Simple Python Clock
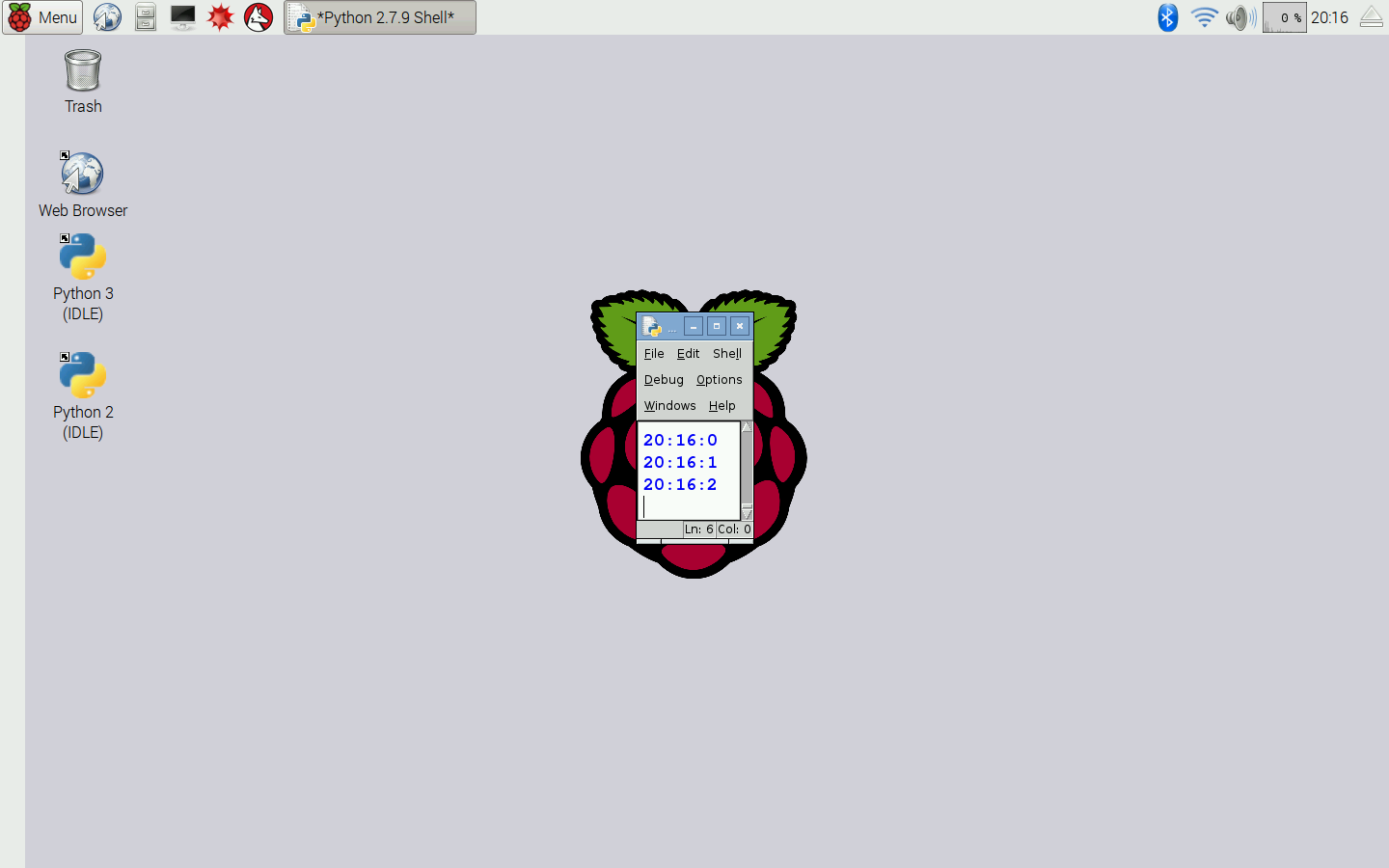
This is my Simple Python Clock that requires only seven lines of code and is very accurate. Beware, this code is written for use with Python 2. Python 3 will not work with this code.
Keep an eye out for Part 2 where I will show you how to use Python 3 in this project.
If you do not want to type all of the entire seven lines of code, I have a pre-written Word document here.
Downloads
Open Python Shell
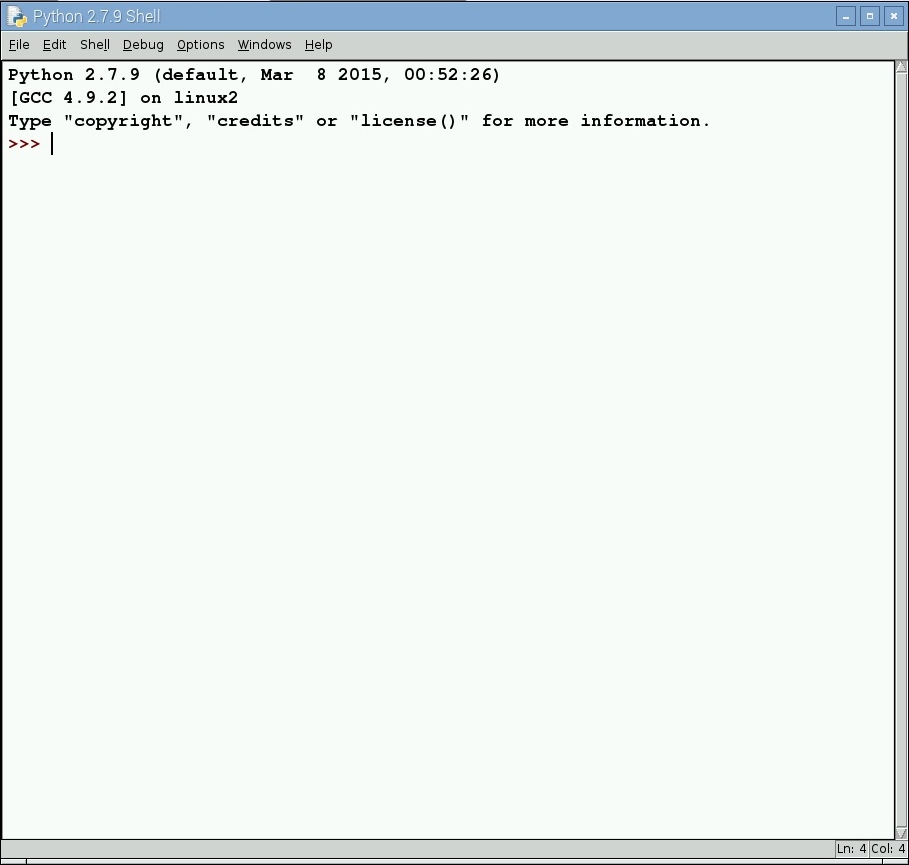
Begin by opening your choice of Python Shell (I personally prefer IDLE 2). Now open a new file.
Import Libraries
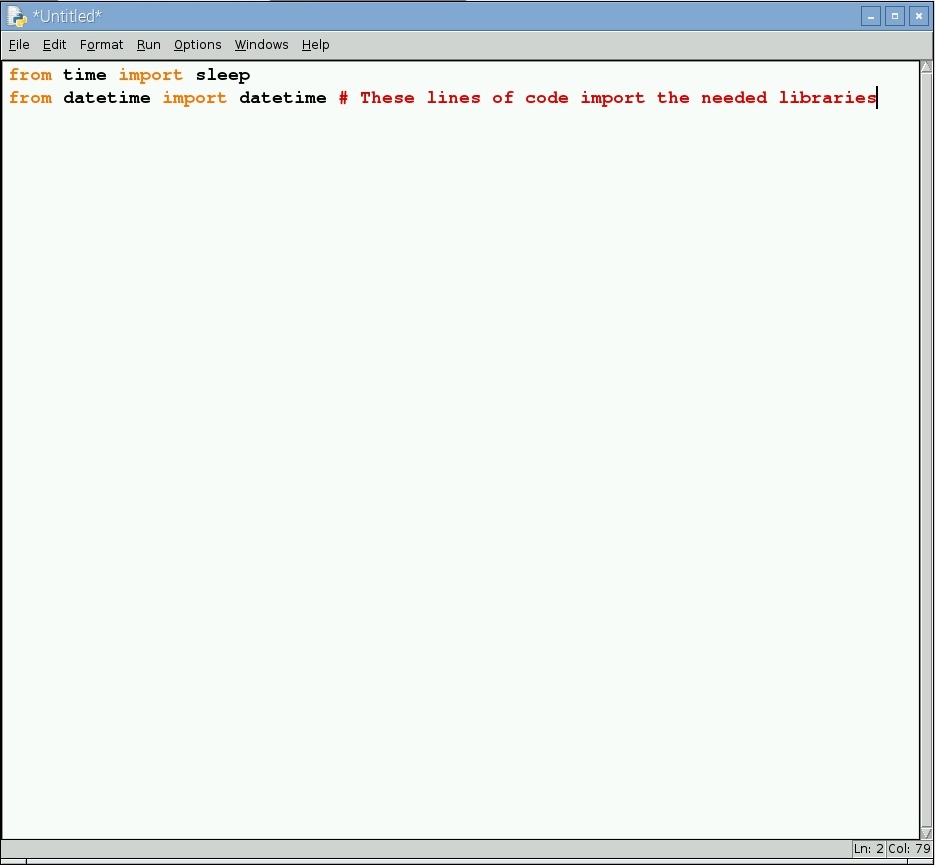
First, we need to import the needed libraries since Python cannot do their function alone.
The libraries we need are 'sleep' and 'datetime'.
from time import sleep
from datetime import datetime
Establishing 'now'
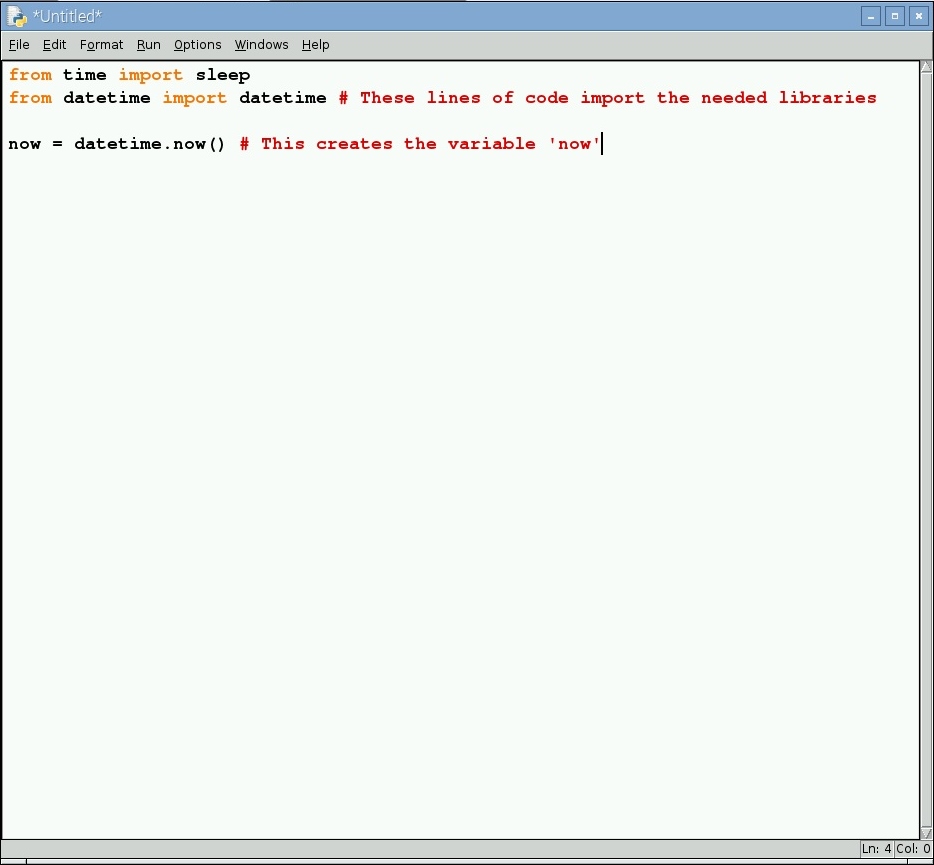
Next, we need to define the current time by using datetime.now and creating a variable.
now = datetime.now()
Making the Loop
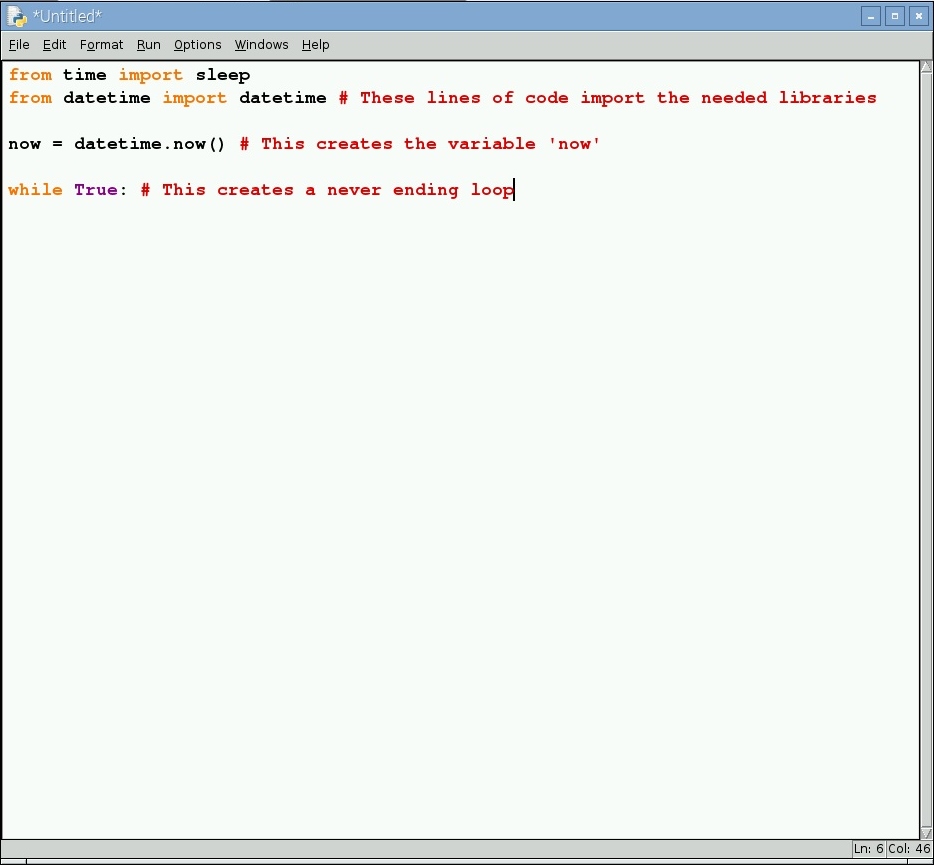
After that we need to make a constant loop so that all indented code below it will run and rerun, in this case, forever.
while True:
The 'print' Command
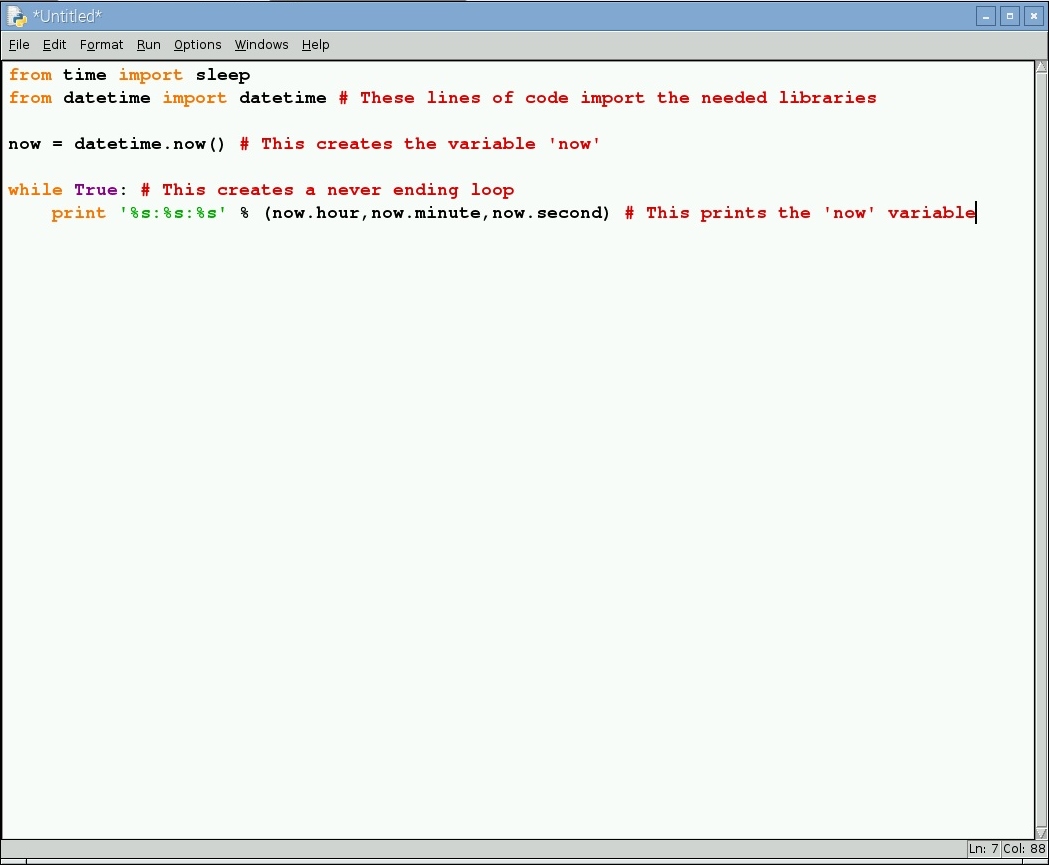
Then we need to tell the computer to print the current time one the screen. Don't worry if you're new to coding and not all of this line makes sense.
print '%s:%s:%s' % (now.hour,now.minute,now.second)
Sleeeep...
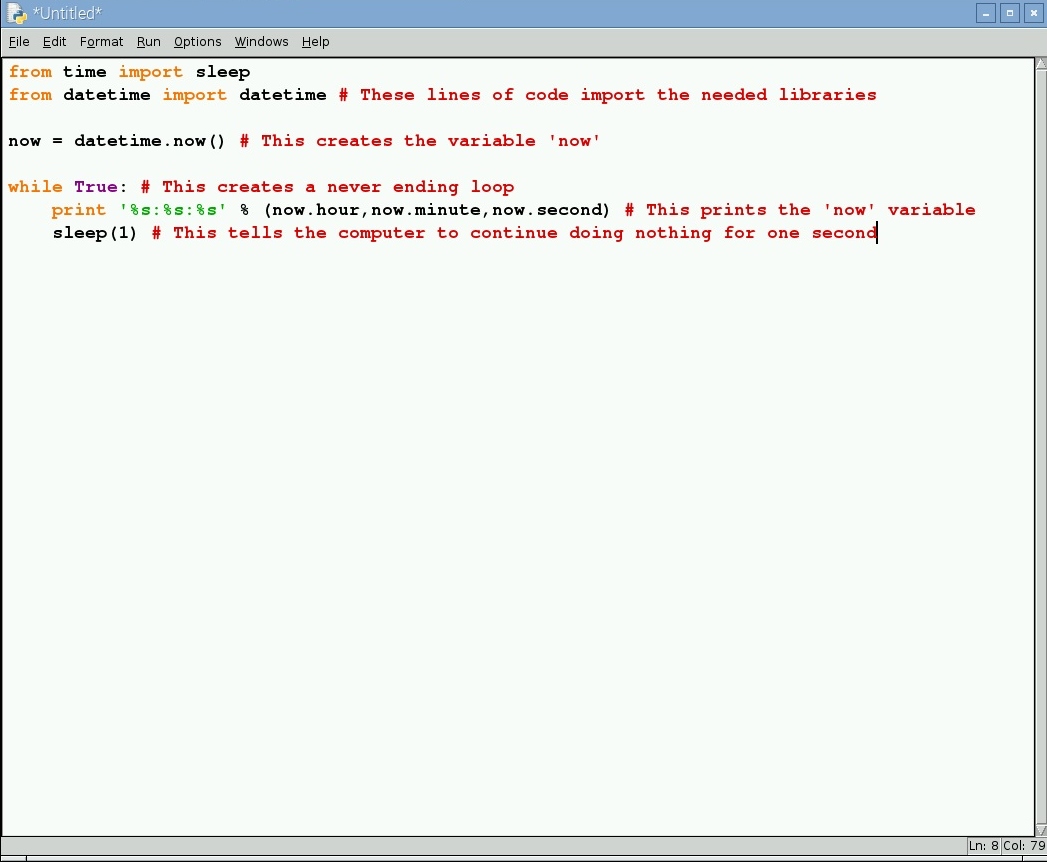
After we give the computer the 'print' command, we need to tell the computer to do nothing for one second.
sleep(1)
Resetting 'now'
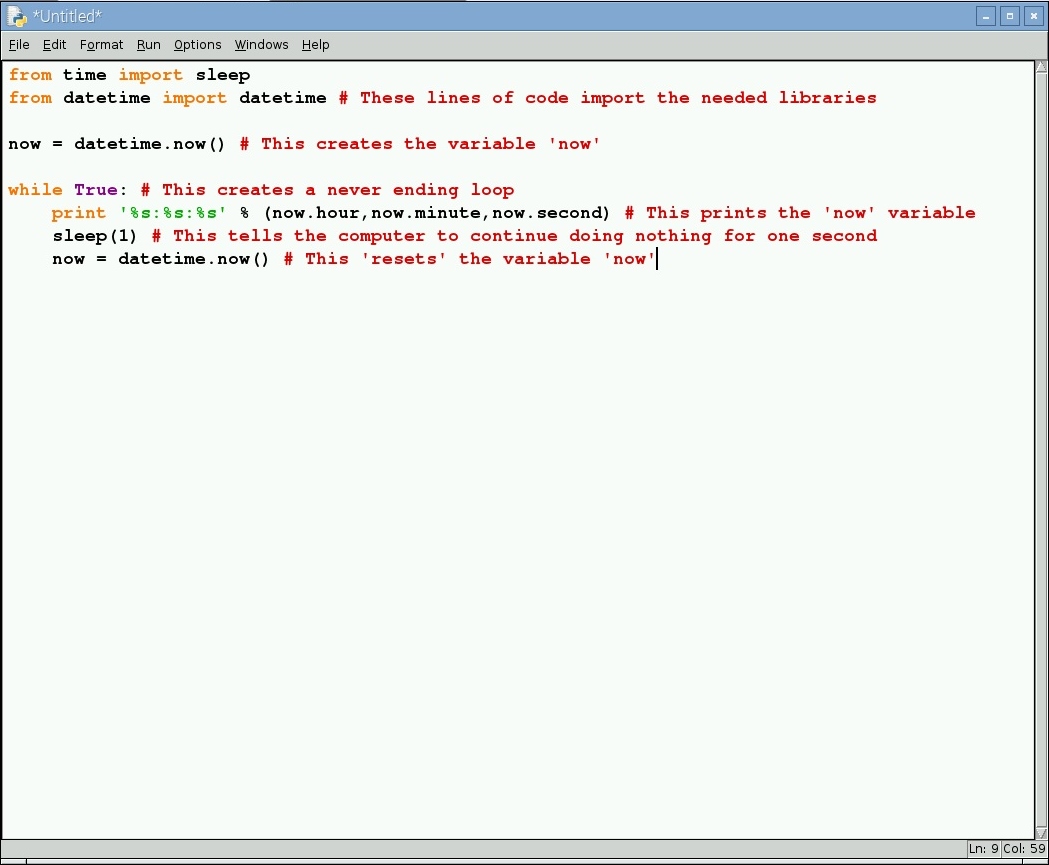
After the computer has slept for one second, our variable 'now' is no longer correct. So we need to reset it.
now = datetime.now()
Finished
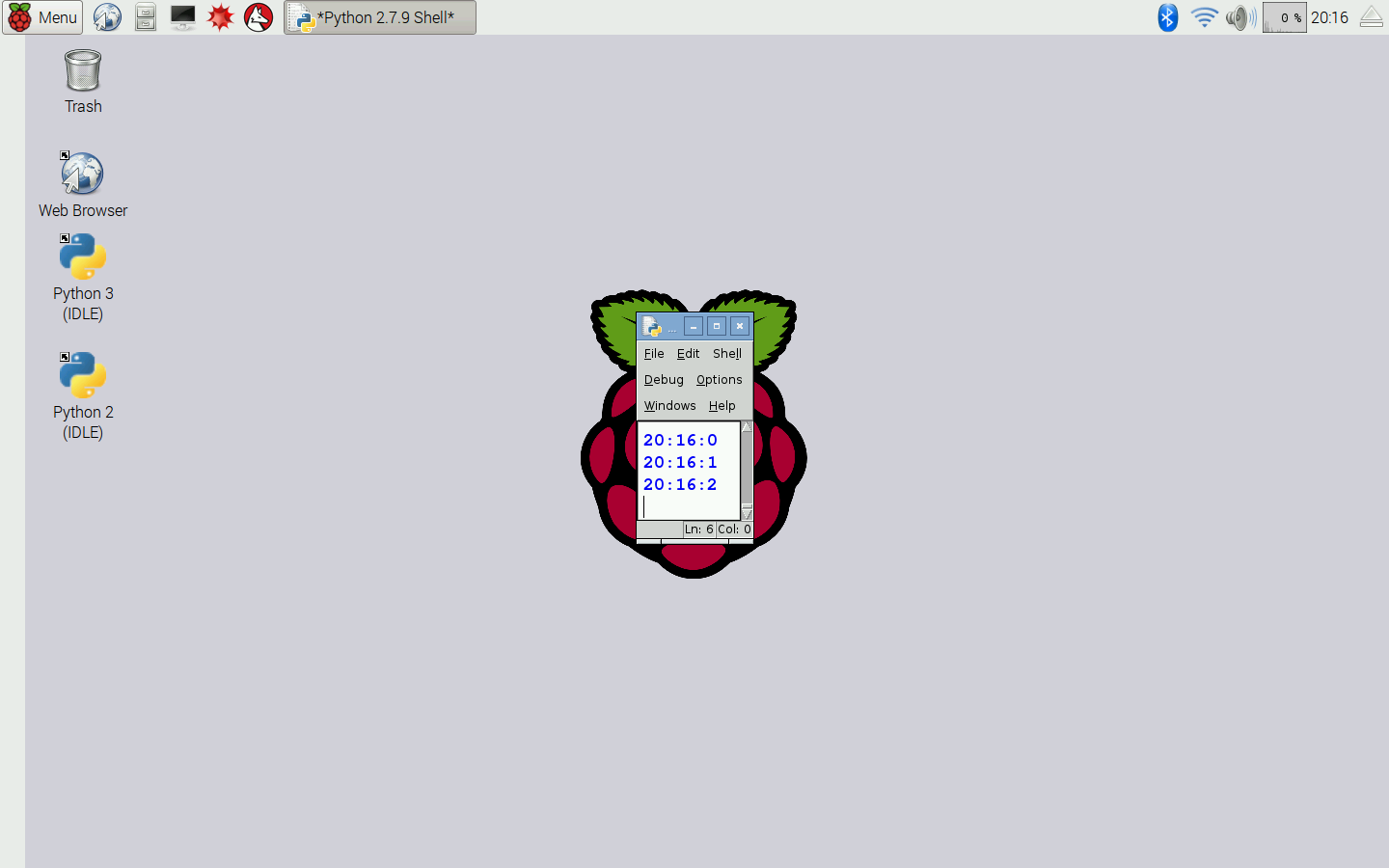
And now you're done! All that is left to do is run the program and resize the window to your preferred size. Happy coding!