Simple Animation Engine (java Program)
by Zach833 in Craft > Digital Graphics
5894 Views, 13 Favorites, 0 Comments
Simple Animation Engine (java Program)
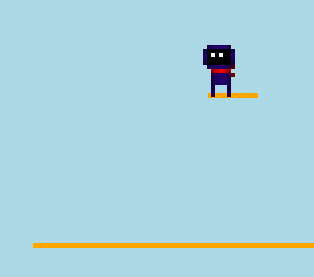
There are many different applications that handle all the background process that animate a character. This is not for any of them. This tutorial will explain how to program a basic animation engine. The tutorial is in Java and uses the jGRASP Integrated Development Environment.The Player.java file has been attached below for assistance.
Downloads
Get Images
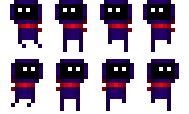
For the tutorial you will need at 8 images.
Jumping left
Standing left
Walking left 1 (front leg up)
Walking left 2 (back leg up)
Jumping right
Standing right
Walking right 1 (front leg up)
Walking right 2 (back leg up)
Set Up Class
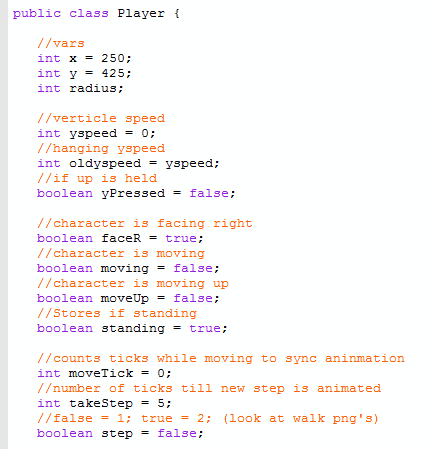
We are animating a player character for this tutorial so I named the class Player variable and declared some variables.
Add Movement
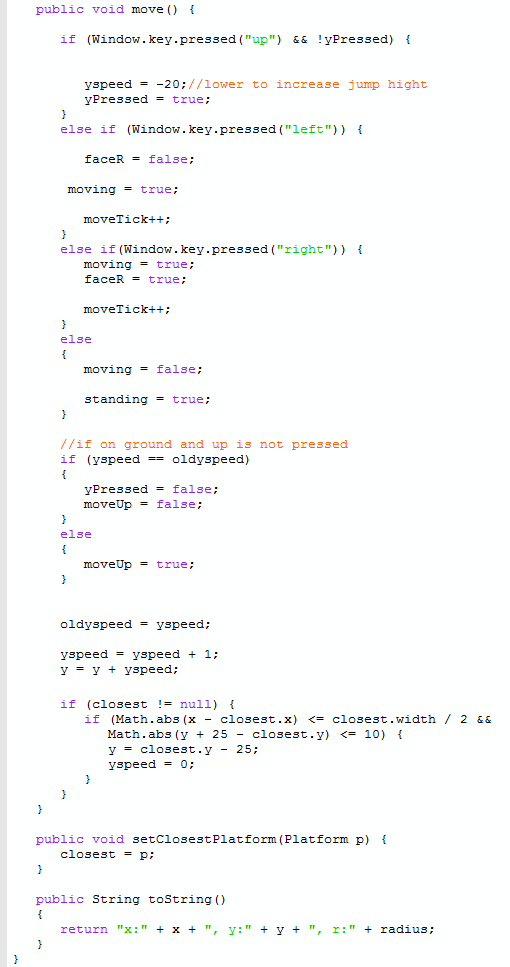
The next step is to program the controls for your character. I created a move method and mapped the movement to the arrow keys for this.
Tweak with the x and y speed to get a nice feeling movement and do not forget to implement the variable updates to moving and standing.
I used the yspeed and oldyspeed to detect if the Player was jumping since the top of a jump has zero y movement the standing image would be played. Using a comparison to the last yspeed prevents this logic error.
The closest detection was to help plant the character on a platform and can be ignored for the purposes of this tutorial
The toString() method is not necessary either but useful when debugging the character and getting the location of the character.
Animate Movement
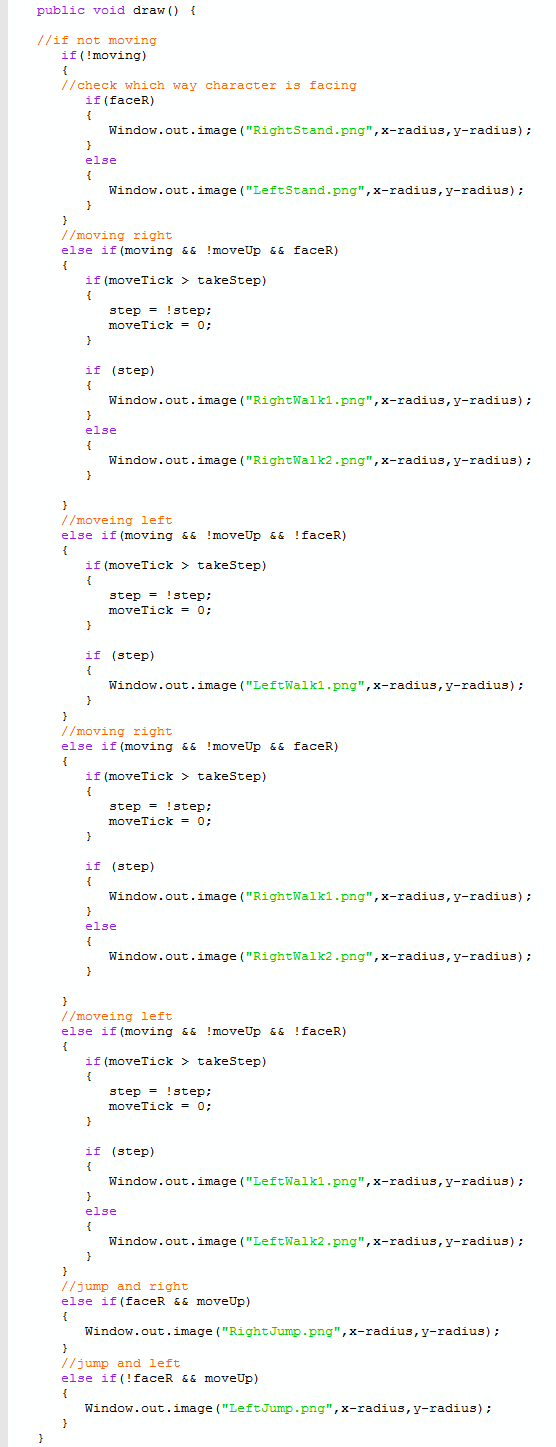
This is it! We can now take all the set up and create a state machine to handle all the animation cases.
The entire method is a sequence of if statements and nested if statements. The first if statement checks if the Player is moving. The variables should be updated with the move method so we don't have to worry about that here. in the first if it checks which way the Player was last moving and uses the correct image to reflect that. Next, We check if the Player is walking. To do this we check is the Player is moving but not jumping and we check if the Player is facing right. Then, we check the same thing but check if the Player is moving left. In the walking cases just discussed we want to update which image is being used to make the Player appear like they are walking. To accomplish this we use a boolean variable, step, and the integer, moveTick. The step method should change once moveTick is greater than takeStep. With all of this the Player should look like they are taking a step and change back and forth between the walking images. Lastly, we handle the jumps and use if statements to check the direction of the Player and if they are moving up. With this the draw method is done and the animation engine should be complete.
Thank You!
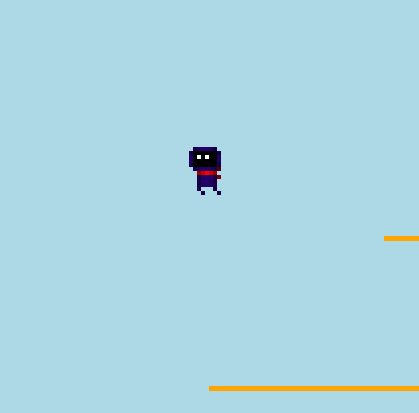
I hope you found this tutorial helpful in some way.