MicroPython on SPIKE Prime
by CEEOInnovations in Circuits > Microcontrollers
15478 Views, 3 Favorites, 0 Comments
MicroPython on SPIKE Prime
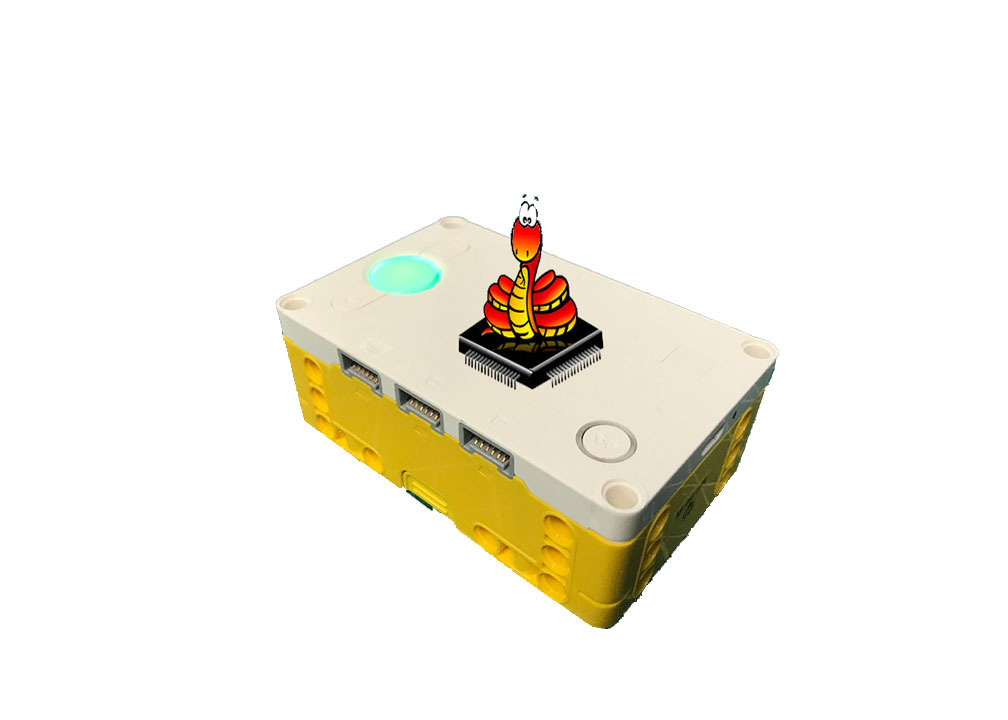
You can code SPIKE Prime using MicroPython which is a subset of python for small microprocessors.
You can use any terminal emulator to code the SPIKE Prime hub.
Supplies
SPIKE Prime hub
Computer with USB Port / bluetooth
USB cable to connect the hub to the computer
Grab a Terminal Emulator
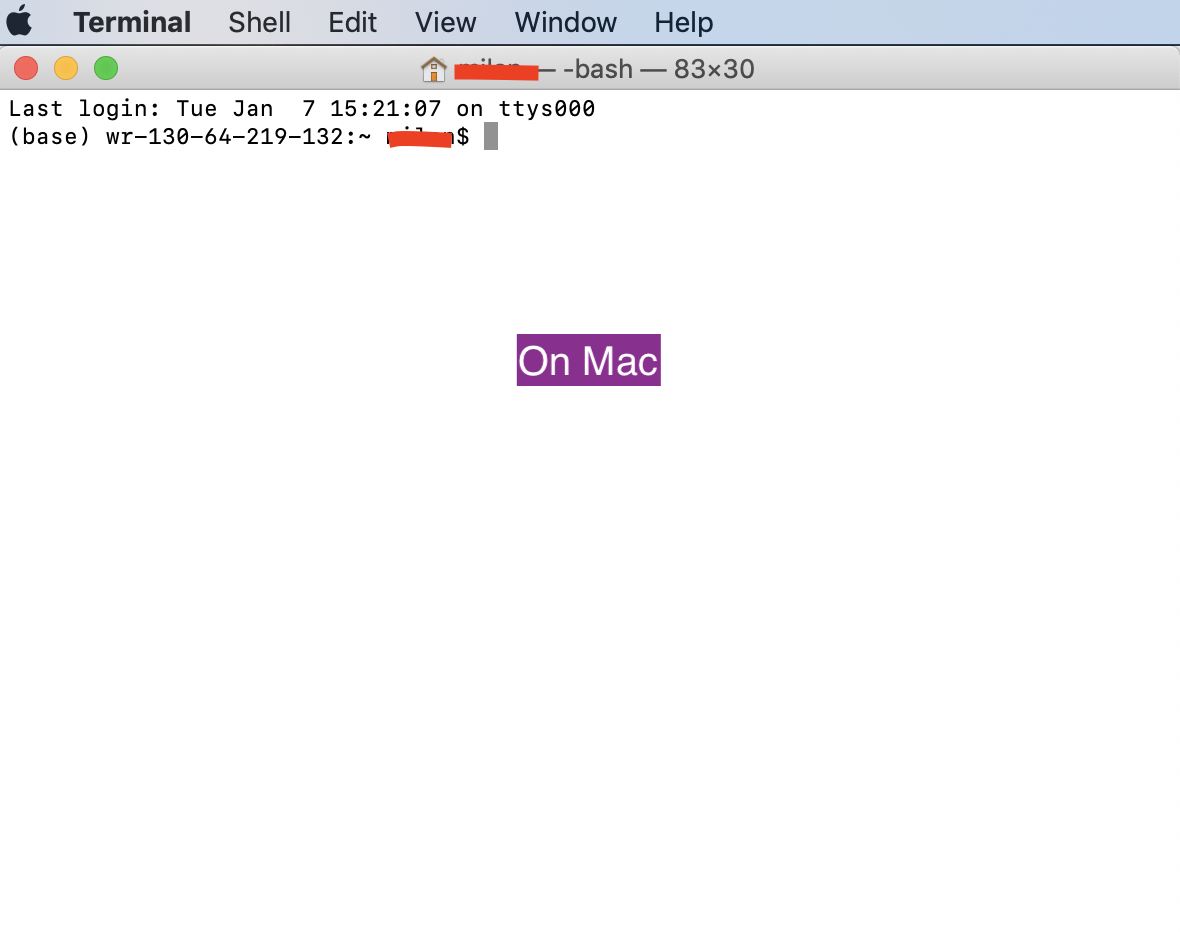
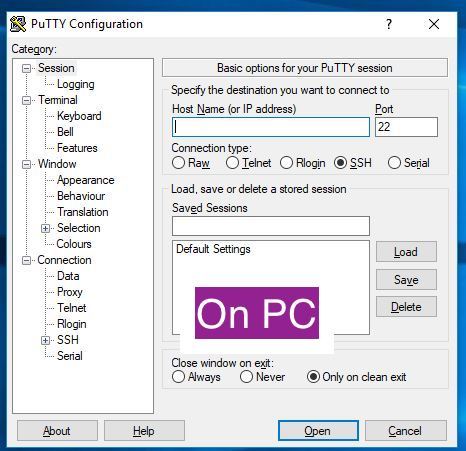
Connect SPIKE Prime to the USB Port
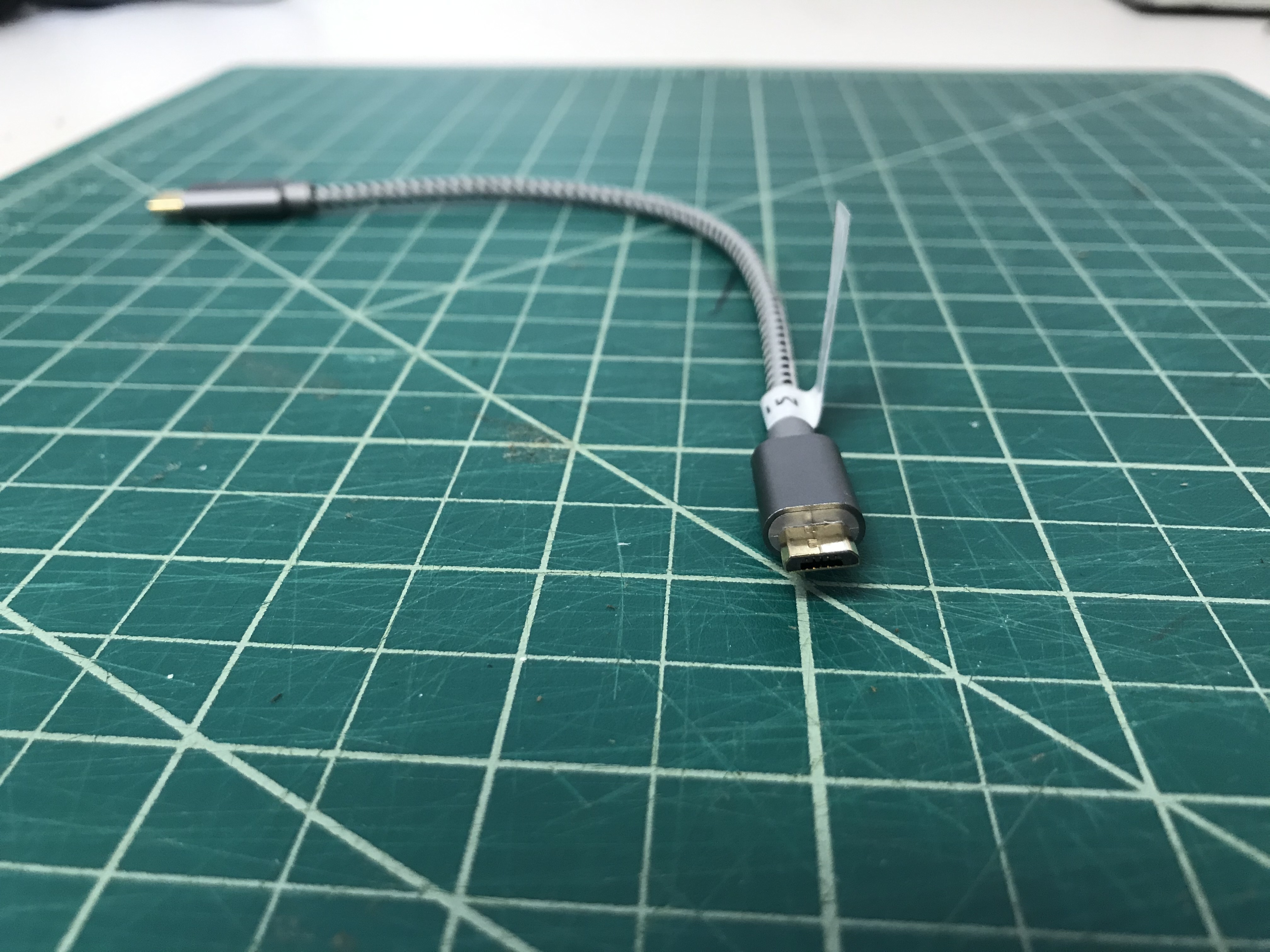
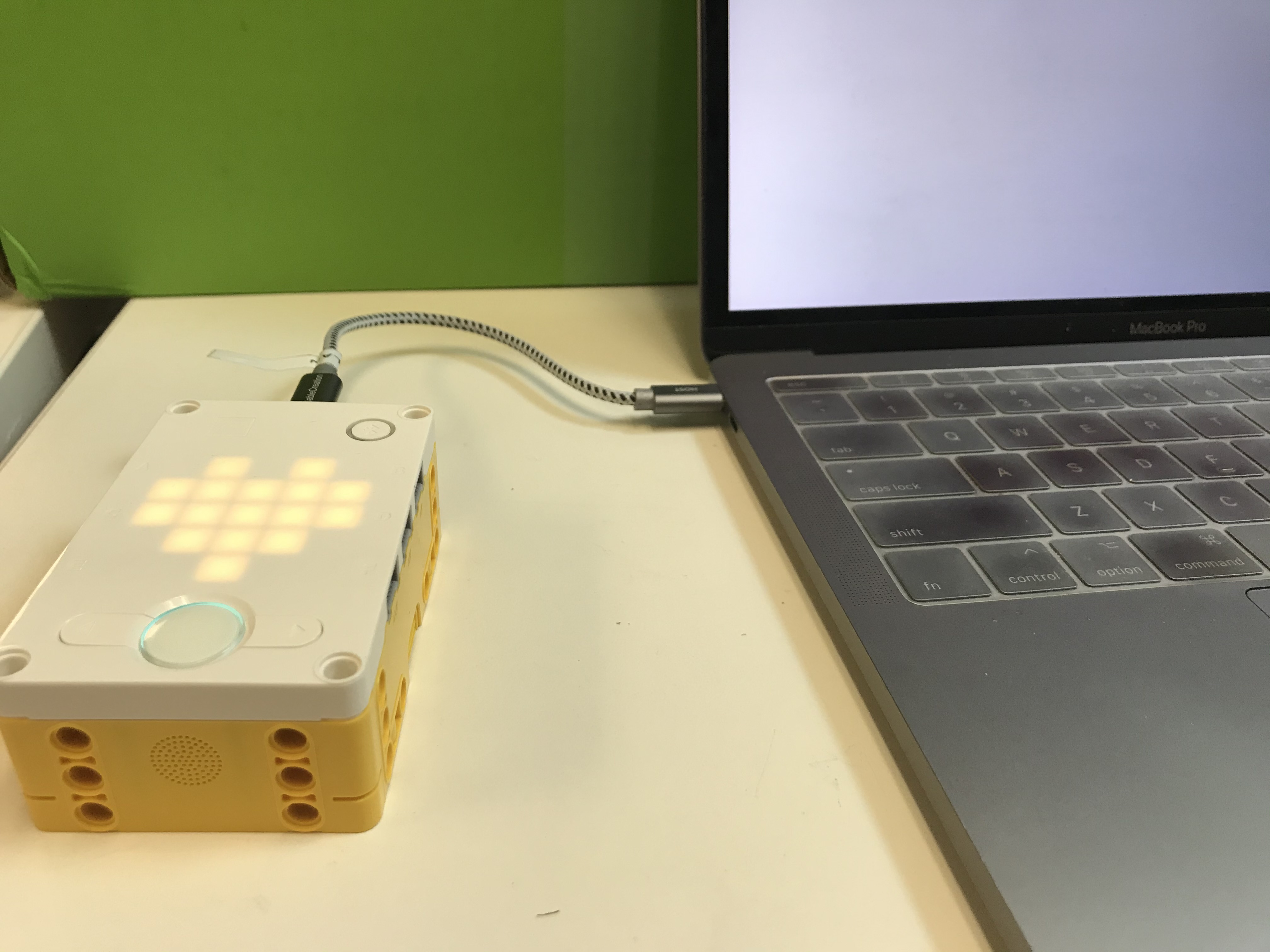
Use a microUSB cable to connect the SPIKE Prime to the computer.
Find the Port
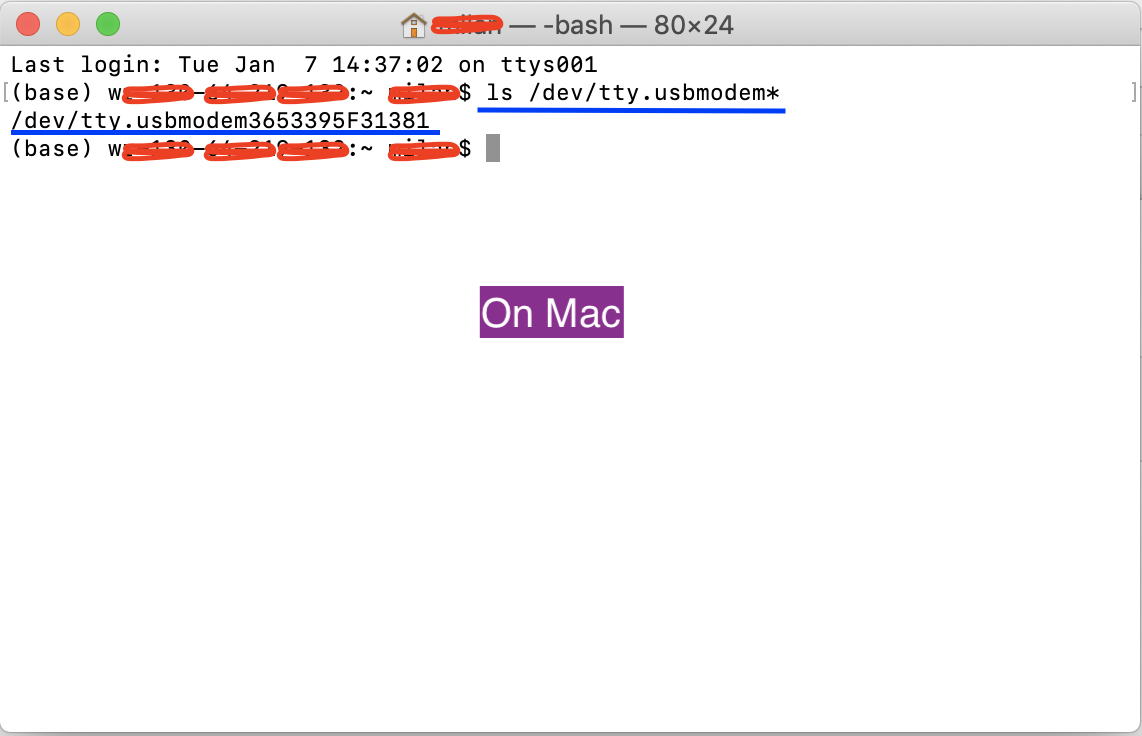
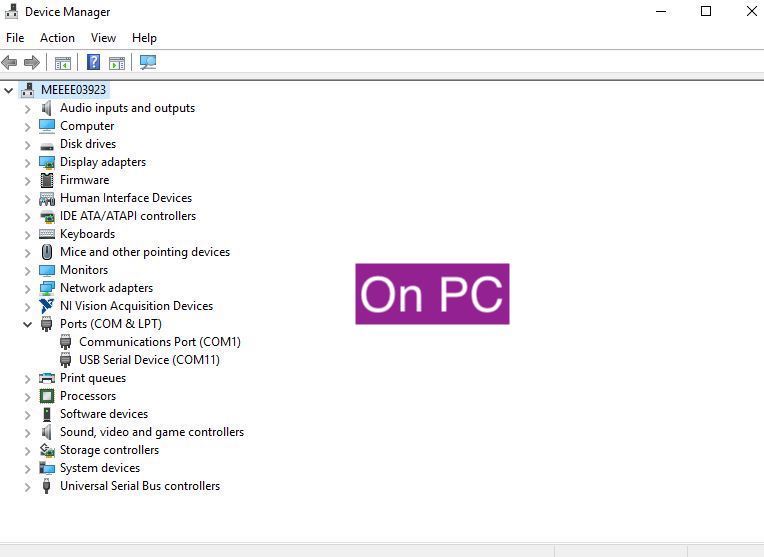
We need to know what serial port the SPIKE Prime hub is connected to.
On mac, type
ls /dev/tty.usbmodem*
On a pc, look in your device manager under serial to see what serial ports you have connected
On pi, it will be something like ttyAMC0 - check in your /dev/folder
Connect Up
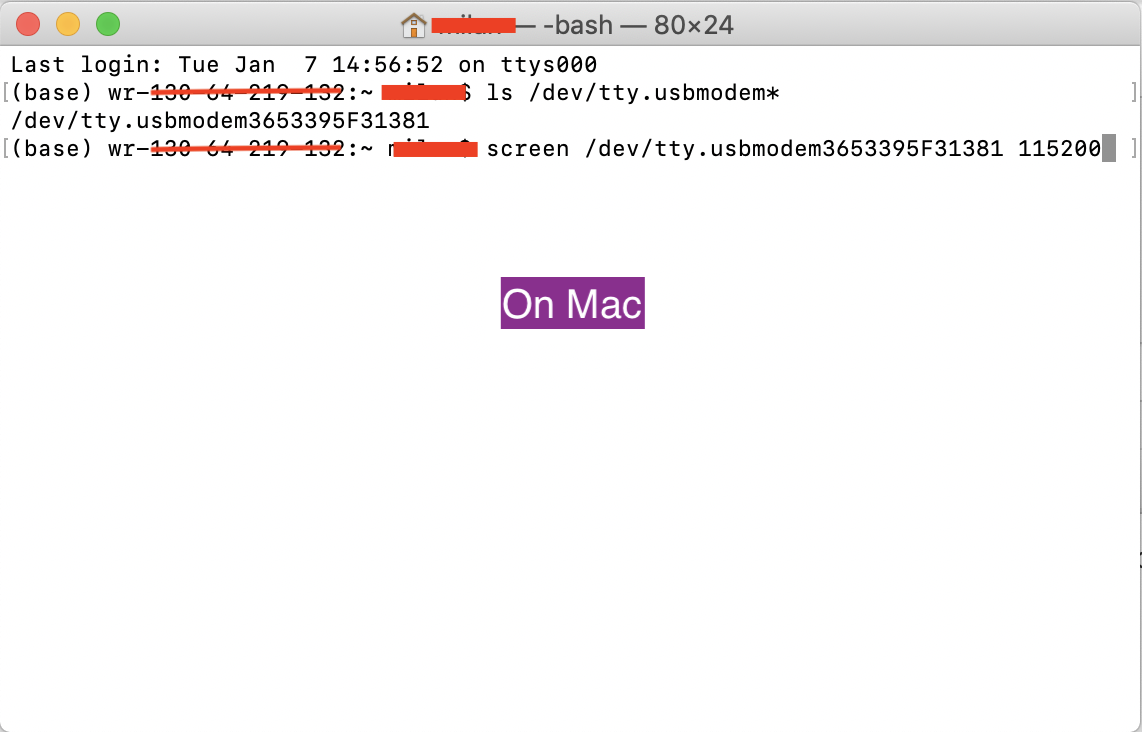
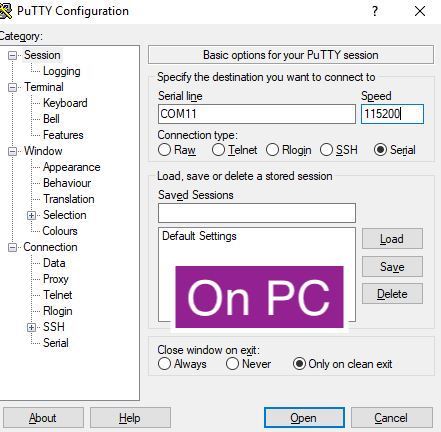
Connect up to the right port (from previous step) at 115200 baud
In Terminal, type
usercomputer$ screen /dev/<portname> 115200
In other IDE ,
hit Open/ connect (after setting up the ports and baudrates)
Note: no parity, 8 data bits, and 1 stop bit
Starting the REPL
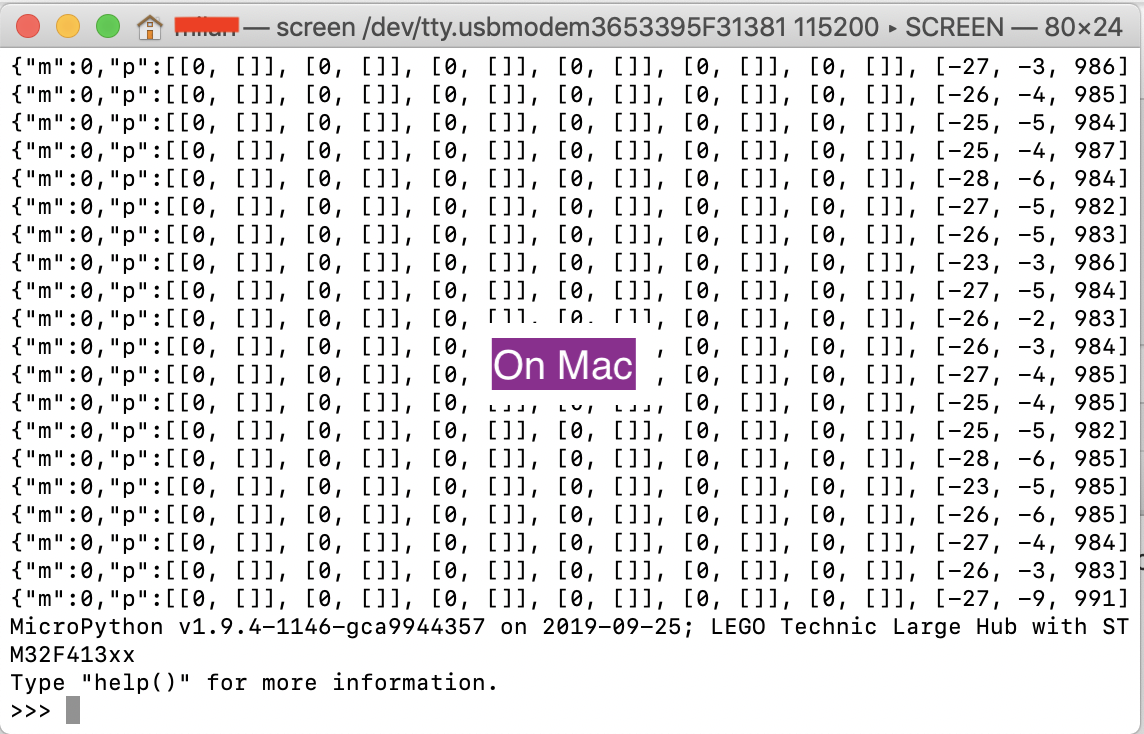
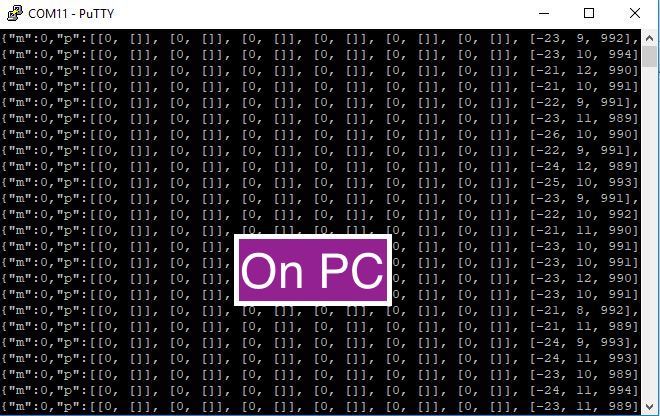
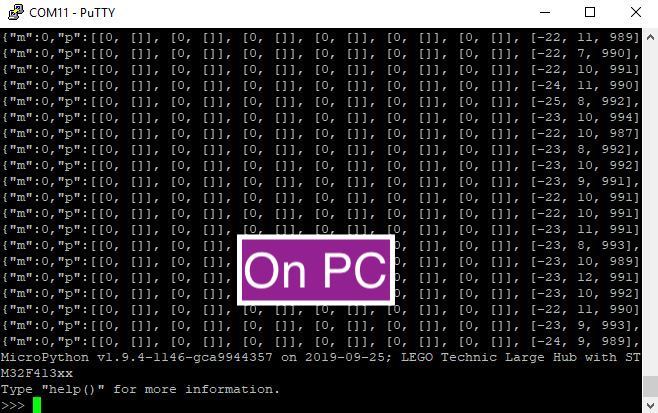
When you connect to the SPIKE Prime from the terminal/ PUTTY you will see a stream of numbers and characters. Those are data from internal sensors of SPIKE Prime hub. To begin press control + c
It will interrupt the serial port and you should see something like this.
MicroPython v1.9.4-1146-gca9944357 on 2019-09-25; LEGO Technic Large Hub with STM32F413xx Type "help()" for more information.
Now you are ready to code.
Your First Code
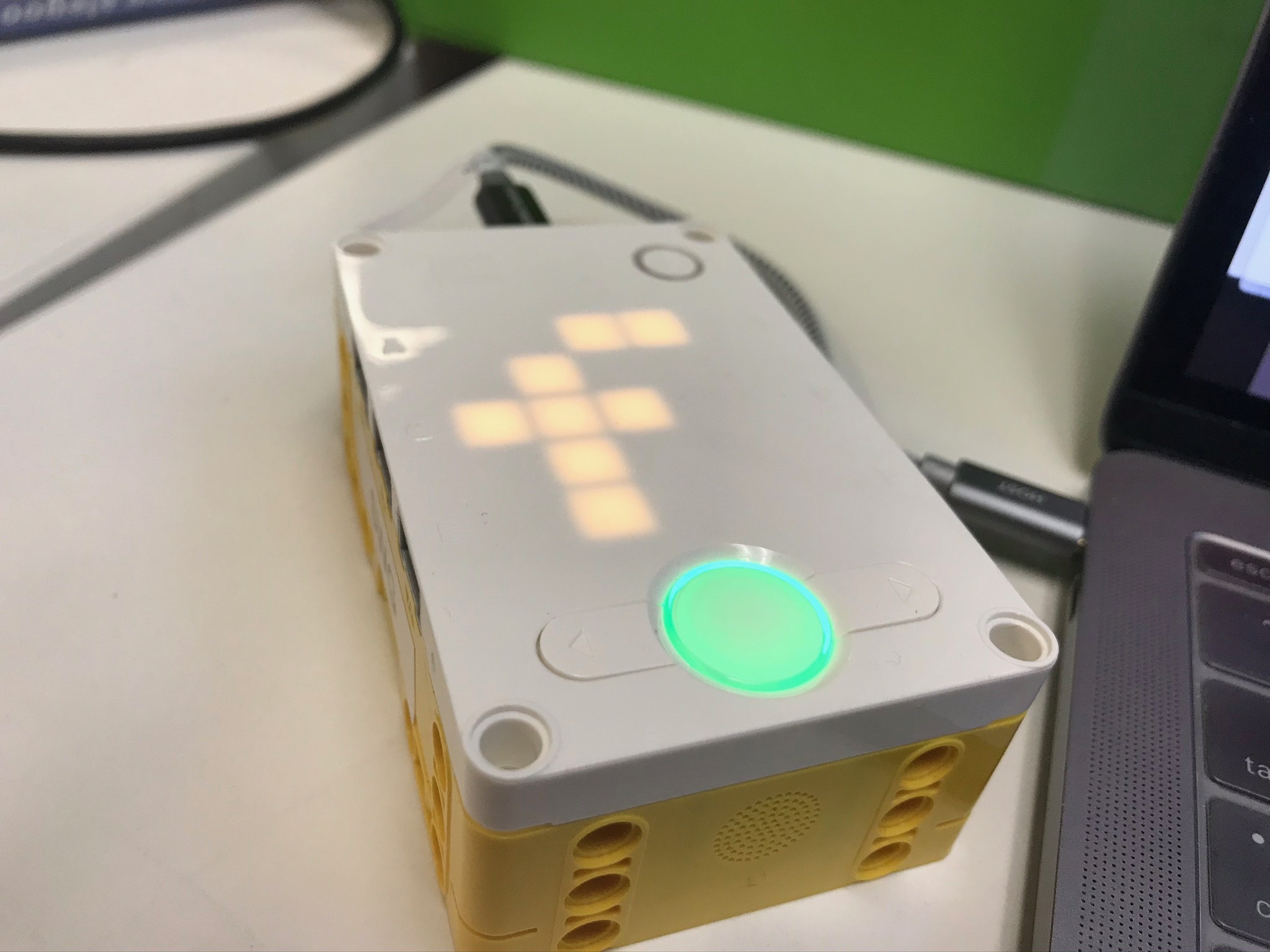
import hub
hub.display.show(‘Tufts’)
Notice the “import” command - that pulls in a python library that lets you talk to SPIKE Prime. You should see Tufts written on the LED matrix on the hub.
Display Your Name
now try typing
hub.display.show('<your name>')
note that since you already imported hub above, it is already in memory. If it were not, you would get an error like:
Traceback (most recent call last):
File "<stdin>" , line 1, in <module>
NameError: name 'hub' isn't defined
Using REPL
One of the more powerful attributes of Python is that you can test anything out before writing code in the REPL (read eval print loop).
It will execute any python command - try typing 2 + 2 below and see what it says:
2+2
Exploring MicroPython on SPIKE Prime
Now it is time to explore.
hub has a lot of functions - you can find out by just typing "hub." (do not forget the period after hub) and then hitting the TAB key in the REPL. That will show you all the different ways you can complete the command.
Challenge: See if you can read the acceleration.
Reading Sensor Values...1
The acceleration data comes back as an array of values. so if you just want the X value, you can try
hub.motion.accelerometer()[0]
or nicer way to code this would be to use variables like this:
import hub accel = hub.motion.accelerometer() xAccel = accel[0] hub.display.show(str(xAccel))
Reading Sensor Values...2
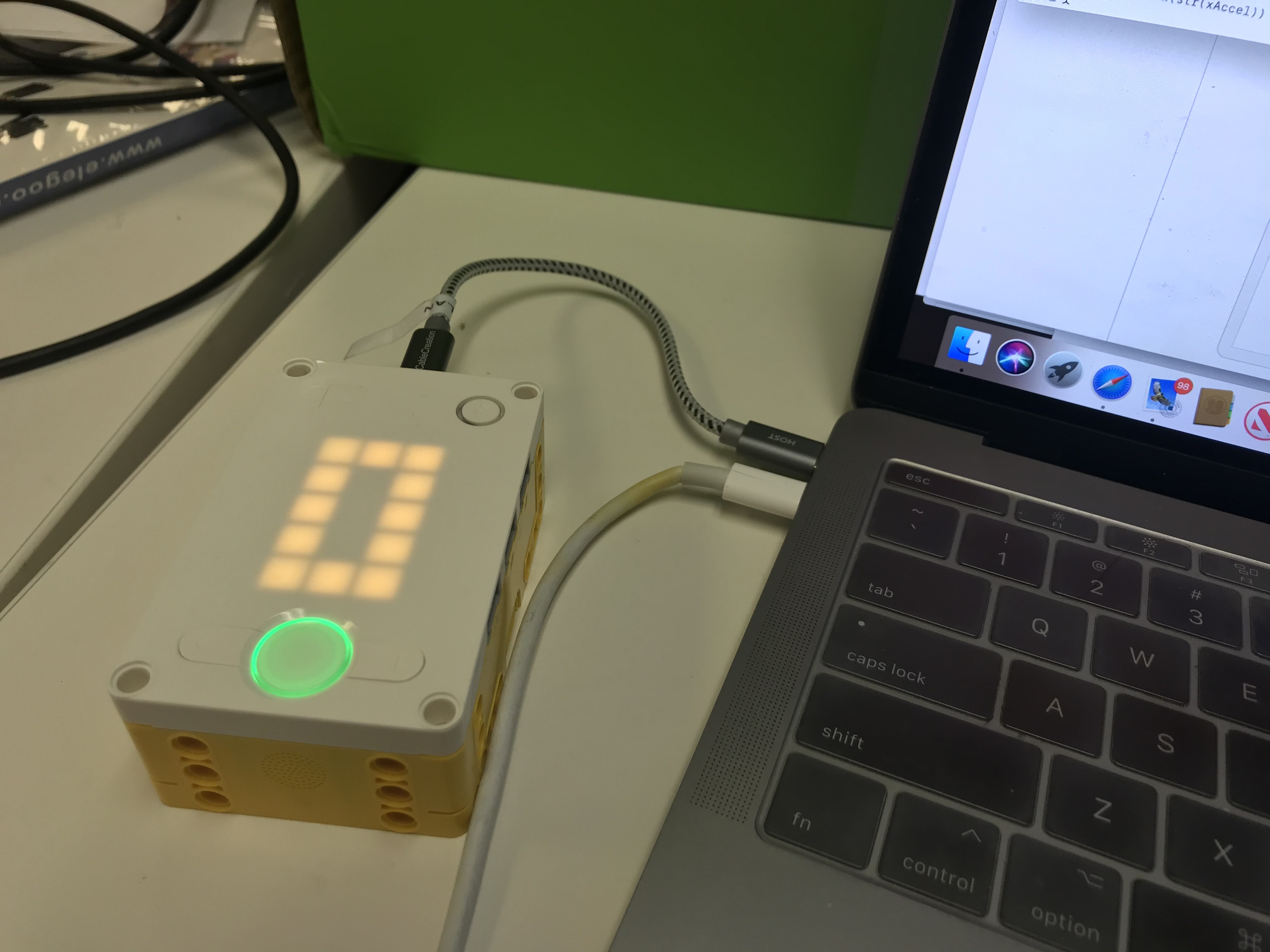
You could also display all three accelerations using a loop.
We will also import the library utime so that we can pause and give you time to read the number on the screen.
Try this code:
import hub,utime
accel = hub.motion.accelerometer() for Acc in accel: hub.display.show(str(Acc)) utime.sleep(0.8)
At this point a few things become important:
spaces - Python is all about indenting right - similar to brackets in other languages, indentation tells you what is inside the for loop and what is not.
when using the REPL, you will notice that when you indent, it no longer executes the line but rather waits for you to finish the lines for the for loop before executing (and the >>> is replaced by …). To finish the for loop, just hit return three times and the loop will be executed.
Challenge
Next, see if you can figure out what the code below does - and try executing it to see if you are right.
import hub,utime while True: accel = hub.motion.accelerometer() for Acc in accel: hub.display.show(str(Acc)) utime.sleep(0.8) if hub.port.B.device.get(): break
Hint -you need a sensor on port B.