How to Create a JAR Software Library in Java
by xanthium-enterprises in Circuits > Software
3184 Views, 0 Favorites, 0 Comments
How to Create a JAR Software Library in Java
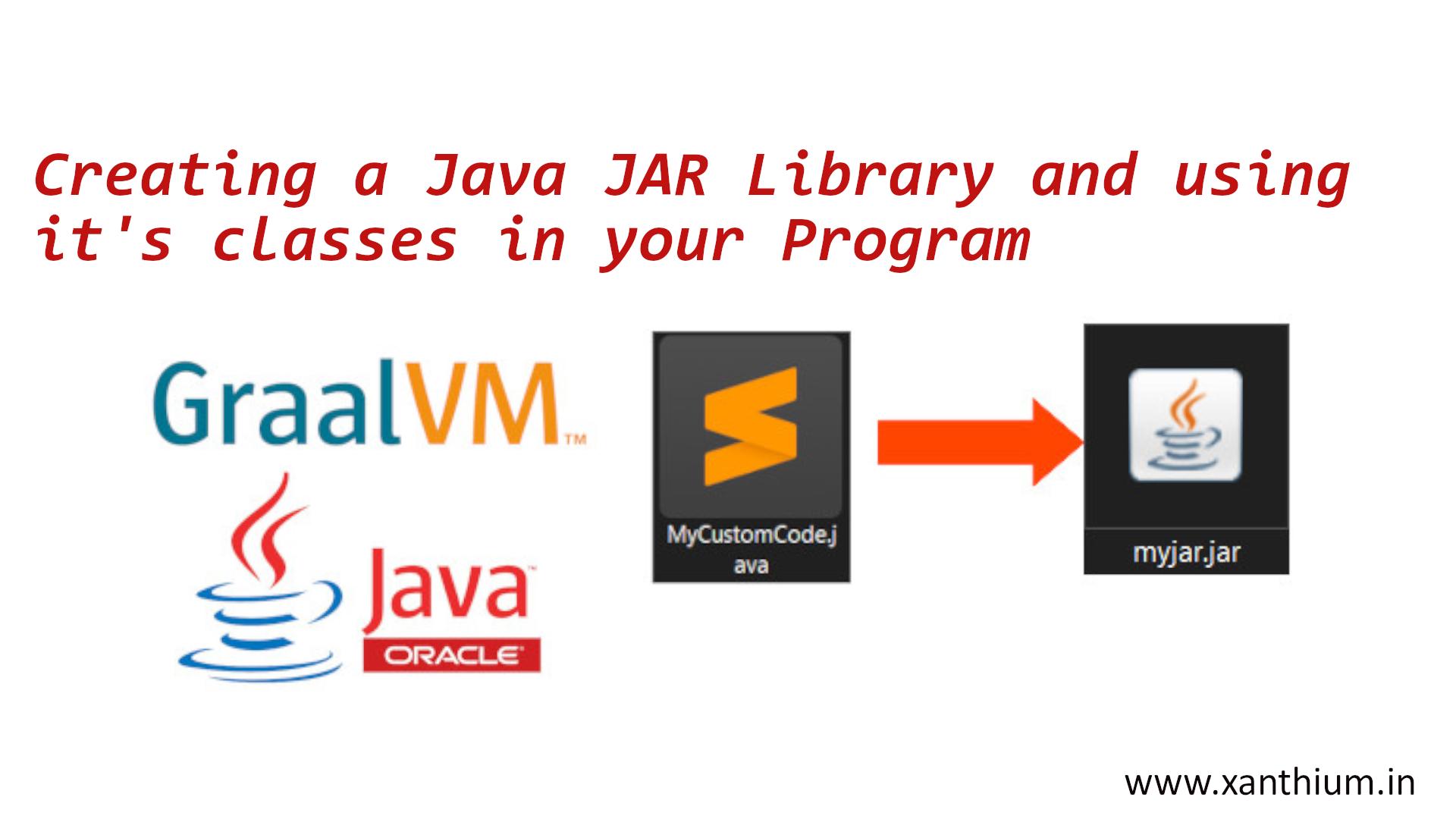
In this tutorial, we will learn
- How to create a JAR Software library using Java and JDK Command line Tools
- How to use the classes inside the created JAR in your Custom program.
We have an online tutorial
Tutorial uses Java Language and Command line tools from JDK.
Tutorial is aimed at beginners who wants to learn how Java works without using any IDE's
Supplies
This is a software tutorial, So you don't have to buy anything.Following items have to be downloaded if you dont have them
- Java Runtime Environment (JRE)
- Java Development Kit (JDK)
- A Text Editor
Video of How to Create Java JAR Software Library Using JDK
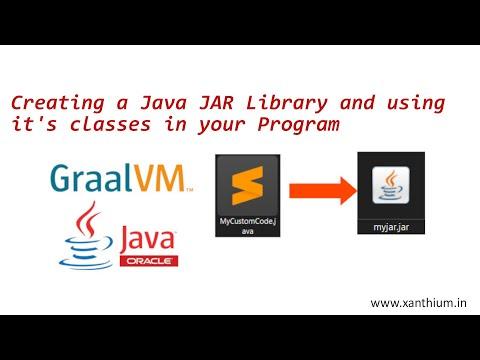
This Youtube video explains the entire process of creating and using a JAR library on a windows platform
Creating a JAR File and Viewing Its Contents Using JDK Tools
You can create a JAR file using the jar utility of the Oracle JDK or GraalVM JDK.
The command for creating the JAR file is shown below.
jar cvf your-jar-file-name.jar input-files
- input-files can be java class files, images icons or other files you want to include with your program
- your-jar-file-name.jar The name you want to give to your jar file.
To view the contents of your JAR file you can use the tf option as shown below.
jar tf your-jar-file-name.jar
Adding the Files
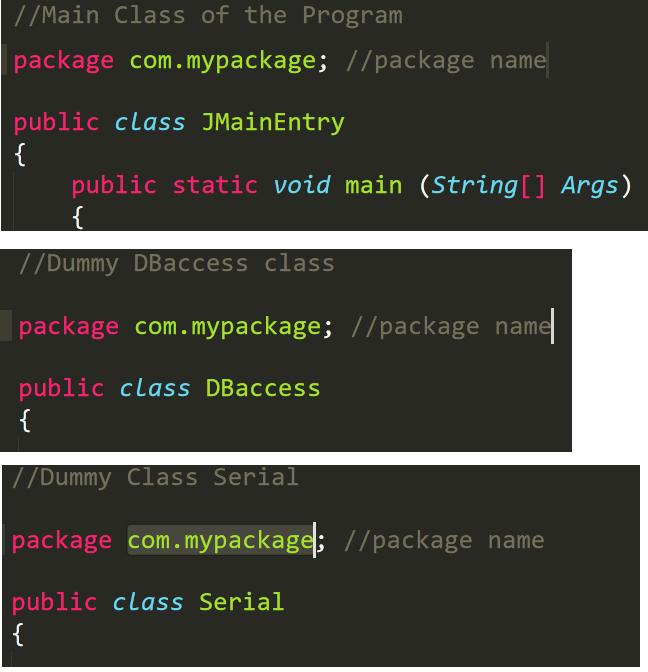
We will learn how to create a multi file class JAR package containing hierarchical package name spaces.
All our source codes belong to the package com.mypackage
There are three source files which we will compile and convert to classes.
For this tutorial we will be creating three java files.
- JMainEntry.java - contains the main class
- DBaccess.java - public dummy class DBaccess
- Serial.java - public dummy class Serial
Compiling the Java Files to Classes
Now we put
- DBaccess.java - public dummy class DBaccess
- Serial.java - public dummy class Serial
file in a directory and use the javac compiler to compile to .class files.
Make sure that all the methods() in Serial.java and DBaccess.java are public.
javac -d . *.java
this would create a directory structure com\mypackage\all your class files
You can run the class file by
java com.mypackage.JMainEntry
Creating JAR File Using JDK
use the jar utility to pack all the files into a jar file
jar cvf MyJarLib.jar *
This will create a jar file named MyJarLib.jar
Using the Jar in Your Custom Program
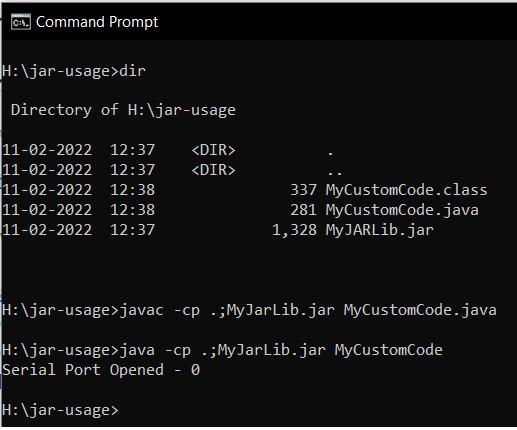
Now create a .java file called MyCustomCode.java that will use the classes inside the jar file.
MyCustomCode.java
import com.mypackage.Serial; // Classes inside JAR import com.mypackage.DBaccess; // Classes inside JAR public class MyCustomCode { public static void main (String[] Args) { Serial S = new Serial(); //Class inside JAR S.open(); } }
You can compile the code as shown below
Compiling Code with JAR library
javac -cp .;MyJARLib.jar MyCustomCode.java
Running the Code with JAR library
java -cp .;MyJARLib.jar MyCustomCode