How to Make a Landing Page With Next.js, Without Coding!
by jungmink623 in Circuits > Computers
92 Views, 1 Favorites, 0 Comments
How to Make a Landing Page With Next.js, Without Coding!
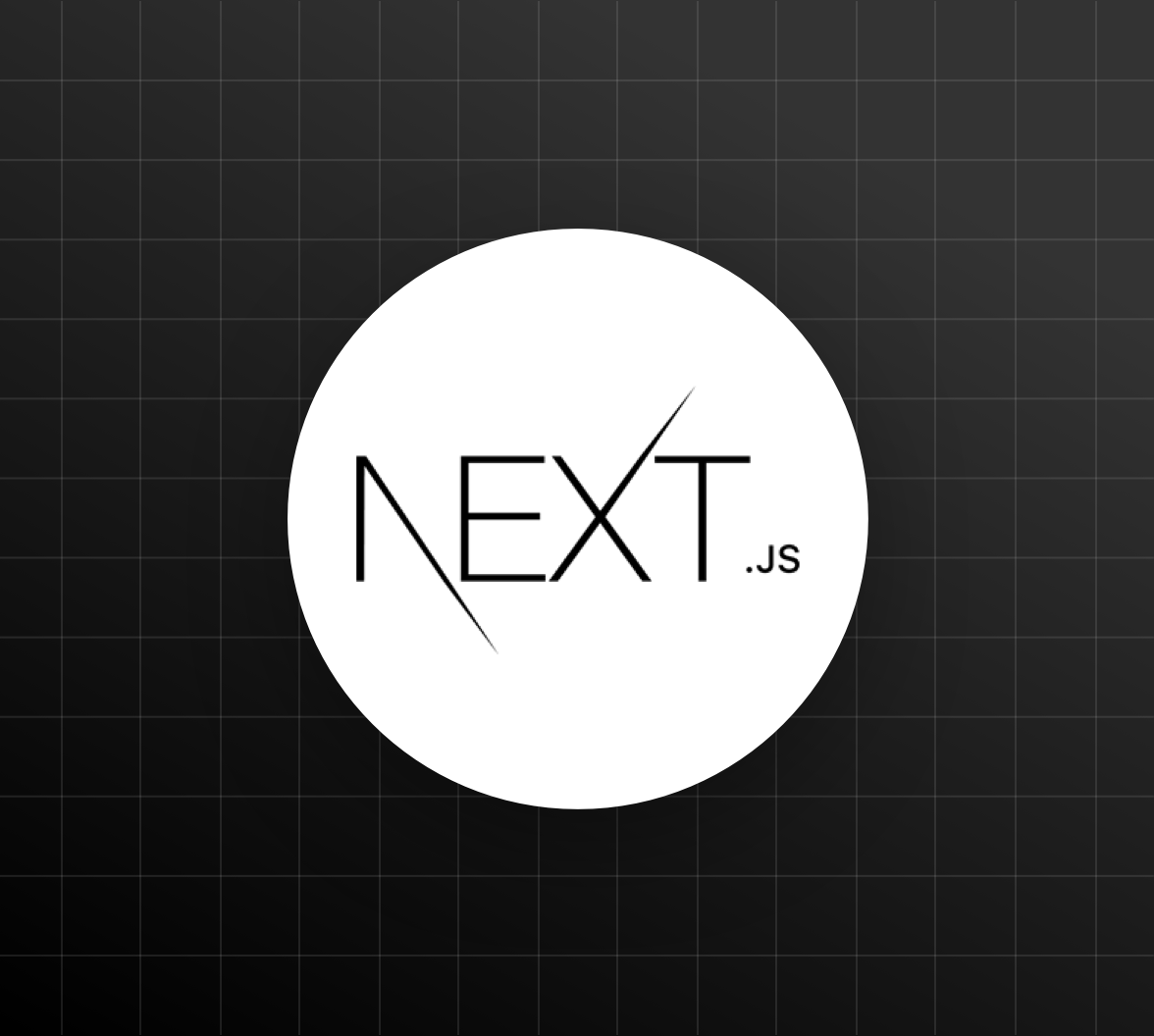
In this guide we will go over how to make a very generic landing page for a website/web application using the Next.js framework. Next.js is a JavaScript framework developed by Vercel that allows developers to create web applications using React, another framework.
In most websites, the first page that users will interact with or see is the landing page. If you are planning on creating and hosting a website, this guide will be useful in guiding you through the steps to make your own generic landing page from scratch!
You will need a decent understanding of JavaScript/TypeScript, HTML/CSS, so I will be skipping over basics. however there any many tools available that help people develop their own websites without having to do much coding, which we will talk about as well. Otherwise, this project should take an hour, so work at your own pace.
Supplies
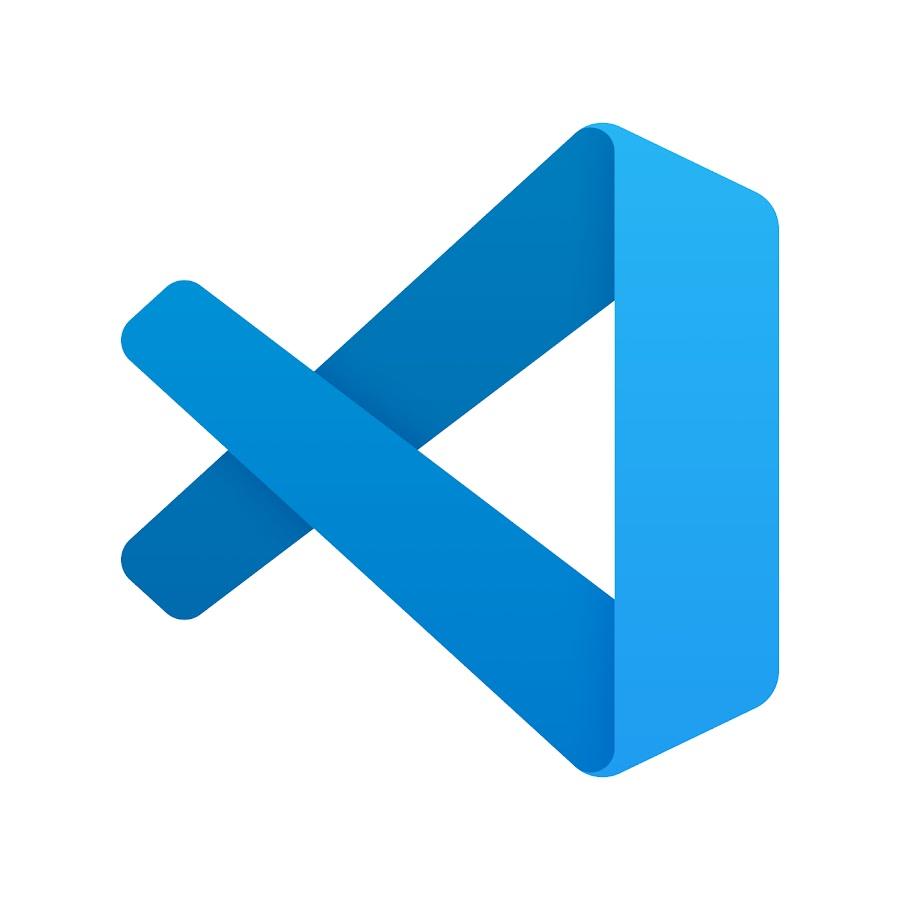
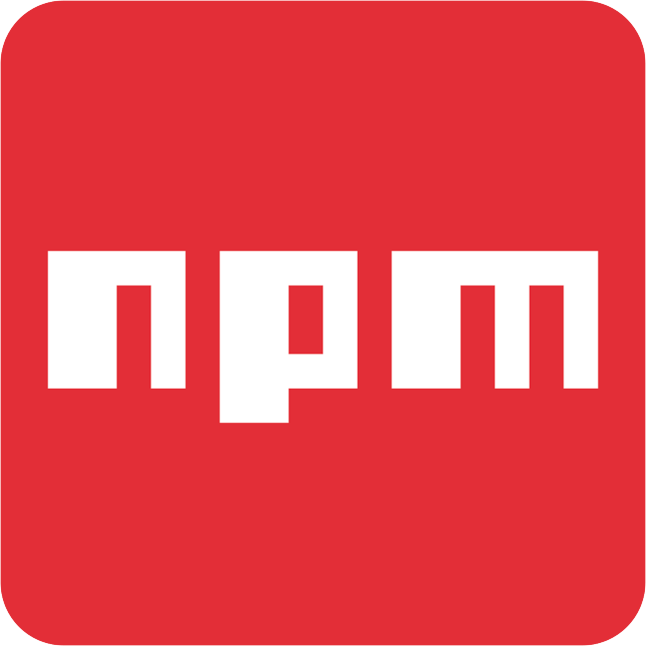
For this project you will need two things:
- VS Code installed (any version works, but latest version is recommended)
- npm installed to install and manage the dependencies you will need for this project
VS Code is a source code editor that you will be doing most of the development in.
npm (Node Package Manager) is a package manager you will be using just to install and keep track of dependencies.
Initialize Your Environment
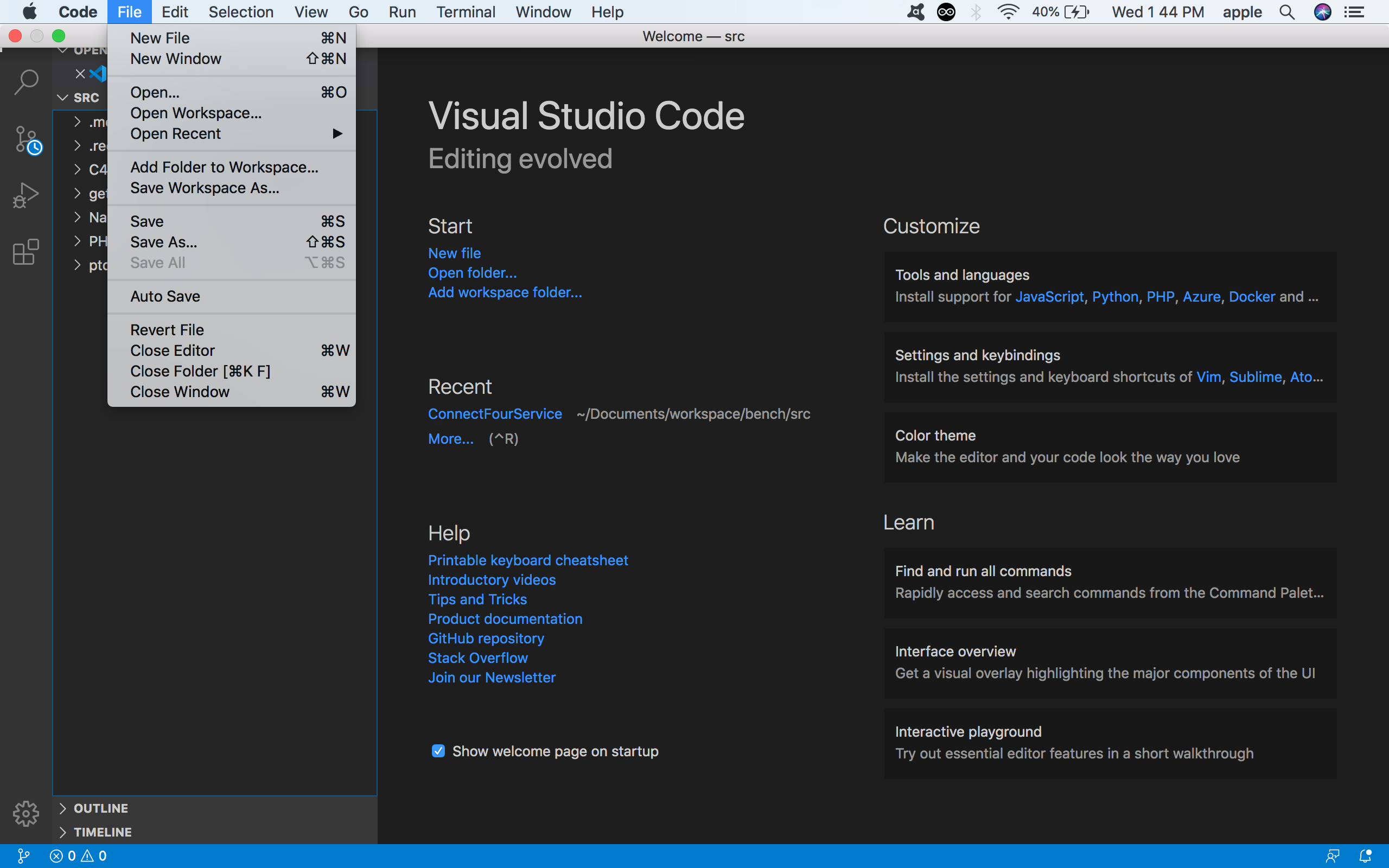
First, you will want to create a Next.js app in VS Code. Start by opening a new project in VS Code. Then, open a new terminal using CTRL + `.
Type the following into the terminal:
For the landing page we will be using Tailwind CSS to make design easier. We can install Tailwind by doing the following two commands:
Find the tailwind.config.js file in the app root folder and add the following code to the file (replace everything):
Finally, let's install shadcn/ui! shadcn/ui is a component library built on Tailwind CSS. The reason we are using shadcn/ui is so that rather than coding components on a website from scratch, such as the navigation bar, we can use a predefined component library that has a bunch of generic components ready to use. Think of it as using a template for a PowerPoint presentation. Let's install shadcn/ui using the following command:
You will be asked a few questions to configure the components.
Congratulations! You have finished the setup. Now you can run:
And you should be able to run your app in your browser, ready to be worked on. For me, it runs on localhost:3000 by default, but check your terminal to see where it runs for yours.
Note: You can use Ctrl + C to kill the web application at any time, then simply run npm run dev to run it again!
The Navbar
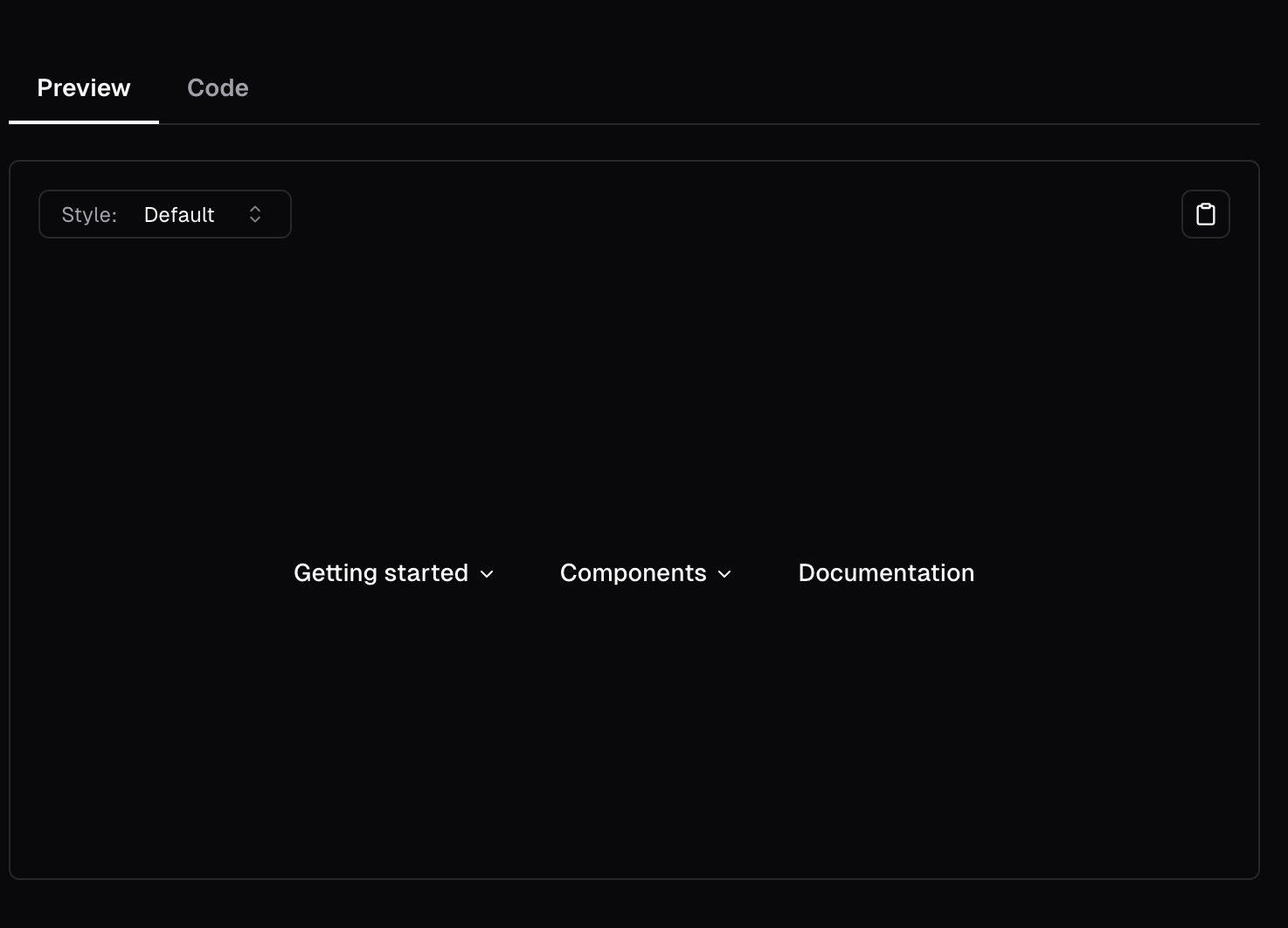
A landing page on a website will typically have three or two main components. The Navbar (Navigation Bar) at the top of the page that helps you navigate the website, the Hero Section, containing all the content of the landing page, and the Footer, which is just the bar at the bottom with trademarks, extra links, etc.
For this step lets start by creating a Navbar component.
Let's use a Navbar from shadcn/ui's library using the following command:
The Navbar should now be in your "components" folder. Feel free to edit the code as you wish.
Then, in your "app" folder, find page.tsx (or .jsx, depending on which configuration you did in Step 1. Replace all the contents of page.tsx with the following code:
All we have done is added the Navbar to the page of the default route, which is just localhost:3000/ with nothing after the slash. So the Navbar should appear when the project is run immediately.
Test how it looks by running your project using:
The Hero Section
The Hero section is the body of the landing page, and the first thing you see on the page just under the Navbar!
Let's use a modified shadcn/ui Hero component, however feel free to make your own hero component! Execute this command:
Then, add the Hero component onto the page.tsx underneath the Navbar:
If you are not already running the page, run:
And you should see both the Navbar and the Hero section on the page!
The Footer
The footer is basically another bar like the Navbar, but at the bottom. You can put more hyperlinks, buttons, maybe copyrights/trademarks, or other information at the bottom in the footer. Lets use a generic footer with some text and place it at the bottom of the page!
Let's add a modified shadcn/ui footer component!
Now we add the component underneath the Hero in the page.tsx file:
Conclusion
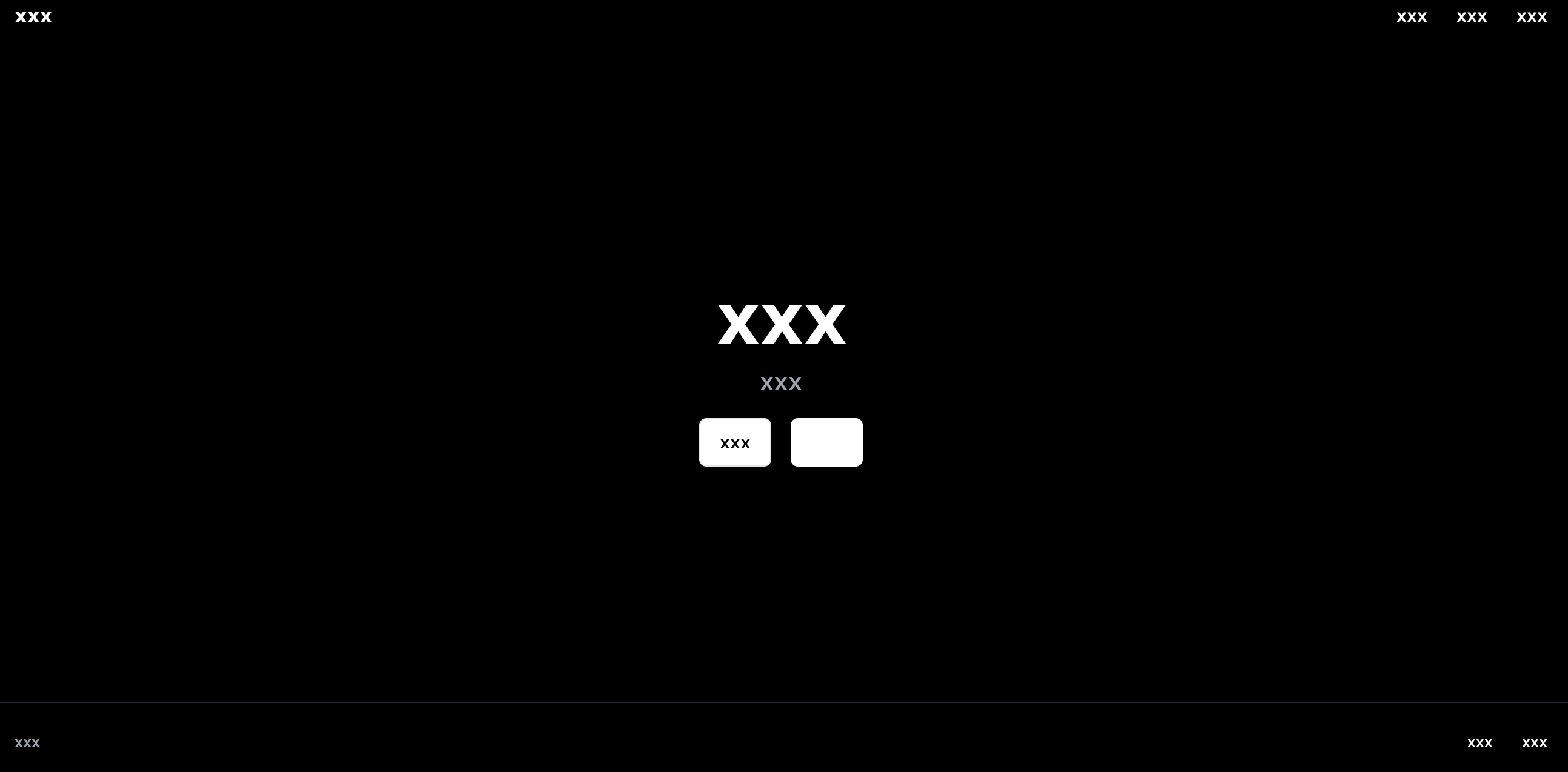
Your page.tsx in the app folder should now look like this:
When you run:
You should now be able to see the landing page with the Navbar, Hero, and Footer components all on the page. For the example, I filled all the text fields with "xxx".
If you look at the code for each component individually in the "components" folder, you can edit the code however you wish to fit your needs.
Feel free to use ChatGPT or, my personal favorite tool, v0.dev (I used this tool for the Hero and Footer), and paste the code of any component, and ask to change it however you like. It is a quick and easy way to make edits to components and pages as a whole built with Next.js or React without having to do any coding!
Good luck!