How to Use L293D Motor Driver Shield With Arduino
by Rachana Jain in Circuits > Arduino
5479 Views, 0 Favorites, 0 Comments
How to Use L293D Motor Driver Shield With Arduino
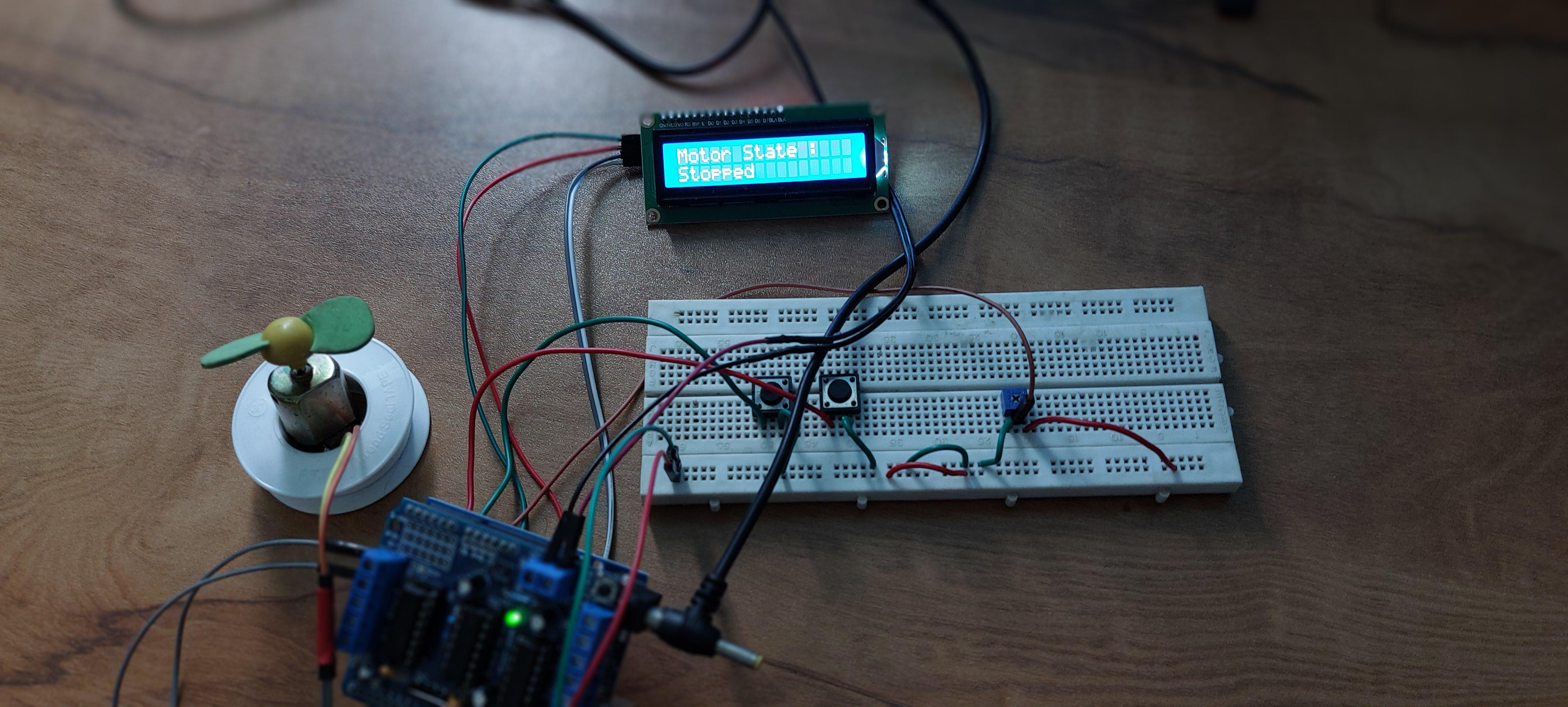
The L293D Motor Driver Shield is a versatile and popular component for controlling various types of motors with an Arduino. This tutorial will cover how to control DC motor using the L293D Motor Driver Shield and Arduino.
Supplies
Arduino UNO
L293D Motor Driver Shield
DC Motor
I2C LCD Display
Push buttons
POT
Breadboard
Jumper Wires
What Is L293D Motor Driver Shield?
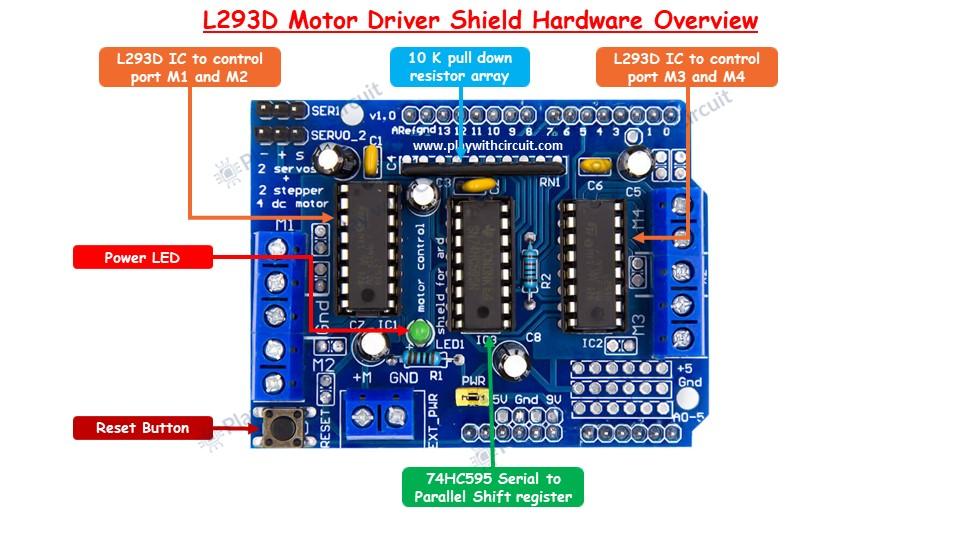
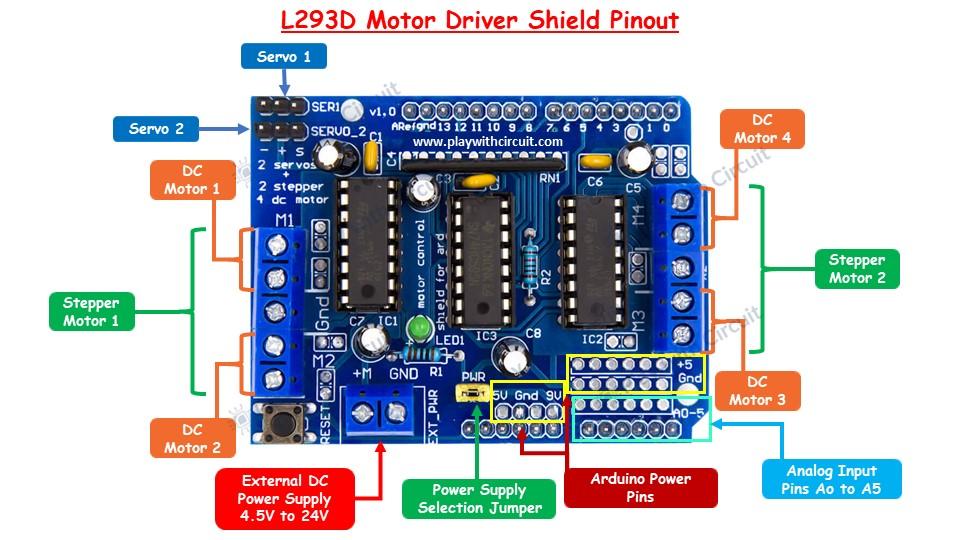
This shield has an L293D IC, a dual H-bridge motor driver integrated circuit (IC), which allows it to drive two DC motors, a stepper motor, or a servo motor. Here’s a detailed breakdown of the L293D Motor Driver Shield.
Key Features of the L293D Motor Driver Shield
- Dual H-Bridge IC: The L293D IC can control up to two DC motors or one stepper motor. It provides bidirectional control for two motors independently.
- Current Handling: Each channel of the L293D can handle up to 600mA of continuous current and peak currents up to 1.2A per channel.
- Voltage Range: The shield can operate at a wide range of voltages, typically from 4.5V to 36V.
- Protection Diodes: The shield has built-in diodes to protect the IC from back EMF generated by the motors.
- PWM Control: Supports Pulse Width Modulation (PWM) for speed control of the motors.
Components on the L293D Motor Driver Shield
- L293D ICs: The shield typically has two L293D ICs to provide four H-bridge circuits.
- Motor Terminals: Connectors for attaching motors. Usually labeled as M1, M2, M3, and M4.
- Power Terminals: Terminals for connecting an external power supply.
- Jumpers: Used for selecting power options and enabling/disabling the motors.
- Control Pins: Pins that interface with the Arduino to control the motors (IN1, IN2, IN3, IN4, ENA, ENB).
L293D Motor Driver Shield Pinout
The L293D Motor Driver Shield has several key pins that you need to be familiar with to control motors. Below is a detailed explanation of each pin and its function:
- Motor Output Pins (M1, M2, M3, M4)
- M1: Outputs for Motor 1
- M2: Outputs for Motor 2
- M3: Outputs for Motor 3
- M4: Outputs for Motor 4
- Control Pins
- IN1, IN2, IN3, IN4: These pins are connected to the Arduino and are used to control the direction of the motors. By setting these pins HIGH or LOW, you can control the rotation direction of the motors.
- ENA, ENB: These are the enable pins for the motor channels. ENA enables M1 and M2, while ENB enables M3 and M4. By applying a PWM signal to these pins, you can control the speed of the motors.
- Power Supply Pins
- VCC: This pin is used to provide power to the motors.
- GND: The ground pin that needs to be connected to the ground of the external power supply and the Arduino.
- Servo Motor Pins: The shield has three sets of three-pin headers for connecting servo motors. These pins provide 5V, GND, and signal connections for each servo motor.
- I2C Pins: The shield includes I2C pins (SDA and SCL) for interfacing with I2C devices. These pins are used for communication between the Arduino and other I2C-compatible devices.
Controlling DC Motor With Arduino UNO and L293D Motor Driver Shield
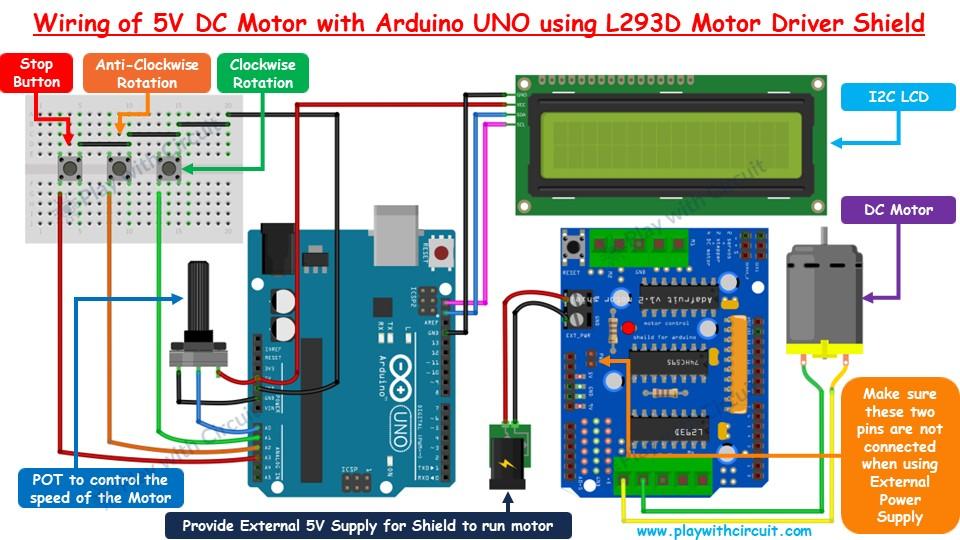
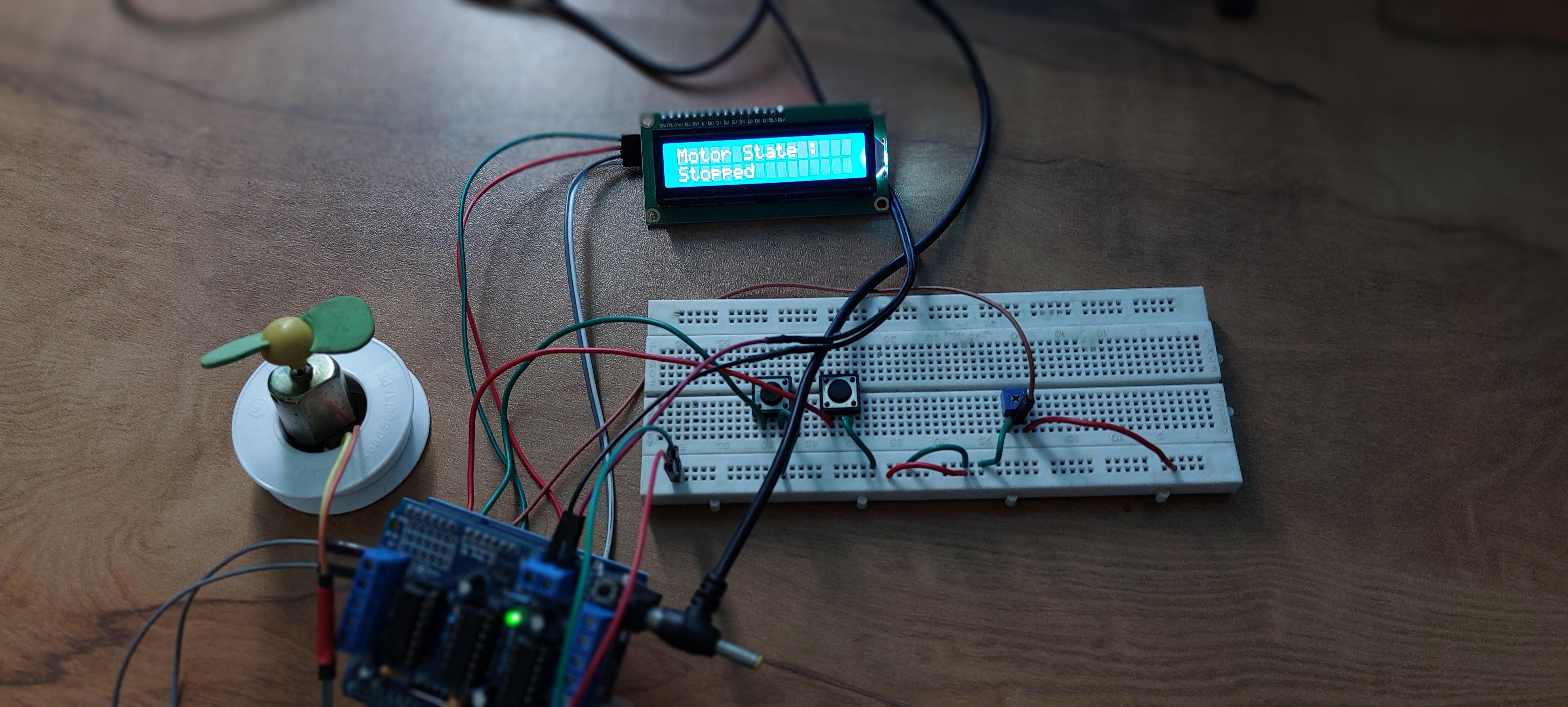
This wiring diagram shows how to connect a 5V DC motor to an Arduino Uno using an L293D Motor Driver Shield, along with additional components such as an I2C LCD display, a potentiometer (POT) to control motor speed, and buttons for motor control.
Connections:
- DC Motor: The DC motor is connected to the M3 motor output terminal on the L293D Motor Driver Shield.
- Buttons: Here we’ve used three buttons:
- Clockwise Rotation Button: Connected to a digital input pin on the Arduino.
- Anti-Clockwise Rotation Button: Connected to another digital input pin on the Arduino.
- Stop Button: Connected to a third digital input pin on the Arduino.
- Potentiometer (POT):
- The potentiometer is used to control the speed of the motor. It’s connected to an analog input pin A0 on the Arduino.
- One side of the POT is connected to GND, the other side to 5V, and the middle pin to an analog input.
- I2C LCD Display:
- The I2C LCD display is connected to the Arduino using the I2C communication pins (SDA and SCL).
- External Power Supply:
- An external 5V power supply is connected to the power terminals of the L293D Motor Driver Shield to provide sufficient power for the motor.
- Ensure the jumper connecting the Arduino’s 5V and the motor shield’s power is removed when using an external power supply.
- Arduino UNO:
- The Arduino is connected to the L293D Motor Driver Shield by mounting the shield on top of the Arduino, ensuring all pins are aligned.
- The Arduino is powered via USB or an external power source.
How It Works
- Motor Control:
- The buttons send signals to the Arduino to control the motor’s rotation direction and stop it.
- The Arduino sends signals to the L293D Motor Driver Shield to control the motor based on the button inputs.
- Speed Control: The potentiometer’s value is read by the Arduino, and it adjusts the PWM signal sent to the L293D Motor Driver Shield’s ENA pin, controlling the motor speed.
- Display: The I2C LCD display shows information such as the current motor speed or direction based on the Arduino’s programming.
- Power Management: The external power supply ensures that the motor gets enough power without overloading the Arduino.
Arduino Code
The following code demonstrates how to control a DC motor using the L293D motor driver shield. The motor’s direction and speed are controlled using three buttons (for forward, reverse, and stop) and a potentiometer to adjust the speed. Additionally, an LCD is used to display the motor’s direction and speed.
#include <AFMotor.h>
// Library to run DC Motor Using Motor Driver Shield
#include <LiquidCrystal_I2C.h>
// Library to Run I2C LCD
LiquidCrystal_I2C lcd(0x27, 16, 2); // Format -> (Address,Columns,Rows )
// Create the motor object connected to M3
AF_DCMotor motor(3);
// Define button pins
const int forwardButtonPin = A1;
const int reverseButtonPin = A2;
const int stopButtonPin = A3;
// Define potentiometer pin
const int potPin = A0;
// Variables to store motor state and direction
bool motorRunning = false;
int motorDirection = BACKWARD; // FORWARD or BACKWARD
// Read the potentiometer value
int potValue;
int motorSpeed;
// Variable to store button states
bool forwardButtonState;
bool stopButtonState;
bool reverseButtonState;
// Inline function to check if button is pressed packed with debouncing logic
inline bool chkButtonState(int pinNum, int checkState, int debounceDelay) {
if (((digitalRead(pinNum) == checkState) ? true : false) == true) {
delay(debounceDelay);
return (((digitalRead(pinNum) == checkState) ? (true) : (false)) == true);
} else {
return false;
}
}
void setup() {
// initialize the lcd
lcd.init();
// Turn on the Backlight
lcd.backlight();
// Clear the display buffer
lcd.clear();
// Set cursor (Column, Row)
lcd.setCursor(0, 0);
lcd.print("DC Motor using");
lcd.setCursor(0, 1);
lcd.print("L293D Shield");
// Set button pins as inputs
pinMode(forwardButtonPin, INPUT_PULLUP);
pinMode(stopButtonPin , INPUT_PULLUP);
pinMode(reverseButtonPin, INPUT_PULLUP);
// Start with motor off
motor.setSpeed(0);
motor.run(RELEASE);
delay(2000);
// Clear the display buffer
lcd.clear();
// Set cursor (Column, Row)
lcd.setCursor(0, 0);
lcd.print("Motor Direction:");
lcd.setCursor(0, 1);
lcd.print("Stopped ");
}
void loop() {
// Read the potentiometer value for changing speed as per Analog input
potValue = analogRead(potPin);
motorSpeed = map(potValue, 0, 1023, 0, 255);
// Read the button states
forwardButtonState = chkButtonState(forwardButtonPin, LOW, 20);
reverseButtonState = chkButtonState(reverseButtonPin, LOW, 20);
stopButtonState = chkButtonState(stopButtonPin, LOW, 20);
// check for Forward run
if (forwardButtonState && (!motorRunning || motorDirection == BACKWARD)) {
// Set cursor (Column, Row)
lcd.setCursor(0, 1);
lcd.print("Clock ");
if (motorDirection == BACKWARD) {
motor.setSpeed(0);
motor.run(RELEASE);
delay(1000);
}
motorRunning = true;
motorDirection = FORWARD;
motor.setSpeed(motorSpeed);
motor.run(FORWARD);
}
// check for Reverse run
else if (reverseButtonState && (!motorRunning || motorDirection == FORWARD)) {
// Set cursor (Column, Row)
lcd.setCursor(0, 1);
lcd.print("Anti-Clk");
if (motorDirection == FORWARD) {
motor.setSpeed(0);
motor.run(RELEASE);
delay(1000);
}
motorRunning = true;
motorDirection = BACKWARD;
motor.setSpeed(motorSpeed);
motor.run(BACKWARD);
}
// Stop motor
else if (stopButtonState && motorRunning) {
// Set cursor (Column, Row)
lcd.setCursor(0, 1);
lcd.print("Stopped ");
motorRunning = false;
motor.setSpeed(0);
motor.run(RELEASE);
}
// Adjust motor speed if running and display speed on LCD
if (motorRunning) {
motor.setSpeed(motorSpeed);
// Set cursor (Column, Row)
lcd.setCursor(9, 1);
lcd.print("SPD:");
lcd.print(((motorSpeed*100)/255));
lcd.print("% ");
}
}
Conclusion
This project combines the power of the L293D Motor Driver Shield and the versatility of the Arduino to control motor. To learn how to control stepper motor and servo motor, visit our comprehensive tutorial Control DC, Servo, and Stepper Motors with L293D Motor Driver Shield & Arduino. Here, you'll find more insights and examples to help you master motor control with the L293D Motor Driver Shield.