PWM With ESP32 | Dimming LED With PWM on ESP 32 With Arduino IDE
by Utsource in Circuits > Arduino
12891 Views, 1 Favorites, 0 Comments
PWM With ESP32 | Dimming LED With PWM on ESP 32 With Arduino IDE
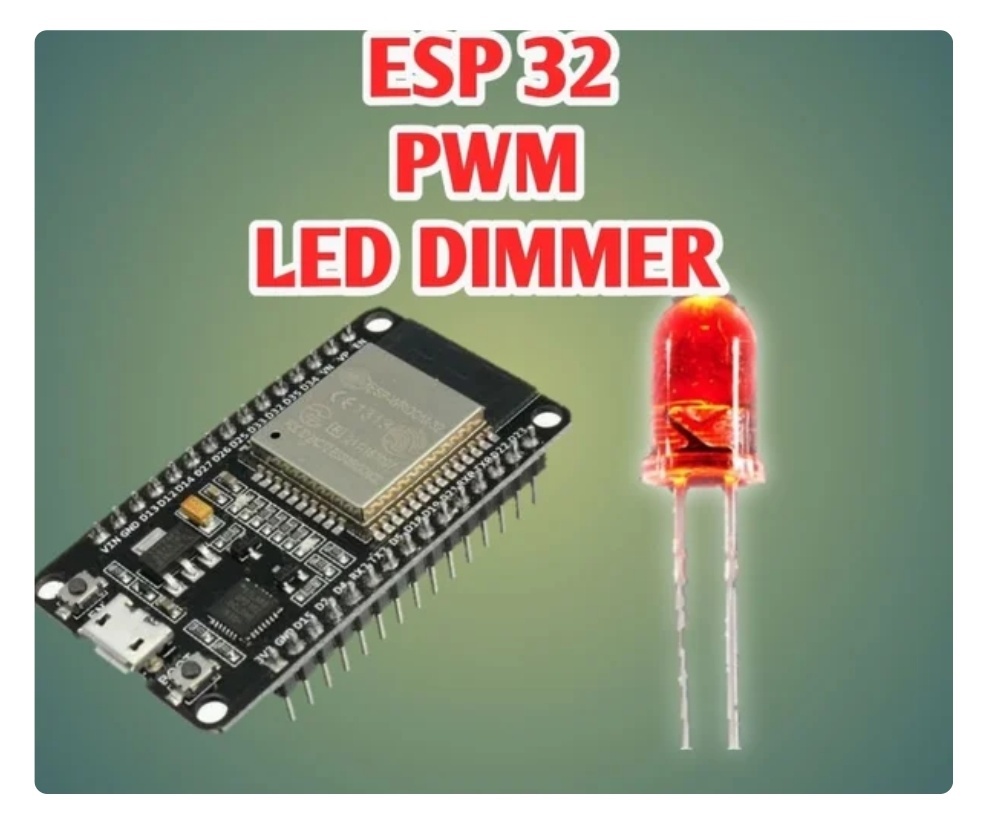
In this instructables we will see how to generate PWM signals with ESP32 using Arduino IDE & PWM is basically used to generate analog output from any MCU and that analog output could be anything between 0V to 3.3V ( in case of esp32 ) & from 0V to 5V ( in case of arduino uno) and these PWM signals (analog output) are used to dim (variable output, lighting the LED at different brightness) the LED.
Things You Need
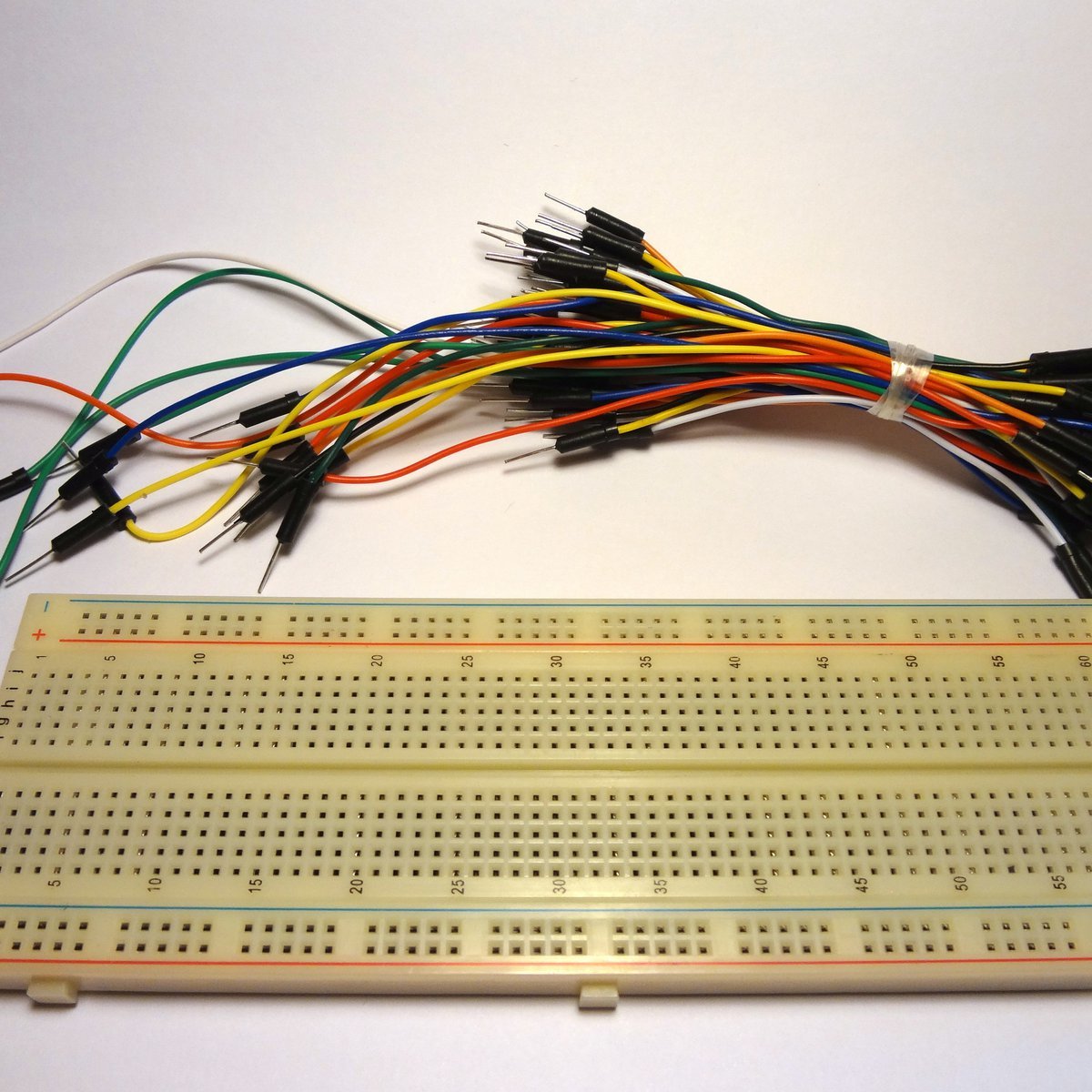
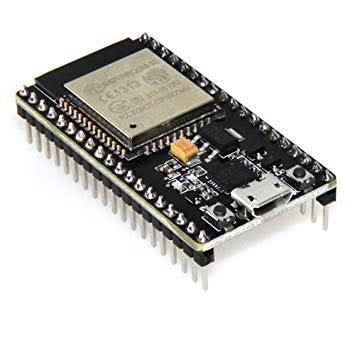.jpg)
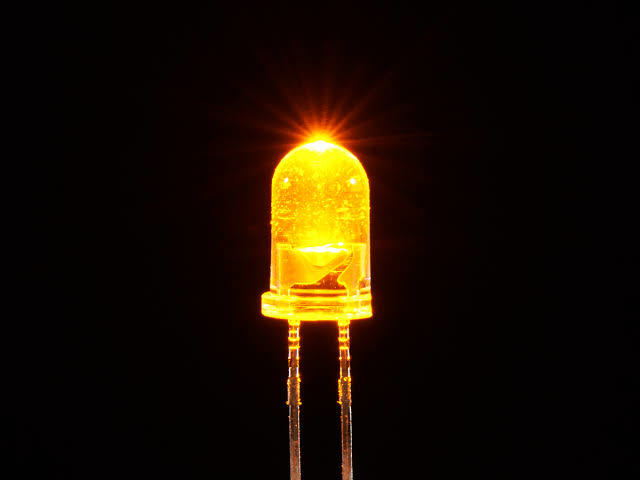.jpg)
For this tutorial you will need following things :
ESP32
Understanding the PWM on ESP32
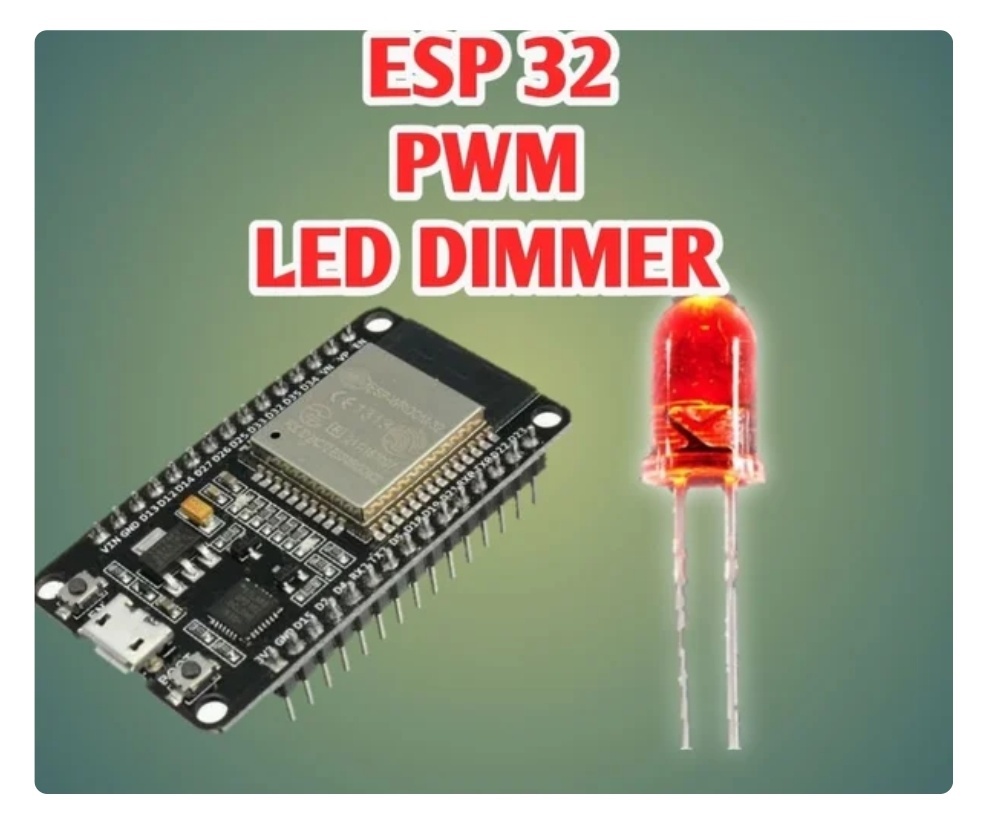
ESP32 has 16 Channel PWM controller and these 16 Channels are independent and can be configured independently to get PWM signals with different properties for different requirements.
Before going through the code & all the process you need to know following things :
>> there are 16 ( 0 to 15) pwm Channels in a ESP32. You need to choose your PWM channel.
>>After this we need to select the frequency for PWM, we can go for 5000hz.
>>Here we have resolution of 1 to 16bits in ESP32 but for this tutorial we will go for 8 bit only which means brightness will be controlled by values 0 to 255.
>>You need to setup the LED for PWM to do that you need to use following line of code and in that you need to mention about the ledchannel (we are using channel 0 of ESP32) you are using for PWM & freq is the frequency (we are using 5000hz) of PWM & resolution you are using ( we are using 8bit resolution).
ledcSetup(ledChannel, freq, resolution);
Values in our case : const int freq = 5000;
const int ledChannel = 0;
const int resolution = 8;
>>then mention which LED pin you need to by using the following command :
ledcAttachPin(ledPin, ledChannel);
- here ledPin is the pin no. Which we will be using & ledChannel is the channel which we have to select for PWM.
5. Finally, to control the LED brightness using PWM, you use the following function:
>>the main important part of the code will be the following command which will write the analog output to LED pin :
ledcWrite(ledChannel, dutycycle);
this above command needs 'ledChannel' & 'dutyCycle' where channel is the channel number we will be using and duty cycle is the value we are writing as output to LED pin.
Before going through the code & all the process you need to know following things :
>> there are 16 ( 0 to 15) pwm Channels in a ESP32. You need to choose your PWM channel.
>>After this we need to select the frequency for PWM, we can go for 5000hz.
>>Here we have resolution of 1 to 16bits in ESP32 but for this tutorial we will go for 8 bit only which means brightness will be controlled by values 0 to 255.
>>You need to setup the LED for PWM to do that you need to use following line of code and in that you need to mention about the ledchannel (we are using channel 0 of ESP32) you are using for PWM & freq is the frequency (we are using 5000hz) of PWM & resolution you are using ( we are using 8bit resolution).
ledcSetup(ledChannel, freq, resolution);
Values in our case : const int freq = 5000;
const int ledChannel = 0;
const int resolution = 8;
>>then mention which LED pin you need to by using the following command :
ledcAttachPin(ledPin, ledChannel);
- here ledPin is the pin no. Which we will be using & ledChannel is the channel which we have to select for PWM.
5. Finally, to control the LED brightness using PWM, you use the following function:
>>the main important part of the code will be the following command which will write the analog output to LED pin :
ledcWrite(ledChannel, dutycycle);
this above command needs 'ledChannel' & 'dutyCycle' where channel is the channel number we will be using and duty cycle is the value we are writing as output to LED pin.
Connections
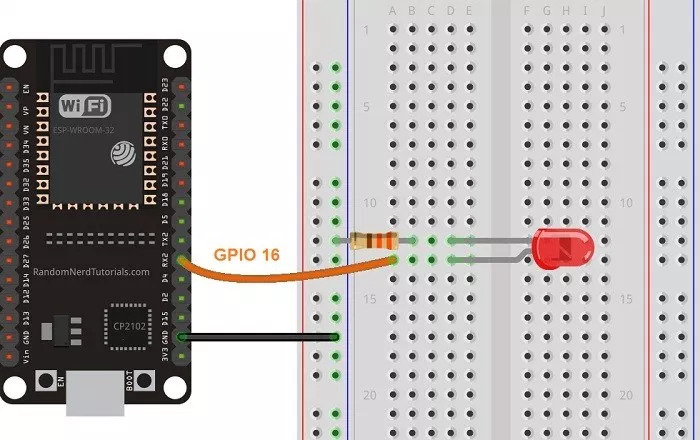.jpg)
The connection part is very easy.
You need to connect a LED with Resistor to GPIO16 as shown in schmatics.
You need to connect a LED with Resistor to GPIO16 as shown in schmatics.
Install ESP32 BOARDS in Arduino IDE
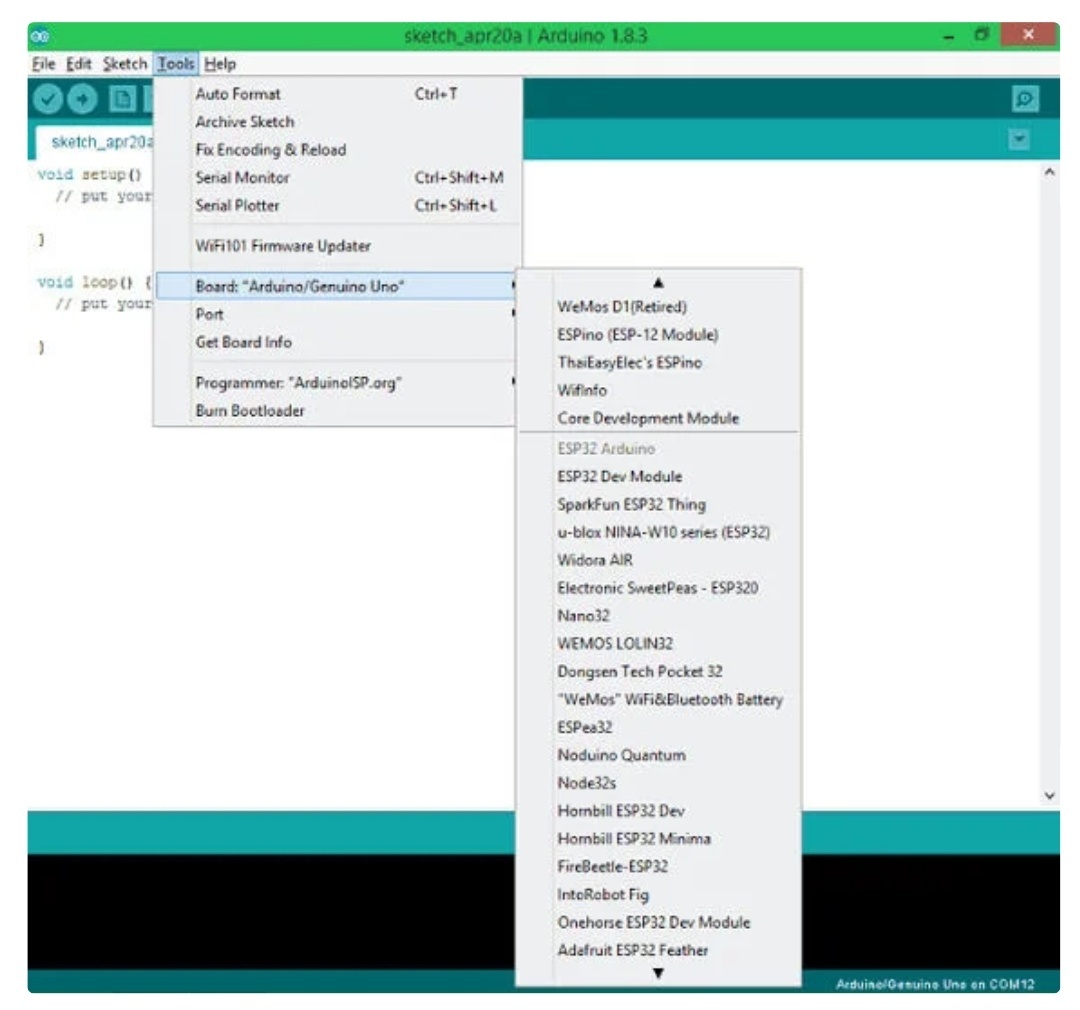
Make sure you have Arduino IDE in your PC and you installed ESP32 Boards in your Arduino IDE, and if it is not the case please follow the following instructables of mine to install it. : https://www.instructables.com/id/Getting-Started-...
Code
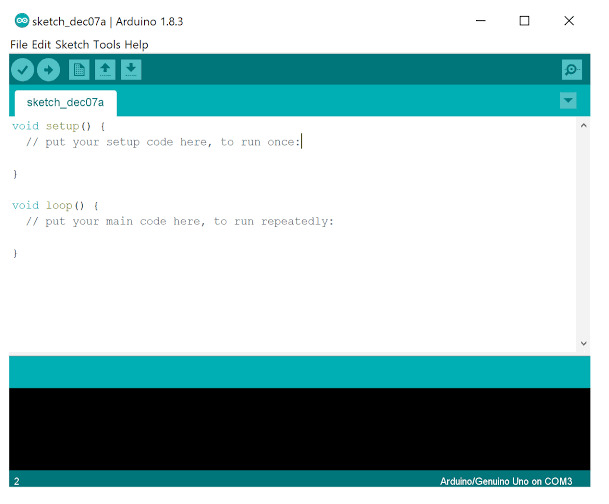.jpg)
Please copy the following code and upload it to your ESP32 :
// the number of the LED pin
const int ledPin = 16; // 16 corresponds to GPIO16
// setting PWM properties
const int freq = 5000;
const int ledChannel = 0;
const int resolution = 8;
void setup(){
// configure LED PWM functionalitites
ledcSetup(ledChannel, freq, resolution);
// attach the channel to the GPIO to be controlled
ledcAttachPin(ledPin, ledChannel);
}
void loop(){
// increase the LED brightness
for(int dutyCycle = 0; dutyCycle <= 255; dutyCycle++){
// changing the LED brightness with PWM
ledcWrite(ledChannel, dutyCycle);
delay(15);
}
// decrease the LED brightness
for(int dutyCycle = 255; dutyCycle >= 0; dutyCycle--){
// changing the LED brightness with PWM
ledcWrite(ledChannel, dutyCycle);
delay(15);
}
}
// the number of the LED pin
const int ledPin = 16; // 16 corresponds to GPIO16
// setting PWM properties
const int freq = 5000;
const int ledChannel = 0;
const int resolution = 8;
void setup(){
// configure LED PWM functionalitites
ledcSetup(ledChannel, freq, resolution);
// attach the channel to the GPIO to be controlled
ledcAttachPin(ledPin, ledChannel);
}
void loop(){
// increase the LED brightness
for(int dutyCycle = 0; dutyCycle <= 255; dutyCycle++){
// changing the LED brightness with PWM
ledcWrite(ledChannel, dutyCycle);
delay(15);
}
// decrease the LED brightness
for(int dutyCycle = 255; dutyCycle >= 0; dutyCycle--){
// changing the LED brightness with PWM
ledcWrite(ledChannel, dutyCycle);
delay(15);
}
}
Testing the PWM Functionality
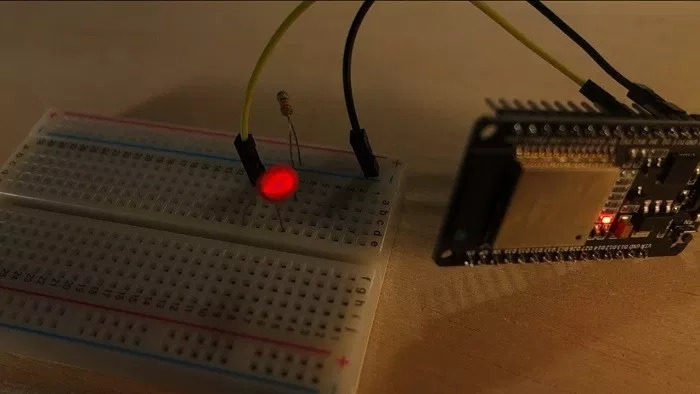.jpg)
After uploading the code you will see your LEDs intensity changing so that takes us to the end of this instructables.
Have fun using PWM with ESP32 in your projects.
Have fun using PWM with ESP32 in your projects.