How to Capture Remote Control Codes Using an Arduino and an IRreceiver
by eliesalame in Circuits > Arduino
33312 Views, 154 Favorites, 0 Comments
How to Capture Remote Control Codes Using an Arduino and an IRreceiver
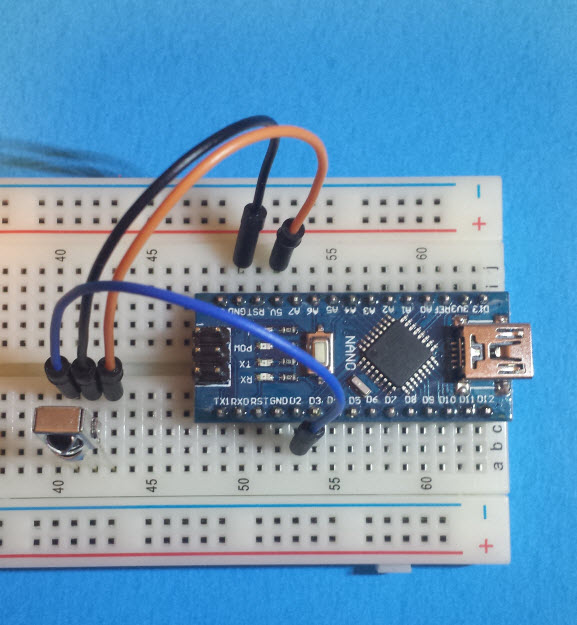
In this instructable I will show you how to write a small code to capture the remote control codes in Hexadecimal and decimal values.
We will do this using the serial monitor that is embedded in the Arduino IDE.
This will introduce you to a library called IRremote.h and few commands like enableIRIn(), decode() and resume().
Here Is What You'll Need in This Project
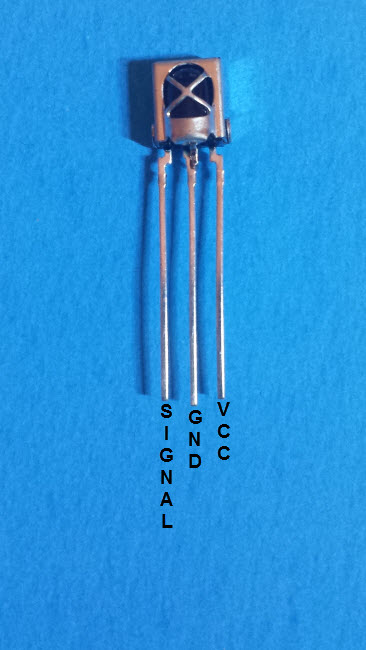
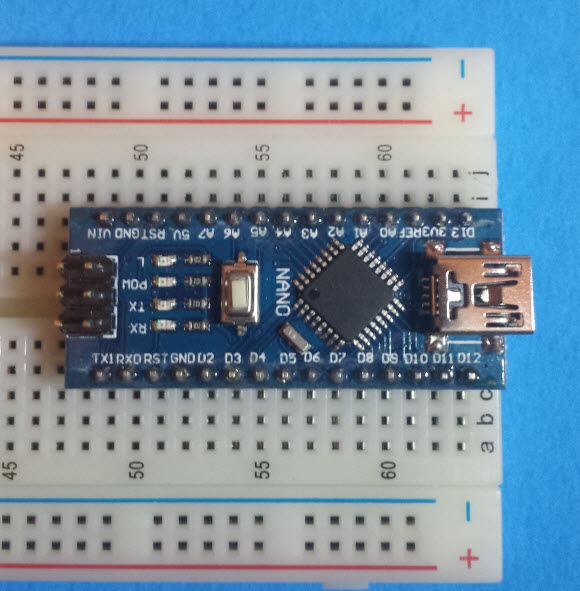
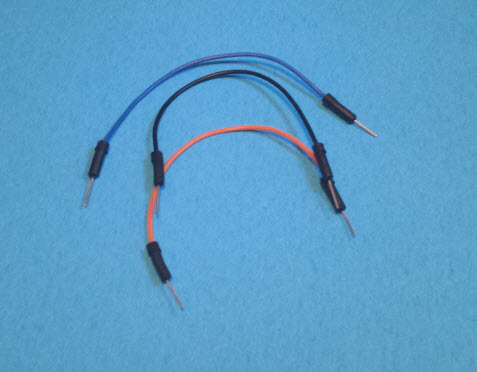
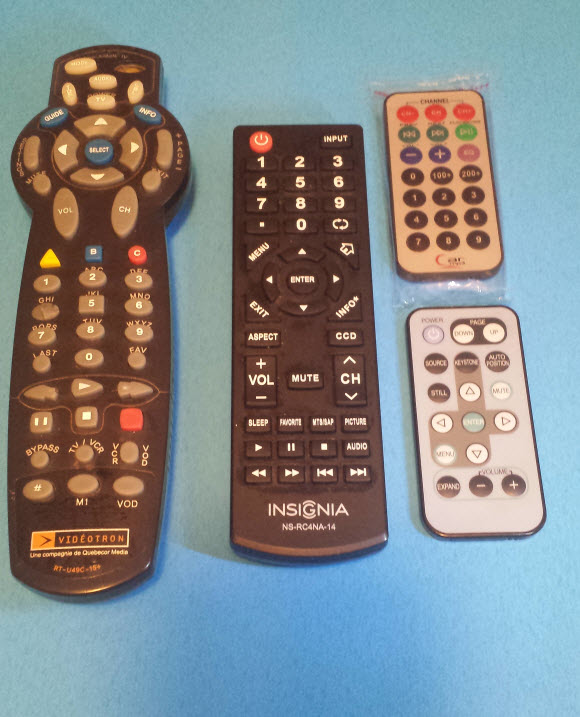
- An IR receiver, I am using the 1838B
- An Arduino, I am using the Arduino Nano
- Three jumper wires
- A remote control, any one you have lying around
I included the datasheet of the IRreceiver 1838B in this step, but this code should be valid for other IRreceivers as well.
Downloads
Connect the Components
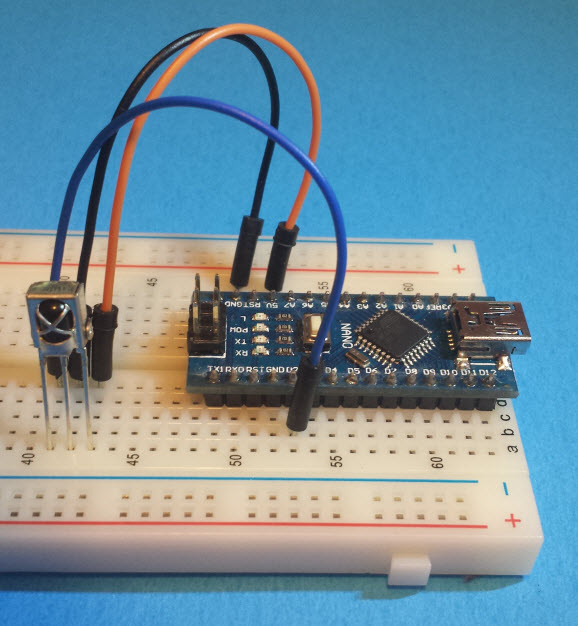
The connection is straight forward.
- Connect the signal pin on the IRreceiver to PIN 2 on the Arduino
- Connect the GND pin on the IRreceiver (middle pin) to the GND pin on the Arduino
- Connect the VCC pin on the IRreceiver to the 5V pin on the Arduino
That's it, connect the Arduino to your computer and lets move on to the sketch
The Sketch
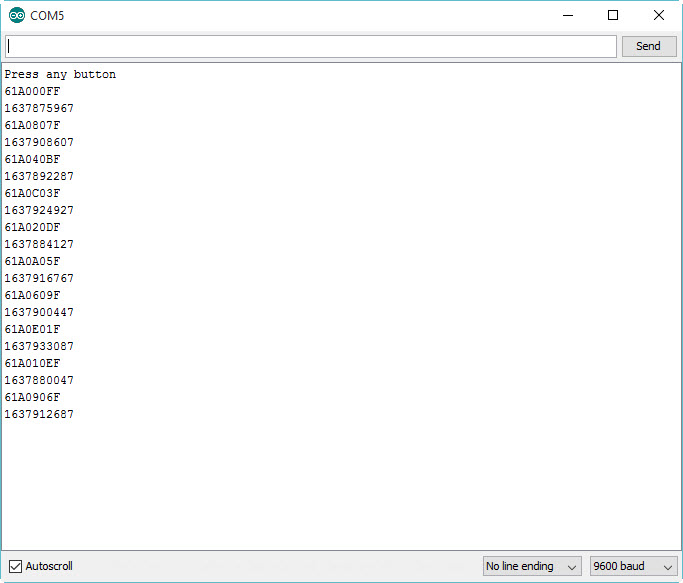
I added the .ino file in a zipped format in this step
The first line is:
#include <IRremote.h>
This line will load the library needed to run the commands, if you don't have it already, you can download it from https://github.com/z3t0/Arduino-IRremote, or send me a message and I will email it to you.
Then we will define an integer on pin 2 called RECV_PIN, this is the signal pin from the 1838B to pin 2 on the Arduino. Another variable to store the data received from RECV_PIN in called IRrecv and the last one is used to decode the results.
int RECV_PIN = 2; //Set the IRreceiver on pin 2 on the Arduino
IRrecv irrecv(RECV_PIN); //Create an instance for irrecv
decode_results results; //Create an instance to store the collected data in
In the void setup, we start the serial monitor and print a line that says "Press any button" and we activate the receiver using the command irrecv.enableIRIn()
In the void loop we check if the IR has received a signal using the: if (irrecv.decode(&results)), if so then print the result on the serial monitor in HEX and in decimal then resume listening using the command irrecv.resume().
In the picture: I pressed the buttons 1 to 0 on the remote and the result was printed on the serial monitor.
This sketch is used to capture information and use it later in other codes. I used it to capture the codes on my remote and saved the information in a text file. here is an example:
HEX values for the light Blue Remote control
Up Arrow = AE2C287E
Down Arrow = 1983CB83
Right Arrow = 903079EF
Left Arrow = CD564618
Power Button = 406A9AD7
Page Down = DF580FF9
Page up = 4B8A0854
Source = 75D0E6F0
Keystone = A6D4A34C
Auto Position = A131C30
Still = AEC585B4
Mute = 60FD51BC
Enter = C29995F7
Menu = 5165E0A
Expand = 3449863D
Volume - = 52DE9202
Volume + = 67E45AC1
Now that we know how to get the codes for any remote control button, I will show you in an other instructable how to use those codes to control items like LEDs, motors, relays etc..