How to Avoid Running Into Things!
by VhsSymptom in Circuits > Electronics
273 Views, 0 Favorites, 0 Comments
How to Avoid Running Into Things!
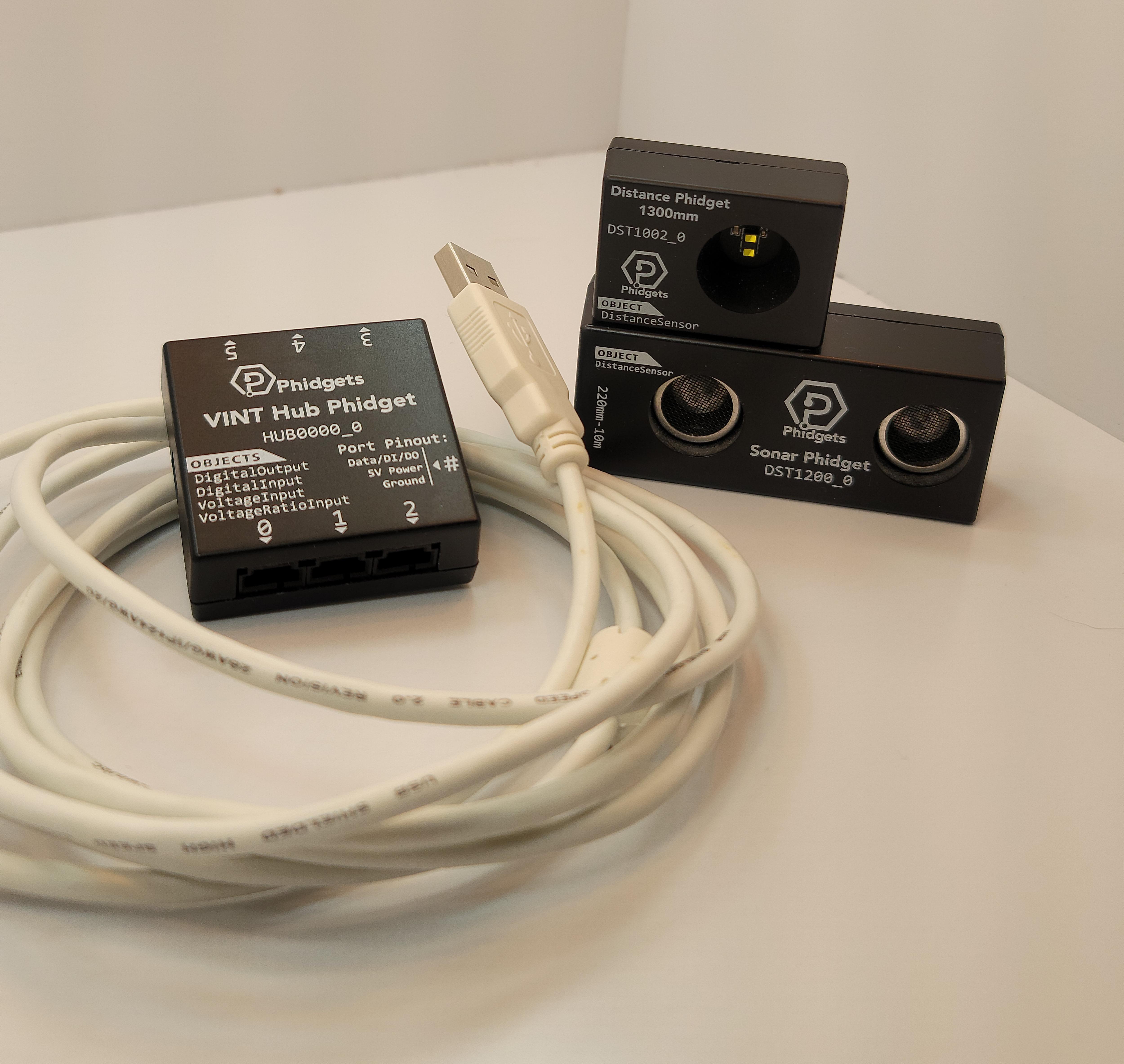
Throughout my work with small semi and full autonomous projects, one question always remains, and that is how to keep your rover, robot, drone etc... from running into something. The answer at first might be simple, but it's not always straightforward.
Throughout this Instructable, I will be going over some of the key strengths and weaknesses of two commonly used sensors, ultrasonic (sonar) or infrared time-of-flight. For this quick walkthrough, I'll be working with Phidgets sensors as they eliminate most of the hassle that other sonar sensors require in order to operate.
Supplies
Here is a list of the hardware I used:
- Sonar Phidget (DST1200_0)
- Distance Phidget (DST1002_0)
- VINT Hub (HUB0000_0)
- 2x VINT Cable
- USB Type-A to Mini-B
Connecting the Sensors
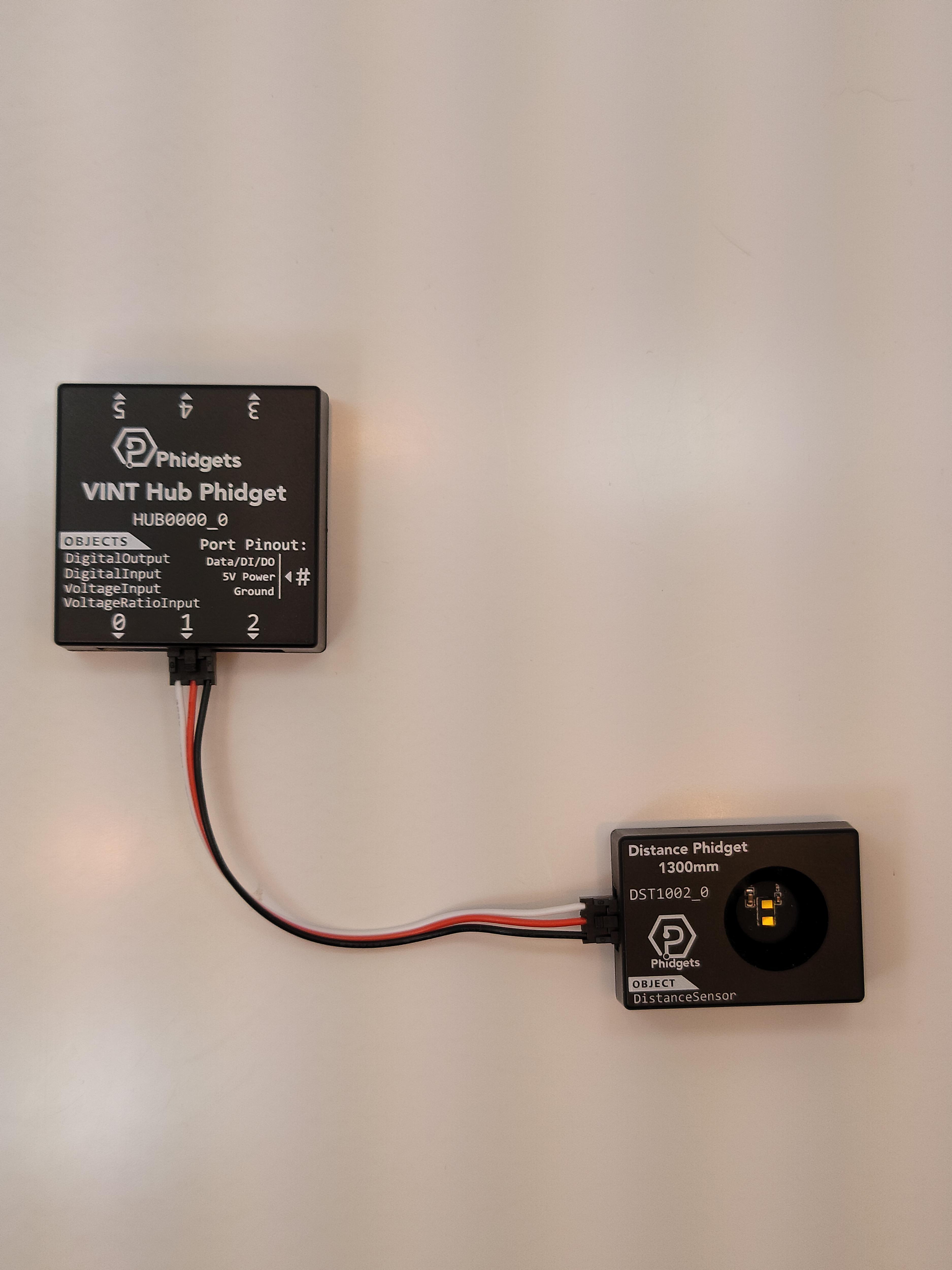
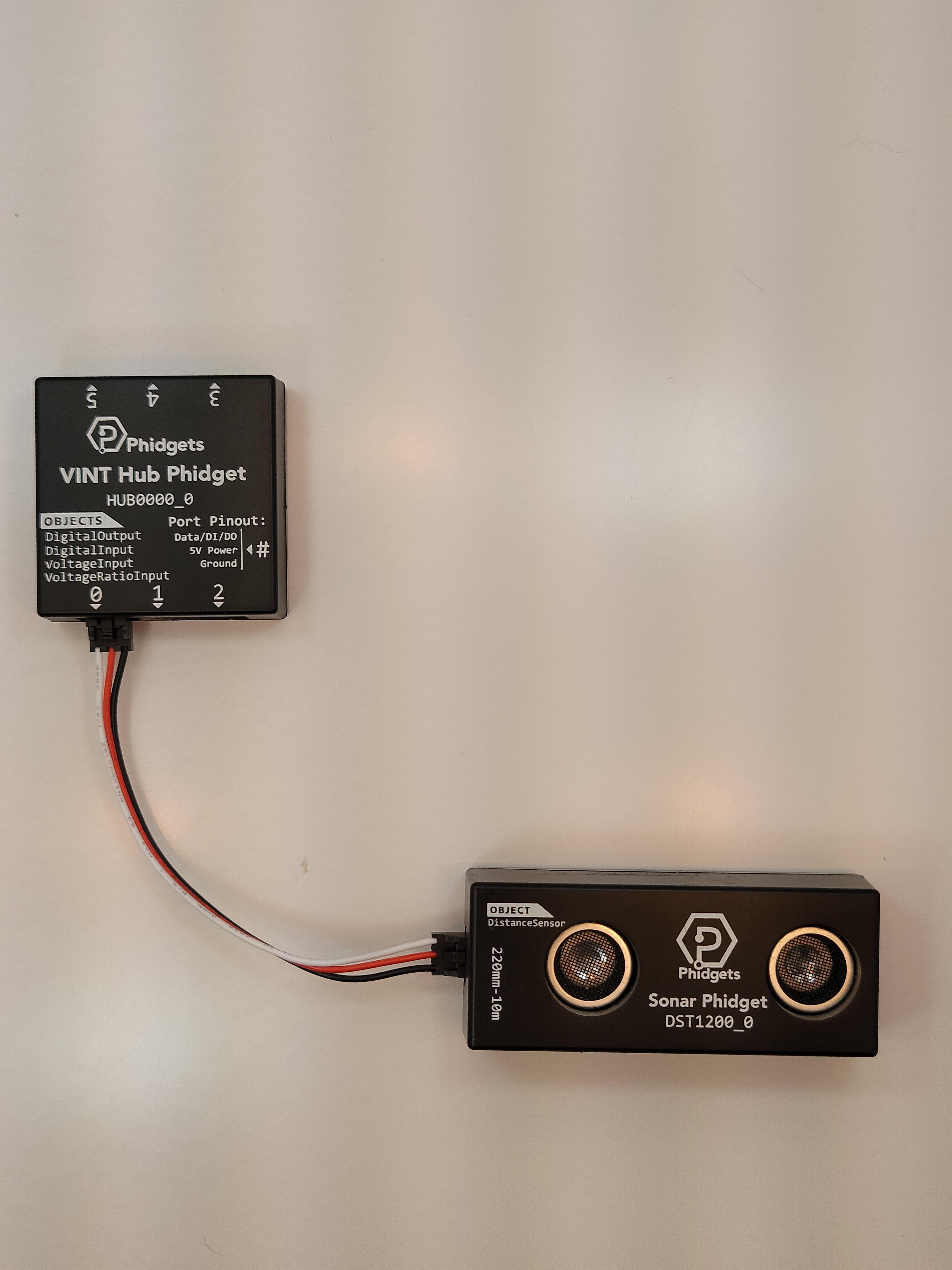
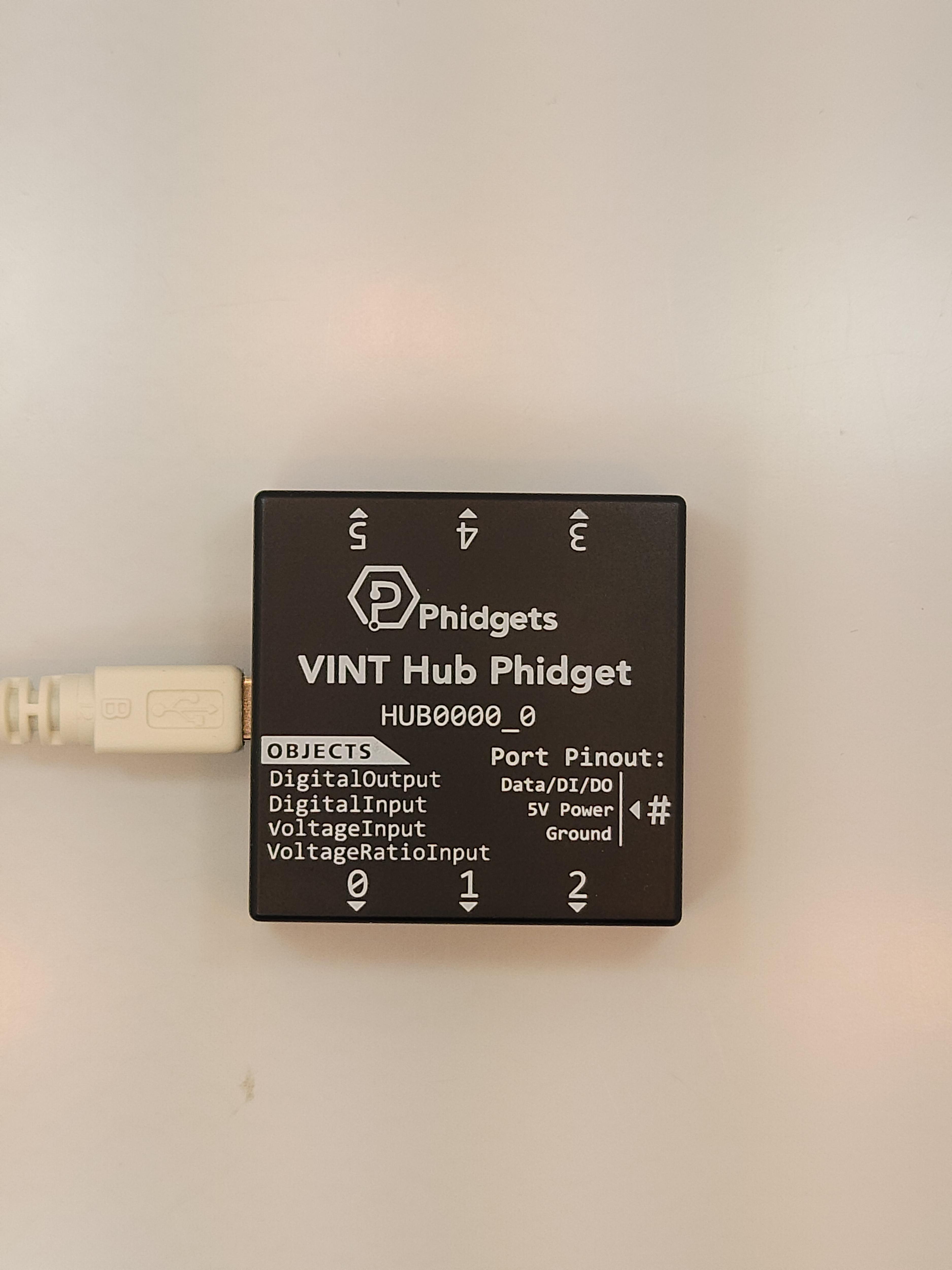
Just in case you have never worked with Phidgets before and want an idea as to the process of getting the hardware connected here is a quick walkthrough.
First, you will need a VINT Hub like the HUB0000_0, or the new HUB0001_0. Then simply insert a VINT Cable into each sensor and connect them to the VINT Hub. For now though only connect the Sonar Phidget, as it will make distinguishing between them easier. You should hear a slight click, but the VINT cable will lock in place once fully inserted.
Finally, plug the Type-A end of the USB cable into your computer and the Type-B end into the VINT Hub. Now you're ready to go.
Running the Sonar
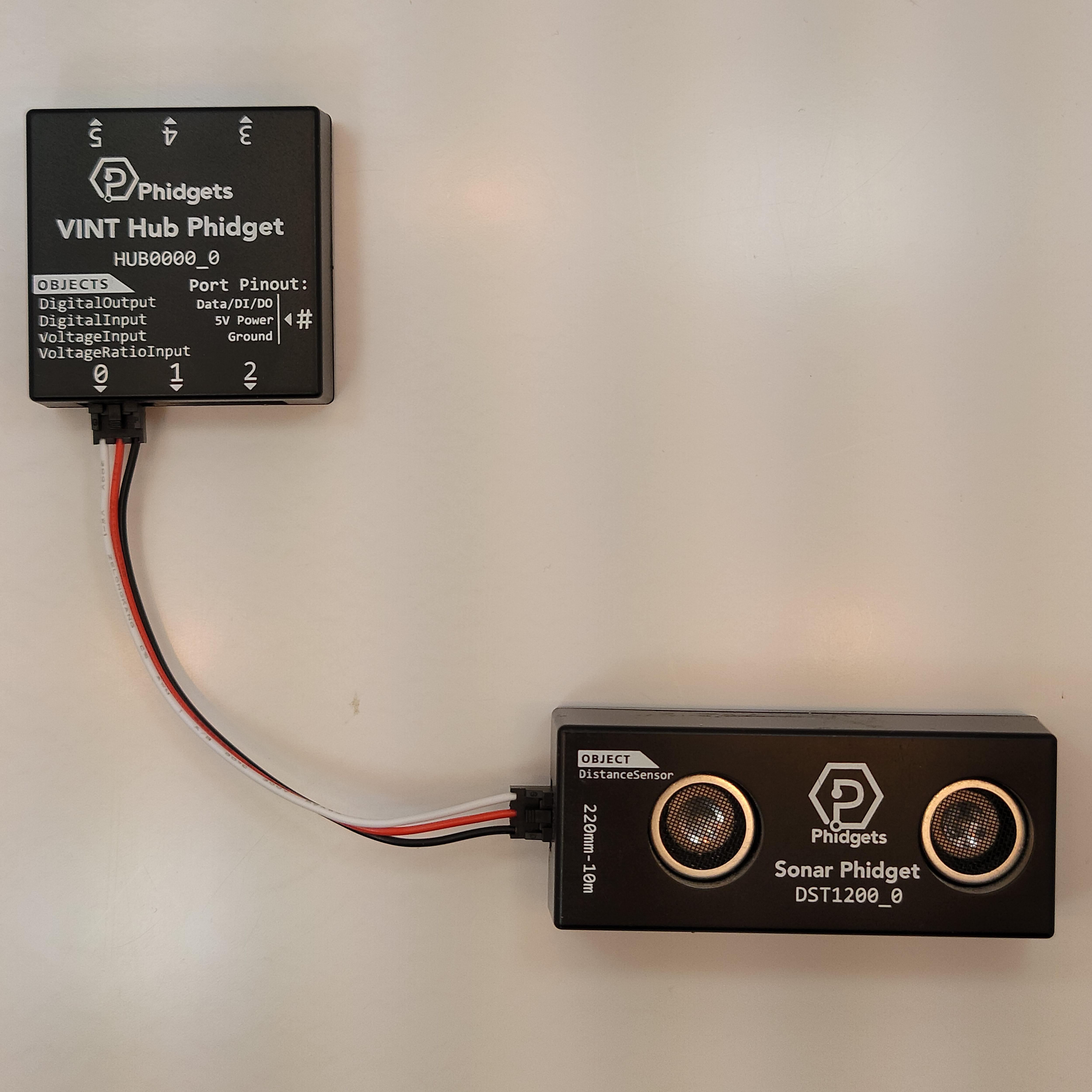
Now that everything is connected we will need a small bit of code to run the Sonar. If you have never worked with Phidgets before you might need to install the Phidget22Native Python package. If you're working in an IDE you can simply search the package like you would any other one, or if you are working through a command prompt simply type
python3 -m pip install Phidget22Native
Next, we need to create a small Python file, in this case I will call it sensortest.py. Within that file we will have to initialize the sensor and read data from it. I'm going to use a simple while True: loop in this case as I simply need the data to be written out. My file looked something like the following:
from Phidget22.Devices.DistanceSensor import * import time # Init new DistanceSensor Obj ds = DistanceSensor() # Open Distance Sensor, it will self attach ds.openWaitForAttachment(1000) # Start Loop while True: # Get and print distance print(f"Distance: {ds.getDistance()}mm") time.sleep(.1)
Once running you should see the distance in mm being printed to your terminal. Laying the Sonar facing up on your work surface start slowly moving your hand closer and closer to the sensor. As you do you may notice one of two things, the first being that the distance outputted to your terminal is decreasing, and the second being that your program might break if you get too close to the sensor.
This brings us to the first, and main limitation that you will run into while working with Sonar. In order for the sound wave to reach the object in front of it and bounce back towards the sensor, there is a minimum distance that it needs, which is marked on the front as well as in the datasheets. I'll talk more about this after we go over the time-of-flight sensor.
Using the Time-of-Flight Sensor
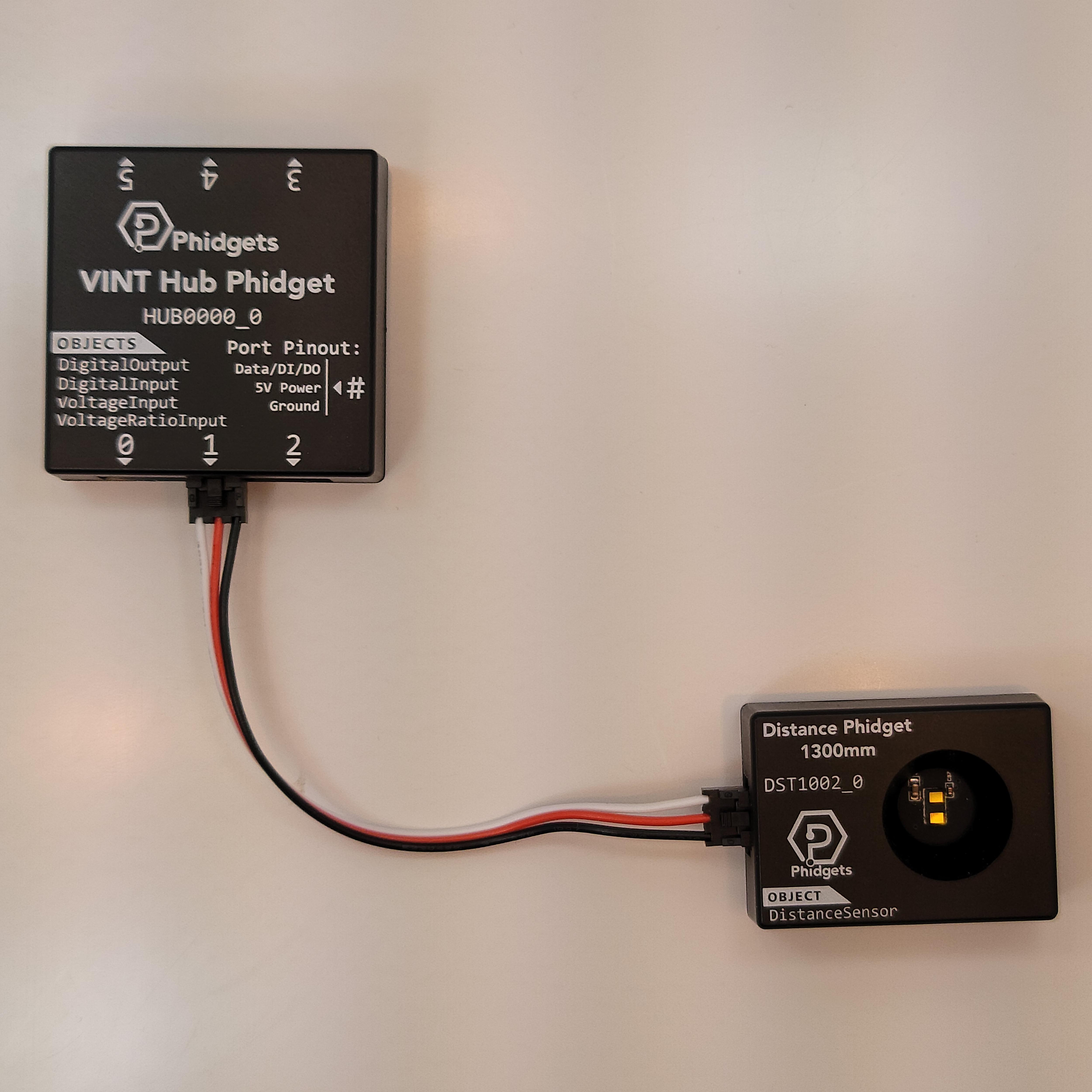
Now that we have an idea of the Sonars limitations we can pick up where we left off using the time-of-flight sensor. To start simply unplug the Sonar Phidget, and plug in the time-of-flight Phidget. Lay it face up on your work surface just like the Sonar, and run your program from Step 2 again.
The first thing you may notice immediately is that your program breaks every time it tries to get distance information, but wait! Try holding your hand about 5cm away from the Phidget, and run your program again. Now you should see distance information just like before. This time if you move your hand closer your program will keep running without a problem, it's when you get too far away that it becomes an issue.
Now we have discovered the main limitation that we will find when working with the time-of-flight sensor. Two major things contribute to this difference. Because the sending and receiving units are much closer together on the time-of-flight sensor than on the Sonar, and the speed of light is around 877,193 times faster than the speed of sound in air, we are able to measure much closer distances using the time-of-flight sensor.
Handling Unknowns
The main drawback of using infrared light over ultrasonic sound is the range in which we can reliably measure the light without interference is much smaller than that of sound. That being said having your program break when things get too close or too far is not helpful at all. Luckily for us, there is a way to fix that. All we need to do is add a simple try-except block around our measurement like so:
# Start Loop while True: # Get and print distance try: print(f"Distance: {ds.getDistance()}mm") except PhidgetException as err: print("Object outside of allowable range!") time.sleep(.1)
Now instead of breaking our program will simply remind us that the object we are trying to measure is outside of the allowable range.
What Do I Do Now?
So this brings up the point, what do you use. Personally, I feel all around that the Sonar Phidget is your best choice for most hobby projects. It allows you to get up to 40mm away from an object without error, but as I've shown we can determine when something is too close or too far simply by catching the exception.
That being said if you want to measure things much closer to the Phidget then the time-of-flight sensor is the way to go. Although for some it may leave a desire for a stopgap, and if you happen to have both sensors available I will show you how you can seamlessly measure distances from 10M to 0mm.
First, we need to plug both sensors in, so connect the Sonar to port 0 on the VINT Hub and the time-of-flight to port 1. Next, replace the code in your sensortest.py file with the following:
from Phidget22.Devices.DistanceSensor import * import time # Sonar Object sonar = DistanceSensor() # time-of-flight sensor ds = DistanceSensor() # Assign Ports sonar.setHubPort(0) ds.setHubPort(1) # Open sonar.openWaitForAttachment(1000) ds.openWaitForAttachment(1000) while True: try: # Get distance from sonar print(f"Distance {sonar.getDistance()}mm") except PhidgetException: try: # if too close get distance from time-of-flight print(f"Distance {ds.getDistance()}mm") except PhidgetException as err: print("Unable to measure distance.") time.sleep(0.1)
Now when running your program as you bring your hand closer to the two sensors you will notice that as you get closer the distance keeps getting reported.
Downloads
Conclusion
So now you know a little more about the difference between an ultrasonic and time-of-flight based sensor. As you may have noticed in Step 5 the program isn't perfect, and by no means is it the best or most efficient way of handling the changeover, but it certainly is one way. I would encourage you to try and write a better stopgap in your preferred language! I hope that the code I provided gives you a good starting point, and that you now better understand the main strengths and limitations of both sensors.
Thanks for taking the time to read this Instructable, and have a great day!