STM32F4 Discovery Board and Python USART Communication (STM32CubeMx)
by BurhanMuhyiddin in Circuits > Microcontrollers
4777 Views, 1 Favorites, 0 Comments
STM32F4 Discovery Board and Python USART Communication (STM32CubeMx)
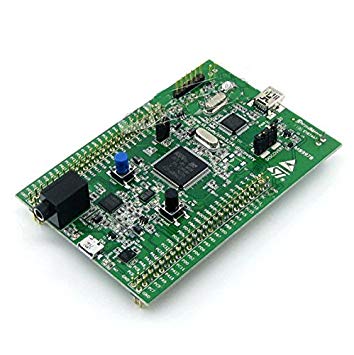
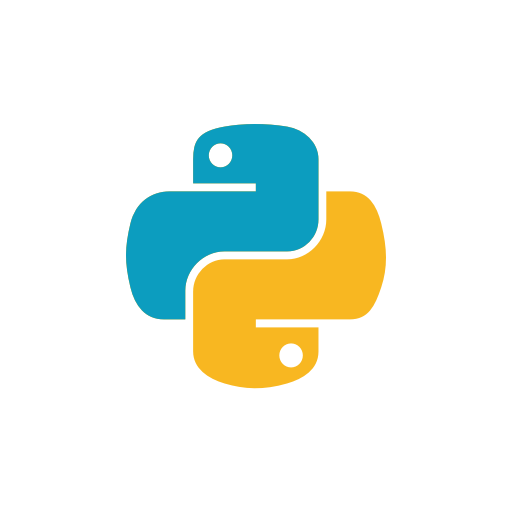
Hi! In this tutorial we will try to establish USART communication between STM32F4 ARM MCU and Python (it can be replaced by any other language). So, let's get started :)
Software and Hardware Requirements
In terms of hardware you need:
- STM32F4 Discovery Board (or any other STM32 board)
- USB to TTL converter
In terms of software:
- STM32CubeMX
- Keil uVision5
- Python with serial library installed
STM32CubeMX Configuration
First let's understand what we want to do. We want to transmit data to board from Python over USART and check if we have correct data and toggle led. So, we need enable USART and Led.
- Enable USART2 from Connectivity tab.
- Change mode to Asynchoronous
- Baud rate to 9600 Bits/s
- Word length to 8 Bits without parity
- No parity bit
- From DMA settings add USART2_RX in cicular mode
- From NVIC Settings enable USART2 global interrupt
- Enable LED by clicking on PD12
Then generate code :)
Keil Software Development
#include <stdbool.h>
#include <string.h>
These libraries will be needed in string operations and to define boolean variable.
/* USER CODE BEGIN 2 */
HAL_UART_Receive_DMA(&huart2, (uint8_t *) data_buffer, 1); /* USER CODE END 2 */
Here, UART receive with DMA started.
/* USER CODE BEGIN 4 */
void HAL_UART_RxCpltCallback(UART_HandleTypeDef *huart) { /* Prevent unused argument(s) compilation warning */ UNUSED(huart); /* NOTE: This function should not be modified, when the callback is needed, the HAL_UART_RxCpltCallback could be implemented in the user file */ if(data_buffer[0] != '\n'){ data_full[index_] = data_buffer[0]; index_++; }else{ index_ = 0; finished = 1; } //HAL_UART_Transmit(&huart2, data_buffer, 1, 10); } /* USER CODE END 4 */
This is ISR which is activated when we get one byte of character. So. we get that byte and write it to the data_full which contains the full received data until we get '\n'. When we get '\n' we make finished flag 1 and in while loop:
while (1)
{ /* USER CODE END WHILE */ if(finished){ if(strcmp(data_full, cmp_) == 0){ HAL_GPIO_TogglePin(GPIOD, GPIO_PIN_12); } memset(data_full,'\0',strlen(data_full)); finished = 0; }else{ __NOP (); } /* USER CODE BEGIN 3 */ }
If finished flag is HIGH we compare the contents of full received data and data we desire and if they are equal we toggle led. After that we clear finished flag and wait for new data and also clear the data_full array in order not to overwrite to the array.
Python Software Development
So, here we want to send our number with '/n' at the end, because Keil software will need to see it in order to know the end.
import serial
ser = serial.Serial('COM17') #check that port on your device from Device Manager
ser.write(b'24\n')
You should see that LED toggles each time you send '24\n'. If you send anything else it should not affect it.
Conclusion
We have reached the end of the tutorial. if you have any problem or question please don't hesitate to ask. I will try to help as much as I can. Thank you very much :)