RTC Time Synchronization With NTP ESP8266
by Manodeep in Circuits > Arduino
9211 Views, 1 Favorites, 0 Comments
RTC Time Synchronization With NTP ESP8266
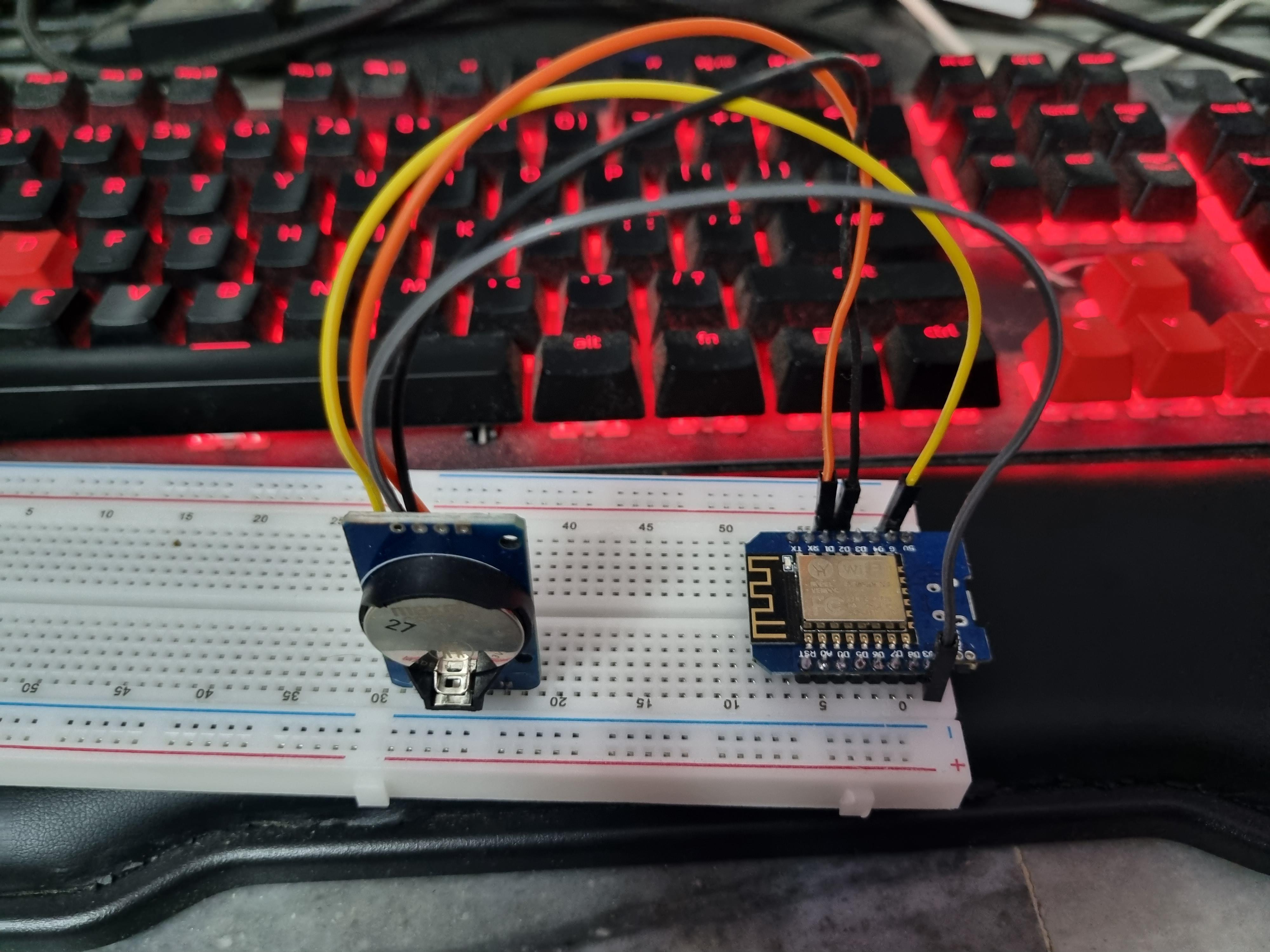
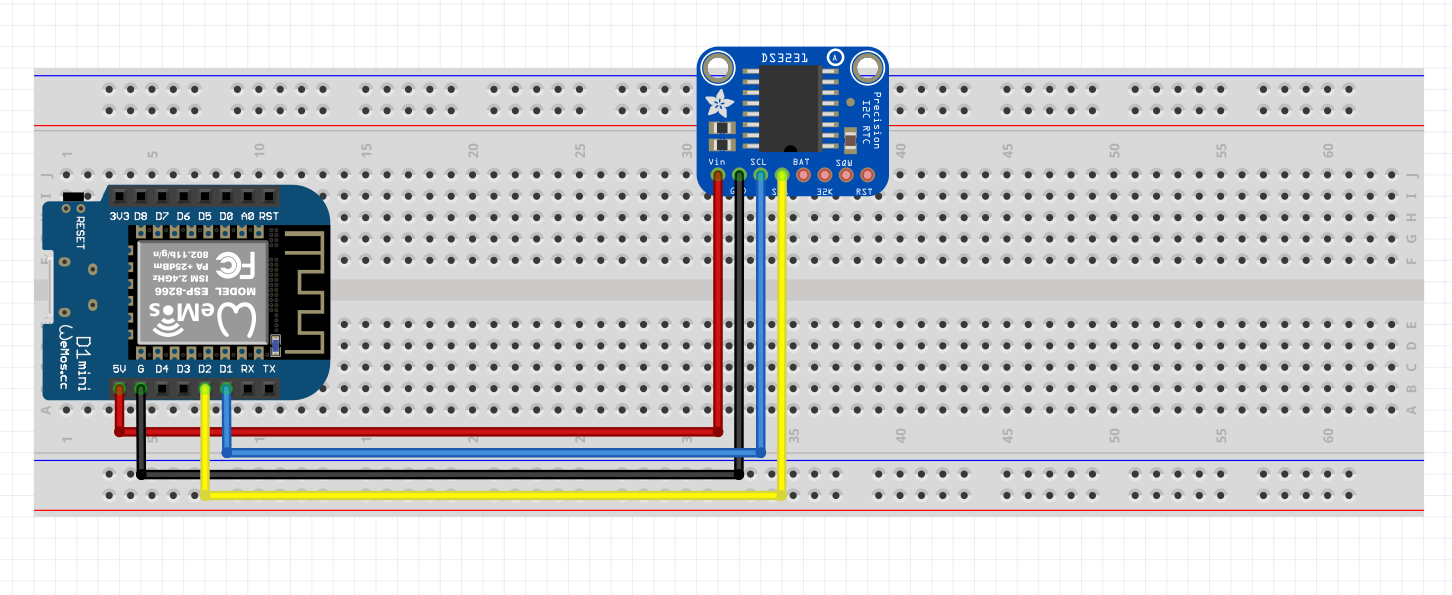
This project demonstrates how to synchronize the real-time clock (RTC) module with Network Time Protocol (NTP) to maintain accurate timekeeping. The code compares the time obtained from NTP with the RTC time and provides synchronization status. It is designed for use with an ESP8266-based board, such as NodeMCU, and a DS3231 RTC module.
Supplies
To run this code, you will need the following supplies:
- ESP8266-based development board (e.g., Wemos D1 Mini)
- DS3231 RTC module
- Jumper wires
- USB cable for the ESP8266 board
- Computer with Arduino IDE installed
- WiFi network with internet access
Hardware Setup
- Connect the ESP8266-based board and the DS3231 RTC module using jumper wires. Ensure the following connections:
- ESP8266 SDA (D2) to DS3231 SDA
- ESP8266 SCL (D1) to DS3231 SCL
- ESP8266 5V to DS3231 VCC
- ESP8266 GND to DS3231 GND
- Connect the ESP8266 board to your computer using a USB cable.
Library Installation
- Open the Arduino IDE on your computer.
- Go to Sketch -> Include Library -> Manage Libraries.
- In the Library Manager, search for the following libraries and install them if not already installed:
- RTClib by Adafruit
- ESP8266WiFi by ESP8266 Community
- WiFiUdp by bportaluri
- NTPClient by Fabrice Weinberg
- TimeLib by Paul Stoffregen
Code Configuration
- Copy the provided code and paste it into the Arduino IDE.
- Modify the following variables in the setup() function to match your WiFi network credentials:
- ssid: Your WiFi network SSID.
- password: Your WiFi network password.
- If necessary, you can change the NTP server in the NTPClient declaration. The default server used is "asia.pool.ntp.org".
#include <RTClib.h>
#include <Wire.h>
#include <ESP8266WiFi.h>
#include <WiFiUdp.h>
#include <NTPClient.h>
#include <TimeLib.h>
RTC_DS3231 rtc;
WiFiUDP ntpUDP;
NTPClient timeClient(ntpUDP, "asia.pool.ntp.org", 19800, 60000);
char t[32];
byte last_second, second_, minute_, hour_, day_, month_;
int year_;
void setup()
{
Serial.begin(9600); // Initialize serial communication with a baud rate of 9600
Wire.begin(); // Begin I2C communication
rtc.begin(); // Initialize DS3231 RTC module
// Connect to WiFi
char* ssid = "SSID"; // Replace with your WiFi SSID
char* password = "PASSWORD"; // Replace with your WiFi password
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED)
{
delay(500);
Serial.print(".");
}
Serial.println("Connected to WiFi");
timeClient.begin(); // Start NTP client
timeClient.update(); // Retrieve current epoch time from NTP server
unsigned long unix_epoch = timeClient.getEpochTime(); // Get epoch time
rtc.adjust(DateTime(unix_epoch)); // Set RTC time using NTP epoch time
DateTime now = rtc.now(); // Get initial time from RTC
last_second = now.second(); // Store initial second
}
void loop()
{
timeClient.update(); // Update time from NTP server
unsigned long unix_epoch = timeClient.getEpochTime(); // Get current epoch time
second_ = second(unix_epoch); // Extract second from epoch time
if (last_second != second_)
{
minute_ = minute(unix_epoch); // Extract minute from epoch time
hour_ = hour(unix_epoch); // Extract hour from epoch time
day_ = day(unix_epoch); // Extract day from epoch time
month_ = month(unix_epoch); // Extract month from epoch time
year_ = year(unix_epoch); // Extract year from epoch time
// Format and print NTP time on Serial monitor
sprintf(t, "NTP Time: %02d:%02d:%02d %02d/%02d/%02d", hour_, minute_, second_, day_, month_, year_);
Serial.println(t);
DateTime rtcTime = rtc.now(); // Get current time from RTC
// Format and print RTC time on Serial monitor
sprintf(t, "RTC Time: %02d:%02d:%02d %02d/%02d/%02d", rtcTime.hour(), rtcTime.minute(), rtcTime.second(), rtcTime.day(), rtcTime.month(), rtcTime.year());
Serial.println(t);
// Compare NTP time with RTC time
if (rtcTime == DateTime(year_, month_, day_, hour_, minute_, second_))
{
Serial.println("Time is synchronized!"); // Print synchronization status
}
else
{
Serial.println("Time is not synchronized!"); // Print synchronization status
}
last_second = second_; // Update last second
}
delay(1000); // Delay for 1 second before the next iteration
}
Uploading and Running the Code
- Select the correct board and port from the Tools menu in the Arduino IDE.
- Click the Upload button to compile and upload the code to the ESP8266 board.
- Open the Serial monitor by clicking the magnifying glass icon in the Arduino IDE's toolbar or going to Tools -> Serial Monitor.
- Set the baud rate of the Serial monitor to 9600.
- You should see the Serial monitor displaying the NTP time, RTC time, and synchronization status.
- If the NTP time matches the RTC time, it will print "Time is synchronized!".
- If the NTP time does not match the RTC time, it will print "Time is not synchronized!".
- Ensure that the DS3231 RTC module is properly connected and functioning.
Conclusion
The provided code allows you to synchronize the DS3231 RTC module with NTP for accurate timekeeping. By comparing the NTP time with the RTC time, you can determine the synchronization status. Follow the detailed steps to set up and run the code on your ESP8266-based board. Feel free to modify the code according to your specific requirements or expand its functionality as needed.