Simple Agriculture IOT With ThingSpeak and Matlab
by nishantsahay1234 in Circuits > Arduino
1028 Views, 1 Favorites, 0 Comments
Simple Agriculture IOT With ThingSpeak and Matlab
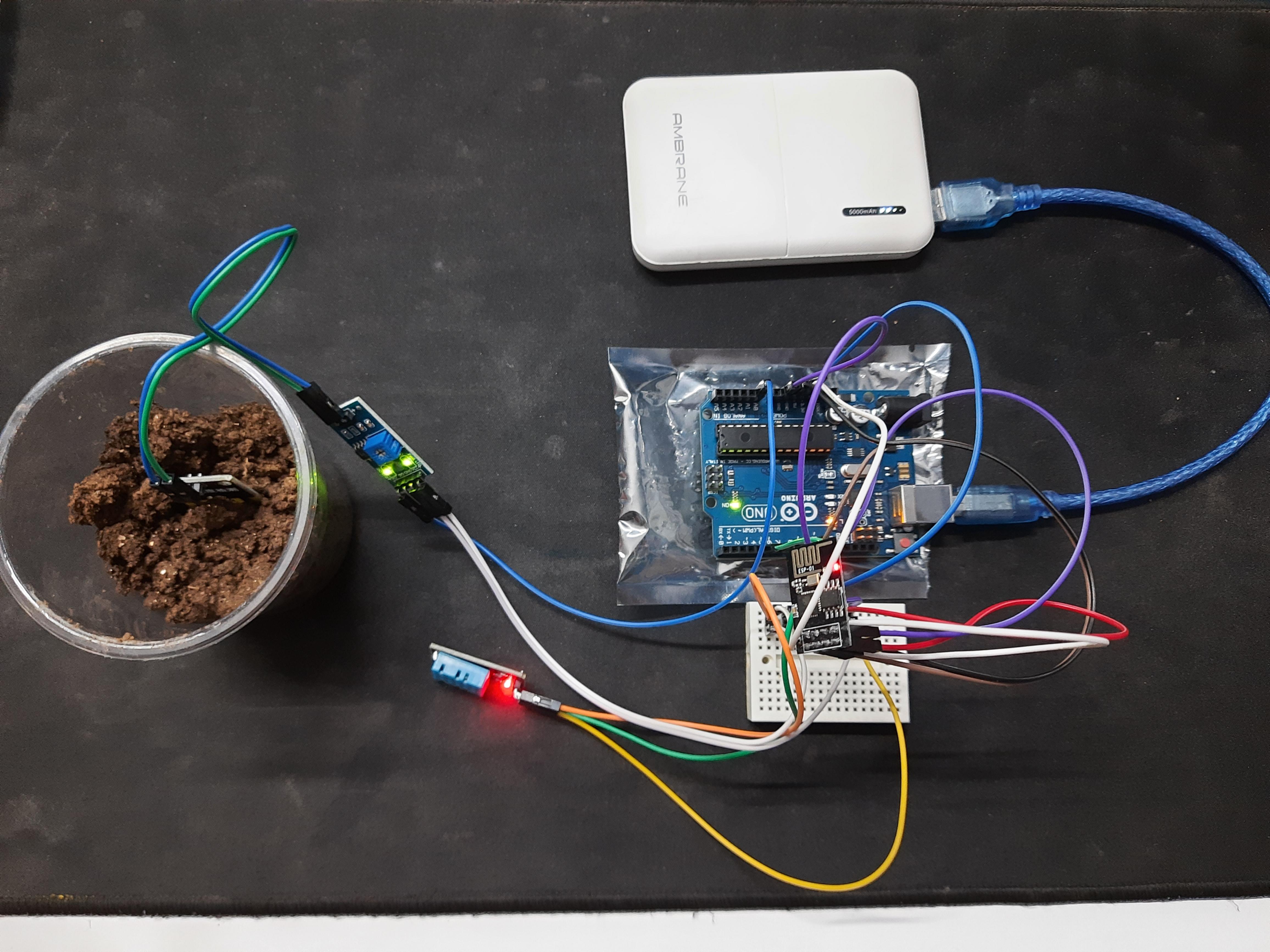
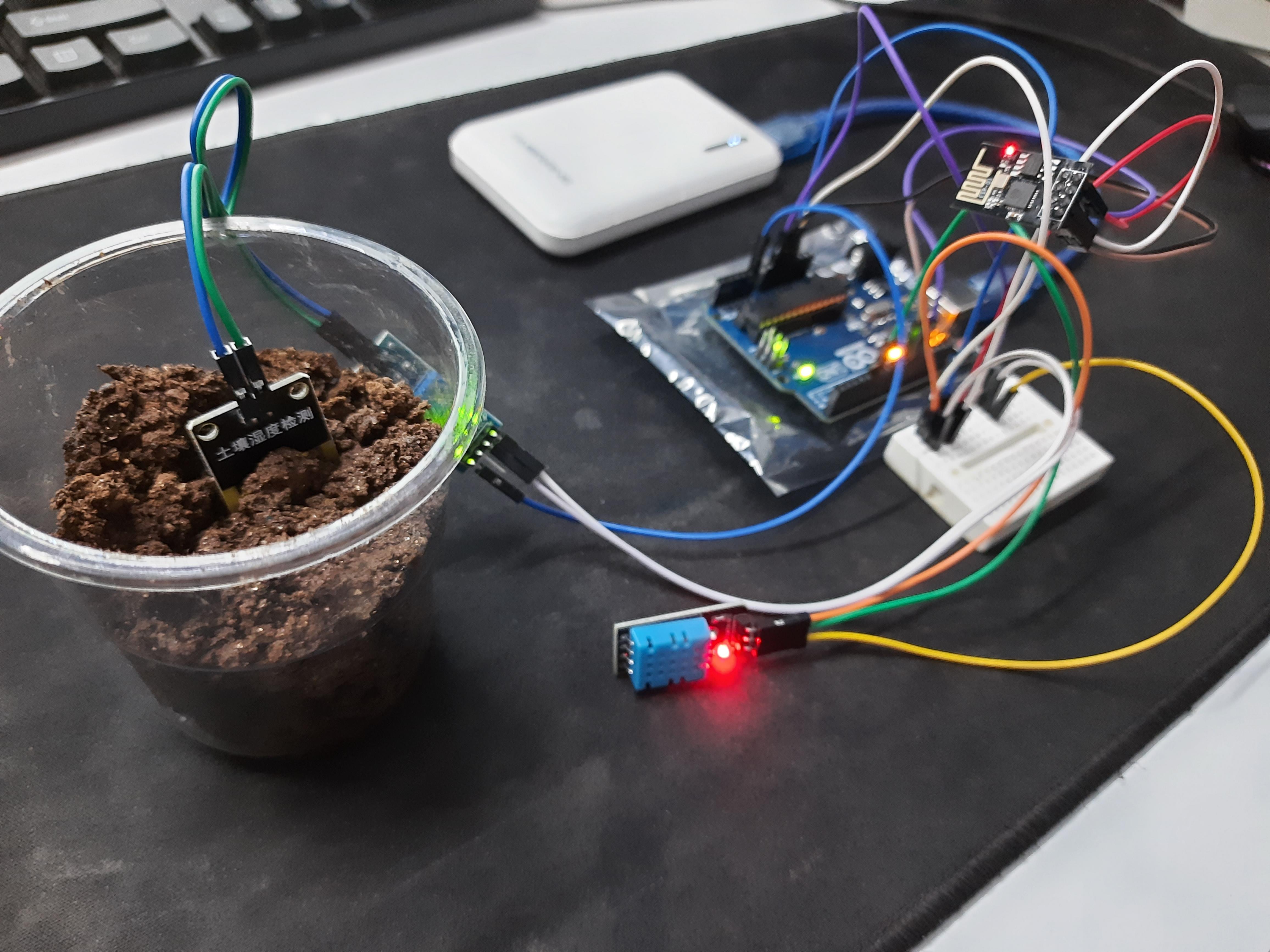
The following project uses a soil moisture sensor, DHT11 temperature sensor, and ESP8266 to collect temperature, humidity, and moisture readings and send them to ThingSpeak. This article will help you learn the basics of using ESP8266 and send a sensor data to a website via API like ThingSpeak.
Sensors Brief
DHT11 Sensor
DHT11 humidity & temperature Sensor Module is a basic, low-cost digital temperature and humidity sensor. It uses a capacitive humidity sensor and a thermistor to measure the surrounding air, and outputs a digital signal on the data pin (no analog input pins needed).
Soil Moisture Senor
Soil moisture sensor module is most sensitive to the ambient humidity is generally used to detect the moisture content of the soil; Module in the soil humidity less than a set threshold value when the DO port output high, when the when soil humidity exceeds the threshold value is set, the module D0 output low; Small plates digital outputs D0 can be directly connected with the microcontroller, microcontroller to detect high and low, and thus to detect soil moisture; Small plates digital output DO shop relay module can directly drive the buzzer module, and which can form a soil moisture alarm equipment; Small plates analog output AO and AD module connected through the AD converter, you can get more accurate values of soil moisture.
Supplies
Arduino UNO
DHT11 Temperature sensor
Soil Moisture Sensor
ESP8266-ESP01 wifi module
Setting Up ESP8266
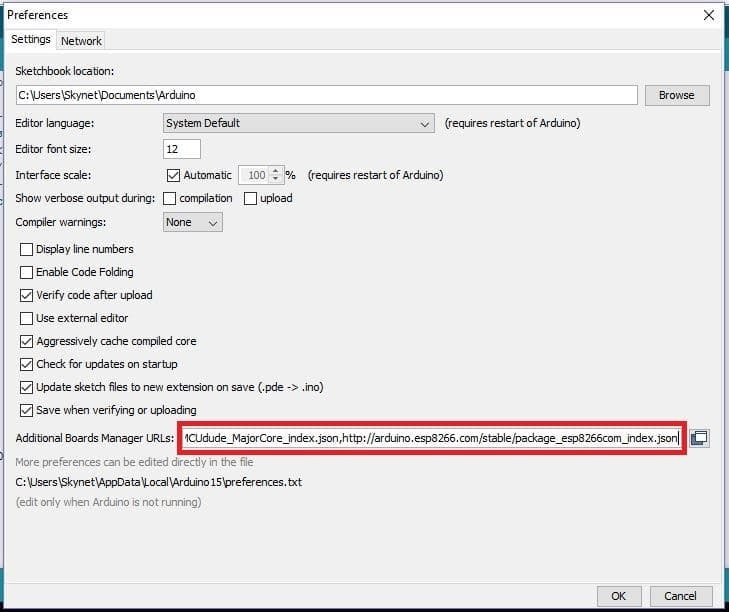
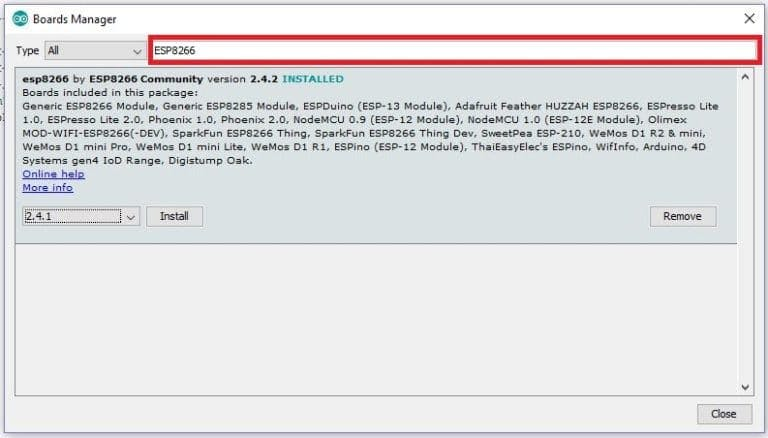
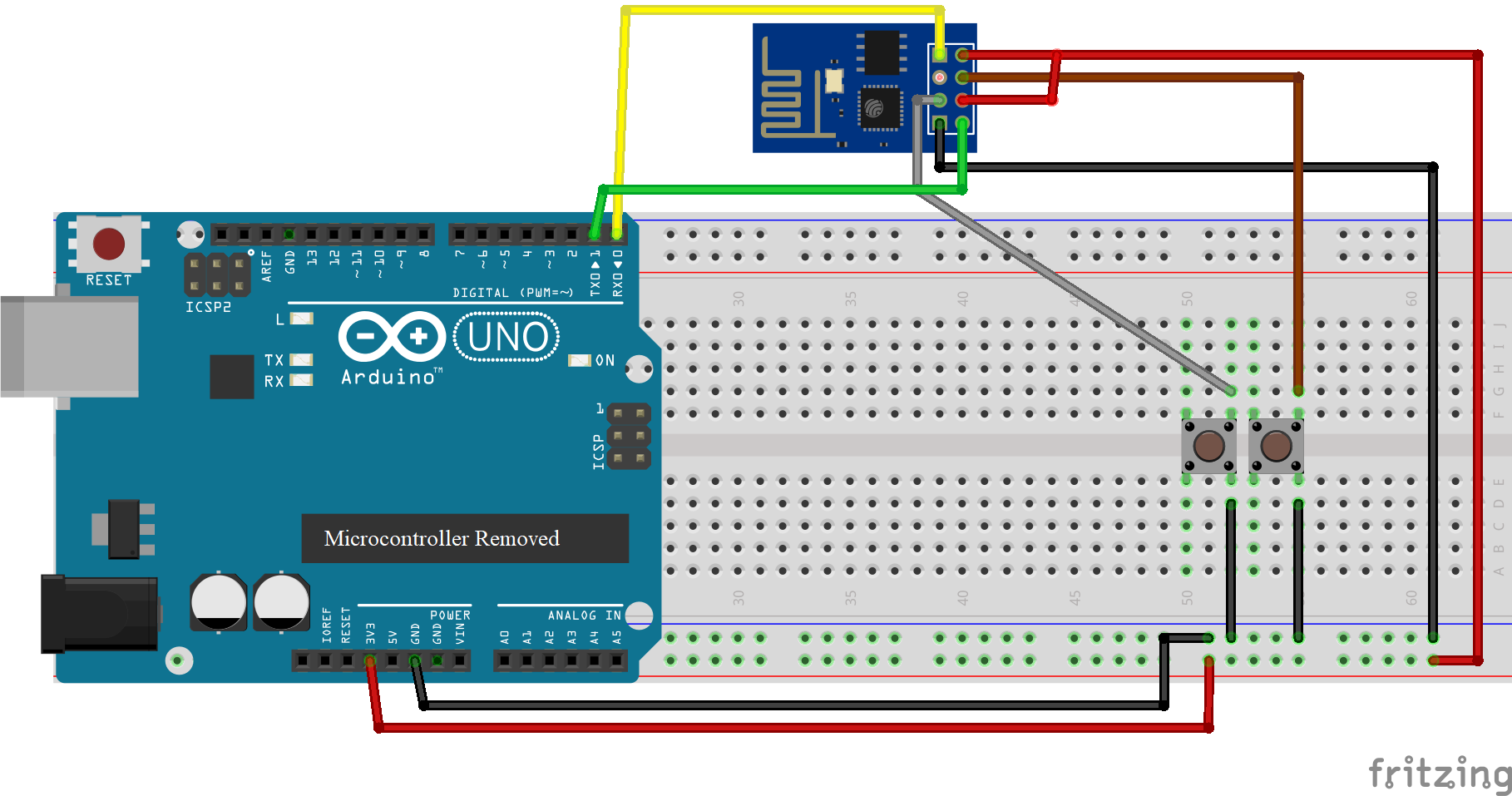
Programming ESP8266
In order to send the data over the internet we need to first connect the ESP8266 with a WI-FI network and then program it to collect values and send it to ThingSpeak API. The code for the same is explained as follows:
First you need to download the libraries for ESP8266 and ThingSpeak, you can find these over here:
ThingSpeak library: https://github.com/mathworks/thingspeak-arduino
In order to download ESP8266 library follow these steps:
- Copy this link: https://github.com/mathworks/thingspeak-arduino
- Now go to Tools > Board > Boards Manager.
- Paste the URL on the box and click “OK”.
- A window will open like this
- Now open Arduino IDE and click on File > Preferences.
- Type “ESP8266” on the box as shown and you will get installation option, select the latest version and click install.
Now, in order to use ESP8266 first configure the following circuit. Also, make sure to remove the microcontroller from the Arduino UNO before you proceed further,
Now, we first need to set the ESP8266 in programming mode, we do this using the following steps:
- Power ON the circuit.
- Press and hold the flash switch.
- While holding the flash switch, press the reset switch once.
- Now, let go of the flash switch.
You may see a blue LED blink in the ESP8266 if you do this process right. Once, these steps are done we can move on to uploading the actual program. Make sure to select Generic ESP8266 Module in board:
#include "ThingSpeak.h" #include <ESP8266WiFi.h>
//------- WI-FI details ----------// char ssid[] = " "; //SSID here char pass[] = " "; // Passowrd here //--------------------------------/
/----------- Channel details ----------------// unsigned long Channel_ID = ; // Your Channel ID const char * myWriteAPIKey = " "; //Your write API key //-------------------------------------------//
const int Field_Number_1 = 1; const int Field_Number_2 = 2; const int Field_Number_3 = 3; String value = ""; int value_1 = 0, value_2 = 0, value_3 = 0; int x, y, z; WiFiClient client;
void setup() { Serial.begin(115200); WiFi.mode(WIFI_STA); ThingSpeak.begin(client); internet(); }
void loop() { internet(); if (Serial.available() > 0) { delay(100); while (Serial.available() > 0) { value = Serial.readString(); if (value[0] == '*') { if (value[7] == '#') { value_1 = ((value[1] - 0x30) * 10 + (value[2] - 0x30)); value_2 = ((value[3] - 0x30) * 10 + (value[4] - 0x30)); value_3 = ((value[5] - 0x30) * 10 + (value[6] - 0x30)); } } } } upload(); }
void internet() { if (WiFi.status() != WL_CONNECTED) { while (WiFi.status() != WL_CONNECTED) { WiFi.begin(ssid, pass); delay(5000); } } }
void upload() { ThingSpeak.writeField(Channel_ID, Field_Number_1, value_1, myWriteAPIKey); delay(15000); ThingSpeak.writeField(Channel_ID, Field_Number_2, value_2, myWriteAPIKey); delay(15000); ThingSpeak.writeField(Channel_ID, Field_Number_3, value_3, myWriteAPIKey); delay(15000); value = ""; }
In order to send the data we first align it in a desired stream and then add the start and stop symbols. This can be explained with an example:
Lets say at a given time the value of temperature, humidity, and moisture is 30C, 71%, and 62% respectively. Each of these values at max can be a 2 digit integer. If all these values are written together in the same order then that would be 30-71-62. Or in the program we would see it as a string '307162'. This way we send our sensor values to the serial port which is later separated by the program in ESP8266. However, we would have to define a start and stop symbol to differentiate between two sets of values. This is done using start symbol as '*' (asterisk) and stop symbol as '#'(hash). So, for this we declare the empty string type variable. You will see in the Arduino Program that when we wish to write the data to this variable, we first send the start symbol to the serial port, then send the data stream, and then send the stop symbol. In the program these values are simply separated using the indices. value_1 holds temperature, value_2 holds humidity and value_3 holds moisture value.
Note, YOU CANNOT SEND DECIMAL VALUES. And this is understandable based on the method we use to send our data. So, now if you have set the ESP8266 in programming mode as described you are ready to upload the code over the ESP8266.
Again, make sure to connect the circuit properly and DO NOT CONNECT ANY PINS TO 5V else you will burn the ESP IC.
Further, remember to remove the microcontroller from the Arduino and select generic module esp8266 as the board. Also, make sure to put the microcontroller back in before moving further.
Connecting Sensor DHT11
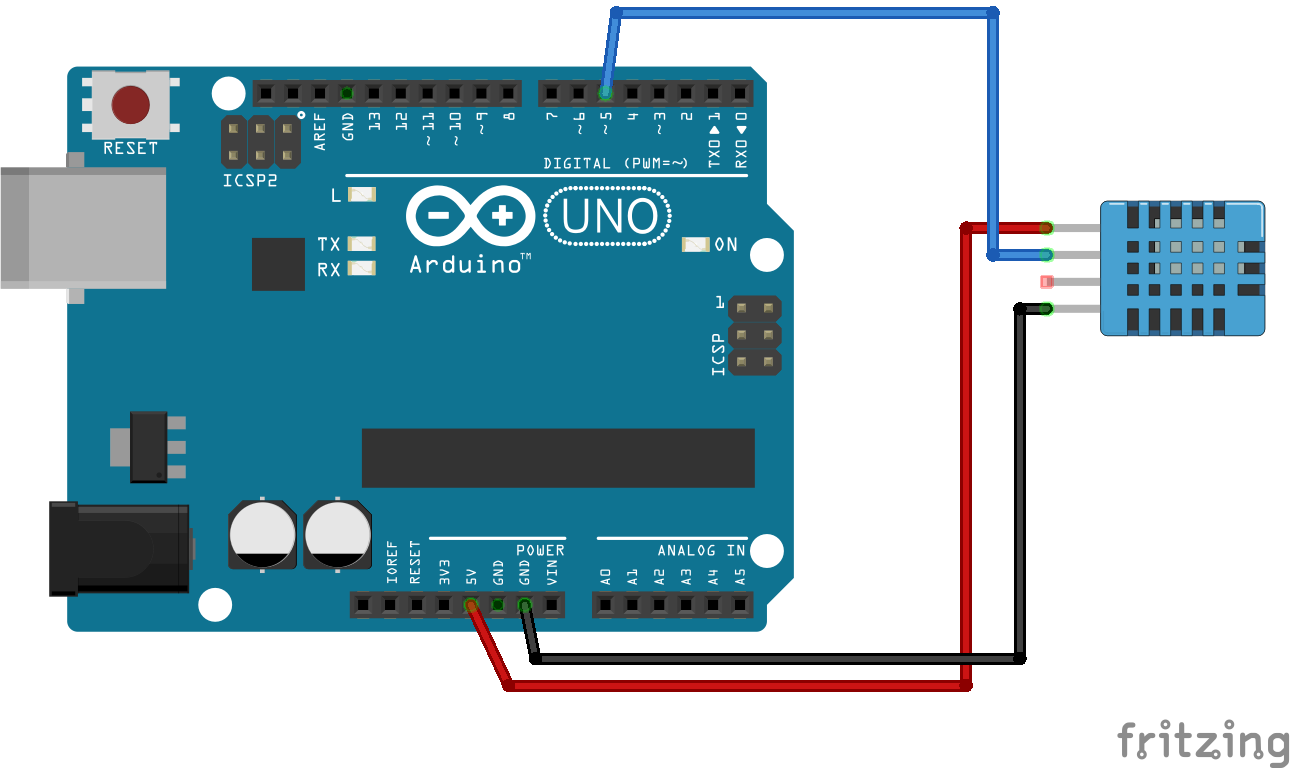
DHT11 is connected to digital pin 5 as per this program here is a sample code that you can run to see if its working properly:
#include <dht.h>
dht DHT;
#define DHT11_PIN 5
void setup() { Serial.begin(115200); Serial.println("DHT TEST PROGRAM "); Serial.print("LIBRARY VERSION: "); Serial.println(DHT_LIB_VERSION); Serial.println(); Serial.println("Type,\tstatus,\tHumidity (%),\tTemperature (C)"); }
void loop() { // READ DATA Serial.print("DHT11, \t"); int chk = DHT.read11(DHT11_PIN); switch (chk) { case DHTLIB_OK: Serial.print("OK,\t"); break; case DHTLIB_ERROR_CHECKSUM: Serial.print("Checksum error,\t"); break; case DHTLIB_ERROR_TIMEOUT: Serial.print("Time out error,\t"); break; case DHTLIB_ERROR_CONNECT: Serial.print("Connect error,\t"); break; case DHTLIB_ERROR_ACK_L: Serial.print("Ack Low error,\t"); break; case DHTLIB_ERROR_ACK_H: Serial.print("Ack High error,\t"); break; default: Serial.print("Unknown error,\t"); break; } // DISPLAY DATA Serial.print(DHT.humidity, 1); Serial.print(",\t"); Serial.println(DHT.temperature, 1);
delay(2000); }
You can find other programs for different DHT modules in examples. For that simply go to File > Examples> DHTlib.
Connecting Soil Moisture Sensor
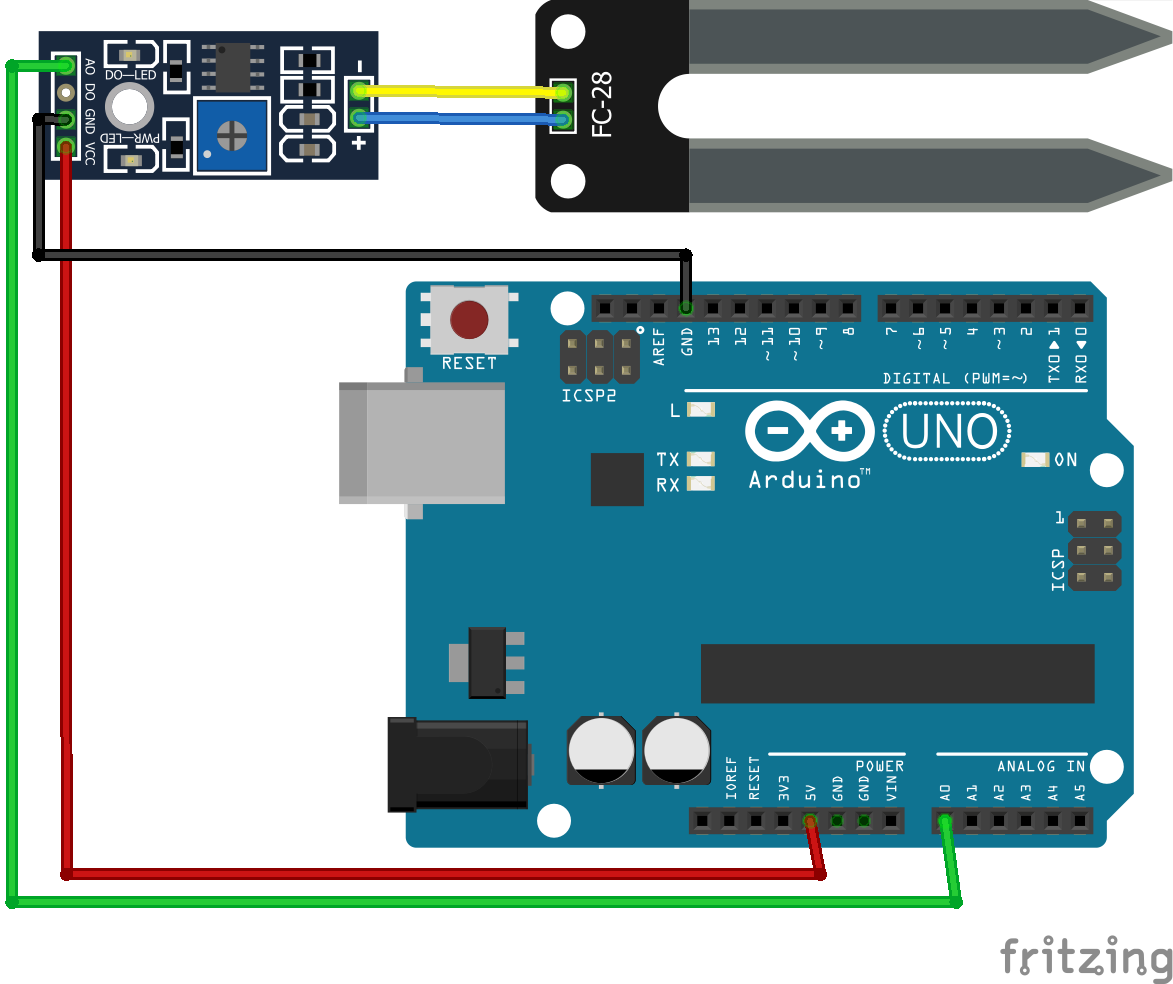
The soil moisture sensor gives its values as analog. So we connect it to analog pin A0.
You can use the following code to check if sensor is working or not:
int op_value; void setup() { Serial.begin(9600); delay(2000); }
void loop() { op_value = analogRead(A0); op_value = map(op_value,550,0,0,100); Serial.print("Moisture(%): "); Serial.print(op_value); Serial.print("%"); delay(1000); }
Furthur if you wish to check both the sensors togehter you can connect them as per the circuits shown before and use this program:
#include <dht.h> dht DHT; #define DHT11_PIN 5 int opval; void setup() { Serial.begin(9600); }
void loop() { DHT.read11(DHT11_PIN);
Serial.print("Humidity: "); Serial.print(DHT.humidity); Serial.print("% "); Serial.print("Temperature: "); Serial.print(DHT.temperature); Serial.print(" C"); opval = analogRead(A0); opval = map(opval,550,0,0,100); Serial.print("\nMoisture level: "); Serial.print(opval); Serial.print("% \n\n"); delay(2500); }
Connecting ESP8266 to Sensors
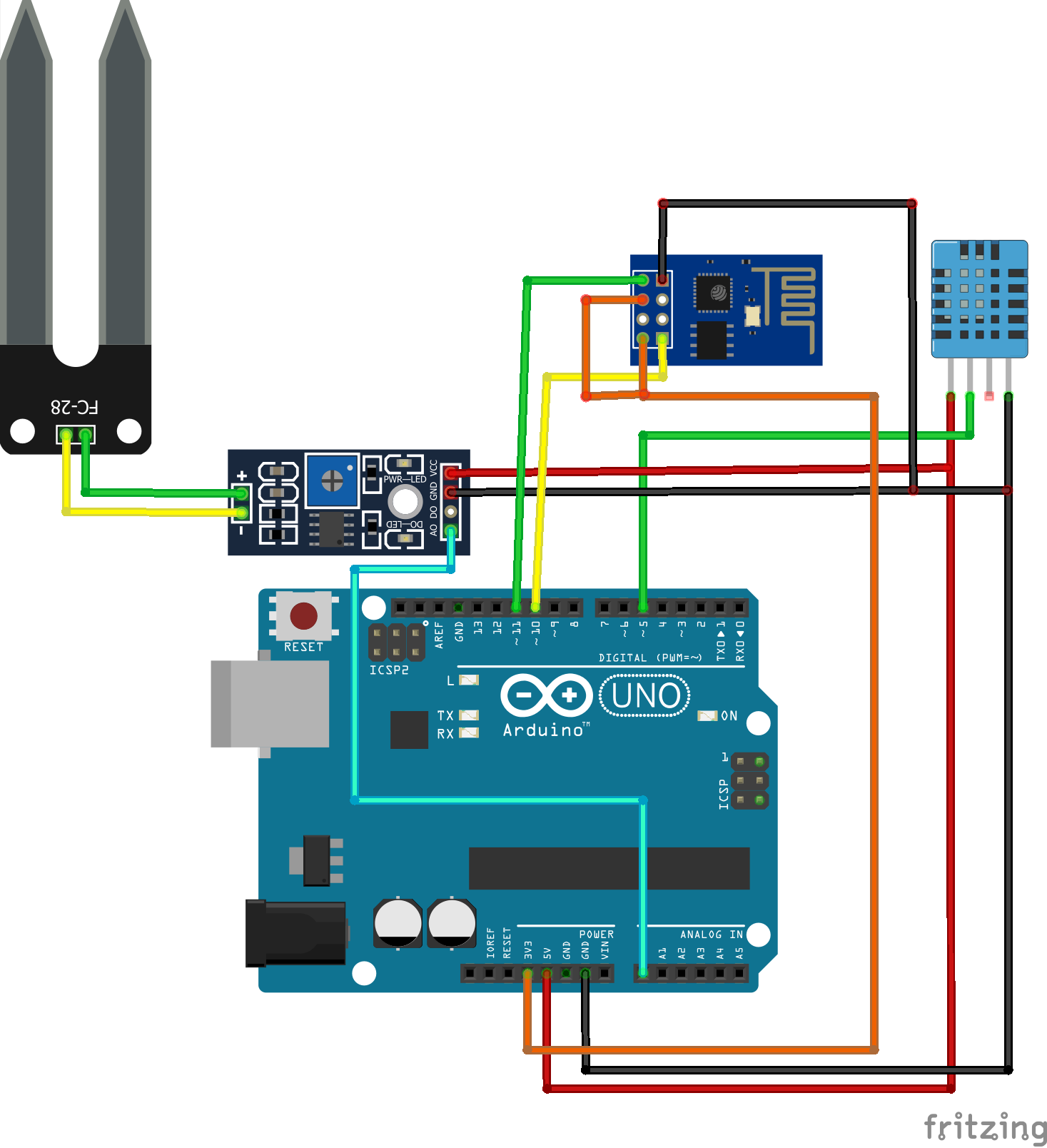
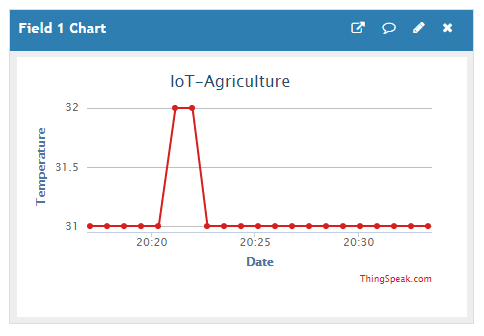
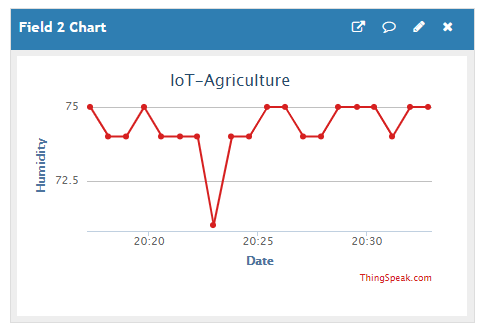
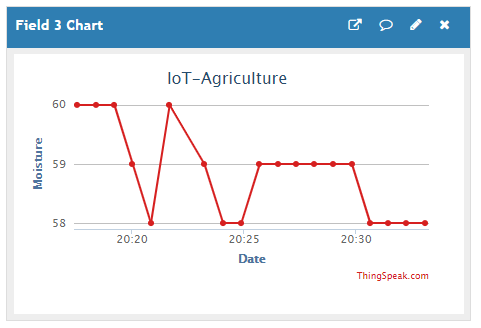
Finally you can connect the ESP as per shown in the circuit. Note, do note connect RST and GPIO0 to ground. Leave them to float.
Use this as final program:
#include <math.h> #include <dht.h> #include <SoftwareSerial.h> SoftwareSerial mySerial(10, 11); //(Tx,Rx) dht DHT; #define DHTxxPIN 5 int op_value;
int ack; void setup() { Serial.begin(9600); mySerial.begin(115200); } void loop() { ack = 0; int chk = DHT.read11(DHTxxPIN); switch (chk) { case DHTLIB_ERROR_CONNECT: ack = 1; break; } if (ack == 0) { Serial.print("Temperature(*C) = "); Serial.println(DHT.temperature); Serial.print("Humidity(%) = "); Serial.println(DHT.humidity); op_value = analogRead(A0); op_value = map(op_value,550,0,0,100); Serial.print("Moisture(%): "); Serial.print(op_value); Serial.print("%"); Serial.println("\n ------------------------- \n"); //------Sending Data to ESP8266--------// mySerial.print('*'); // Starting char mySerial.print(round(DHT.temperature)); //2 digit data mySerial.print(round(DHT.humidity)); //2 digit data mySerial.print(op_value); //2 digit data mySerial.println('#'); // Ending char //------------------------------------// delay(2000); } if (ack == 1) { Serial.print("NO DATA"); Serial.print("\n\n"); delay(2000); } }
You can then see the data being displayed on ThingSpeak as well.
Final Circuit connections will look like this:
DHT11
- Datapin-->pin5
- Vcc------->5V
- GND------>GND
Soil moisture Sensor
- A0-------->A0
- Vcc-------->5V
- GND------->GND
ESP8266
- 3.3V------->3.3V
- EN--------->3.3V
- GND------>GND
- Rx--------->pin10
- Tx--------->pin11
- RST------->disconnected
- GPIO0----->disconnected
- GPIO2------>disconnected
You can find a link to the Program files here:
- ESP8266 Programming: https://create.arduino.cc/projecthub/code_files/53...
- Arduino Program: https://create.arduino.cc/projecthub/code_files/53...