Python Guessing Game
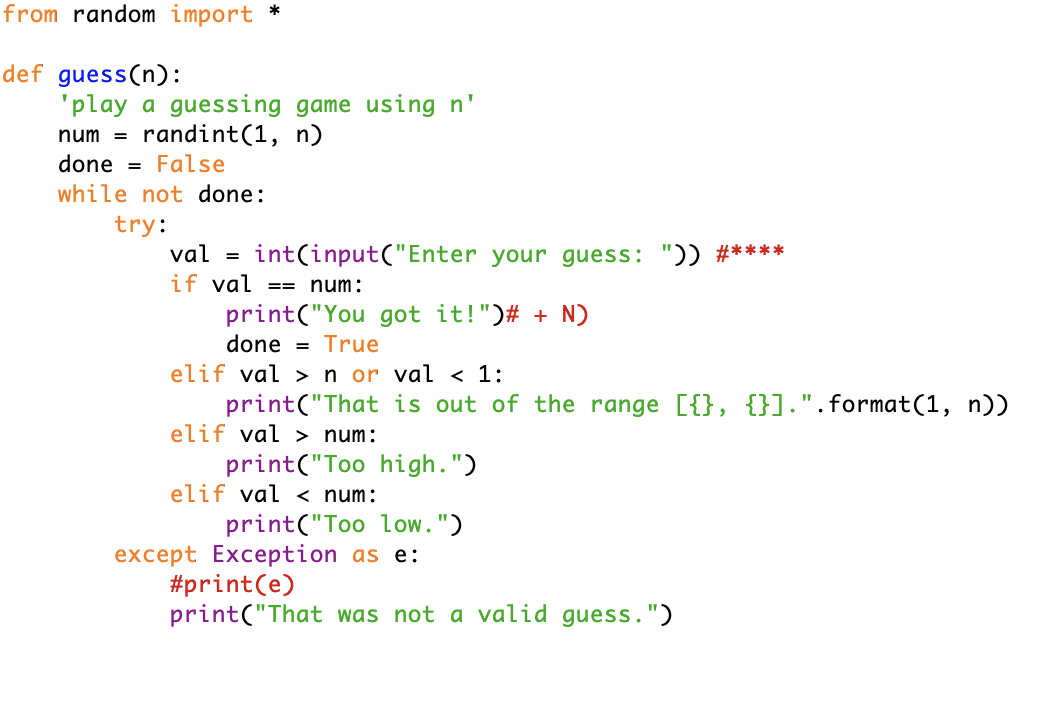
The following instructions provide step by step guidance on how to write a program using the random module and creating a guessing game that interacts with the user. To get started you would need to have python installed on your computer and also open a separate file that you would write the code which you will eventually run in the python window.
Random Import Statement
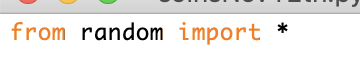
Before starting with the code for the program make an import statement on the top which says from random import * this will allow you to use some functions of the random module without having to right random in front of those functions
Function Name

Start by creating the function name and then make it have one variable which will be the number that the random guess will stop at.
Generate a Random Number

Next what we need to do is generate a random number from 1 to n with the randit function (where n is the number the user put in when calling the function) and store it into a variable.
Starting the While Loop

Then make a while loop that stops when it’s False. So, assign done to False and then make the while loop to go until done isn’t False.
User Input

When in the while loop make an input statement for the users and then store it into a variable. Make sure to turn what the user inputs into an integer.
Exiting the While Loop of the User Guessed Correctly
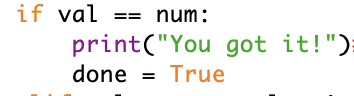
When the user guesses the right number make them get out of the while loop by assigning done to True.
If/else Statements
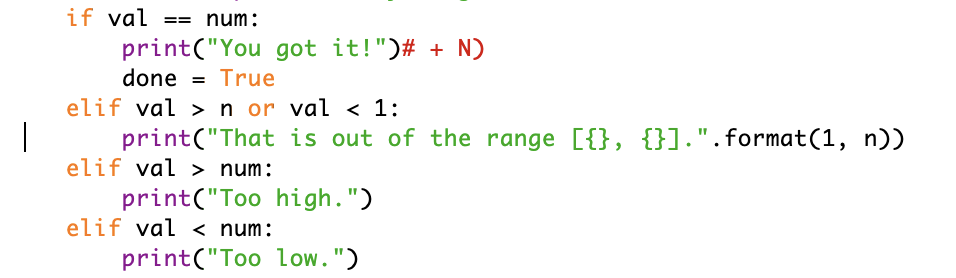
After that make if/elif statements that will print a message that is relevant to what the user guessed.
Try/except Statements
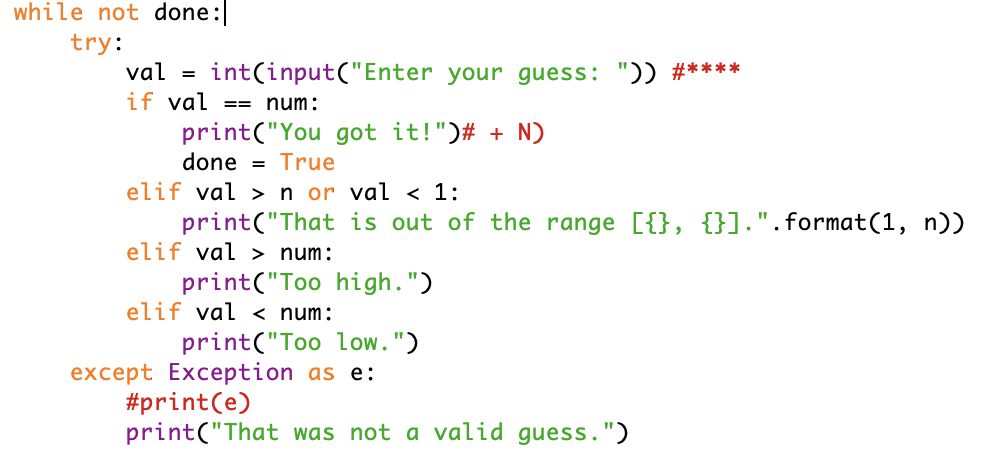
Lastly, put a try/except inside the while loop that will print a message when the user inputs an invalid guess.
Congratulations
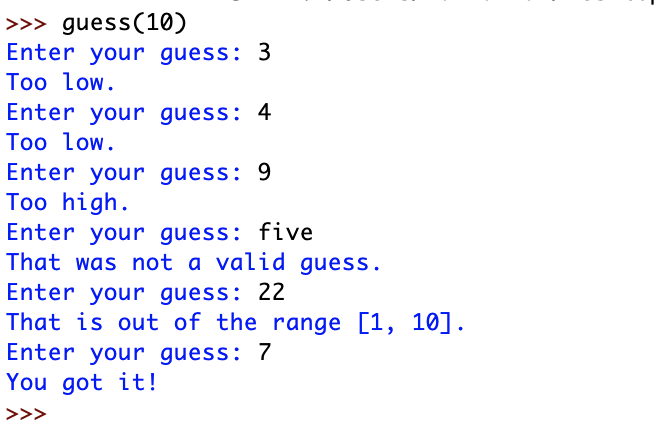
You are now all done with the guessing game and you are ready to test it! This is how it’s supposed to work.
This was a program that interacts with the user and makes the guess a number until they get to the number that was randomly generated by the computer! Enjoy!