How to Build a Field Independent Pitching (FIP) Calculator in Python 3.10
by Batzinga in Circuits > Computers
444 Views, 1 Favorites, 0 Comments
How to Build a Field Independent Pitching (FIP) Calculator in Python 3.10
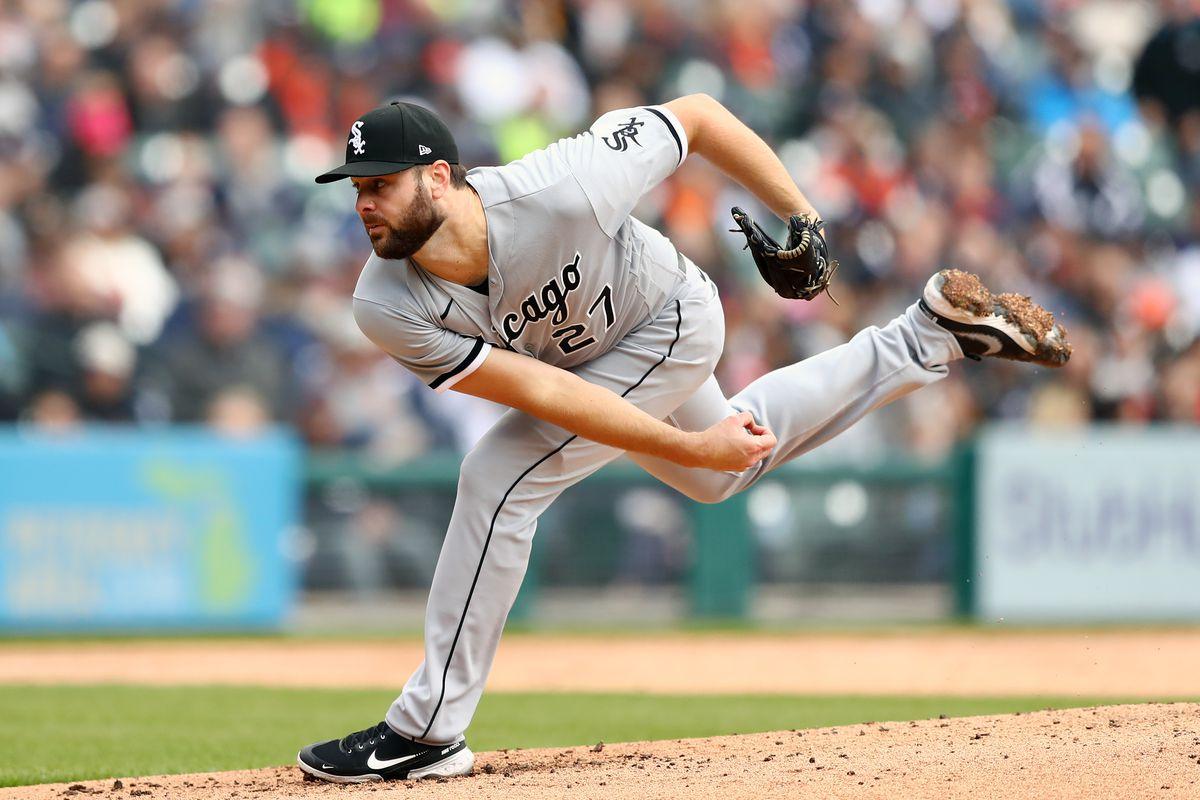
Baseball is America’s pastime. For over a hundred years, millions of people have gathered to watch pitchers and batters face off in a duel of physical strength and mental fortitude. However, the game has changed significantly in recent decades. In 1980, statistician Bill James developed sabermetrics, advanced statistics that could be used to more accurately analyze players.
One such statistic is Field Independent Pitching (FIP). This sabermetric allows one to analyze a pitcher’s performance in isolation from his teammates. In contrast to the more conventional statistic Earned Run Average (ERA) which is the number of runs allowed per nine innings pitched, the FIP equation only uses stats that the pitcher has control over. Stats like hits are excluded from FIP because hits are often dependent on the skill of the fielders. Instead, FIP focuses on stats like home runs and walks.
FIP is extremely important in today’s game, and it’s important to know how to calculate the metric. In this tutorial, I will teach you how to build a FIP calculator in Python 3.10. You do not need to have any previous coding experience.
By the end of this tutorial, you will be able to use your calculator for any baseball game from the MLB to Little League. You will also gain exposure to the basics of Python like input() and print().
Note: This tutorial requires that you have Python and IDLE installed on your computer. If you do not, check out this tutorial to get Python up and running.
https://realpython.com/installing-python/
Define the Function
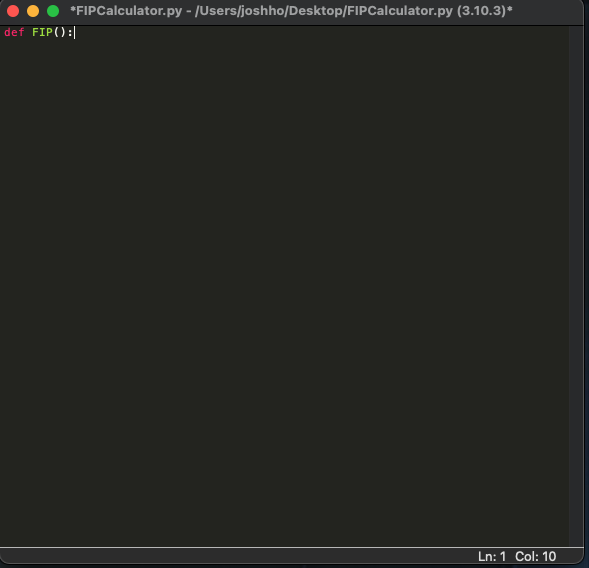
In order to operate our calculator, we first need to define the function. We do this by writing def followed by the name of our function and ():. Let’s call the function FIP since that’s the stat we are trying to calculate.
Write a Docstring.
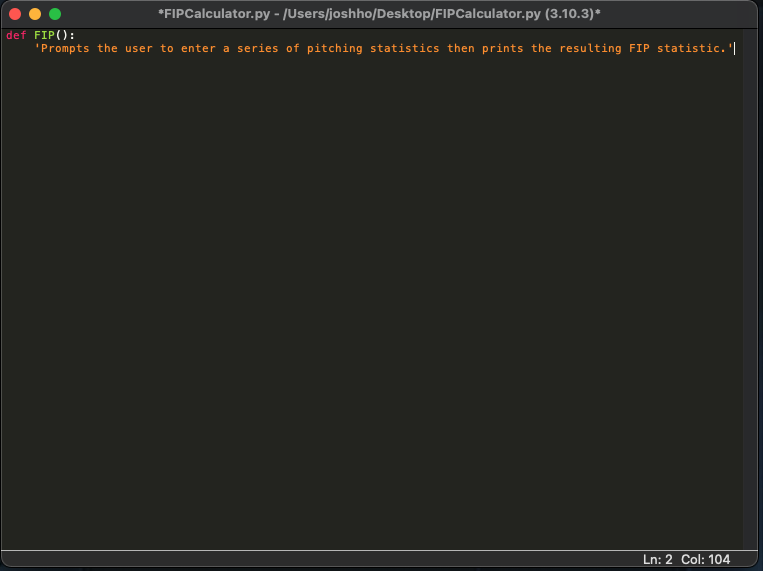
Docstrings are extremely important in coding. They explain the function’s purpose to the user. They should be brief, no more than a sentence or two. An example of a docstring for this project would be ‘Prompts the user to enter a series of pitching statistics then prints the resulting FIP statistic.’ Write the docstring on the line directly beneath the function, indented.
Define Our Variables
The FIP formula relies on many other statistics like home runs and walks, so we need to create a way for users to input this data into the calculator. We do this by naming a variable and setting it equal to a user’s input. We need to do this for every single variable in the equation. Make sure that your lines of code line up with the docstring.
Home Runs
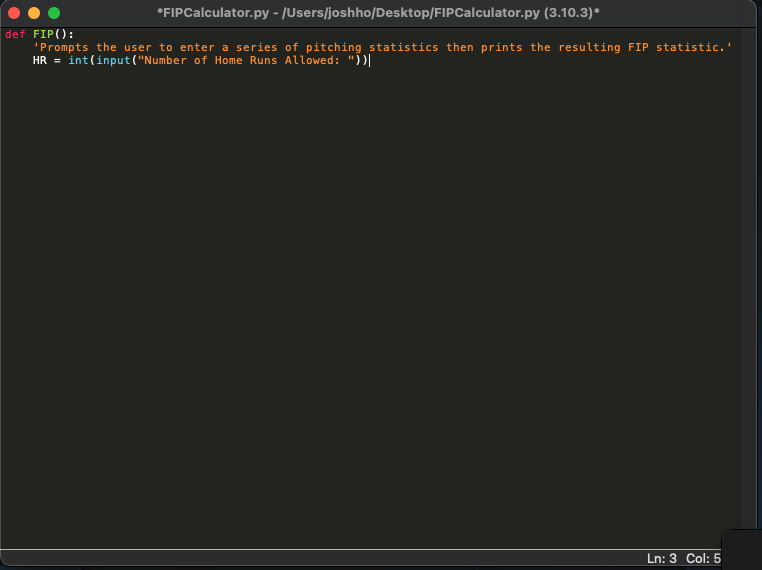
- First, write the variable name. For home runs, the standard abbreviation is HR.
- Follow the variable name with an equals sign and then int(). This tells Python to treat the HR variable as an integer. Since our calculator will be doing math with our variables, we need to make sure our variables behave like numbers.
- Inside the parentheses after int, type input(). This allows users to input information that we will use for our calculator.
- For clarity and aesthetic purposes, inside the input parentheses write “Number of Home Runs Allowed: “. This text will show up when the calculator runs, then the user can enter a number.
Walks
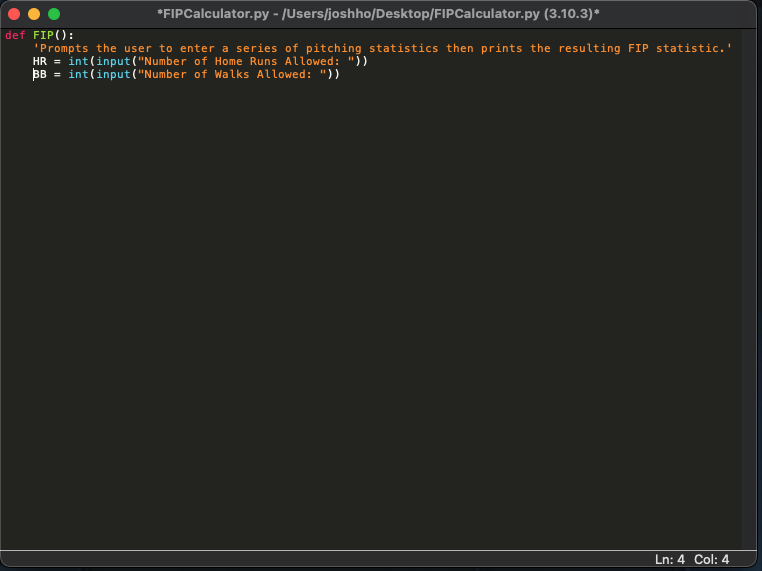
- Follow the same steps in step 4, replacing HR with BB (universal abbreviation for walks) and Number of Home Runs Allowed with Number of Walks Allowed.
Hit by Pitch
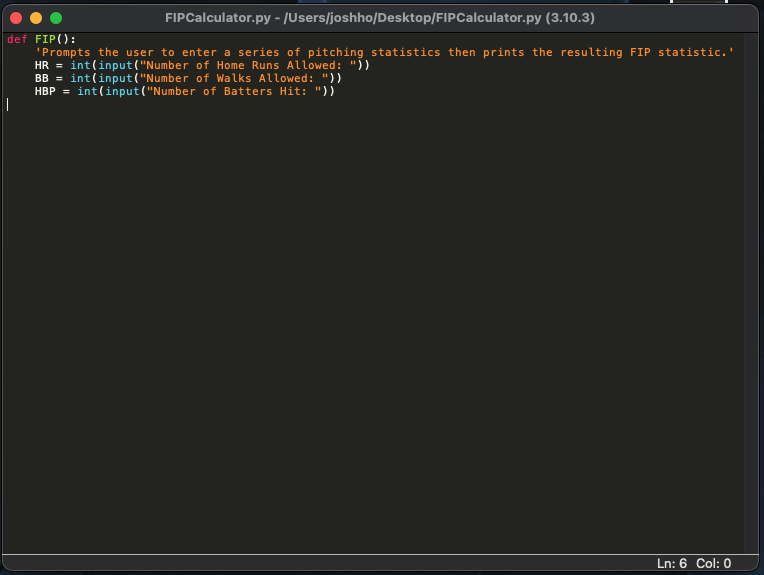
- Follow the same steps in step 4, replacing HR with HBP (hit by pitch) and writing “Number of Batters Hit: “ inside the input().
Strikeouts
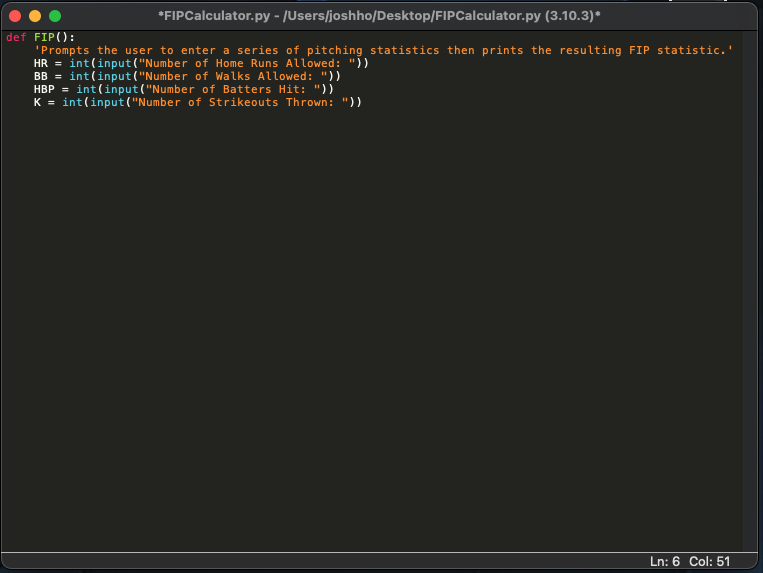
- Follow the same steps in step 4, replacing HR with K (universal abbreviation for strikeouts) and writing “Number of Strikeouts Thrown: “ inside the input().
Innings Pitched
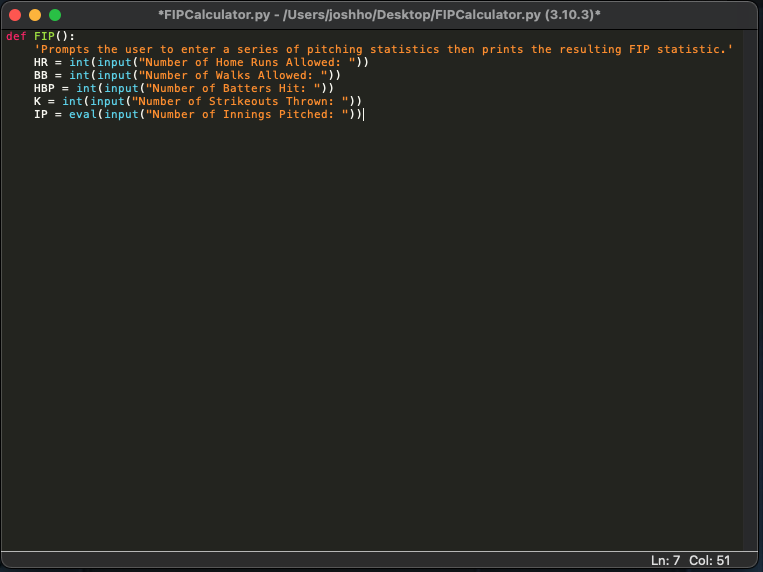
This variable varies slightly from the previous ones. In contrast to stats like home runs and walks, which only come in integers, innings pitched is not always an integer. For example, if a pitcher was pulled in the 5th inning with 2 outs, he technically pitched 4.67 innings.
- Follow the same steps in step 4, except replace int() with eval() to reflect our change in data. Also replace HR with IP and write “Number of Innings Pitched : “ inside the input().
FIP Constant
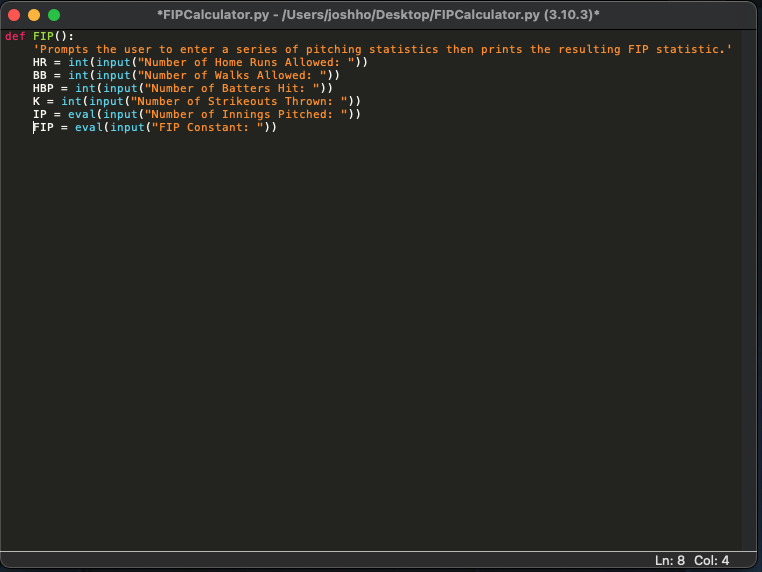
Like the previous step, the FIP constant also takes floating point values (not integers).
- Follow the same steps as step 8, except replace IP with FIP and write “FIP constant: “ inside the input().
Note on the FIP constant:
The FIP constant is an arbitrary number used to convert FIP to the same scale as ERA, a more traditional metric. This allows you to directly compare FIP and ERA. The FIP constant varies slightly every year based on MLB pitcher performance, but it typically falls between 3.1-3.2. Feel free to use any value in this range for your calculations.
Build the FIP Equation
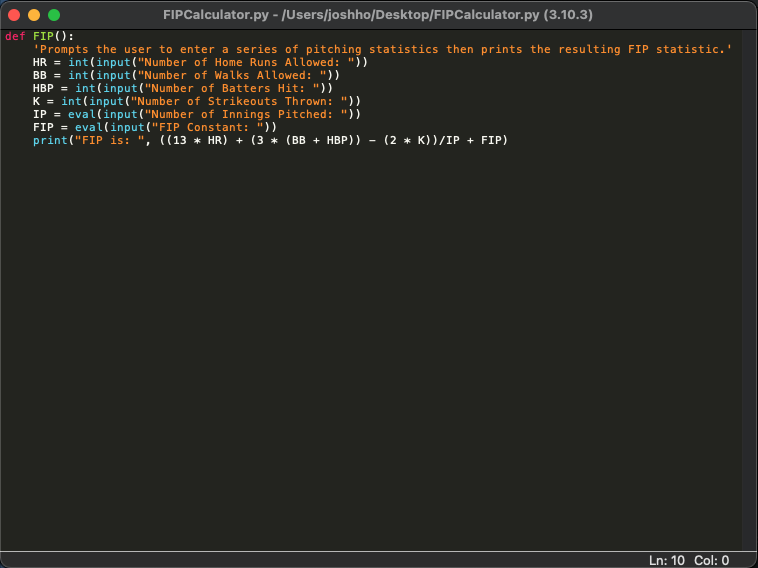
The equation is as follows:
FIP = ((13*HR)+(3*(BB+HBP))-(2*K))/IP + FIP constant
To view our calculated FIP value, we are going to use the python print() function.
- Write print(“FIP is:”,
- Build the equation after the comma by typing the following:
- 13 * HR
- + 3 *(BB + HBP)
- - 2*K
- Put parentheses around what has been typed so far
- /IP to divide everything by innings pitched
- Put another set of parentheses around what has been typed so far
- + FIP
- Add a ) to close the parentheses.
The calculator is now ready to be used!
Using the Calculator
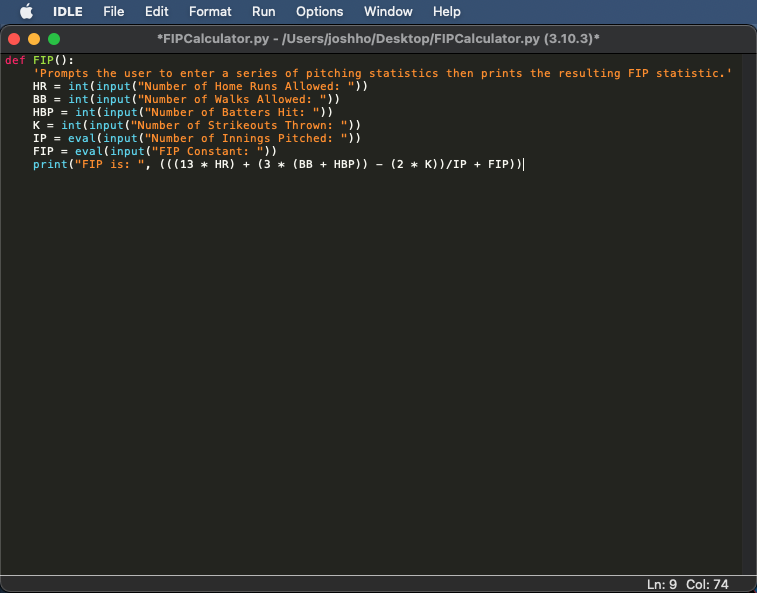
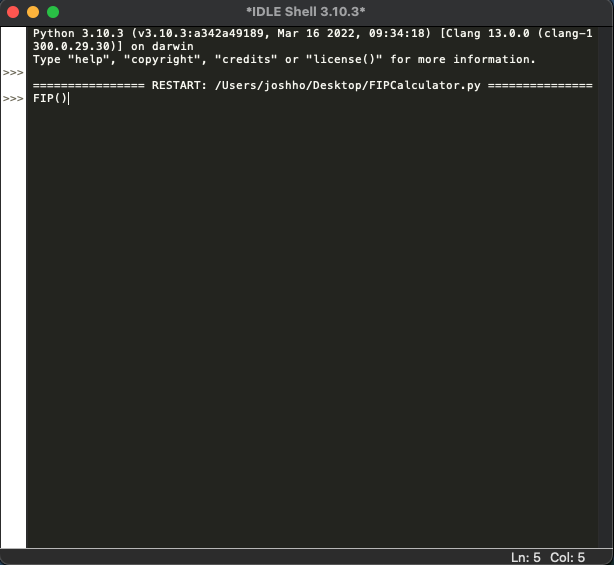
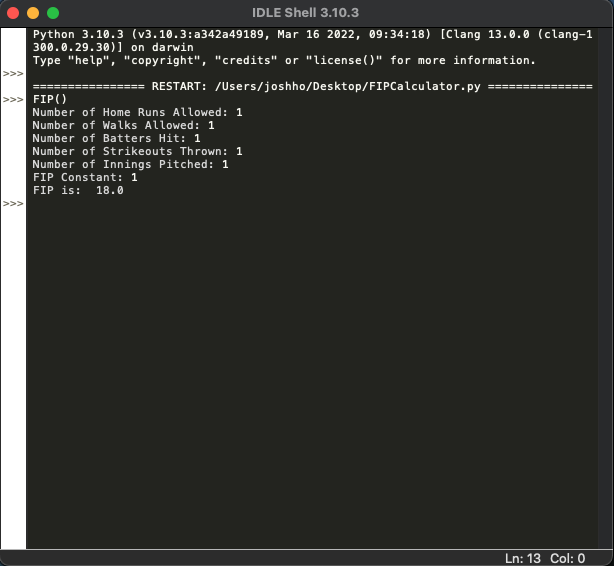
To use the calculator, we need to run our program.
- Hit F5 or the Run button at the top of your screen to bring up IDLE, where the code will be run.
- Type FIP() to call the function and activate our calculator.
- Enter your data points as prompted by the calculator.
- Once you enter all of your data, the calculator will print the resulting FIP value.
The last picture is an example of what the calculator should look like if you enter 1 for every value.
If your value does not match the one in the picture, double-check your equation in step 11. Ensure that all of the parentheses are in the right place.
Conclusion
Congratulations on completing your FIP calculator! Feel free to take your new creation out into the real world and use it to calculate your favorite pitcher’s FIP during the MLB season. Remember that, like all baseball statistics, FIP is not the final say on a player’s ability. Rather, it is a useful tool to evaluate player performance in conjunction with all of the other advanced analytics out there. If you’re a coach, player, or just a fan, remember to use FIP wisely and make informed decisions. And if you’re an aspiring programmer, hopefully this short exercise gave you a good foundation in strings, functions, and basic Python syntax.