MPU6050-Accelerometer+Gyroscope Sensor Basics
by ArjunG32 in Circuits > Arduino
2359 Views, 1 Favorites, 0 Comments
MPU6050-Accelerometer+Gyroscope Sensor Basics
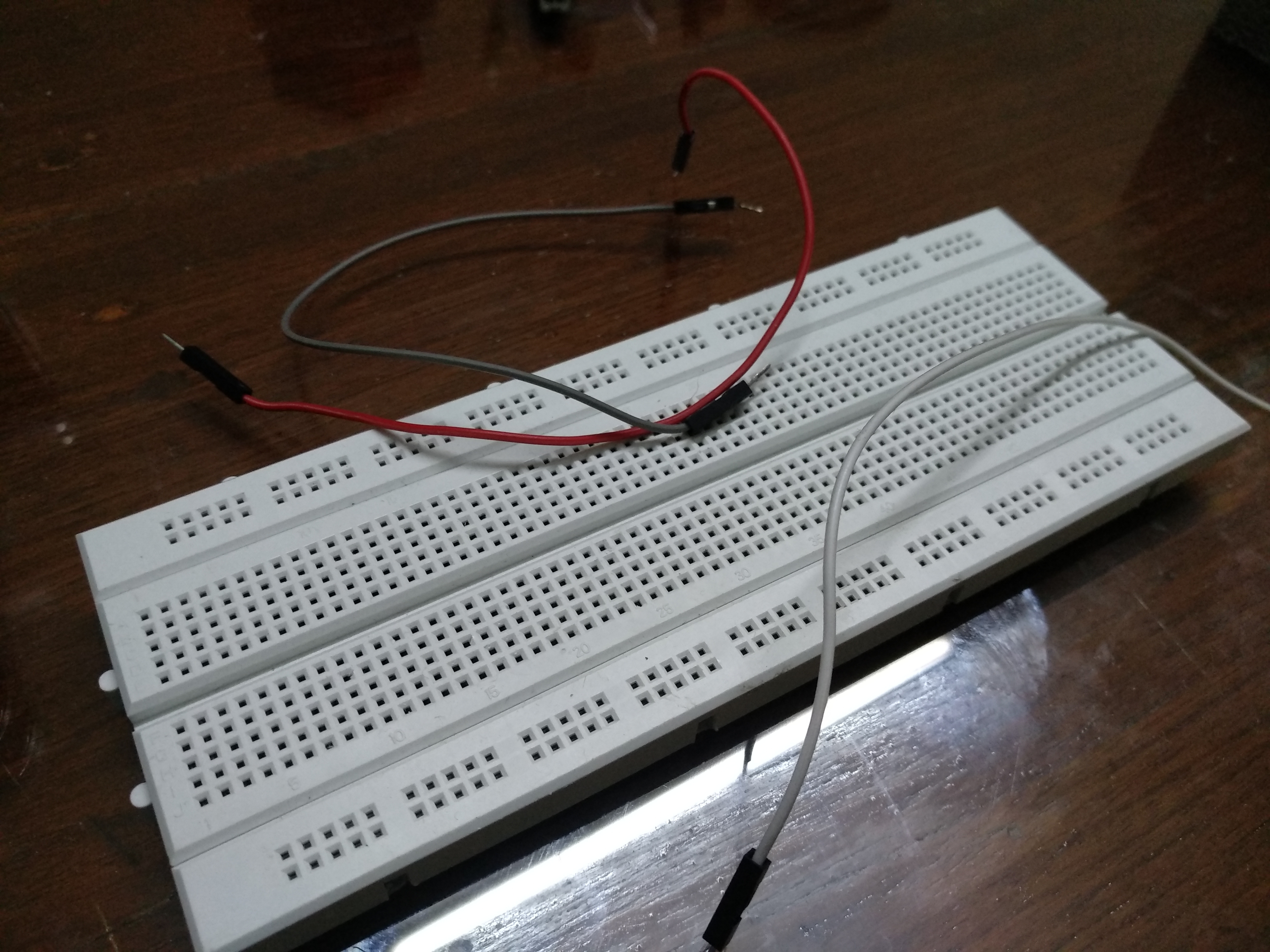



The MPU6050 is a very useful sensor .
The mpu 6050 is an IMU:An inertial measurement unit (IMU)
is an electronic device that measures and reports a body's specific force, angular rate, and sometimes the orientation of the body, using a combination of accelerometers, gyroscopes.
It is a 6 axis device
3 of the axis can measure acceleration and the other 3 are for angular acceleration measurements.
Using the acceleration and angular acceleration it is possible to get a fairly accurate estimate of the angle
In this tutorial we will be exploring how we can use the MPU6050 with a library to make things a lot easier.
Supplies
- Arduino board
- MPU6050
- Jumper wires
- Breadboard
Complete the Circuit

The sensor uses a protocol known as I2c to communicate with the Arduino to send it the values.
The A4 pin is used for SCL- serial clock and should be connected to SCL of the sensor and
,A5 to SDA-Serial data line.
The Vcc is connected to 5v and the Gnd is connected to ground
Coding

#include <MPU6050_tocn.h>
#include <Wire.h>
Before I begin, this library is not written by me, I just think it is the simplest one there and love using it.
These are the header files ^^, wire.h is used to establish an i2c communication
MPU6050 mpu6050(Wire);
here we name our gyroscope, or create an object for those who are familar with OOPs.
void setup() { Serial.begin(9600); Wire.begin(); mpu6050.begin(); mpu6050.calcGyroOffsets(true); }
Initially we calculate offsets since all the angle readings are going to be with respect to the initial orientation.
void loop() { mpu6050.update(); Serial.print("angleX : "); Serial.print(mpu6050.getAngleX()); Serial.print("\tangleY : "); Serial.print(mpu6050.getAngleY()); Serial.print("\tangleZ : "); Serial.println(mpu6050.getAngleZ()); }
Each gives us the measure of the angle.
Other Functions

The library contains other functions
like:
mpu6050.getTemp()//gives the temperature(not very accurate)
mpu6050.getAccX()//Linear acceleration in X direction
(similar functions are mpu6050.getAccY(), mpu6050.getAccZ())
mpu6050.getGyroX()//Angular acceleration about the x axis
(similar functions are mpu6050.getGyroY(), mpu6050.getGyroZ())