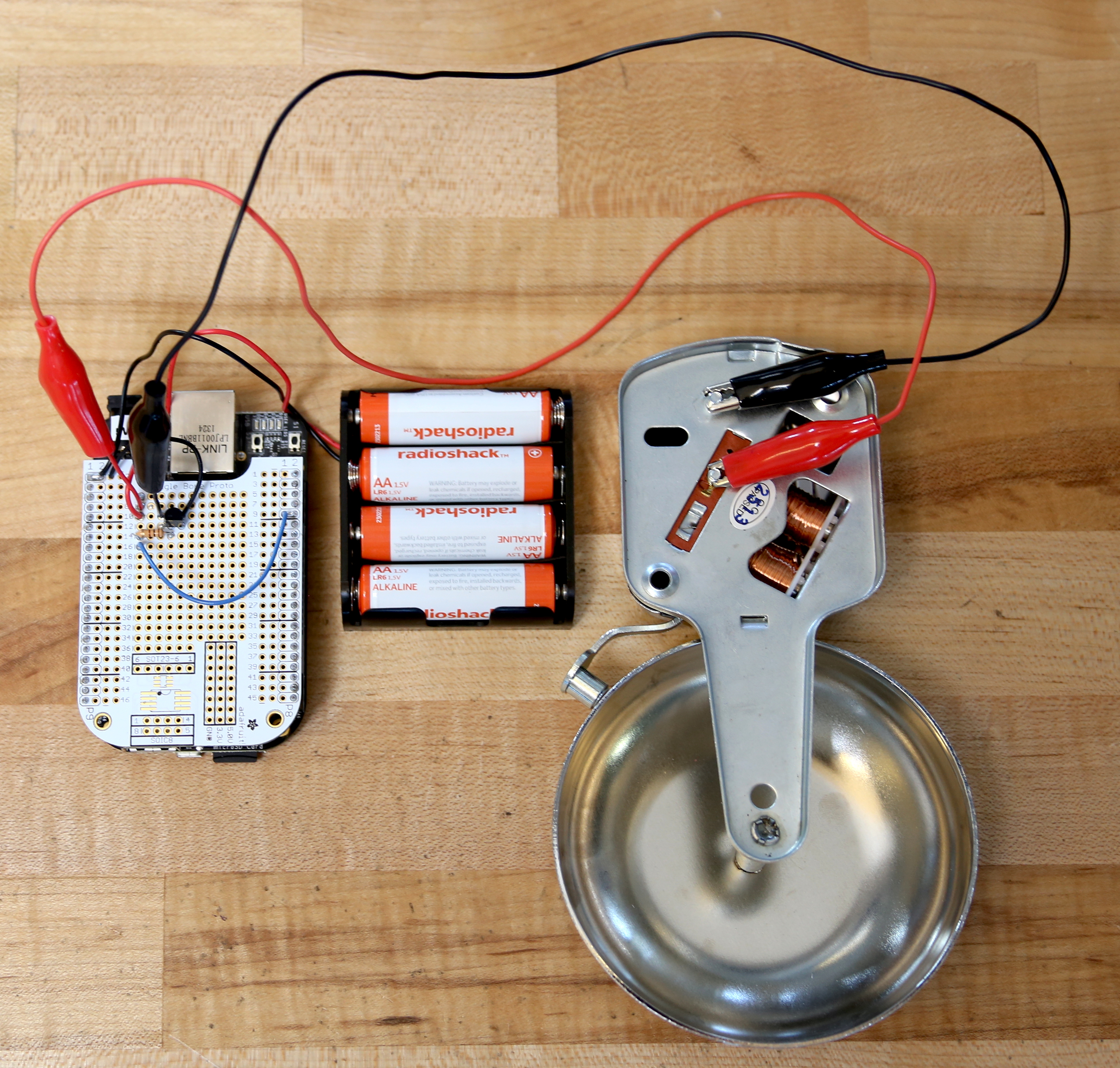
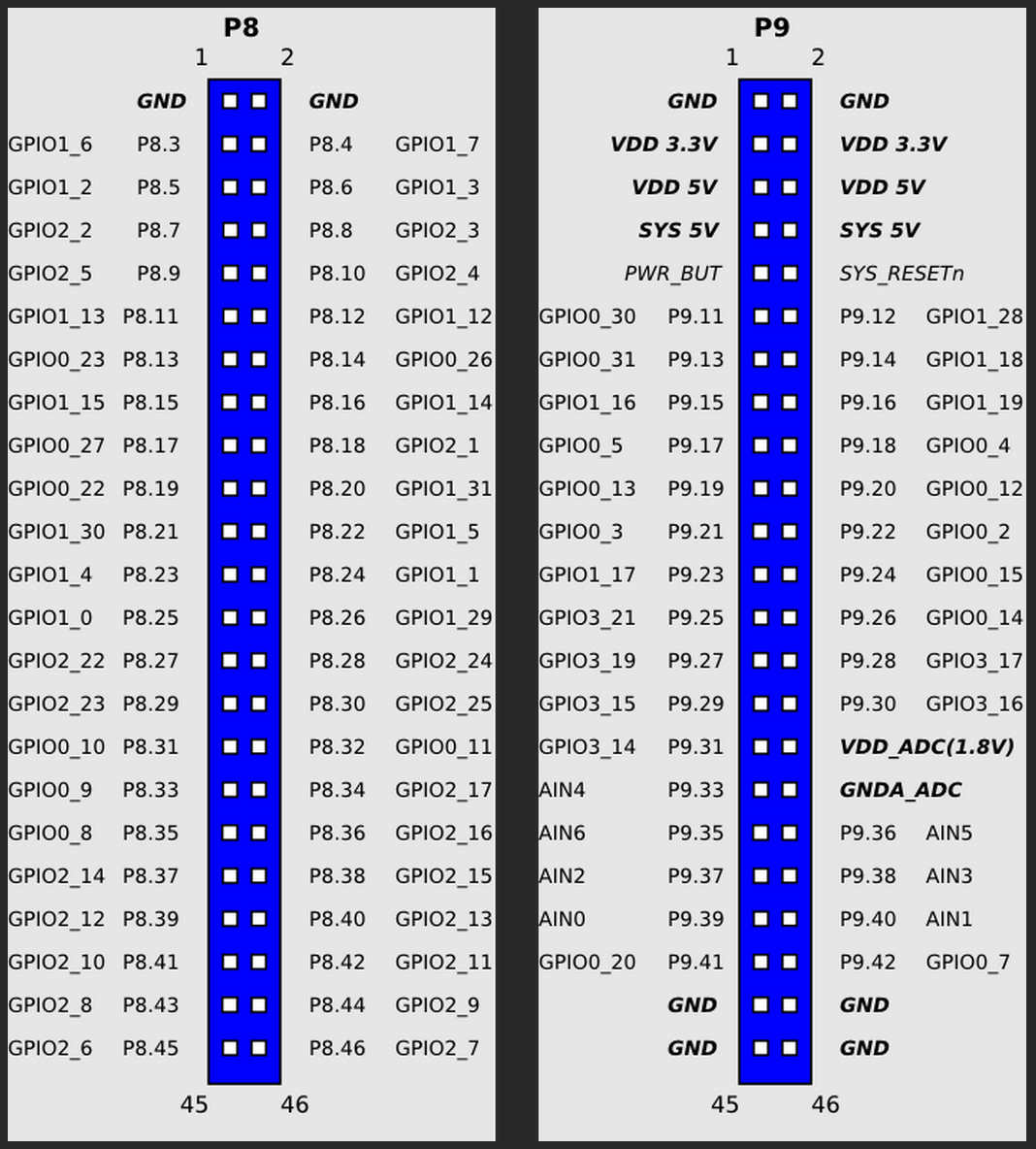
Solder the circuit illustrated earlier into the BBB protocape. Make sure to solder the transistor's base to pin 'P8_10' as shown in the diagram. To connect the electric bell to the protocape, I soldered two wires into the board. Alligator clips connect the wires to two small metal nubs on the back of the electric bell.
Powering the BeagleBoneBlack
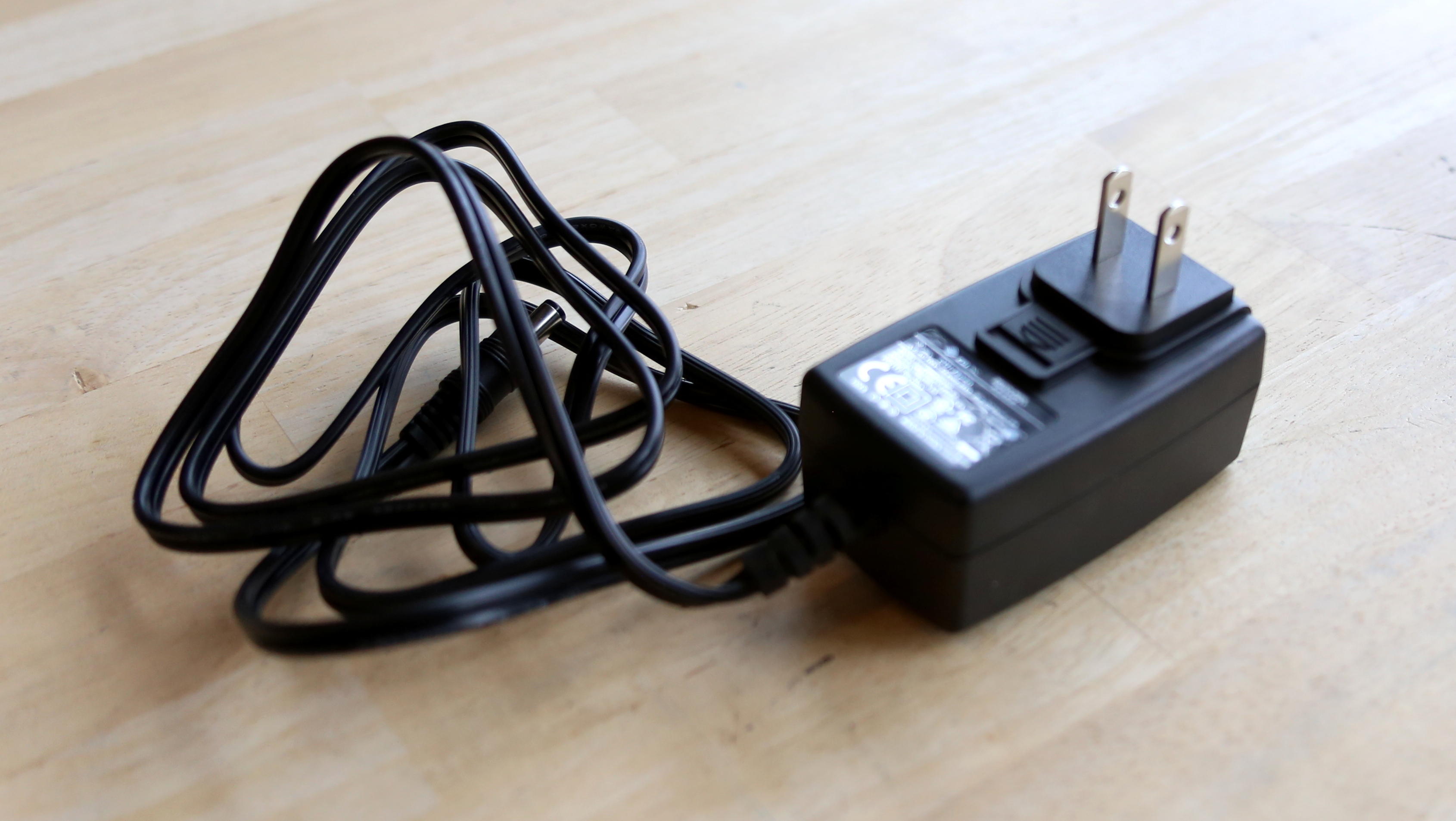
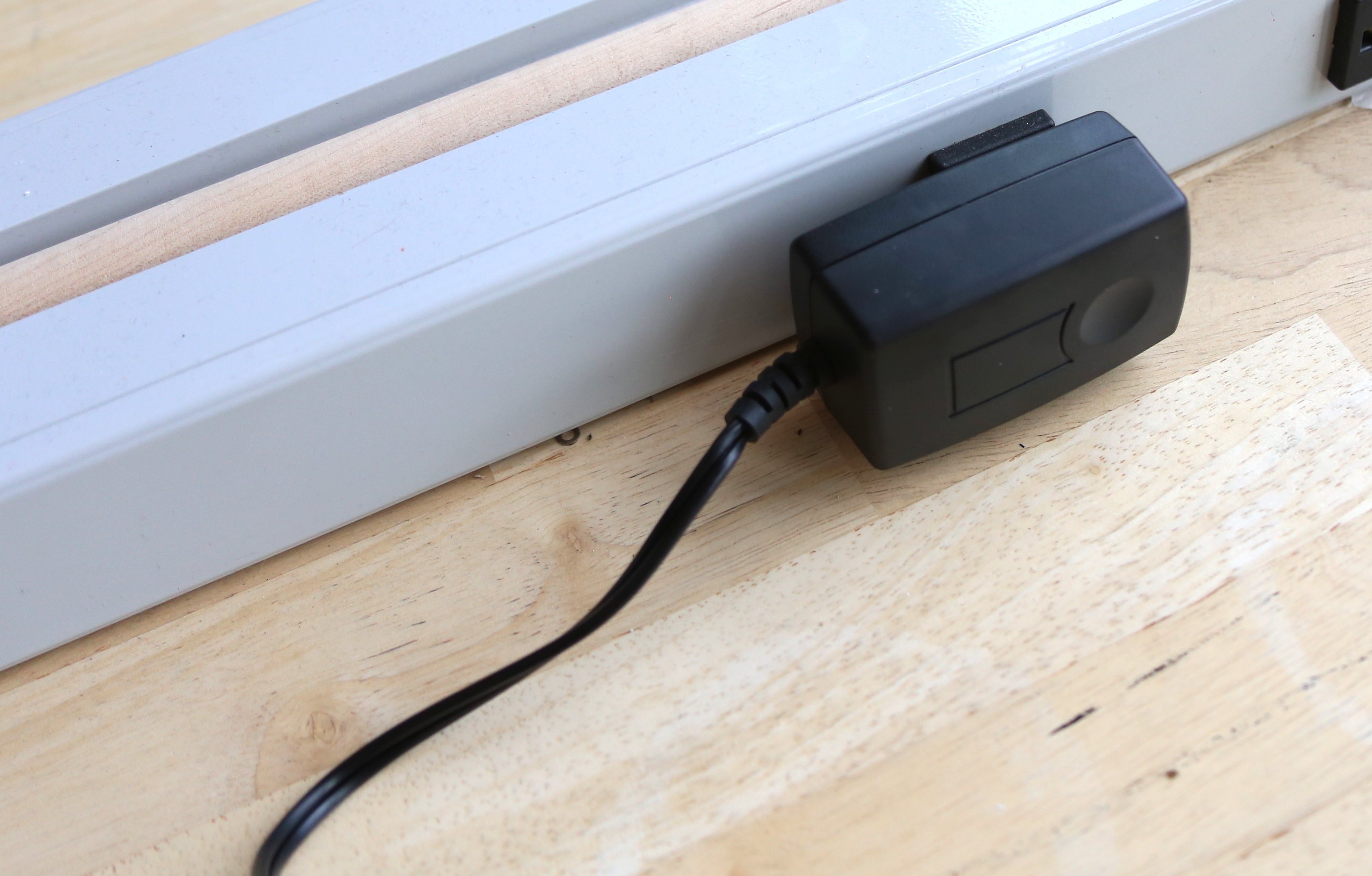
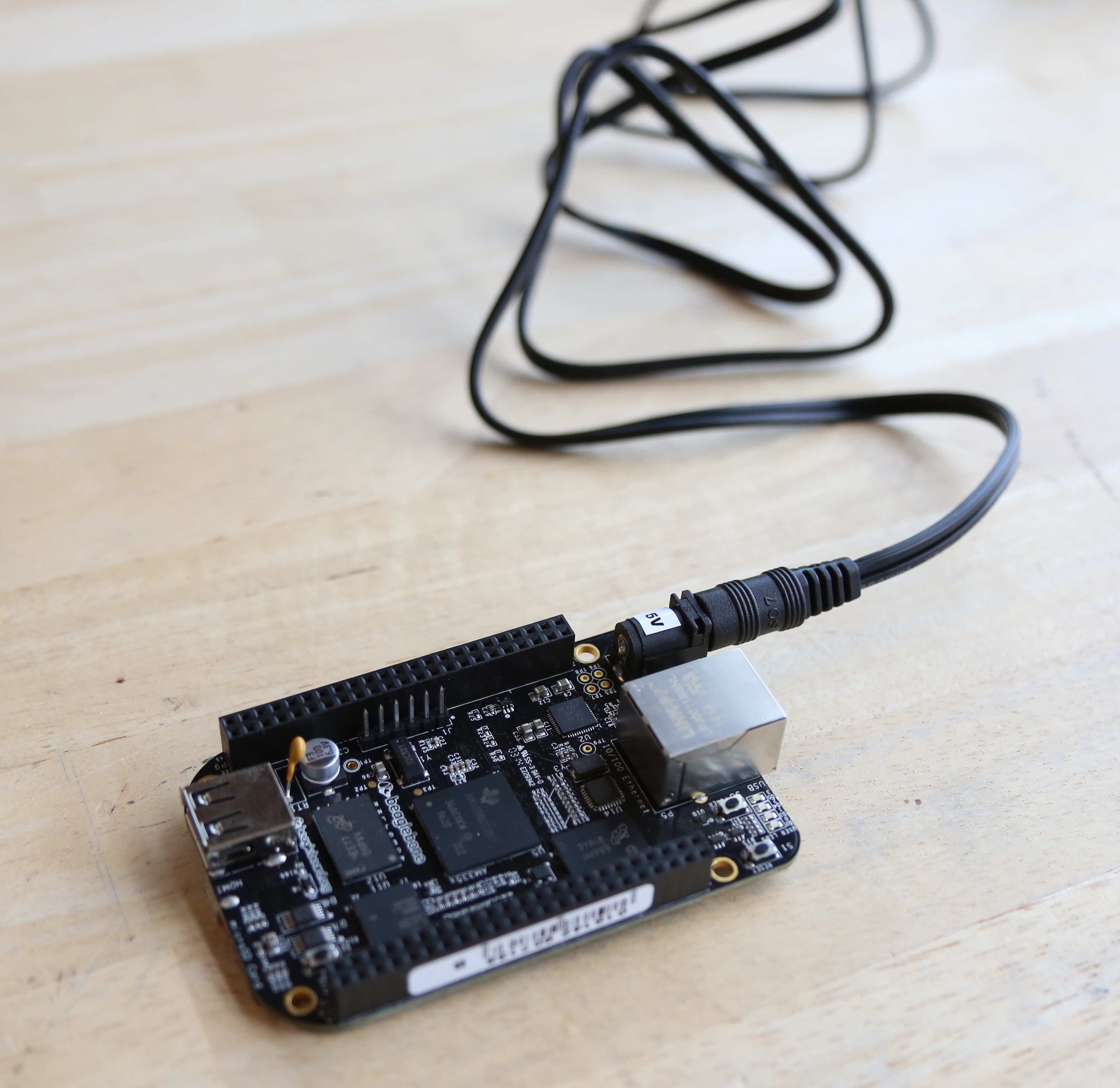
Power the BBB with a 5V 2-3A AC power adapter. Plug the barrel connector into the 5V barrel socket on the BBB.
see section 7.2 at http://www.adafruit.com/datasheets/BBB_SRM.pdf
Cutting the Box
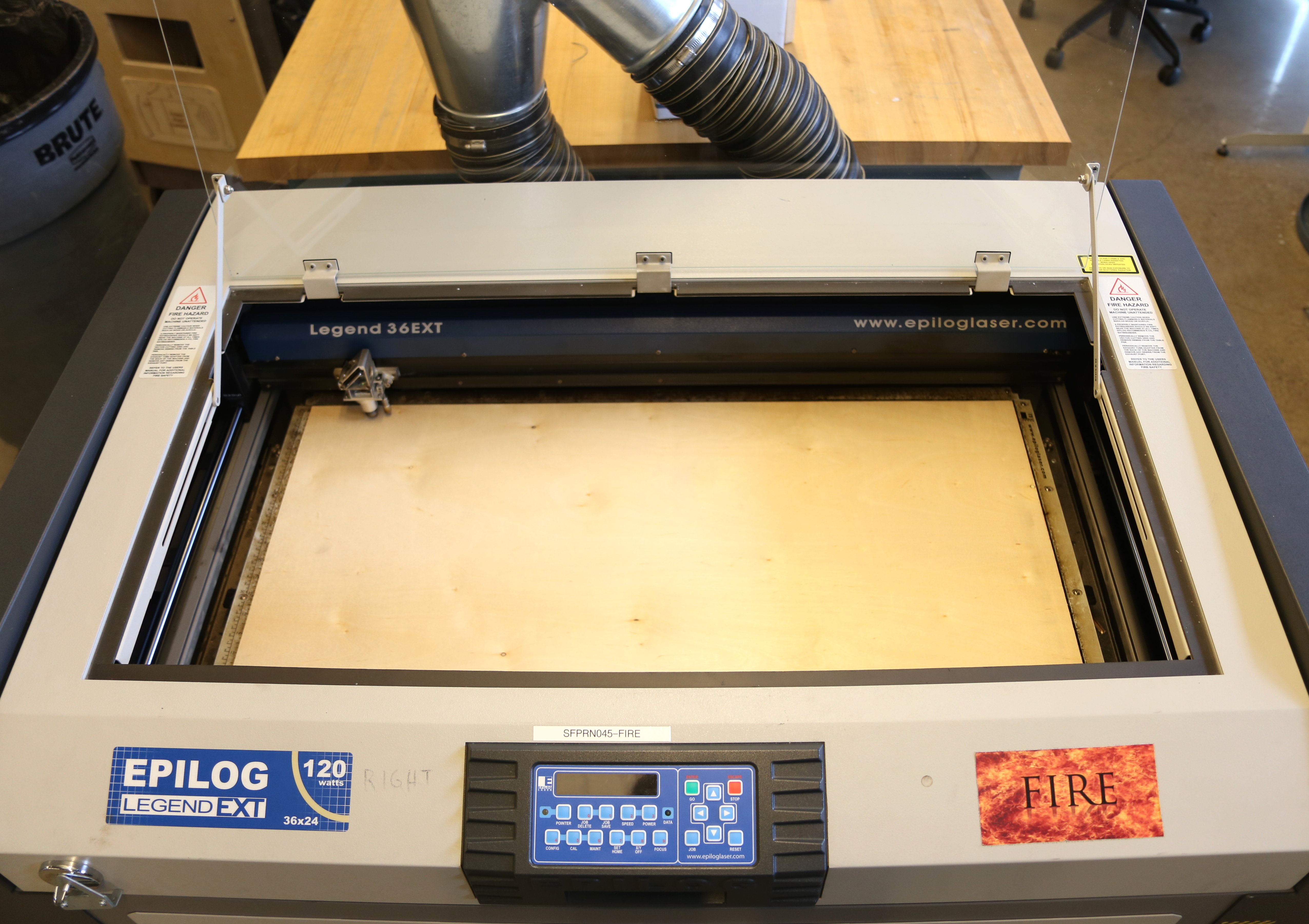
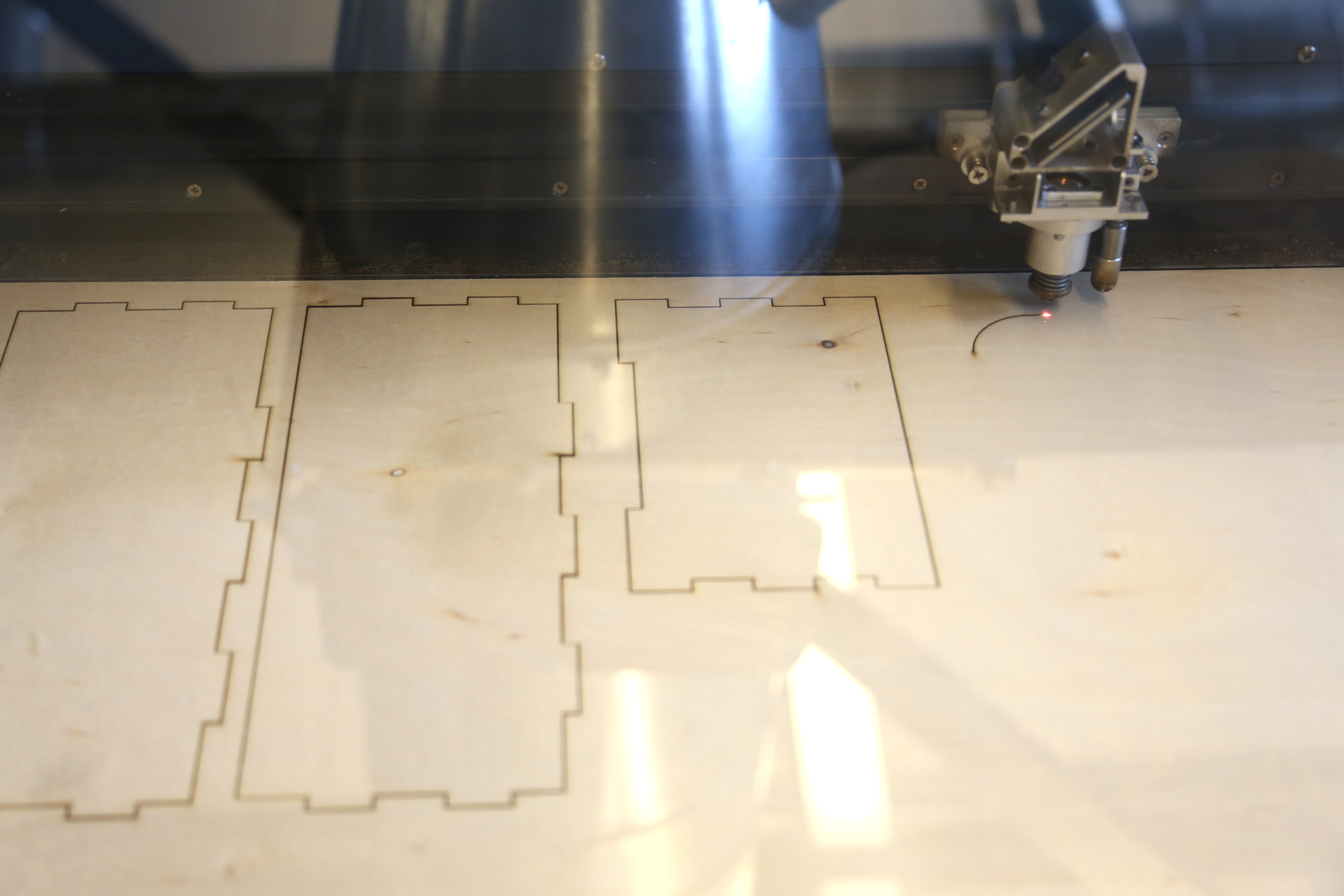
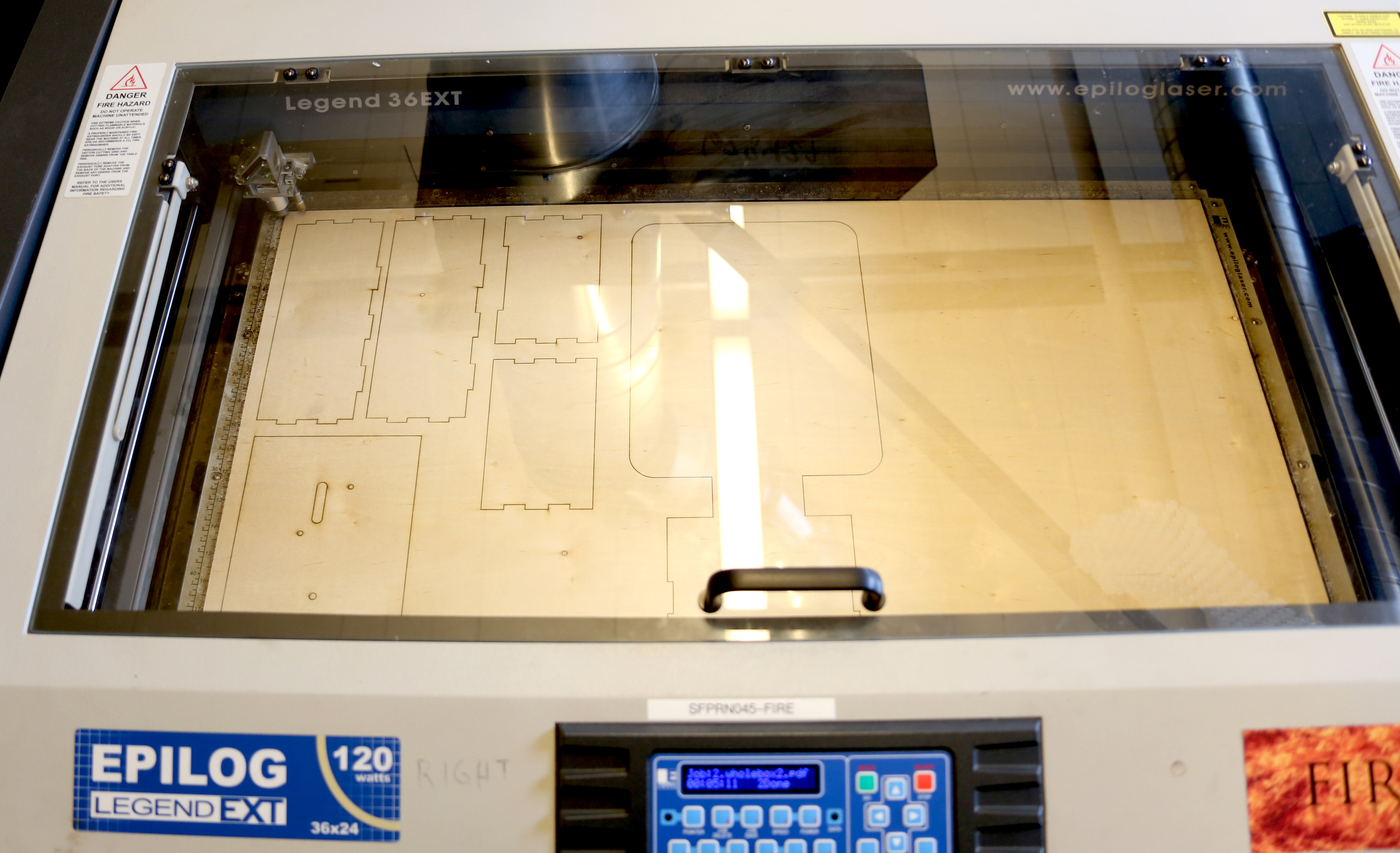
I built a custom enclosure for the electronics with our in-house laser cutter. To do this, I modeled what I wanted the box to look like in Inventor, converted the pieces to pdf files, and brought them into Abode Illustrator to be printed on an Epilogue Laser Cutter. However, it is also just as easy to use a pre-made box as an enclosure.
I have included pictures of the CAD model and pdfs of each Inventor part if you wish to recreate this exact box.
Mounting the Bell
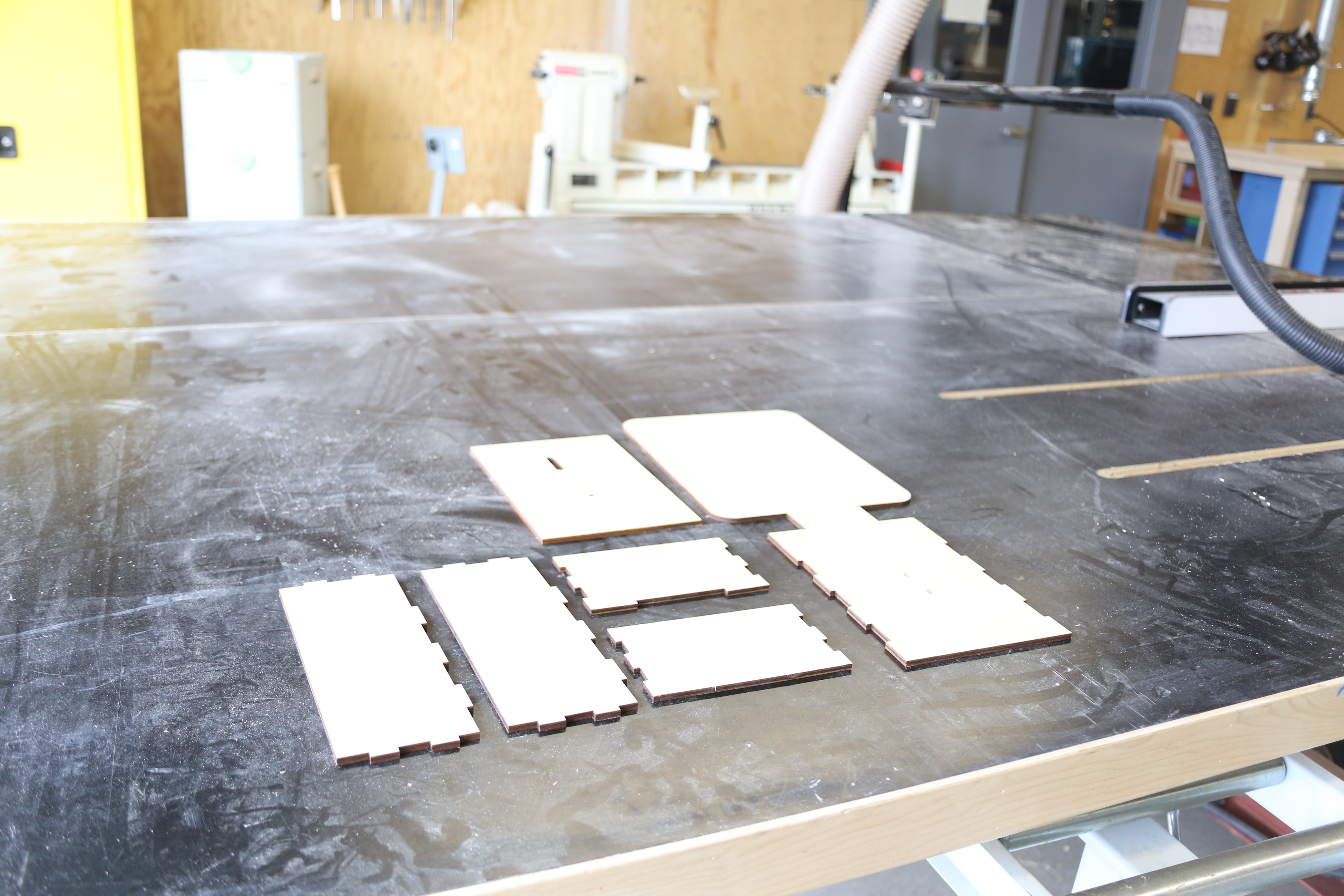
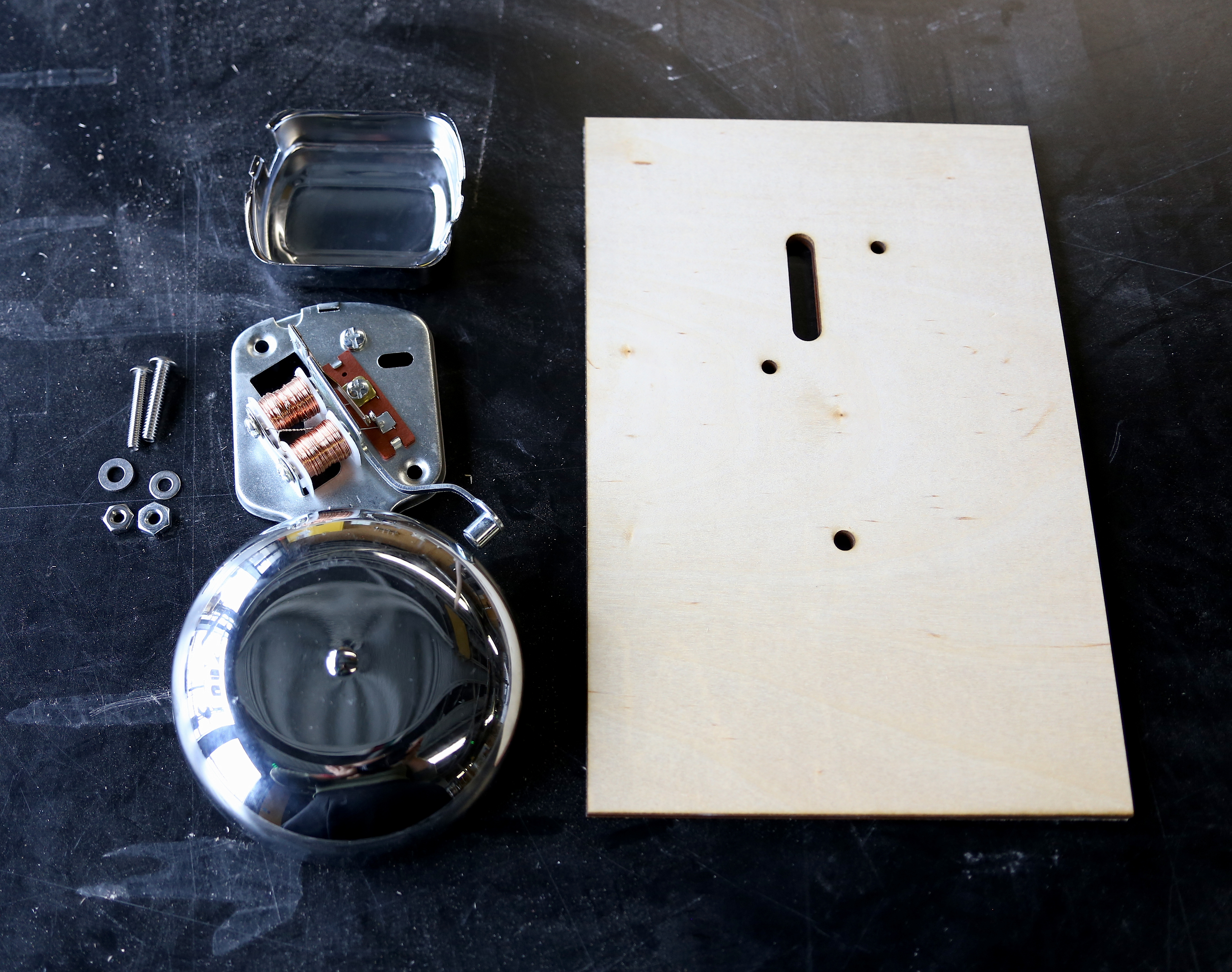
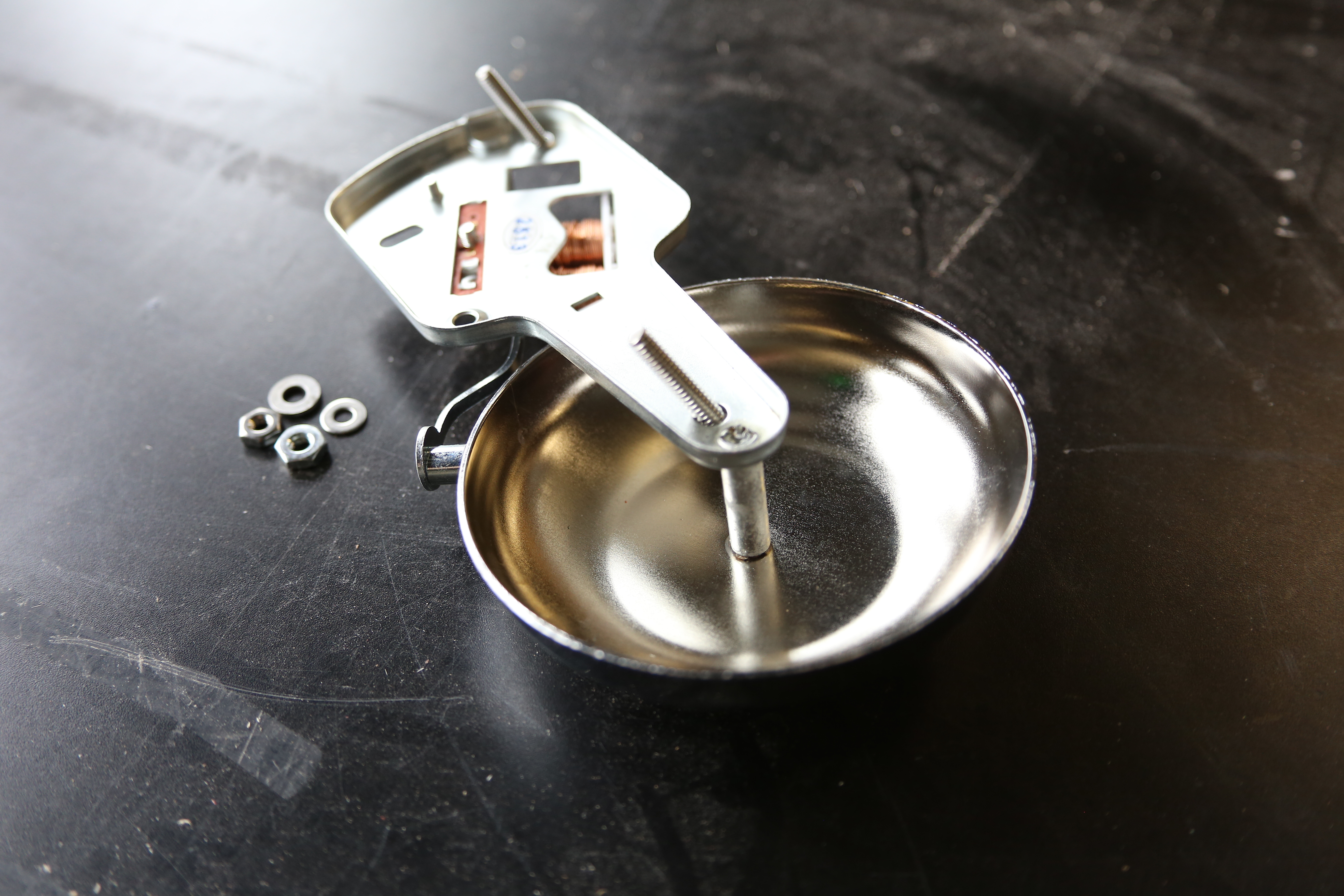
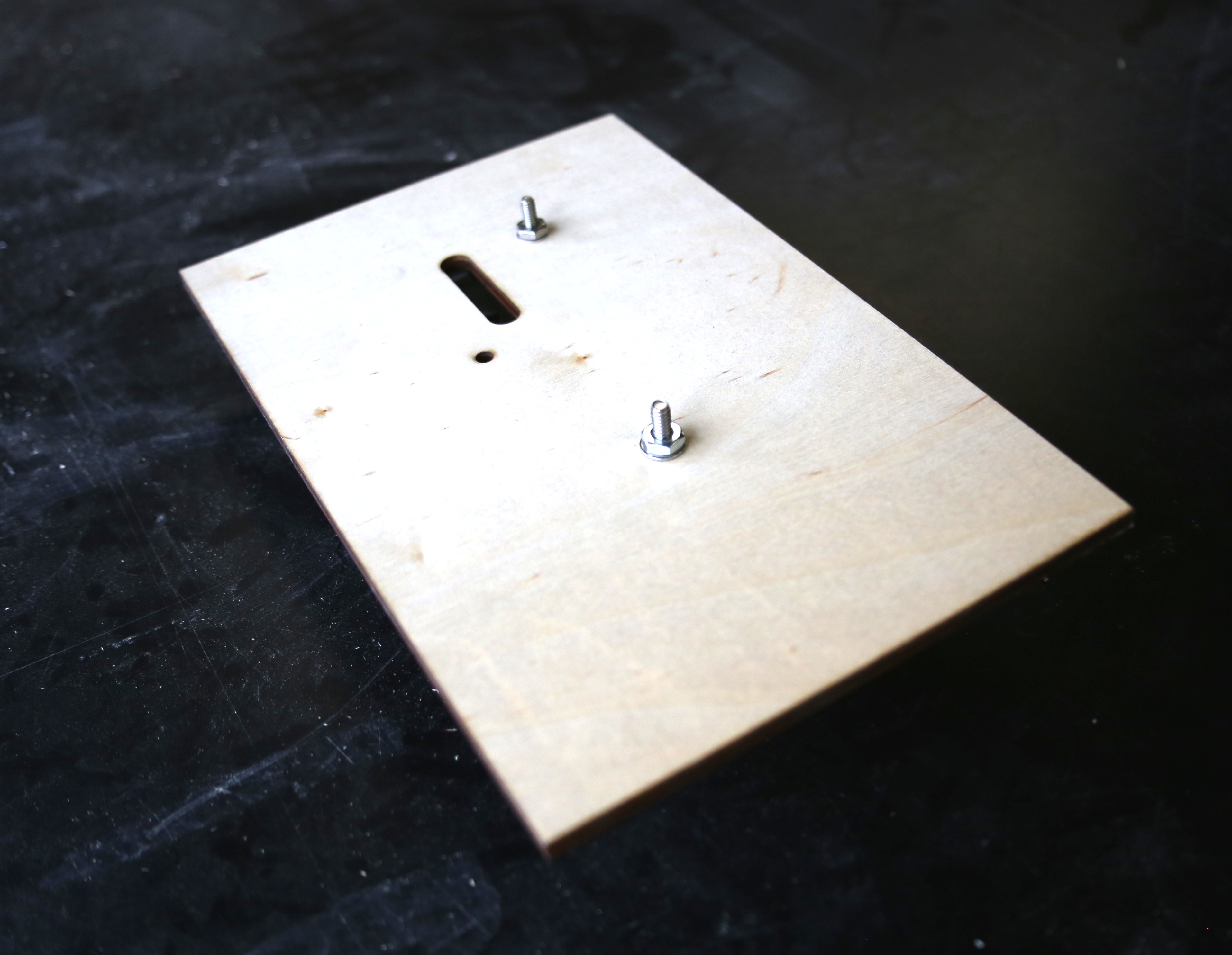
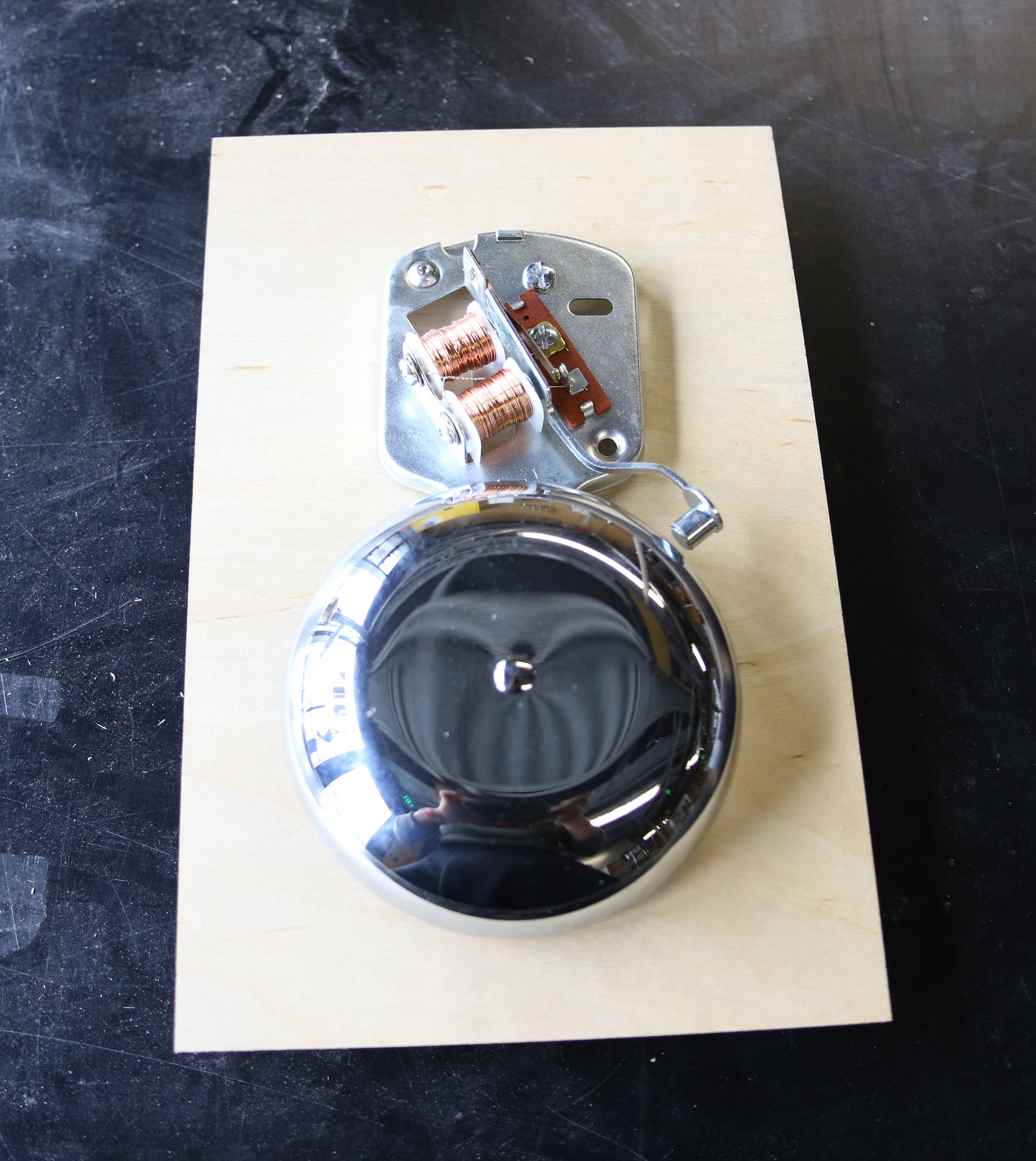
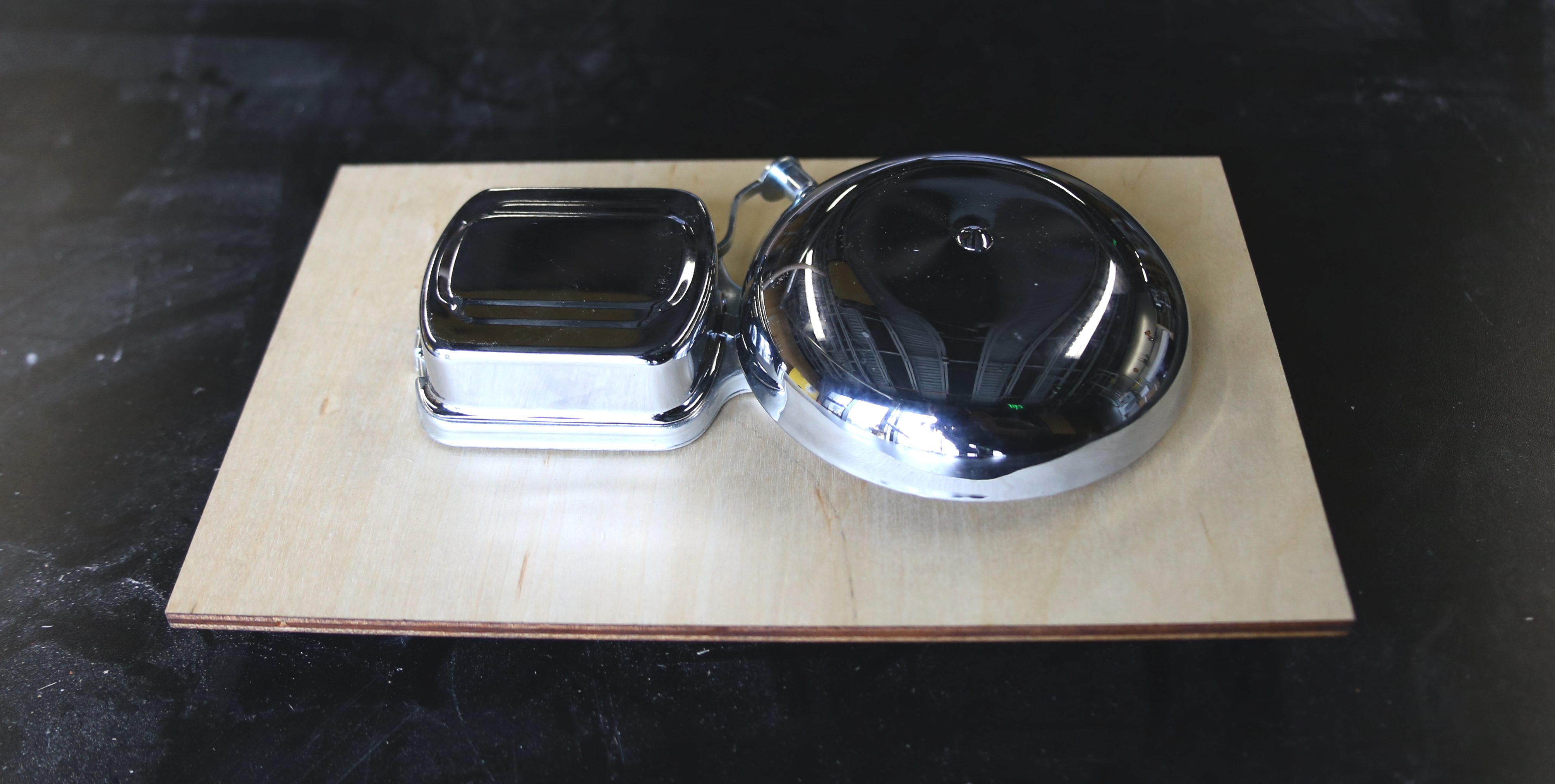
The front face of the box is designed as a mount for the bell. The electric bell comes with three holes on its back plate for this purpose. Remove the face plate over the bell's electromagnet to access it top mount hole. Thread the screws through the top and bottom holes, as shown in the image. I only ended up using two of the three holes I cut into the board. The large slot in the board slides over two metal nubs on the bell that will be wired to the board. It's important that you can still access these nubs once the bell is mounted. Use a pair of washers and nuts to screw the bell to the board. Depending on the type of bell you buy,the hole spacing may be different, so use a pair of calipers or a ruler to take measurements. The bell should now be mounted firmly to the board. Replace the bell's metal casing to protect the electromagnet.
Gluing the Case
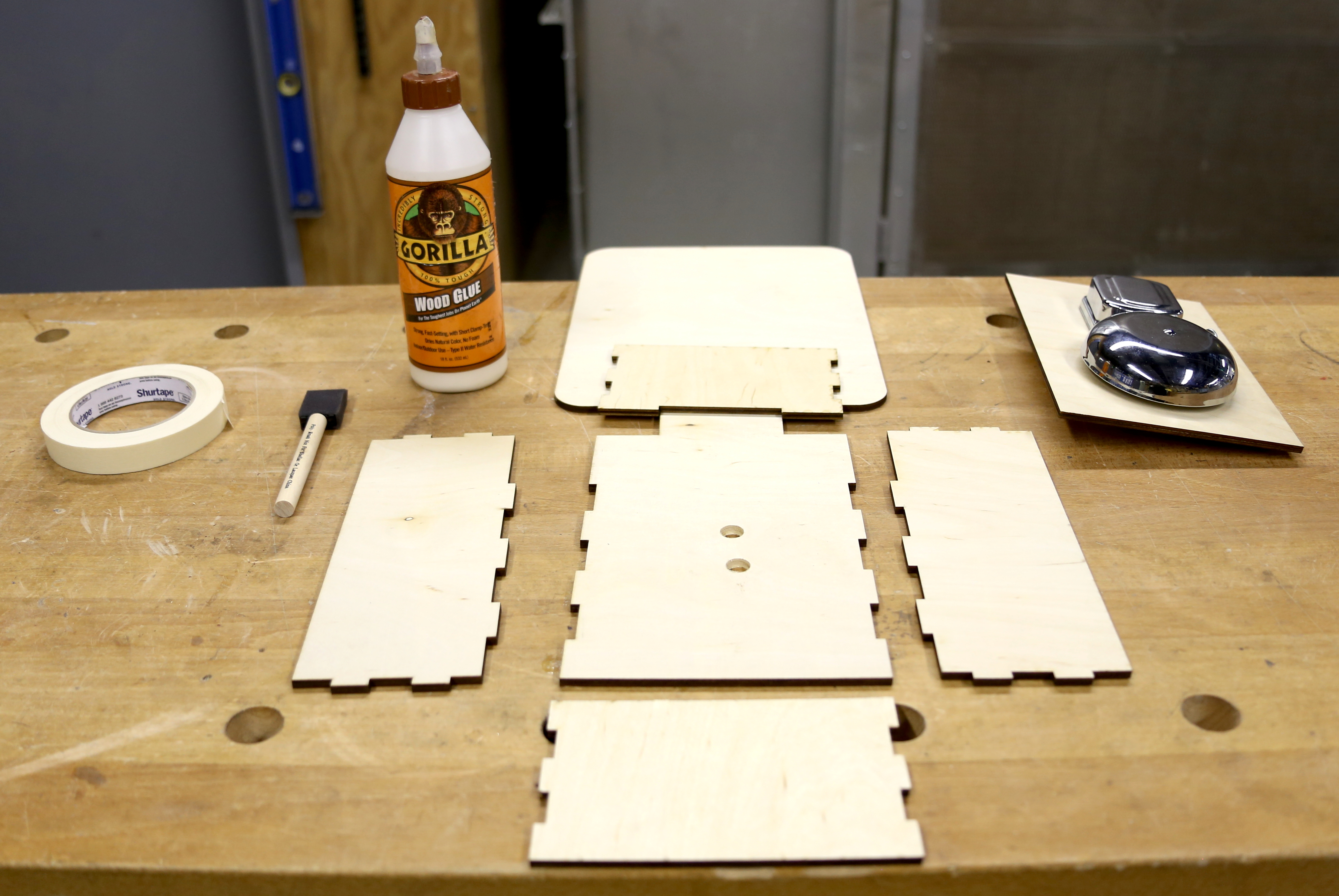
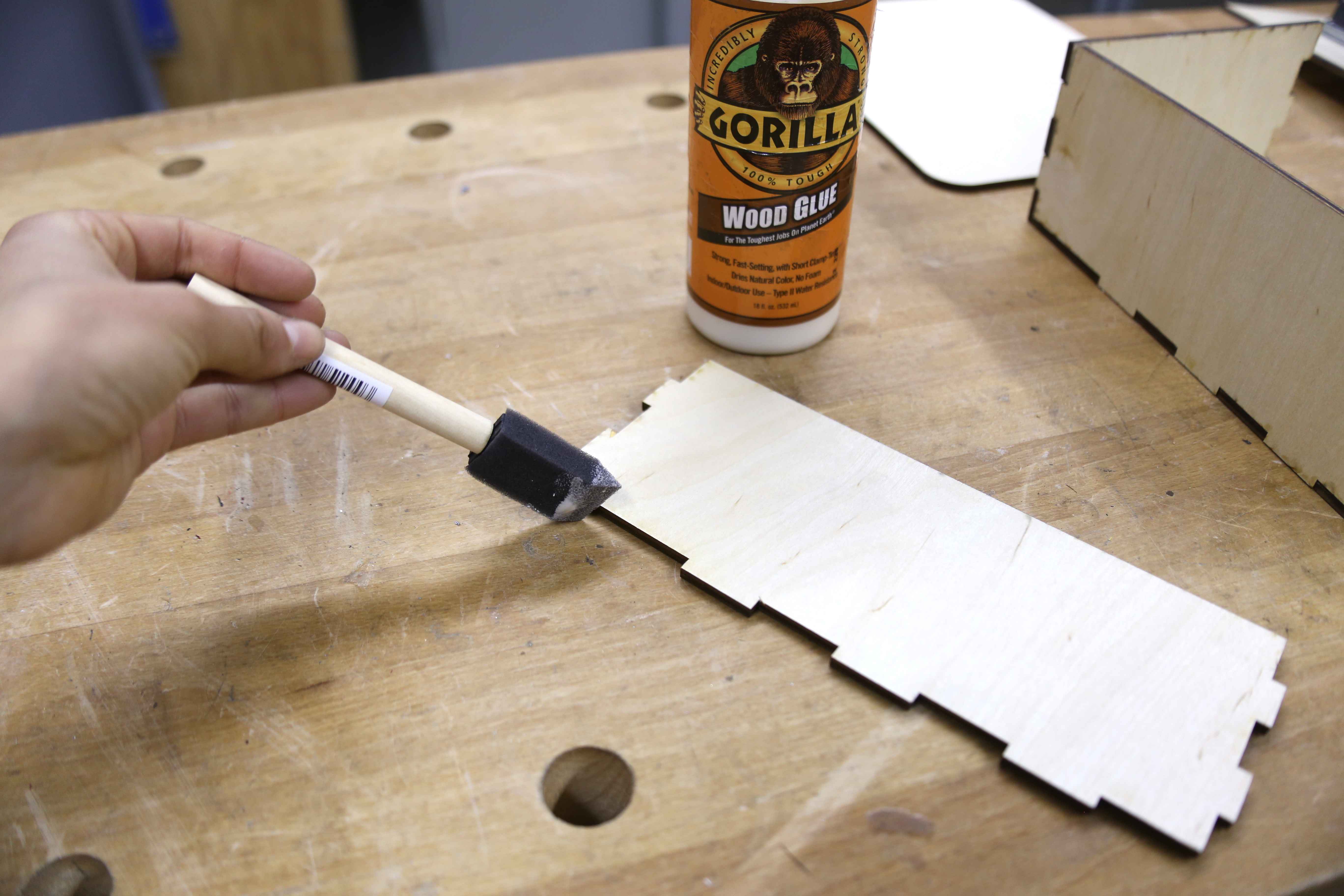
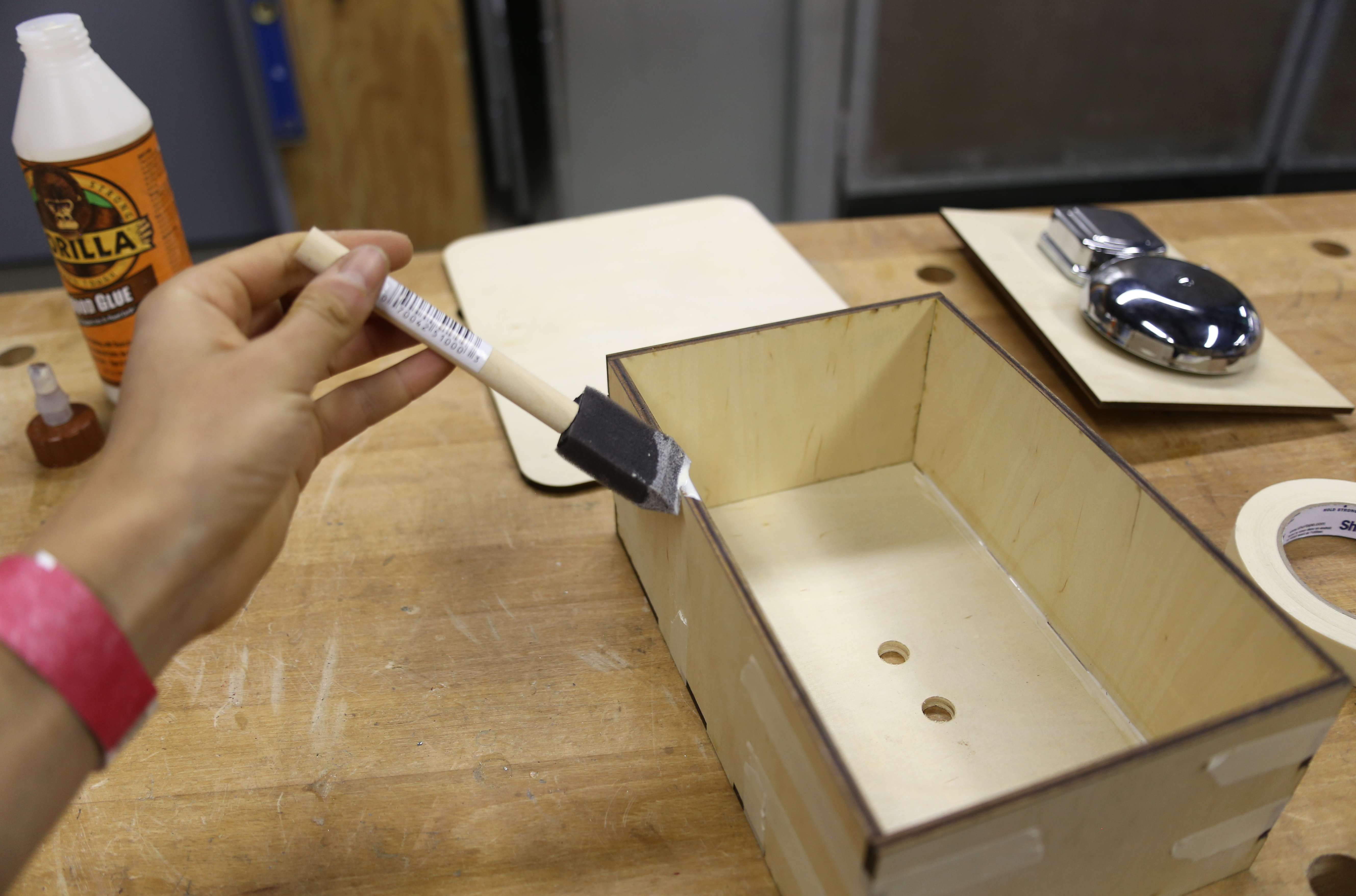
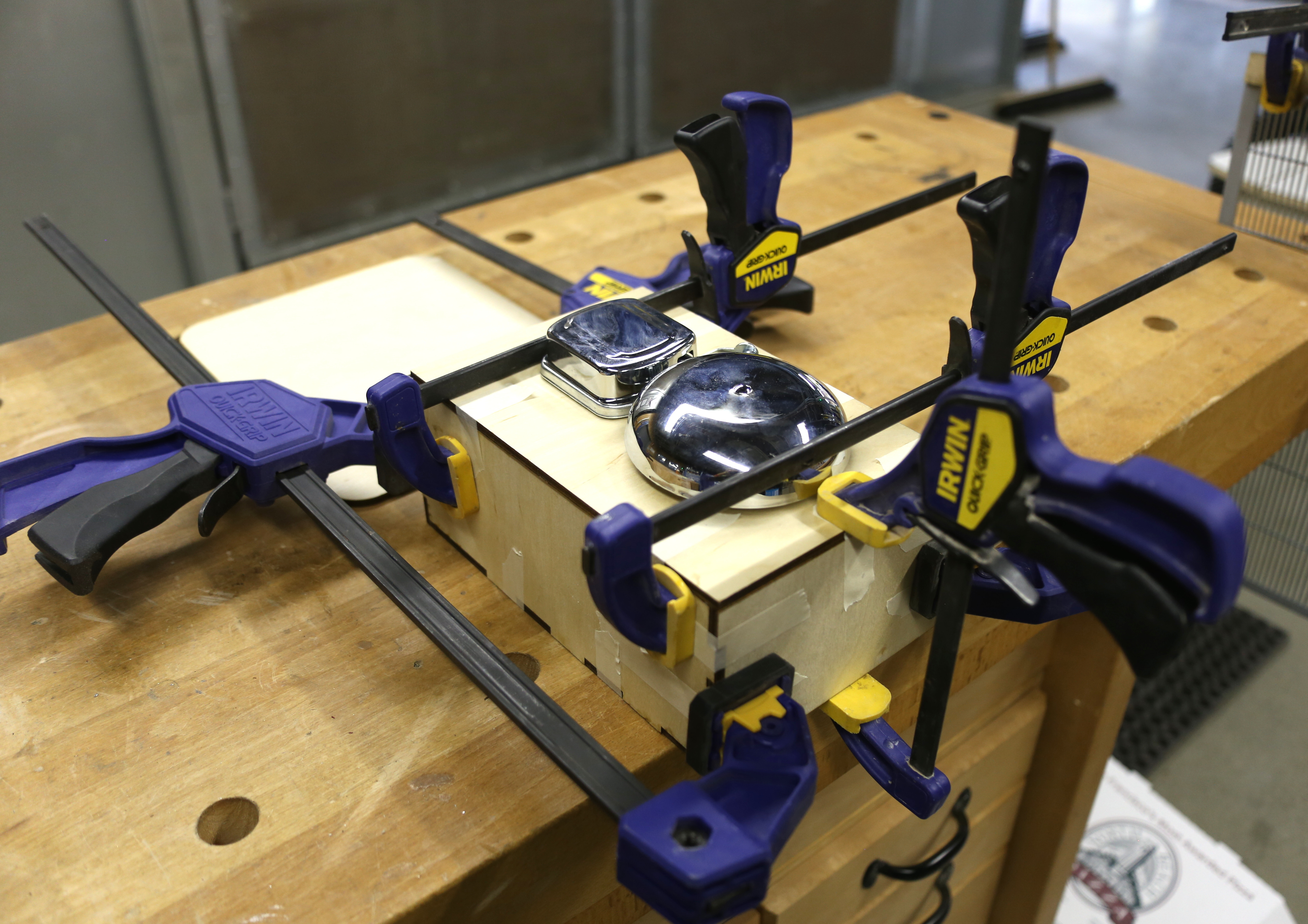
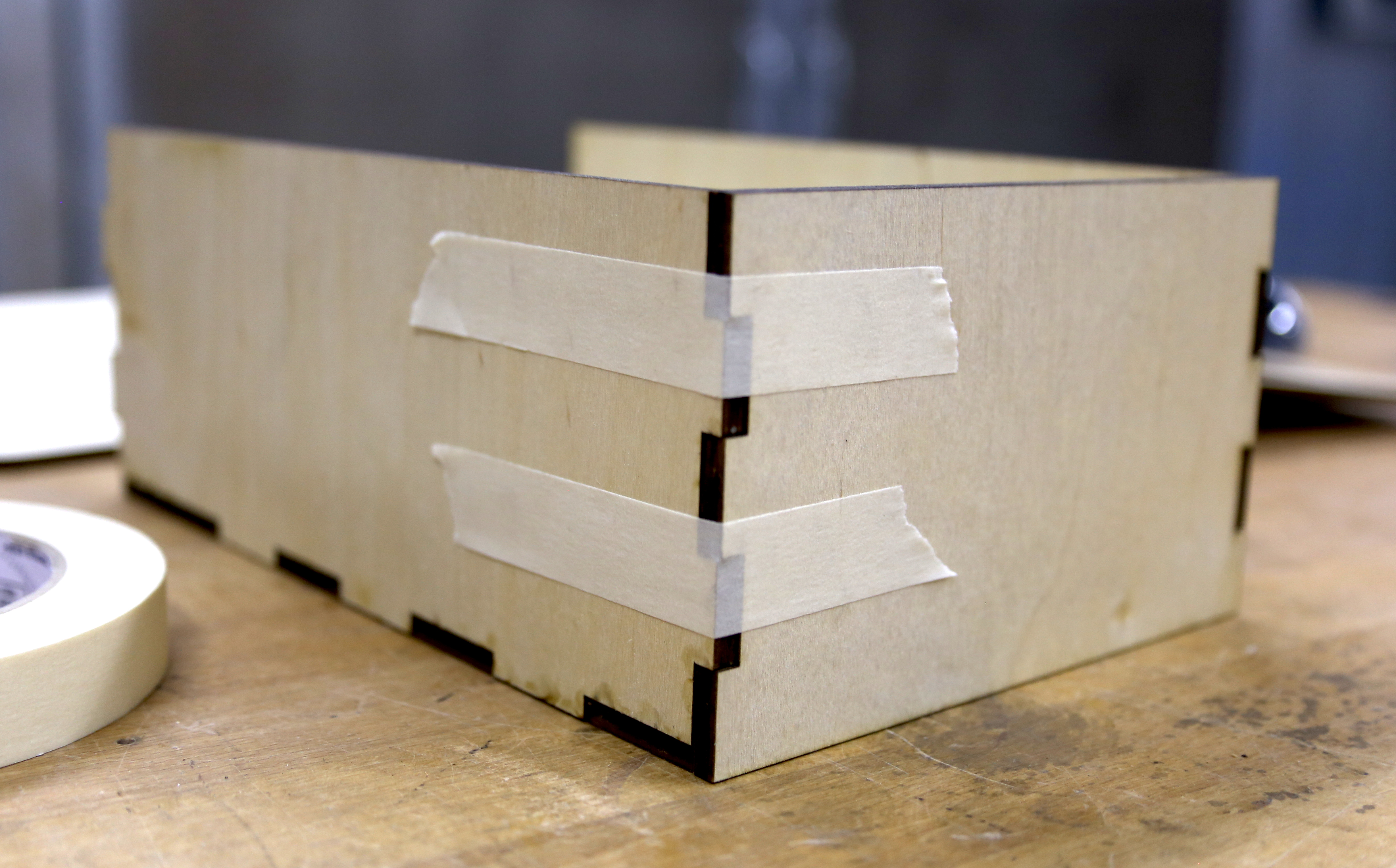
Paint the box's inner teeth and edges with wood glue, and apply masking tape to adjacent edges to hold them in place while drying. To be safe, I also clamped opposite walls together. Wait a minimum of 30 minutes for the box to dry.
Decoration
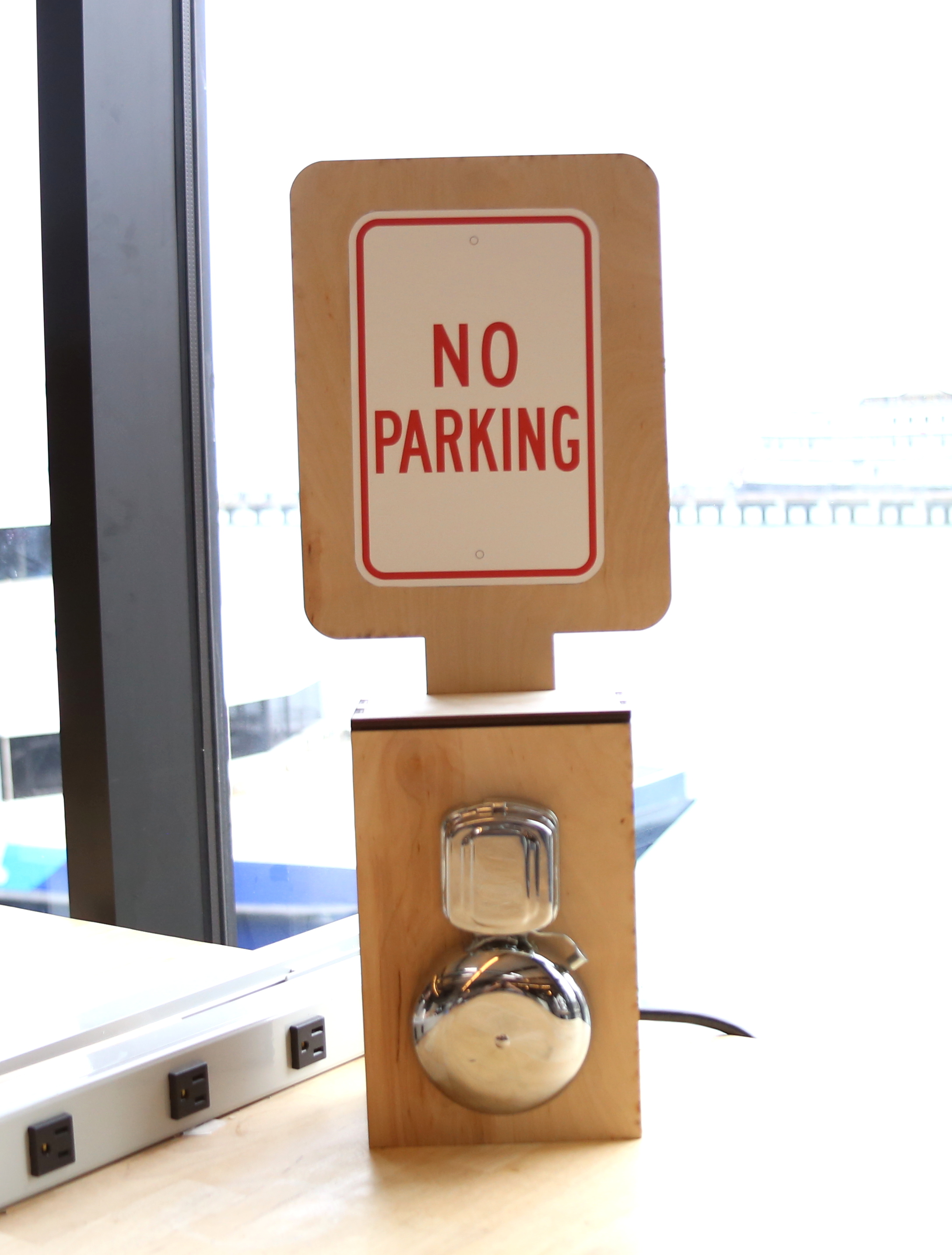
I designed the back panel of the box to look like a "no parking sign", and vinyl cut a sticker to be placed on this panel. You can easily buy 8.5" x 11" injet photo stick paper, print out a no parking sign, cut it out with an x acto knife, and stick it to this panel.
Putting It All Together
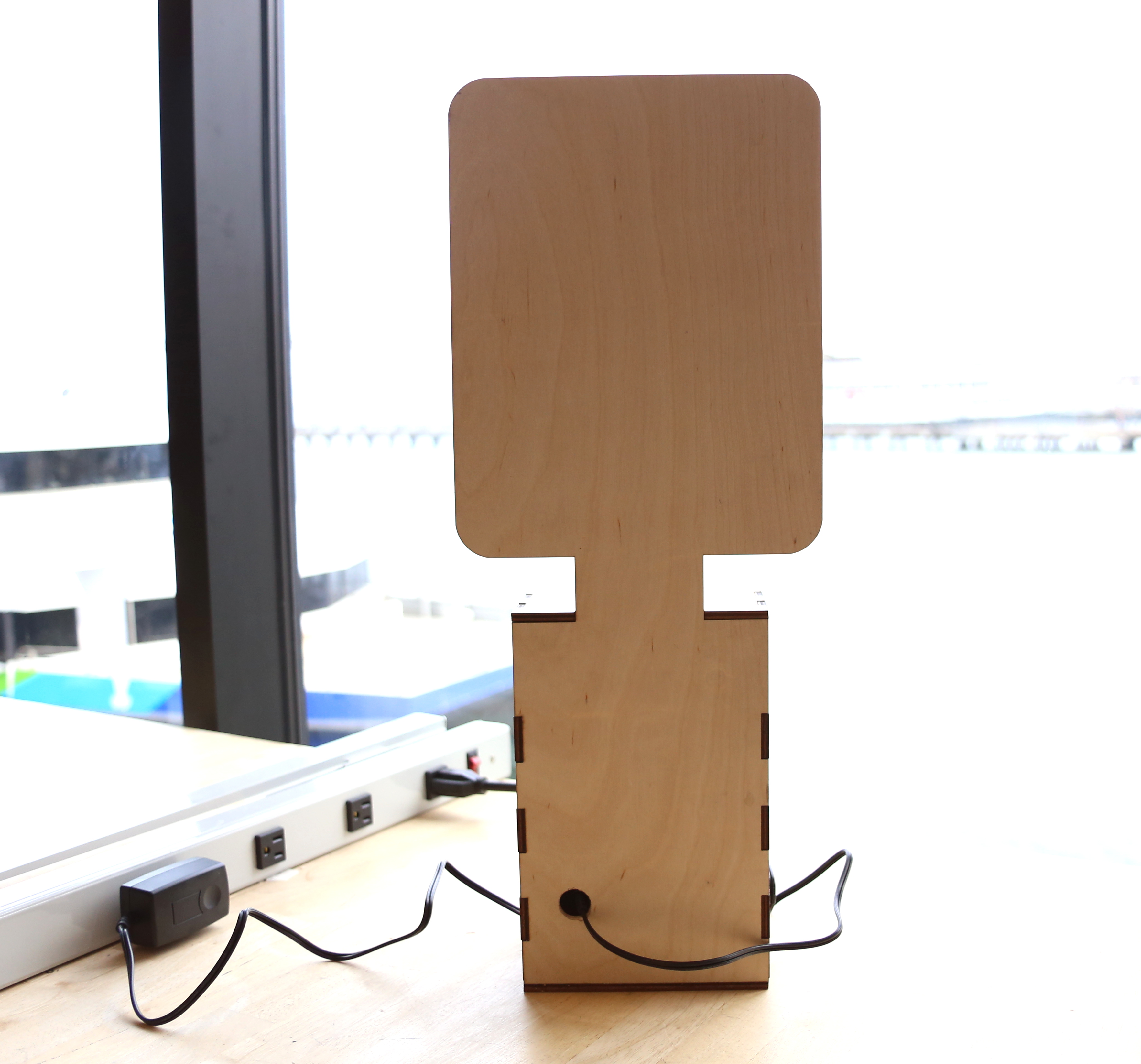
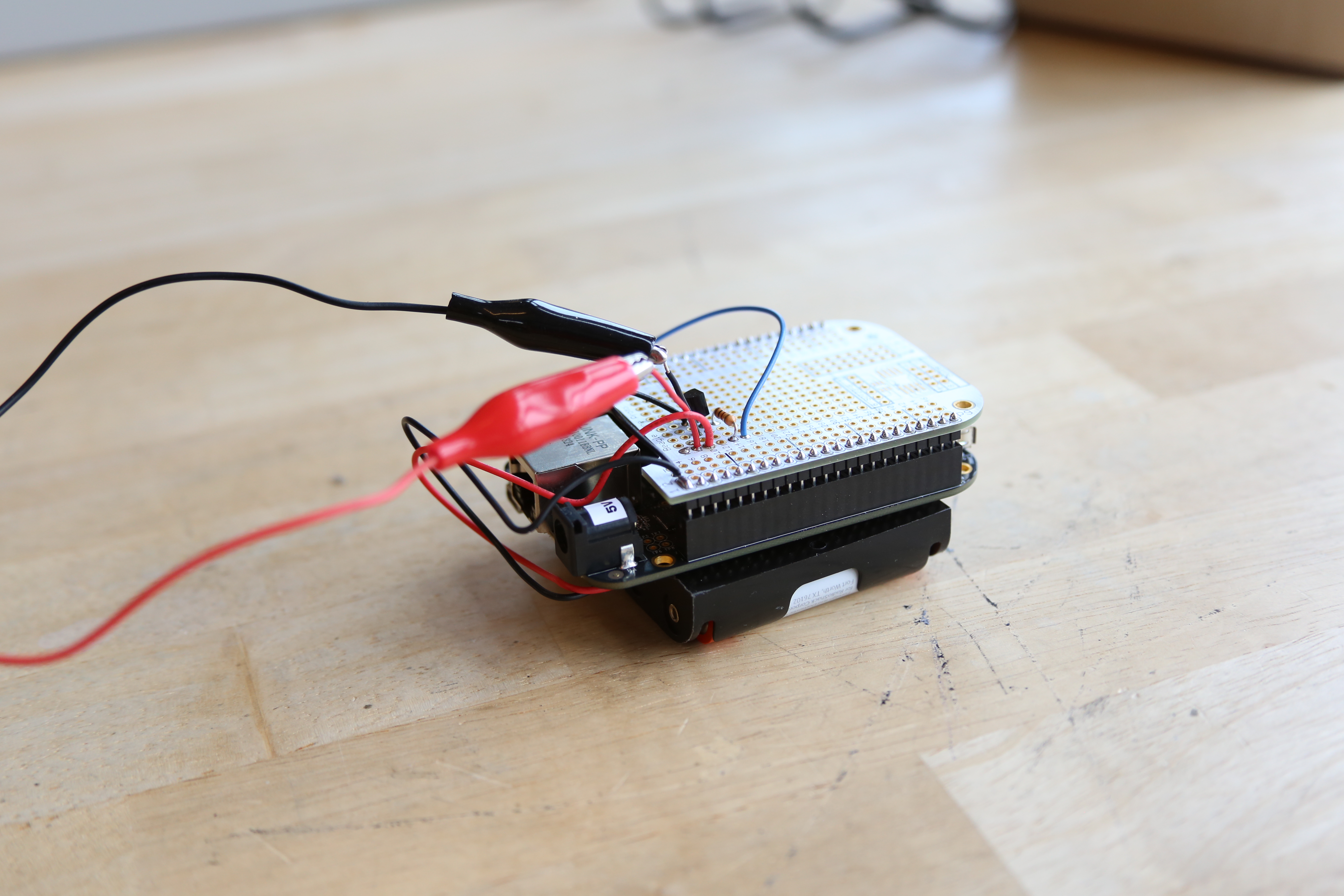
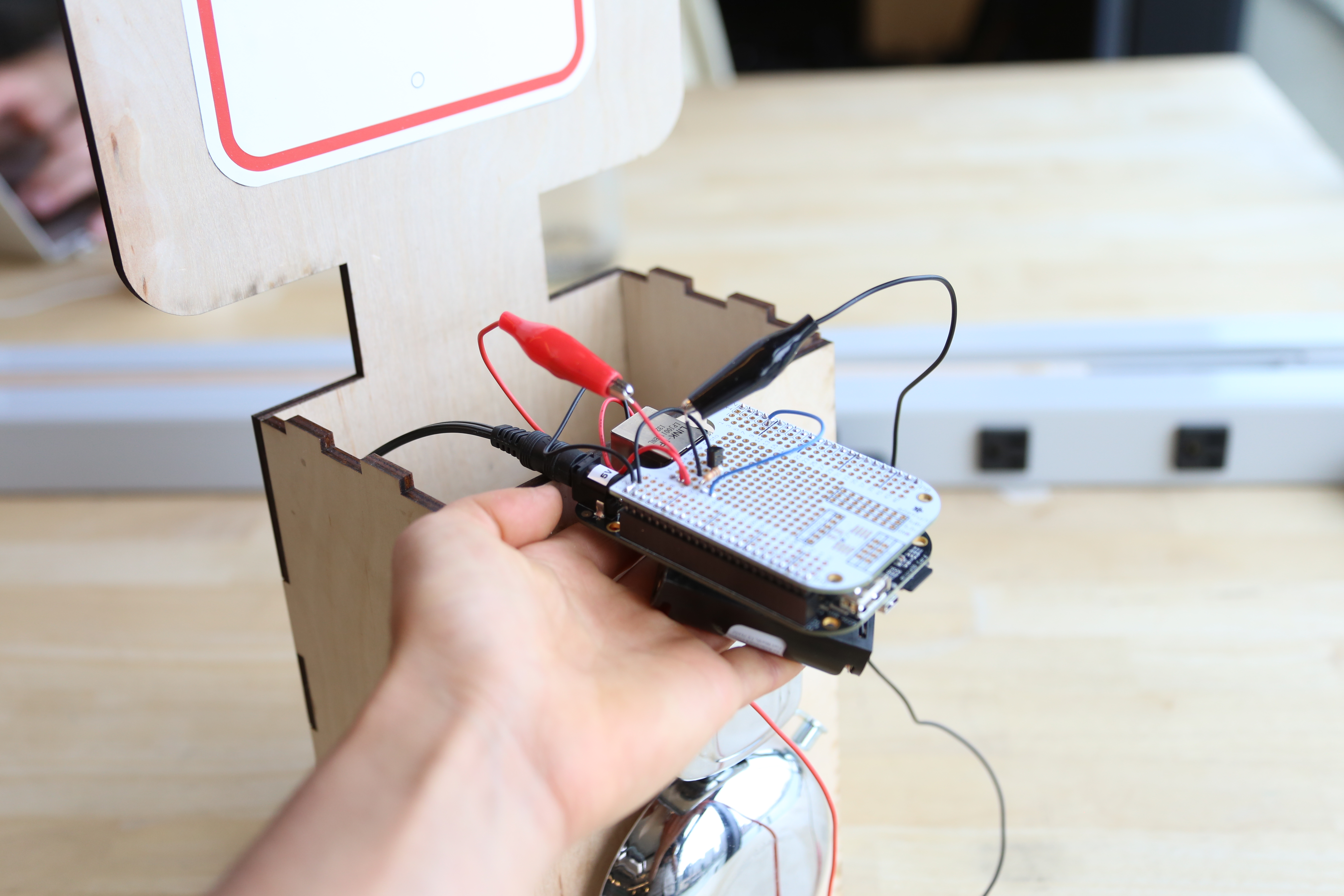
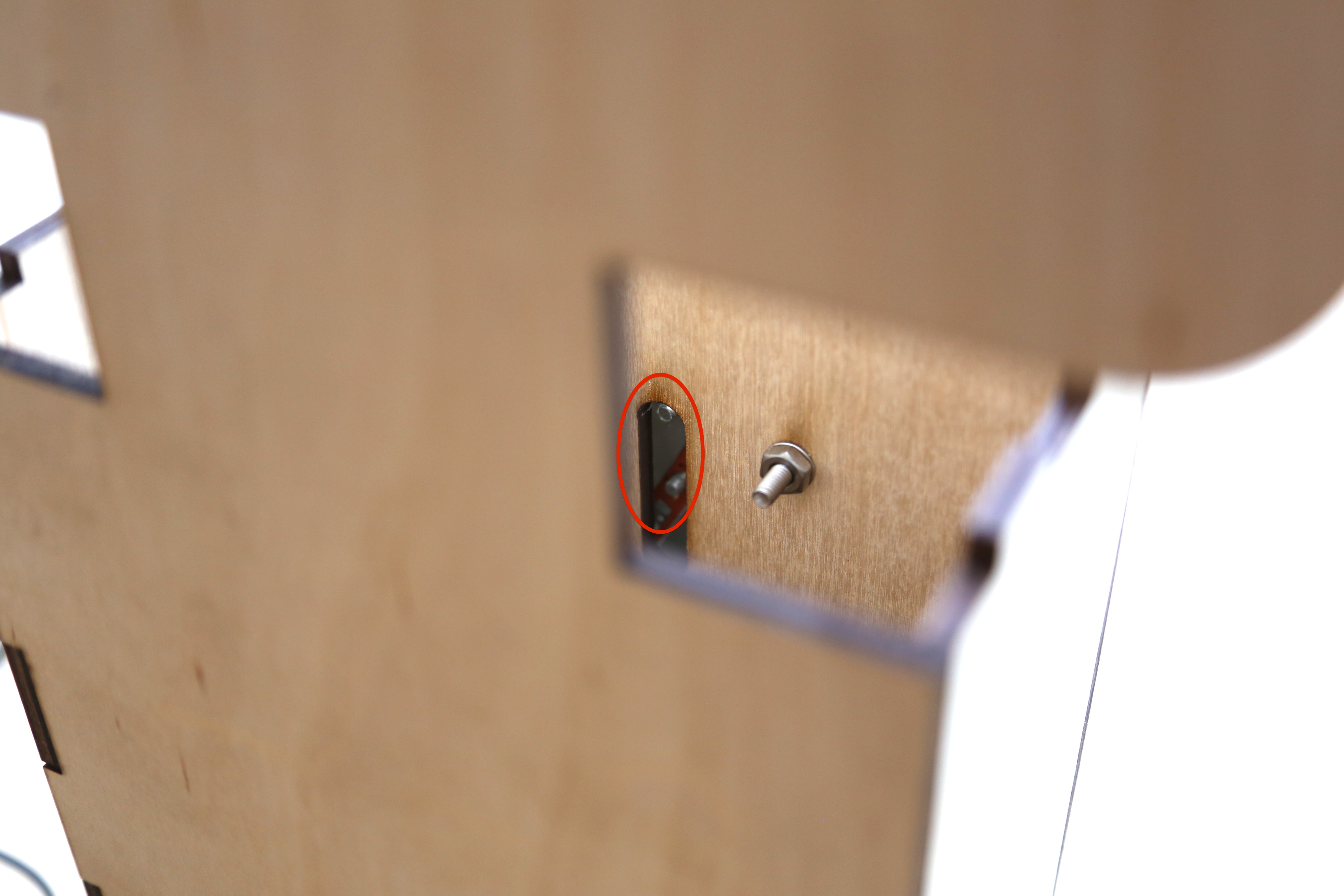
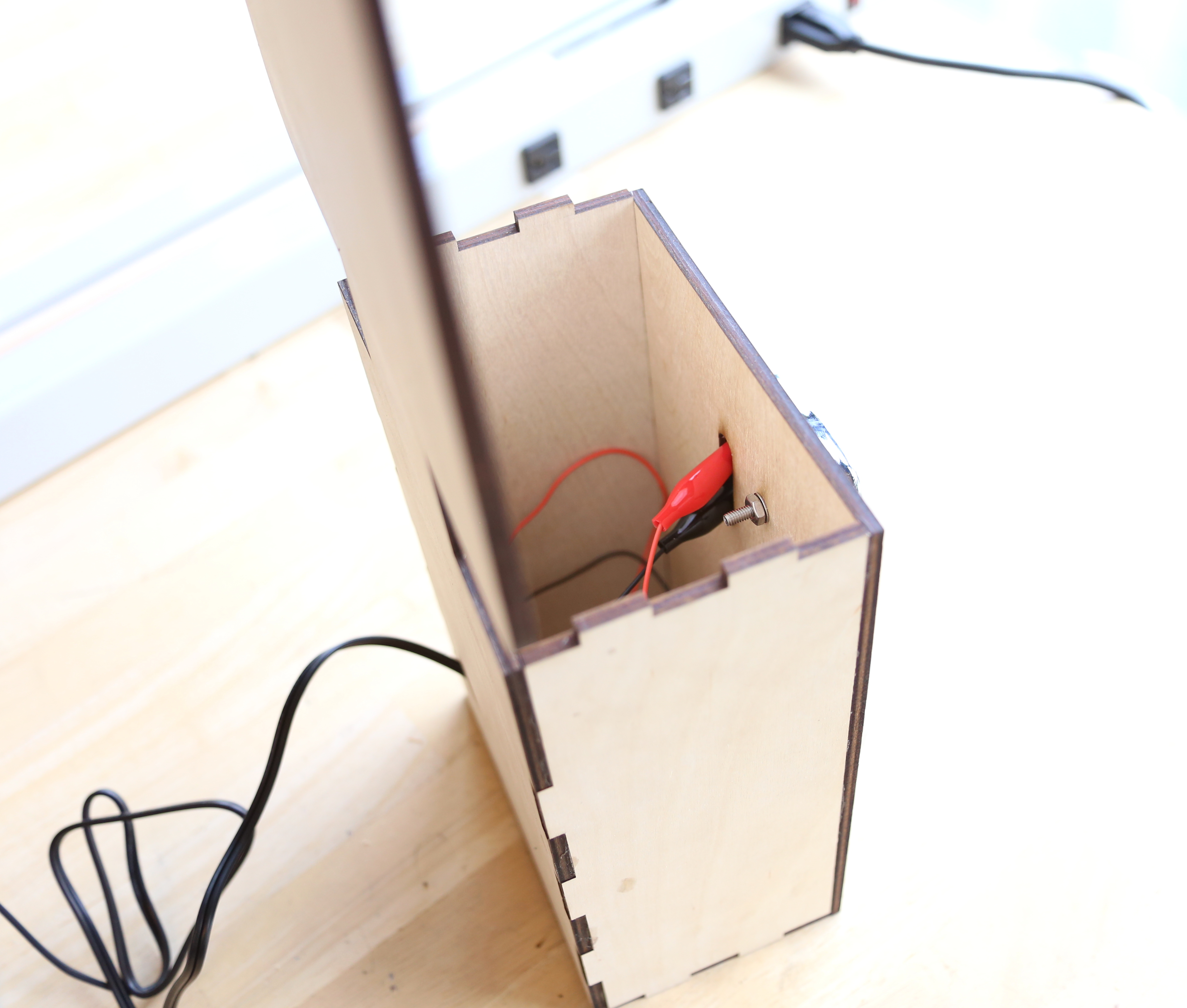
Thread the power plug through the back of the box into the case. Then stack the BBB on top of the external battery pack and place it in the case. Find the two nubs on the electric bell poking through the case (circled in red in the photo). Take the the alligator clips and attach each to one of the nubs.
Optional Mounting
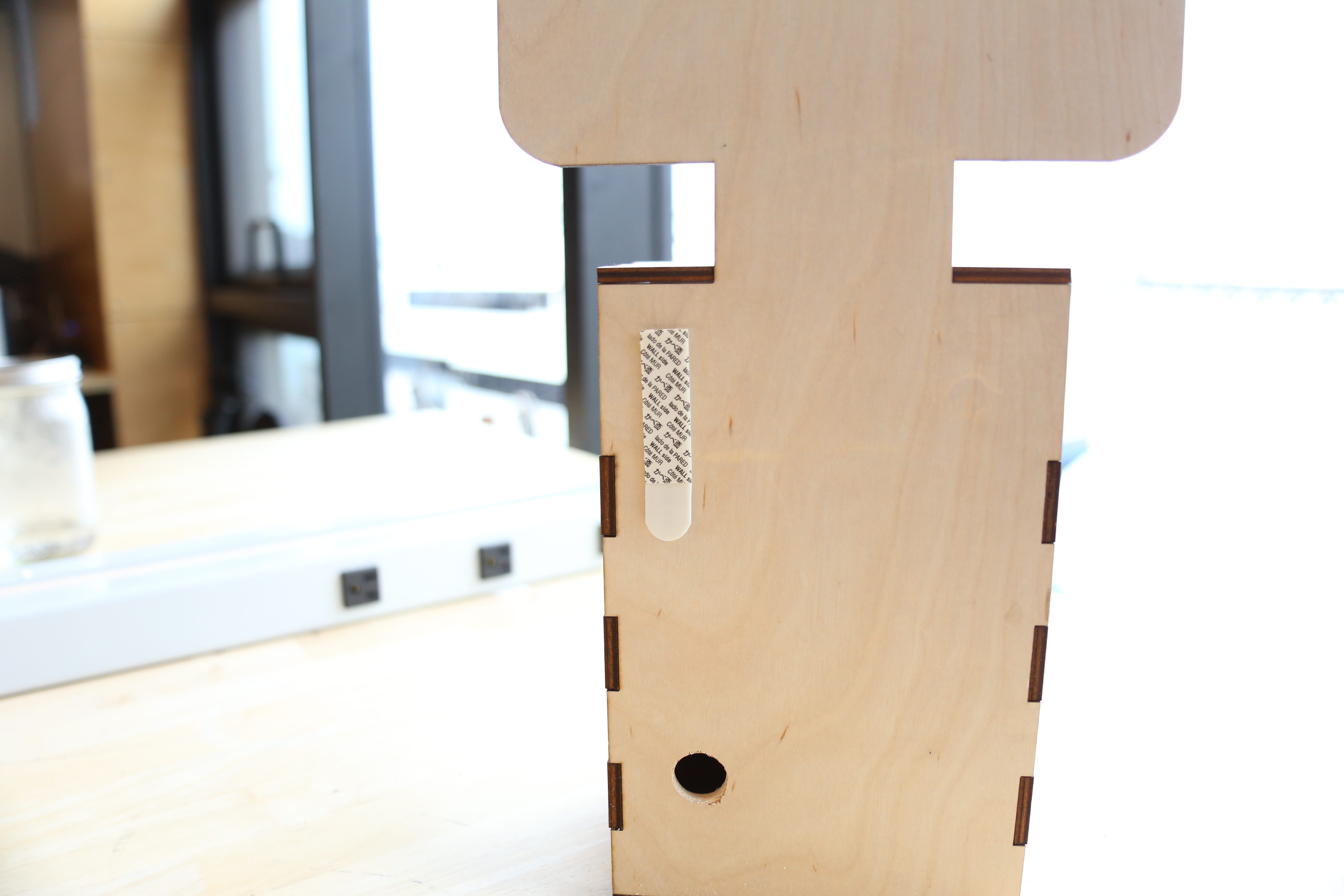
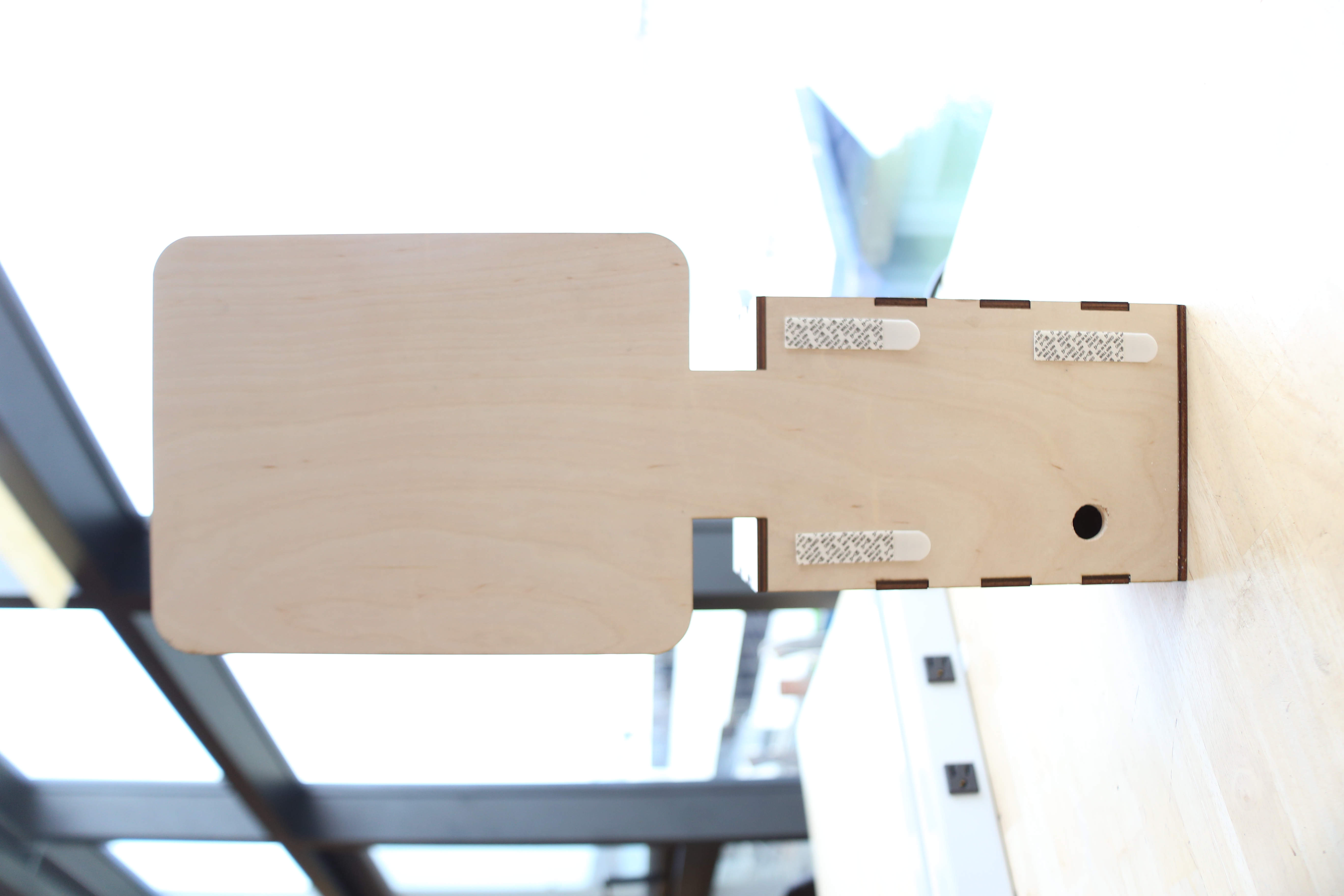
This alarm can sit on top of your desk or be mounted to your wall. Simply buy a pack of command strips and stick them to the alarm's back panel.
The Final Product
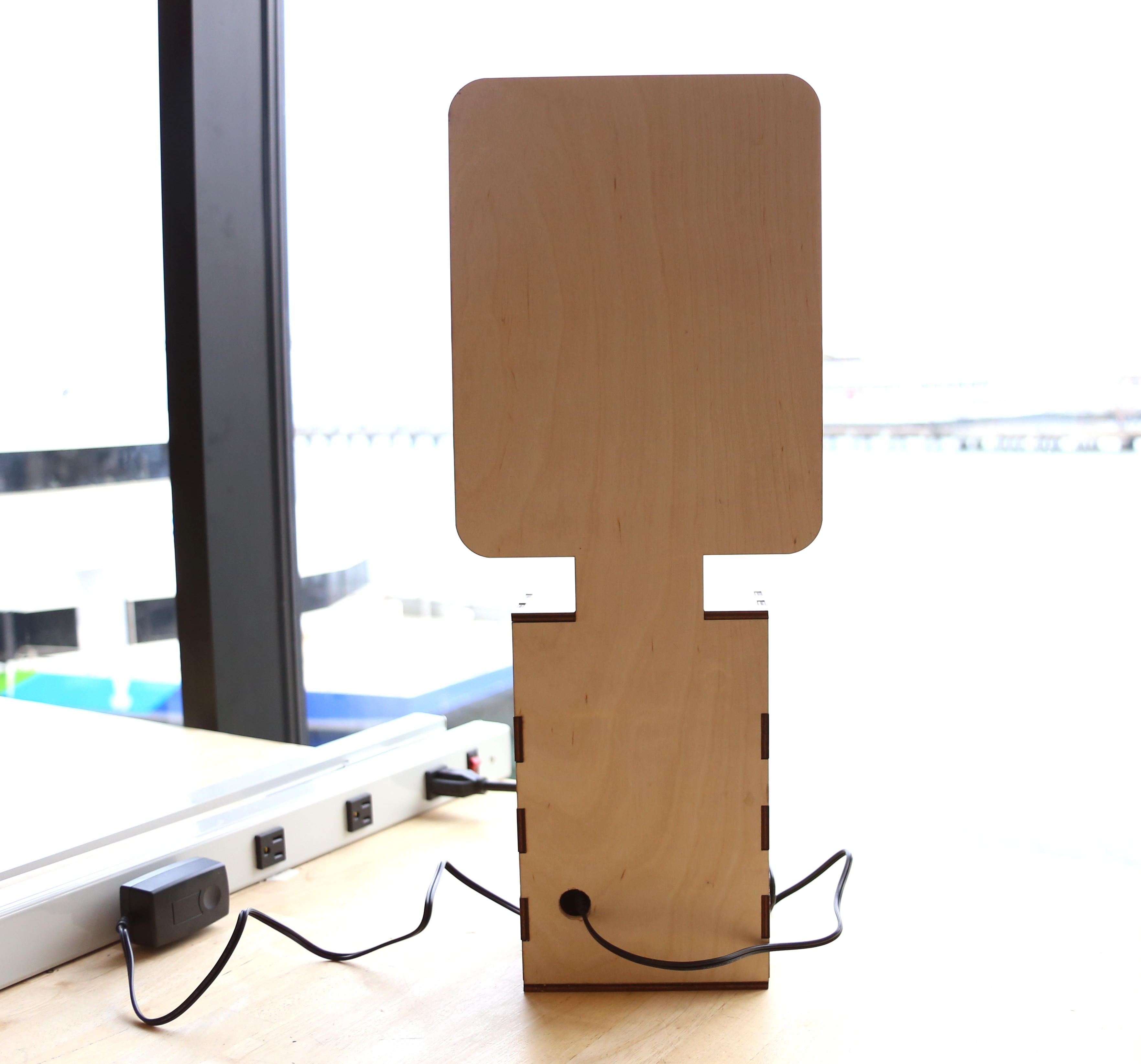
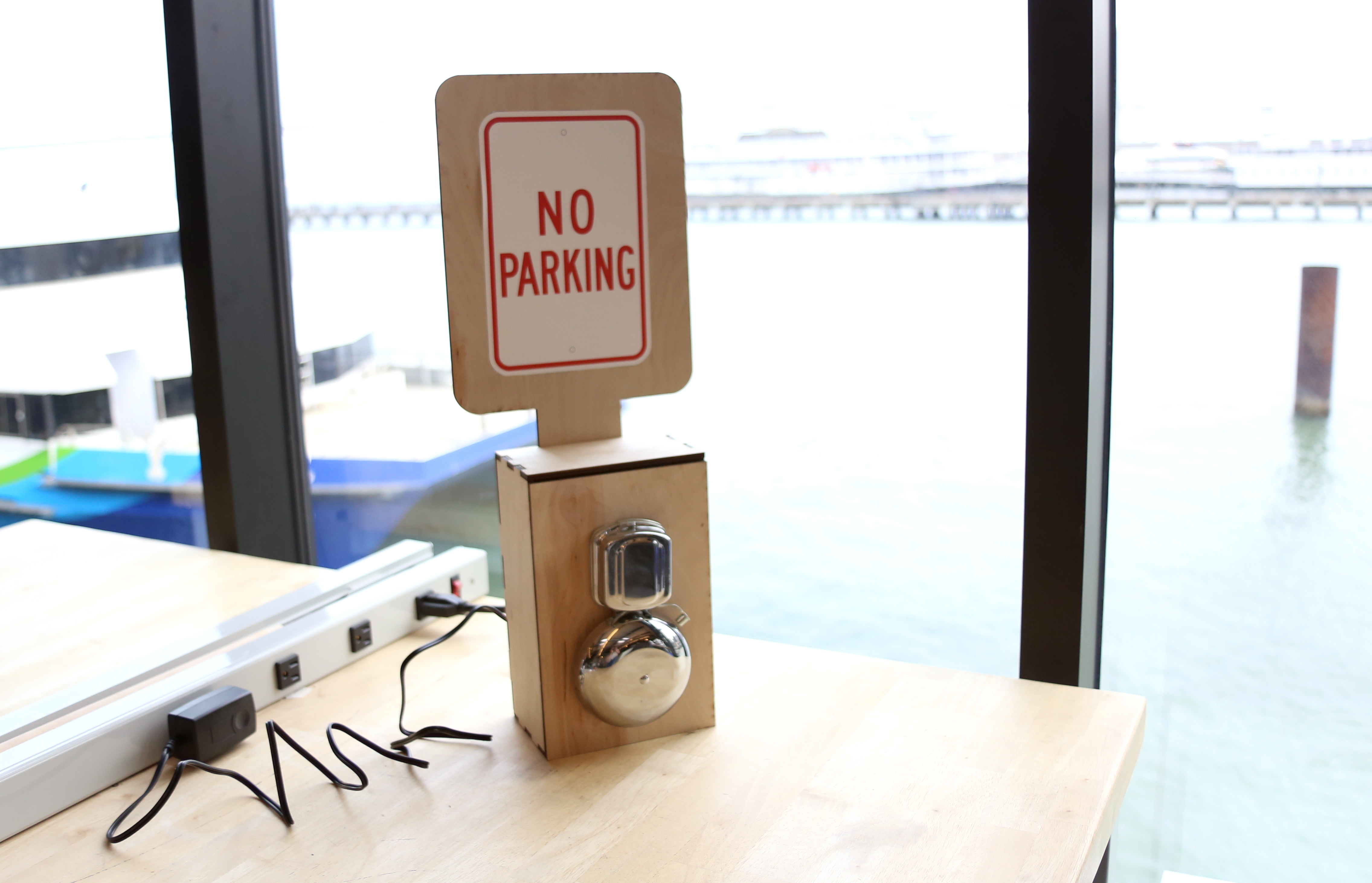
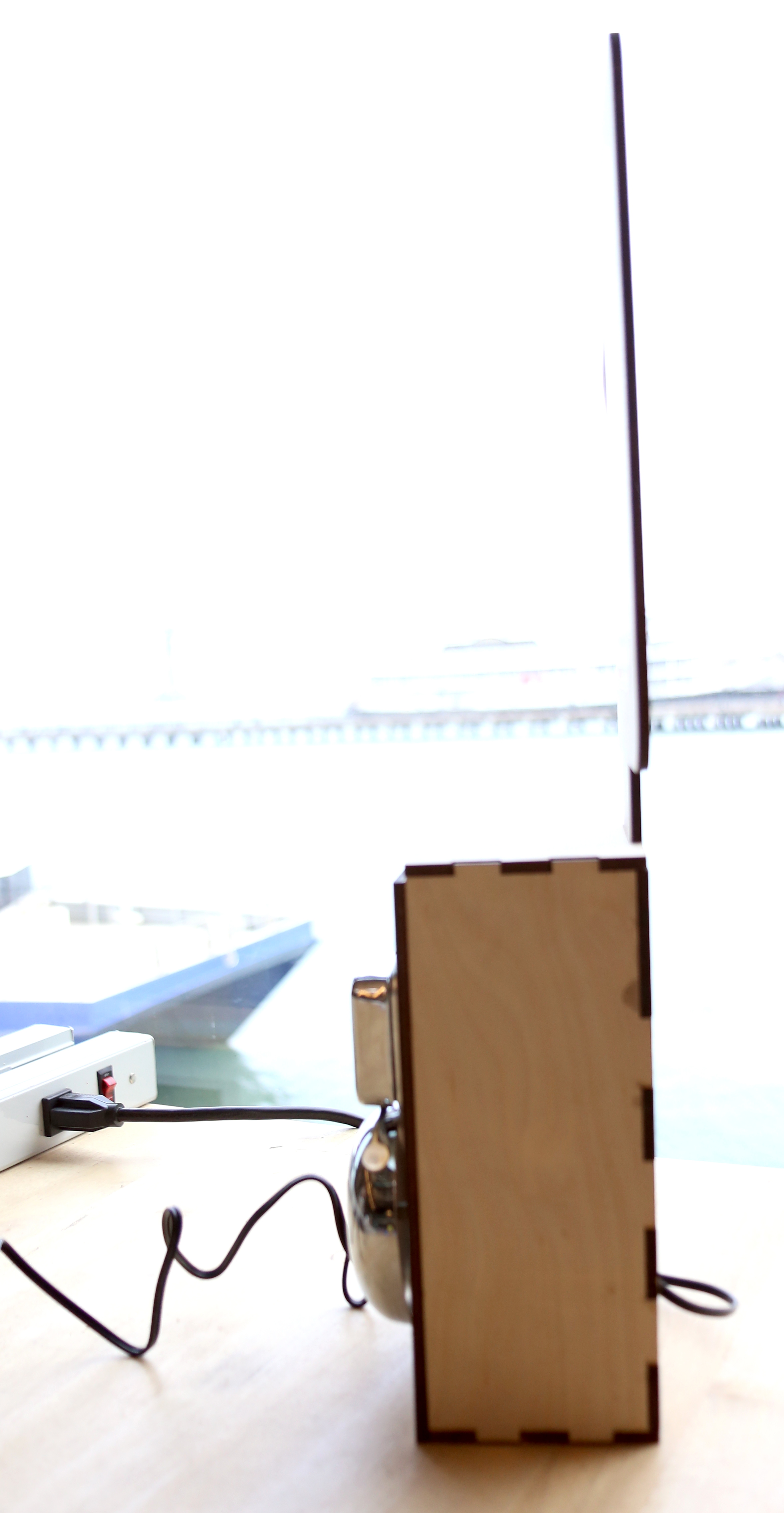
The Street Sweep Saver is complete!