Field of View Changer for Call of Duty: Mw2 (2009)
by nschumac in Teachers > University+
86 Views, 1 Favorites, 0 Comments
Field of View Changer for Call of Duty: Mw2 (2009)
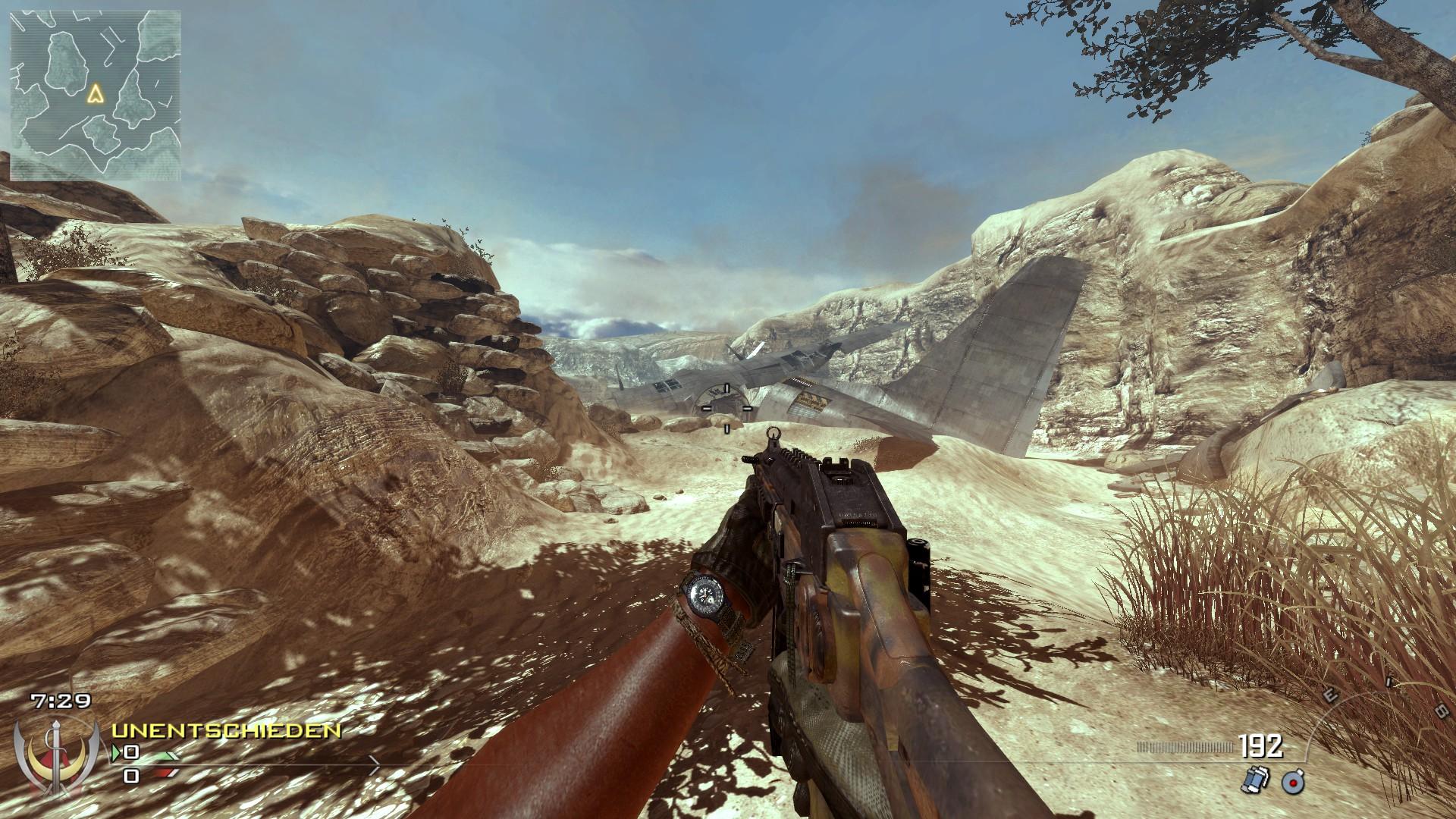
I've been a 'gamer' for quite a long time (currently i don't play as much anymore, but i used to) and occasionally I would run into so called 'Hackers'. I always wondered "how" they made the game change in weird ways, how they controlled it, seemingly magically.
Luckily i was able to talk with one who told to first learn the programming language c++, this is what got me into the whole world of coding.
After having spent a good year learning coding i wasn't really closer to writing a "hack" myself since there are a lot more things that go into it. After trial and error and a lot of 'learning', i finally managed to write something that does something in the game. EUPHORIA!
I'm writing this to get people interested in 'hacking' (it doesn't always have to be bad ;))
The program we're going to create modifies in-game memory of Modern Warfare 2 to give you more or less field of view. (this is not possible in the vanilla game)
Supplies
We will use Visual Studio Community to write our simple C++ "trainer" that will change the in-game field of view of Call of Duty: Mw2 (can be found on Steam).
To find the correct address we write to we need to find them in memory first of all and here is where use IDA a disassembler.
Installing Visual Studio Community
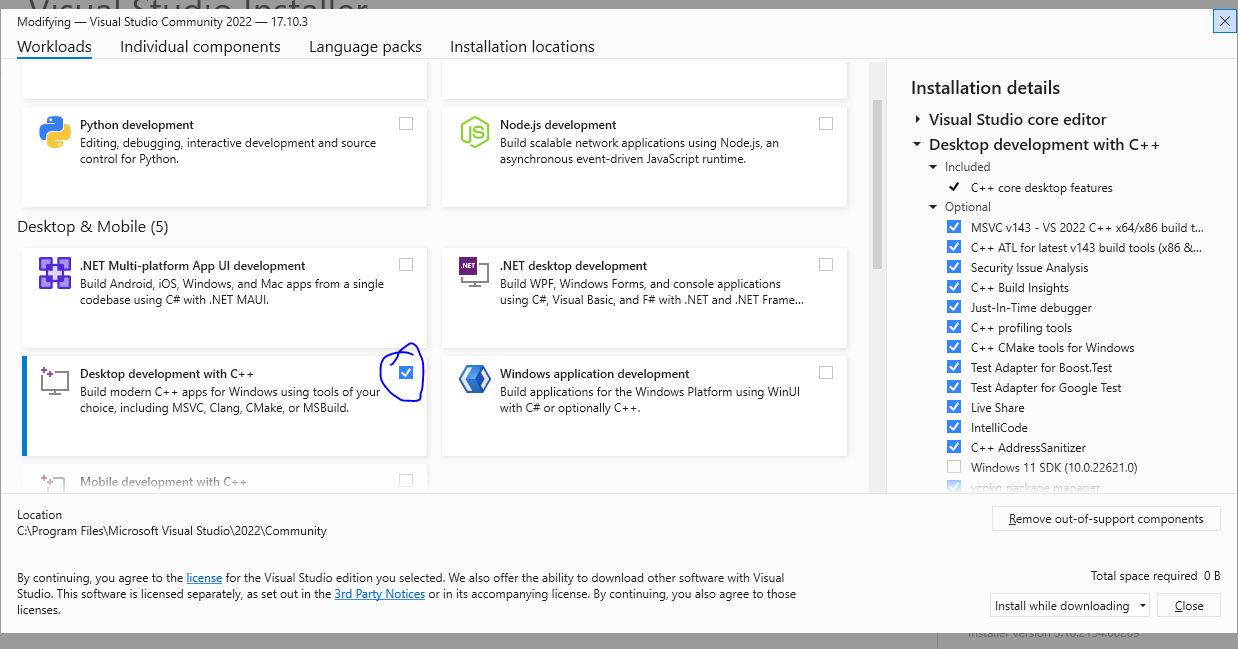
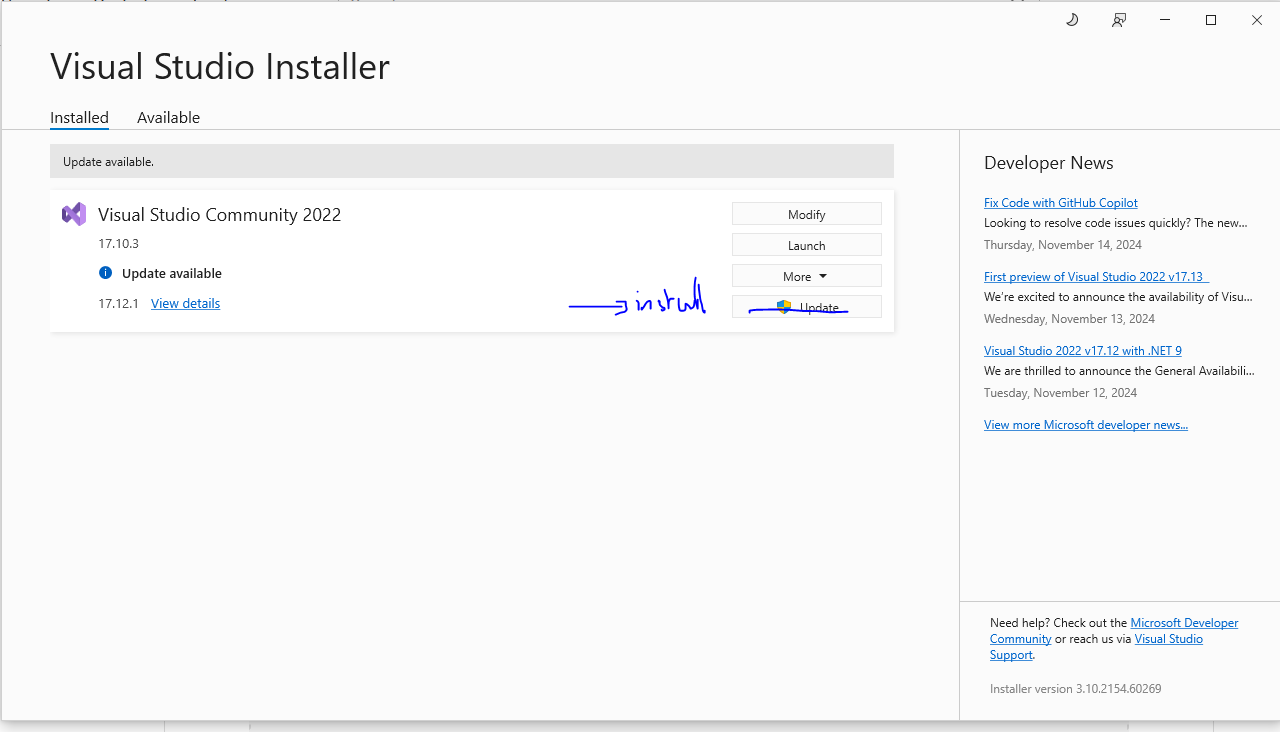
Please use the link provided and download the visual studio community installer.
Upon opening the installer please press modify and select the c++ development.
After which you can install visual studio
Might take some time ;)
Downloading Steam and 'installing' Mw2
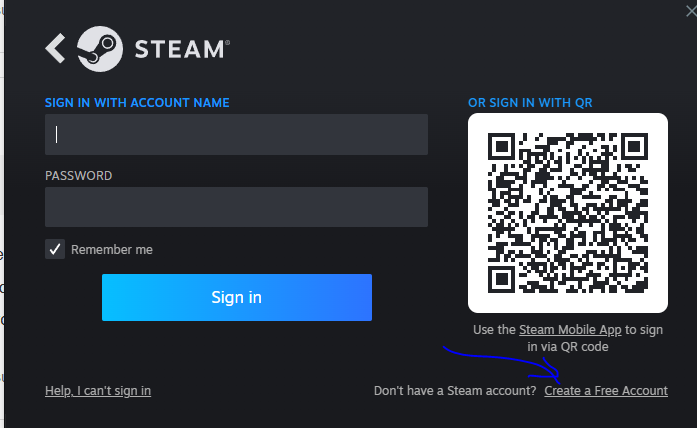
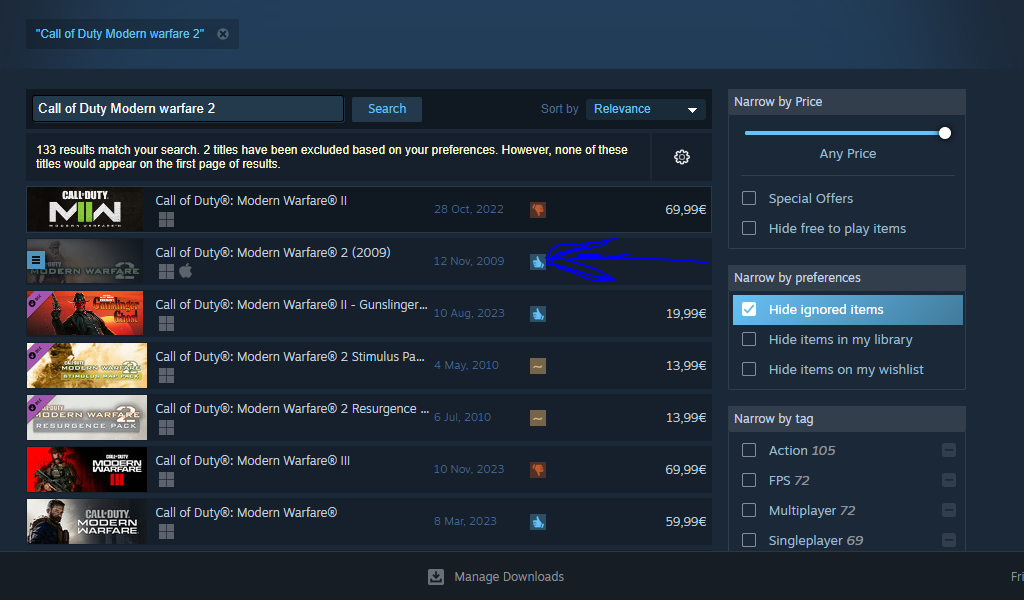
Please use the link provided to install the steam installer and run it.
Once it's complete create a steam account (free)
After which, locate the 'store' page (Top Left) and search (Top right) for 'call of duty: modern warfare 2 (2009)'
Locate your library and download the game :)
Install IDA
Use the link and download / install the free version of IDA
Writing a 'Hello World' Console Program in C++
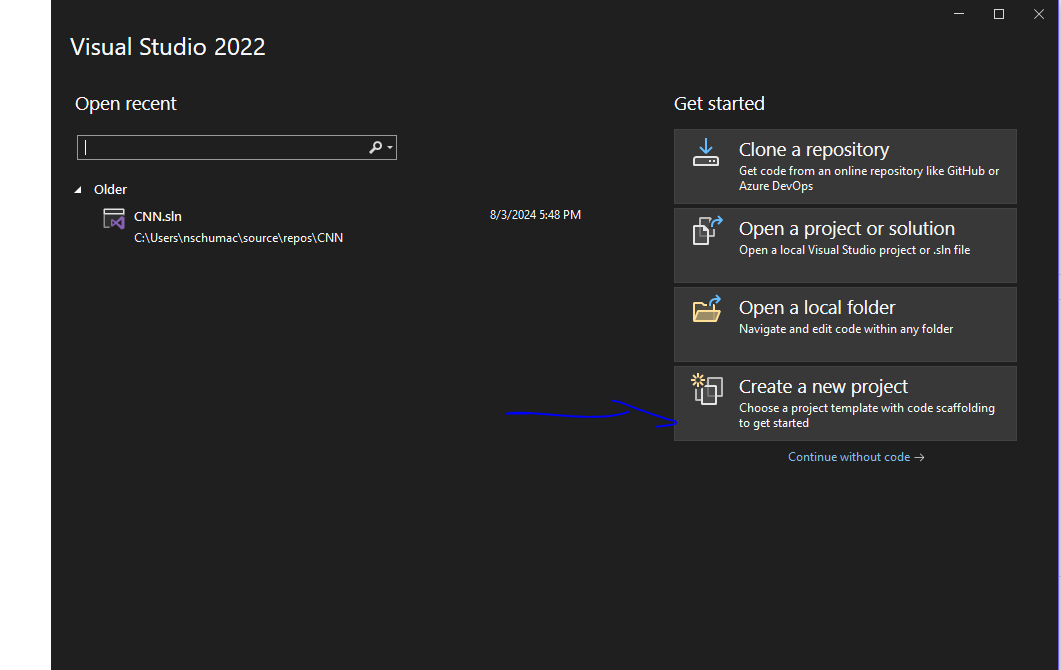
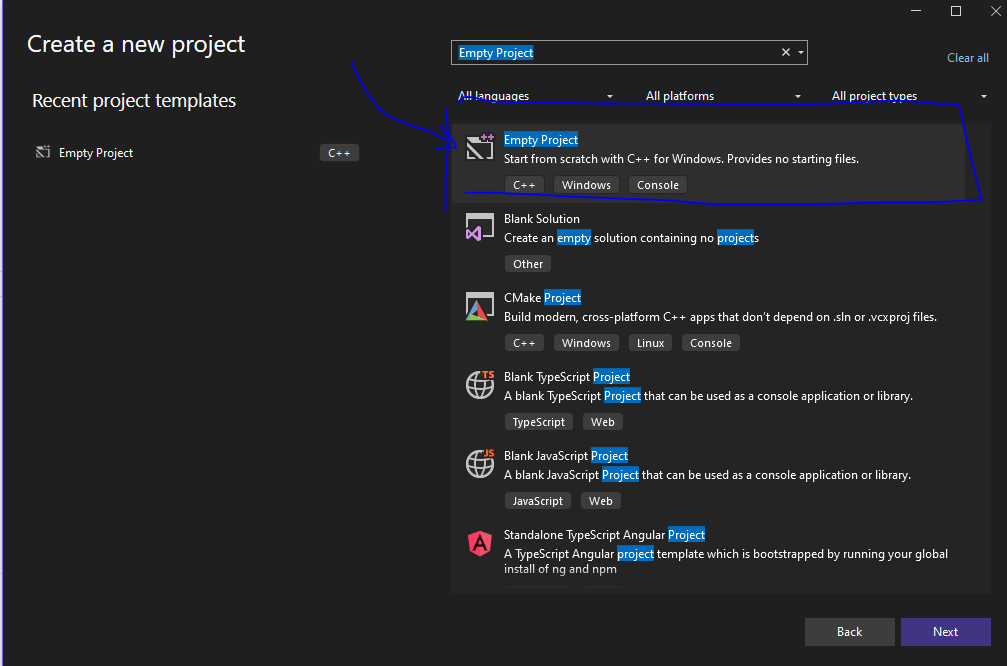
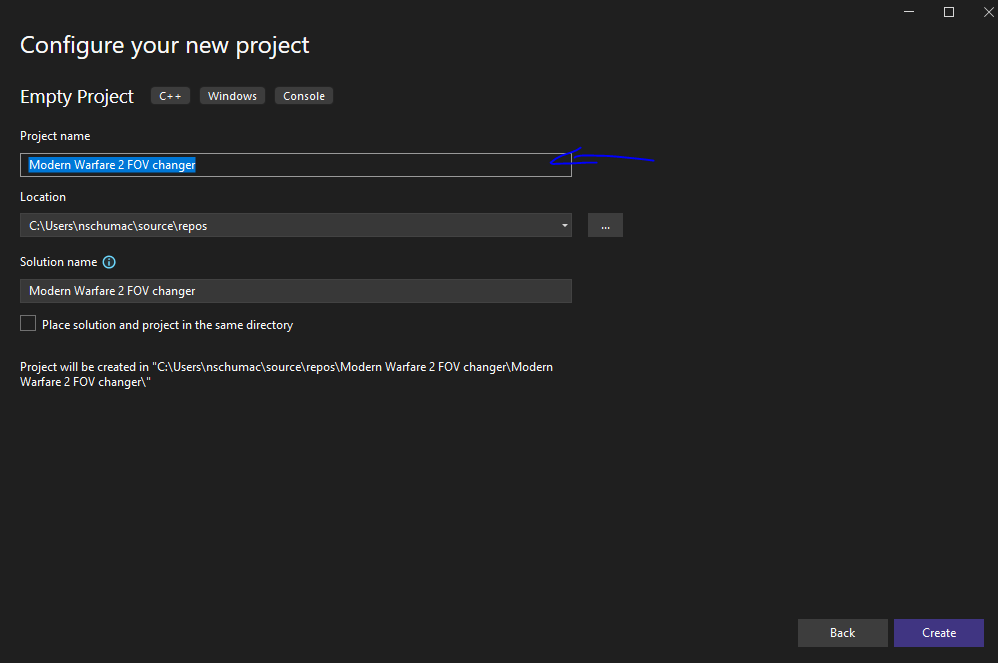
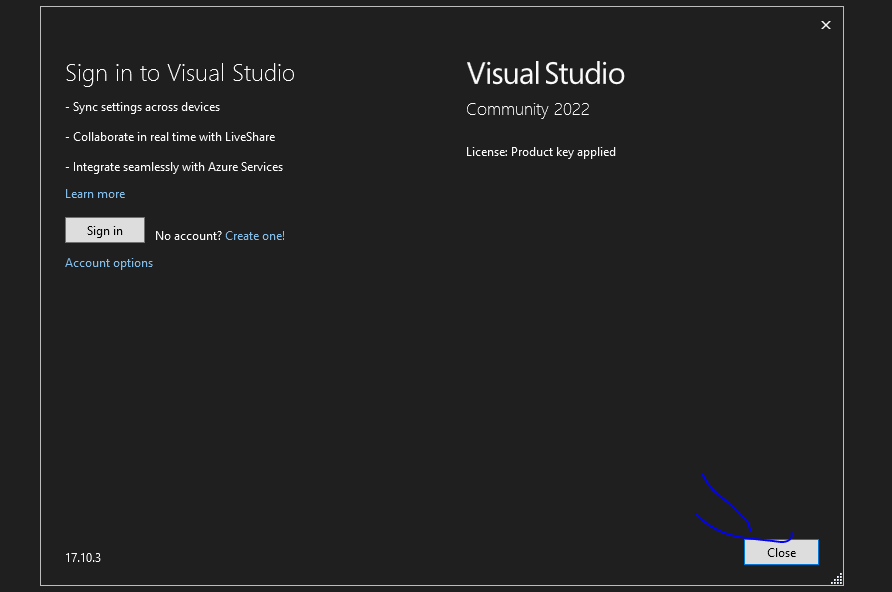
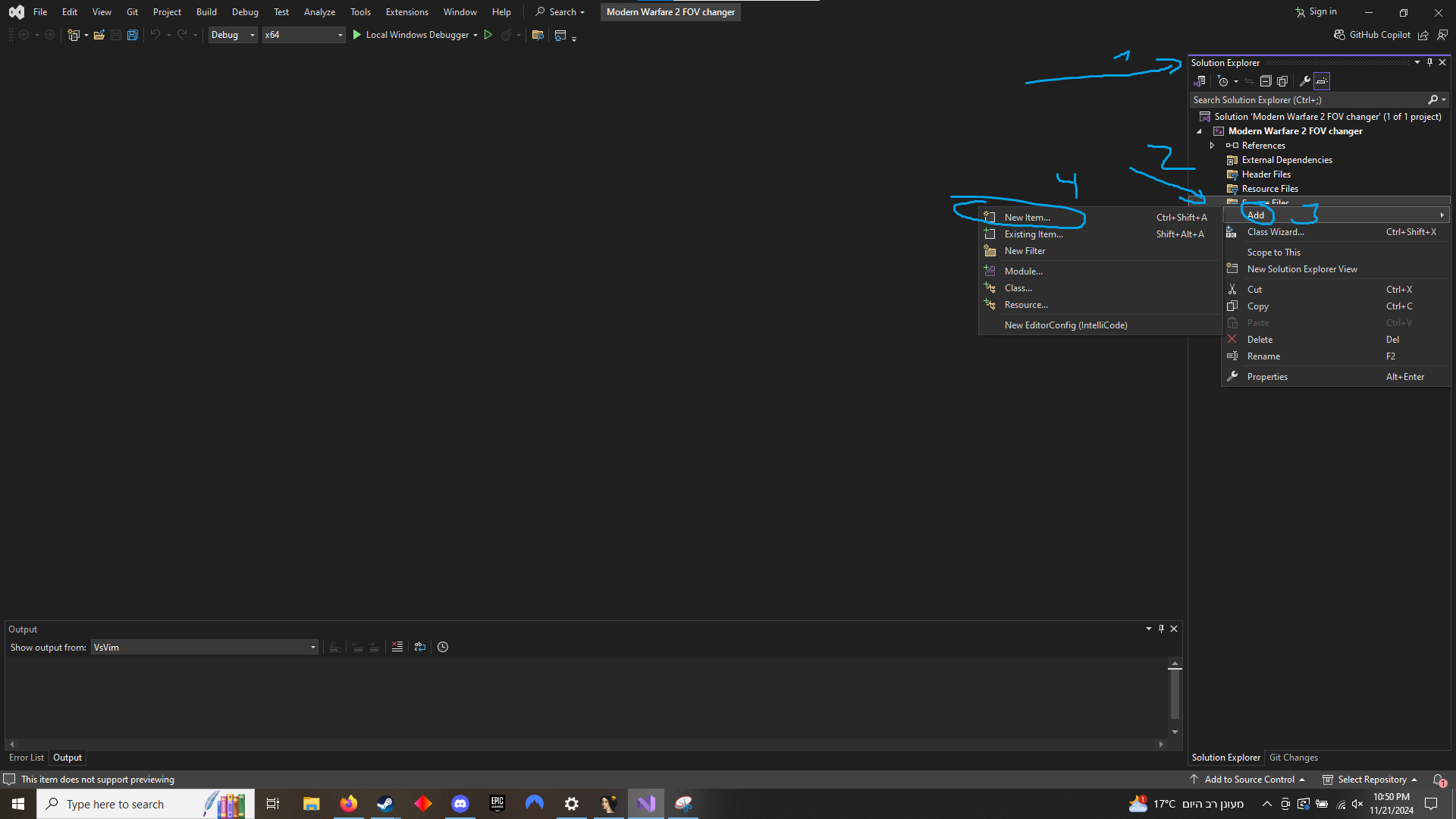
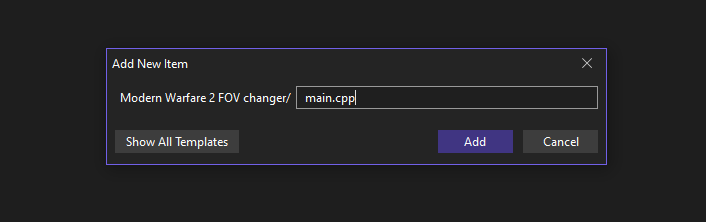
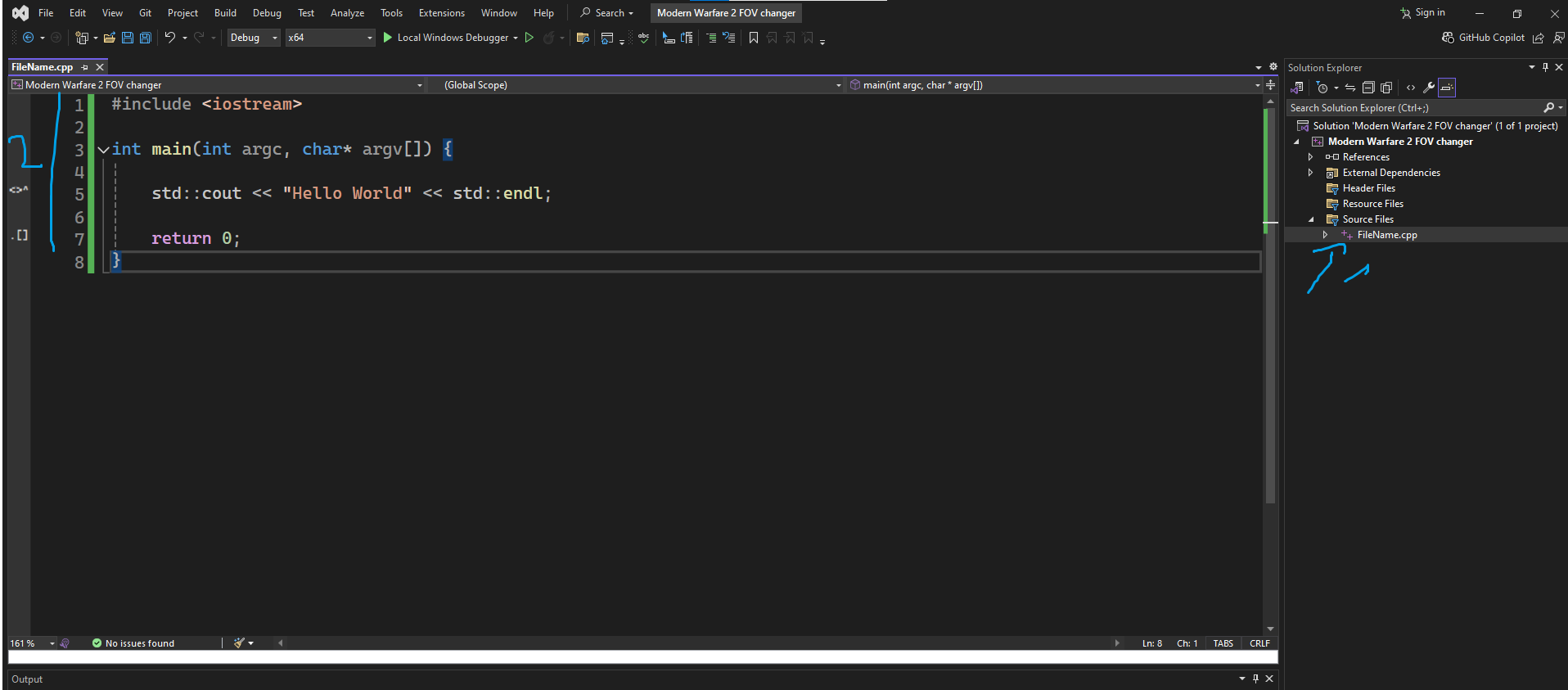
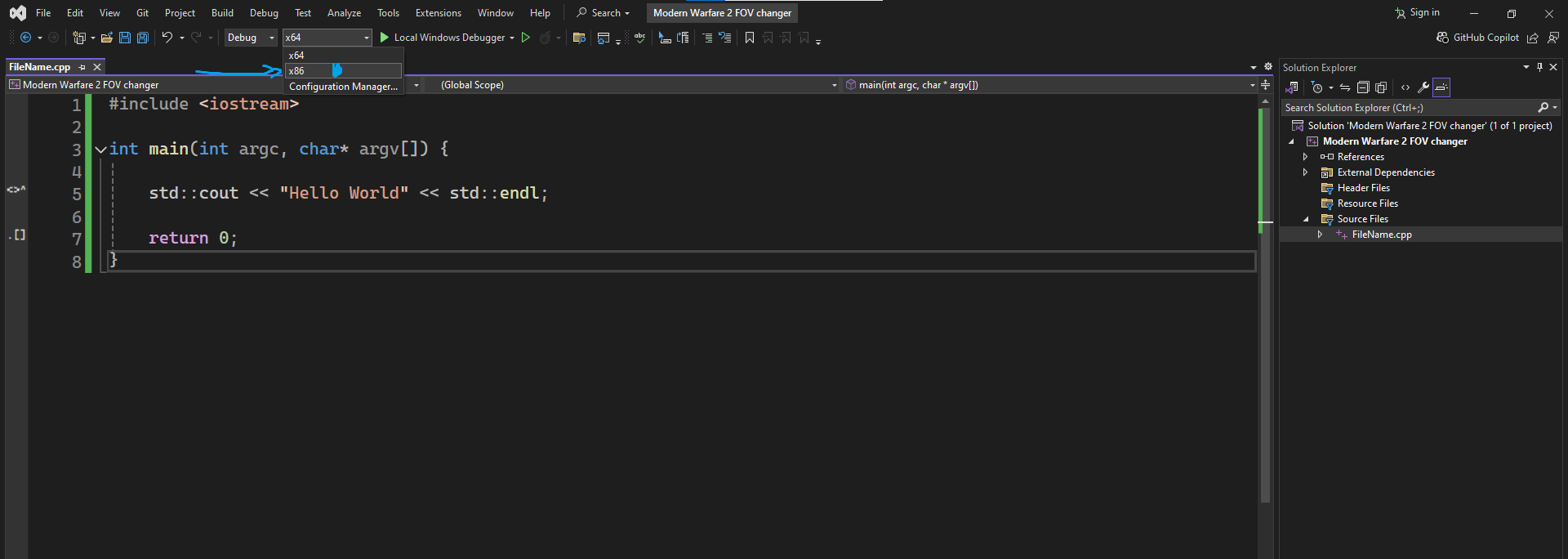
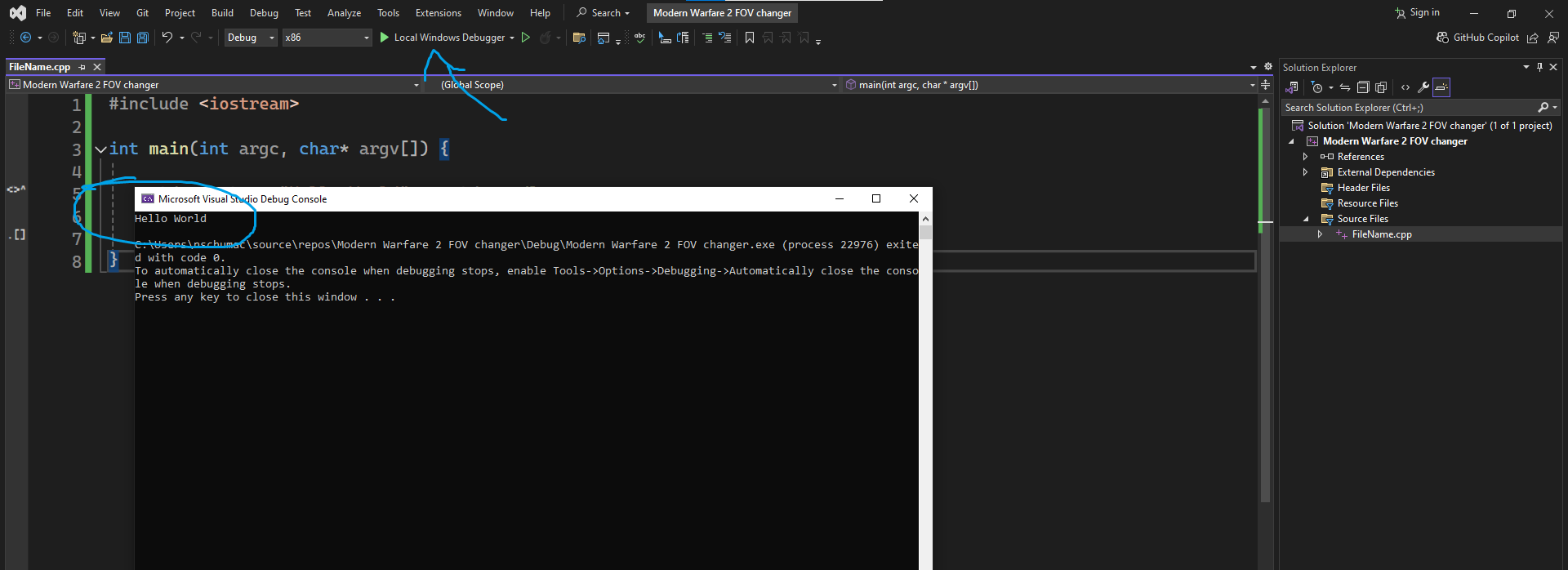
Open visual Studio Community
Select create a new project -> empty project c++
Name it and maybe select a folder where it should save the project files
Once it's finished opening the new project right click on the solution explorer and add a new 'item'
Add and Open the new .cpp located int he solution explorer
This is a simple c++ program that.
- Execution starts in the main function
- we include the iostream header which houses the std::cout function (used to print to out stream)
- then we return 0; from main
Then Select the x86 compiler and run it via the local debugger button which will spawn a console and show you hello world :)
CONGRATS you wrote a c++ program!!!
Finding the FOV 'offset' / 'Address'
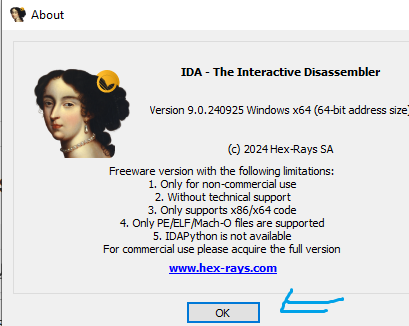
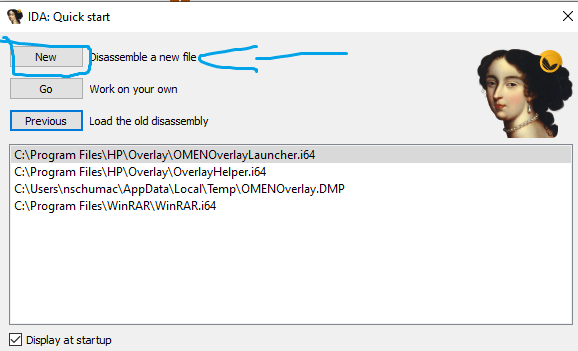
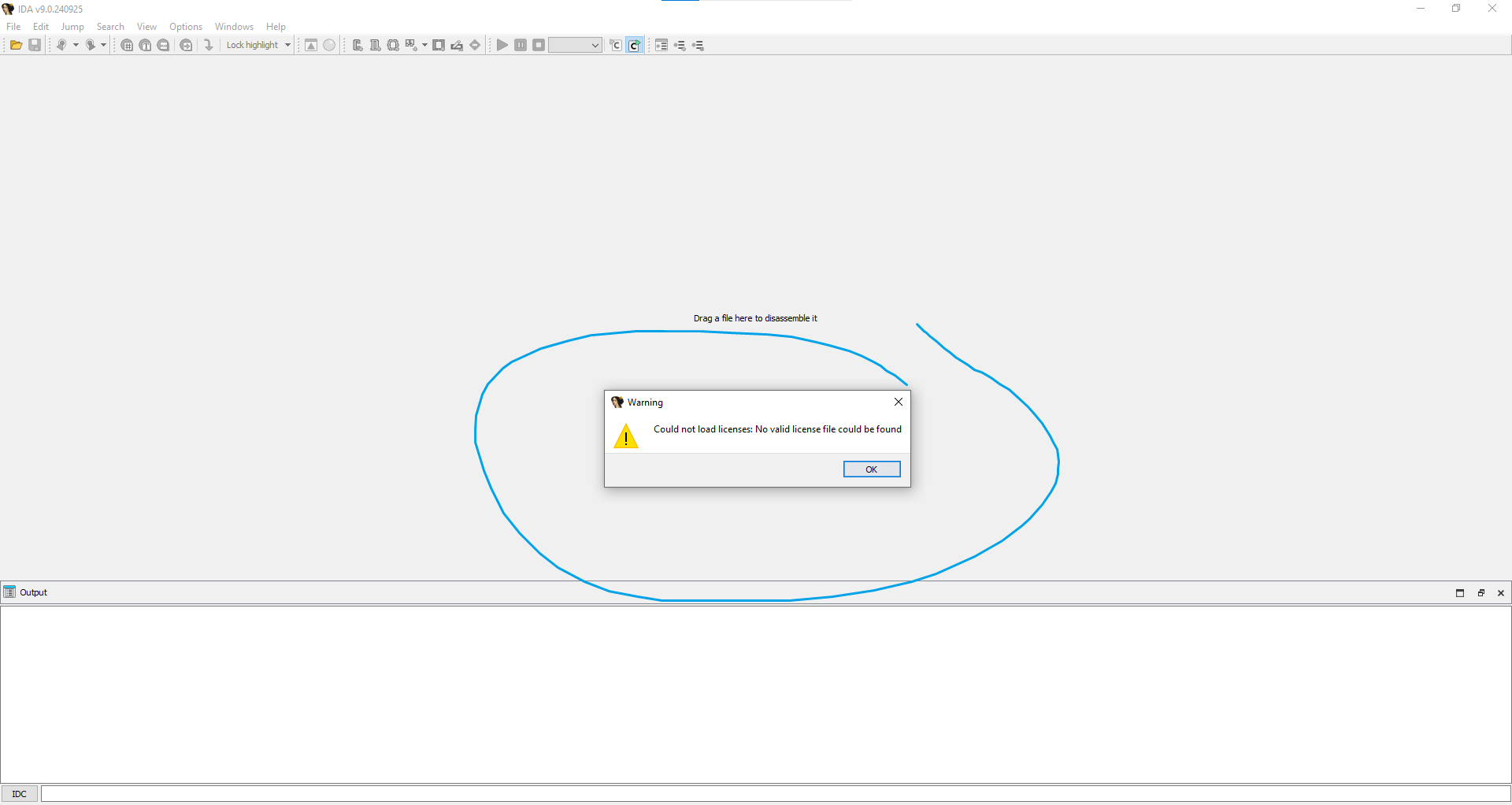
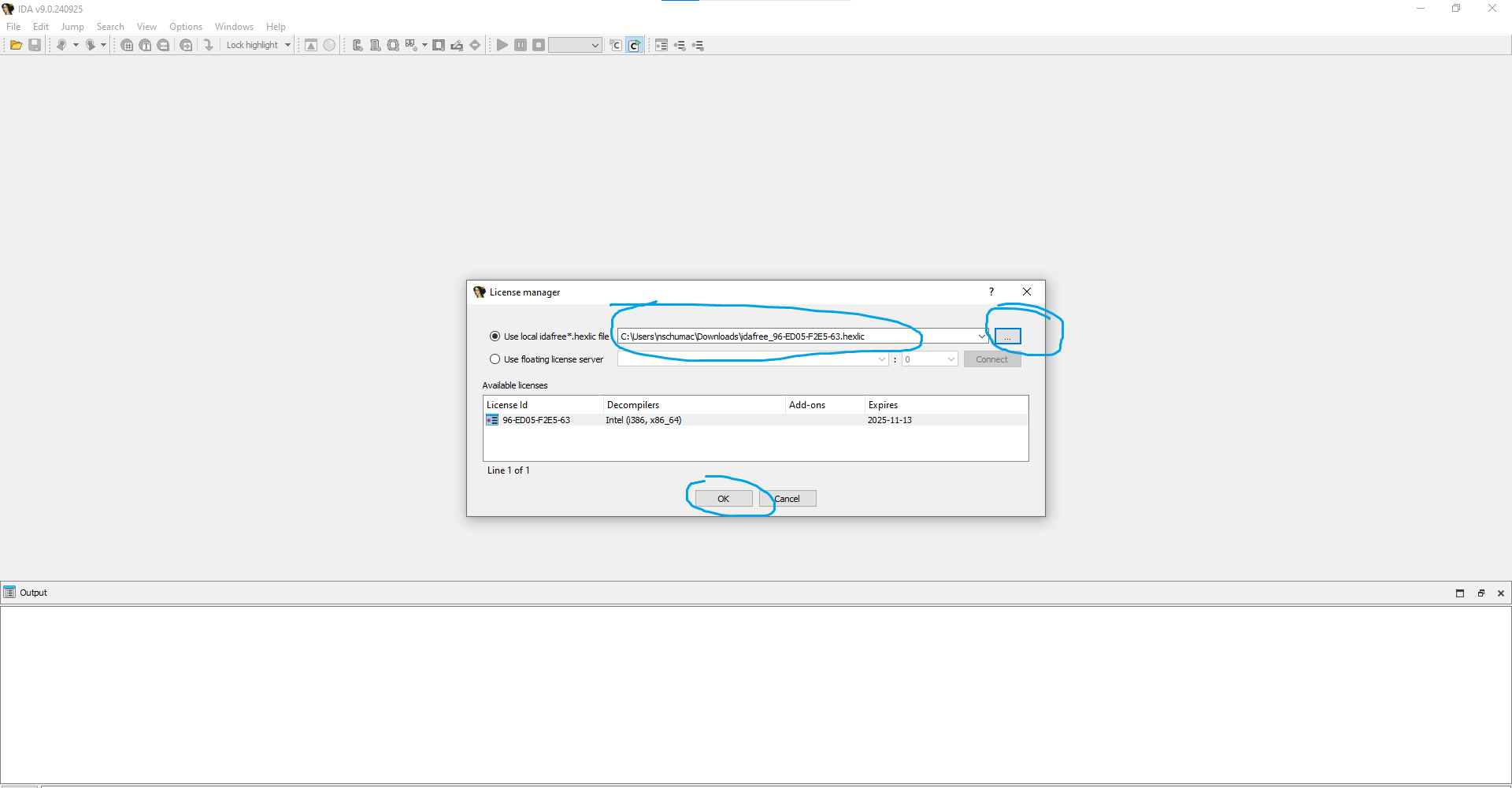
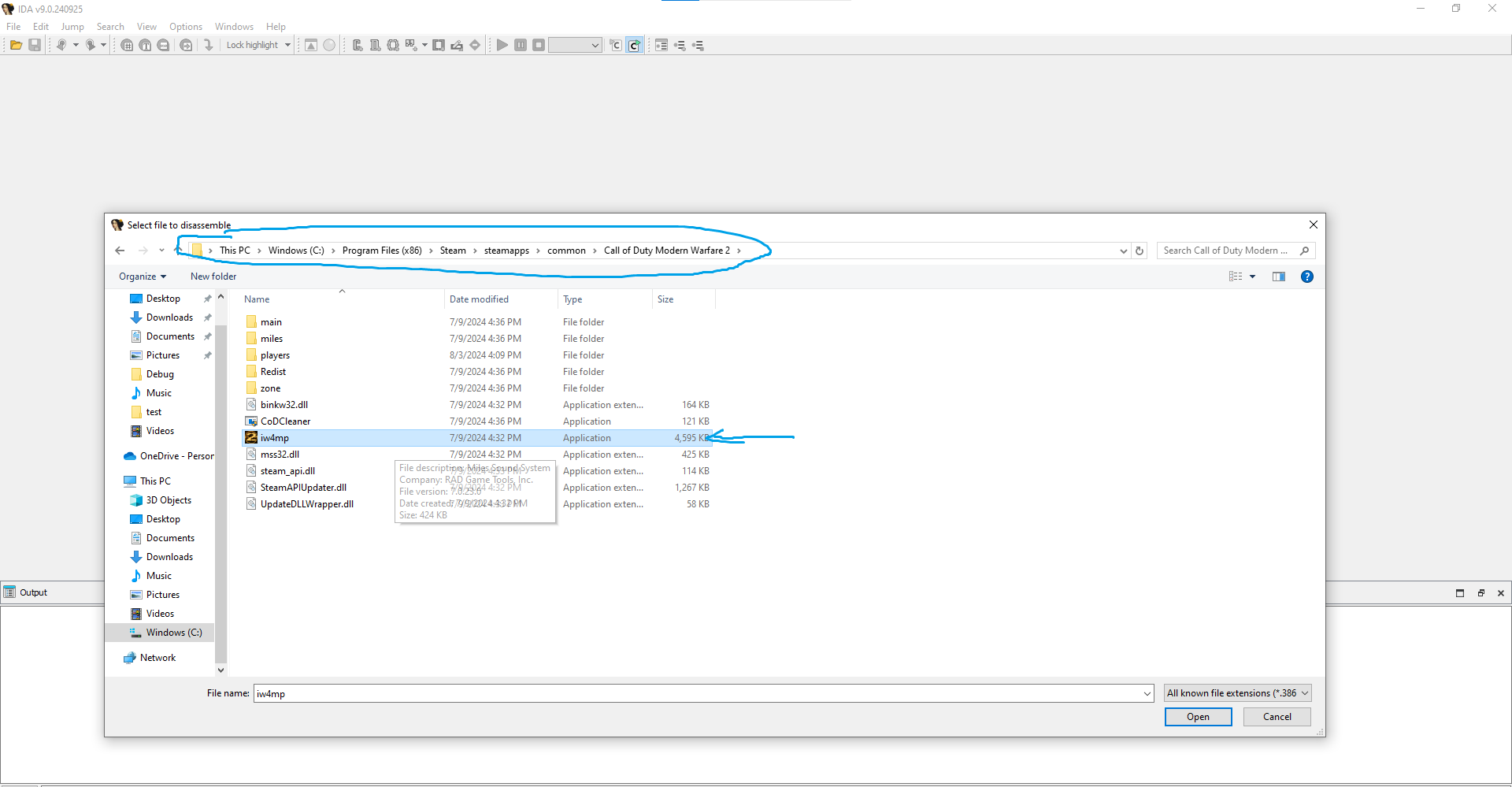
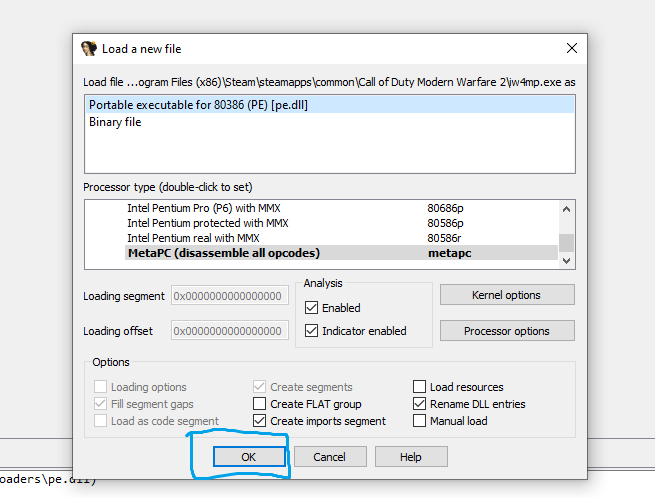
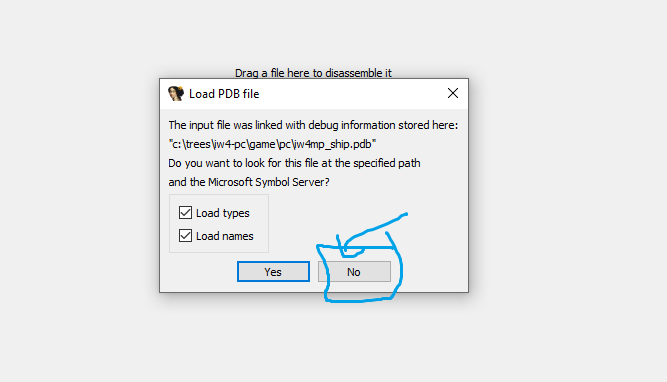
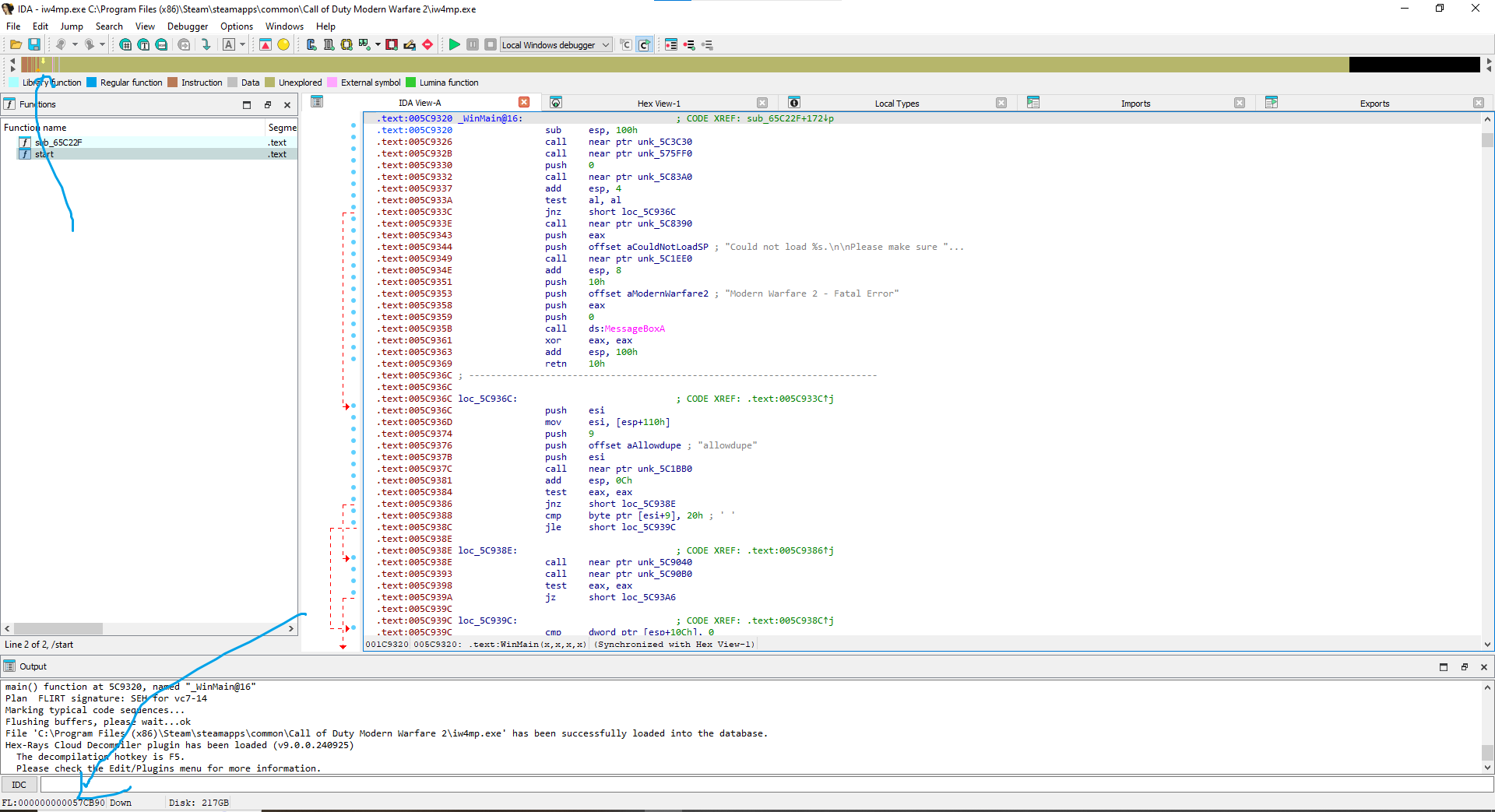
Open IDA which should be installed otherwise see step 3.
Launch IDA
Select new which will open the dissassembly window
It might request you to add the license, don't worry download it from your IDA account and select it.
Once open it'll request a file to dissasemble select iw4mp.exe foudn in the common directory of the steamapps
press ok, IDA automatically knows what file type it is from the signature.
Press no, as there are no PDB files to load (these are created normally for debugging purposes, but ofc won't be shipped with the game)
wait for it finish indexing / loading hte exe :)
Finding Cg_fov Dvar Address
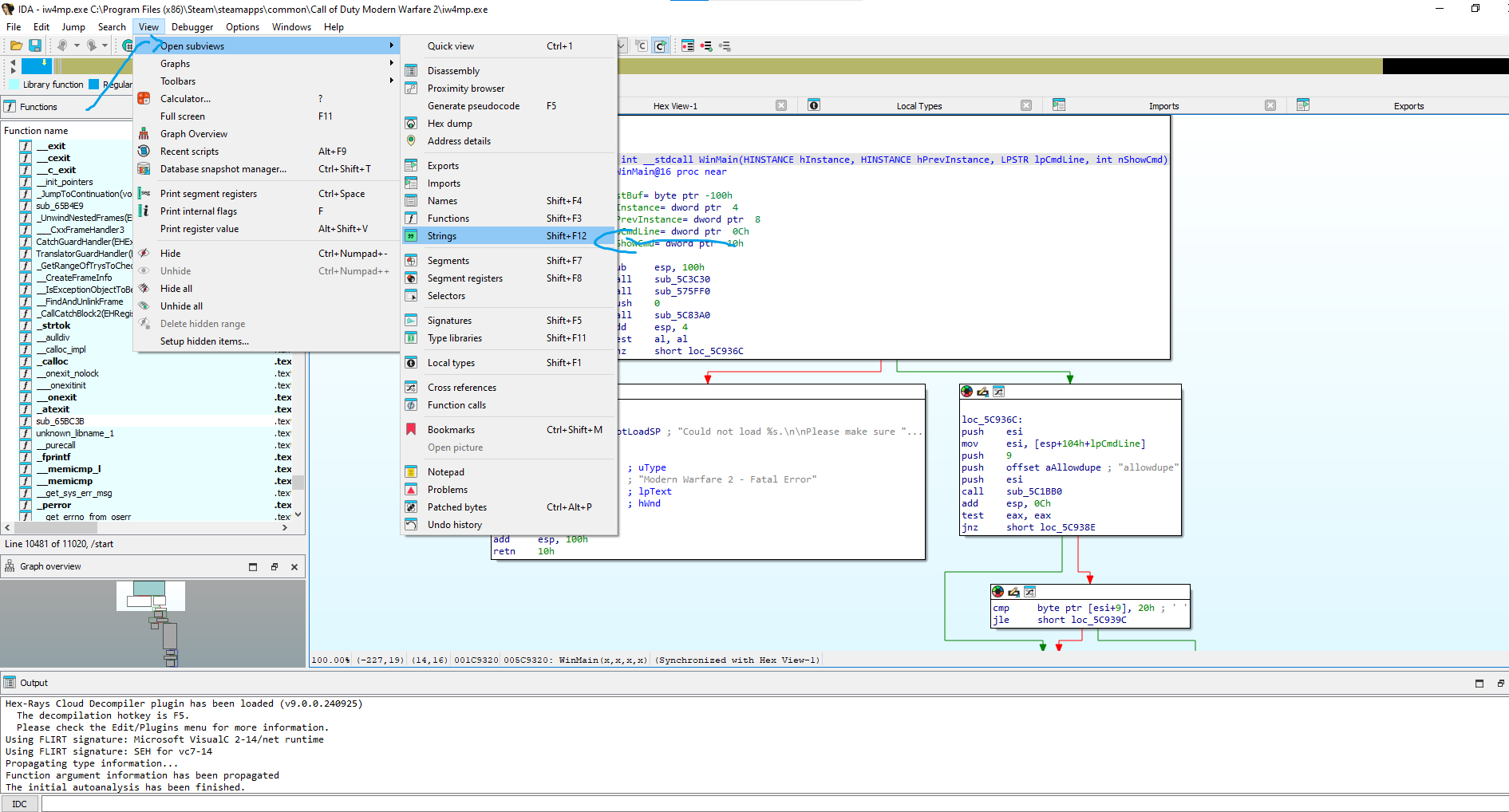
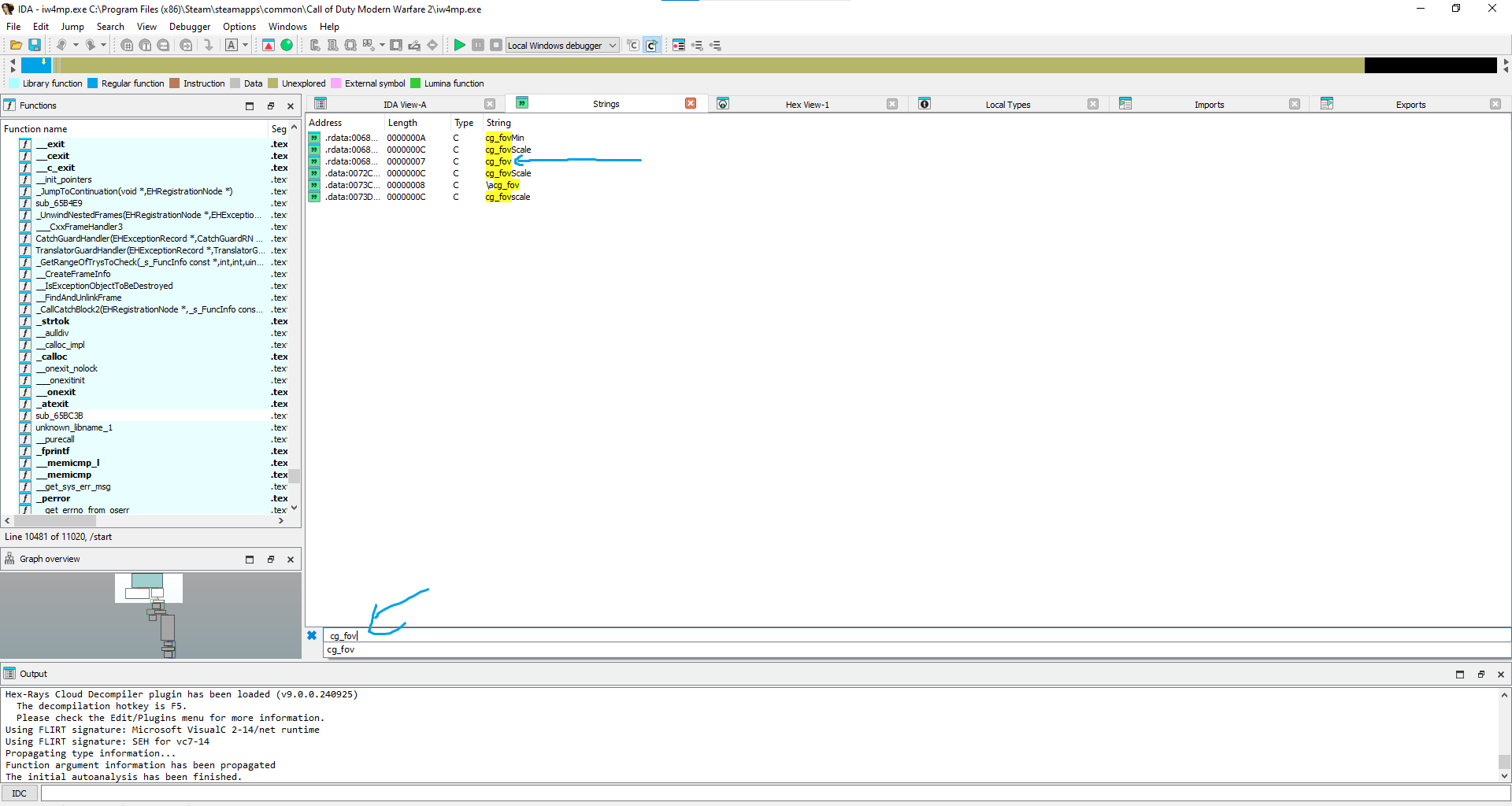
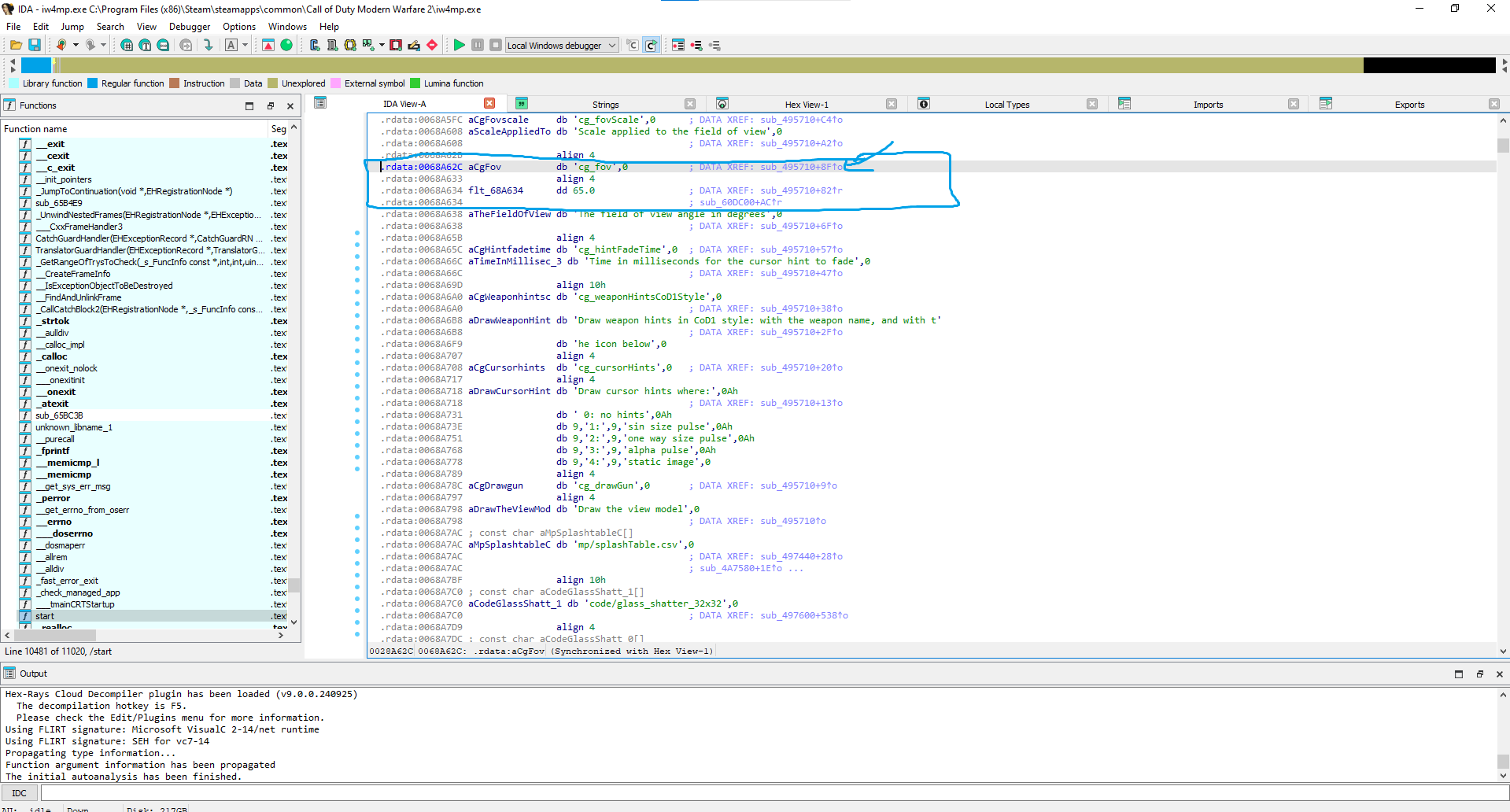
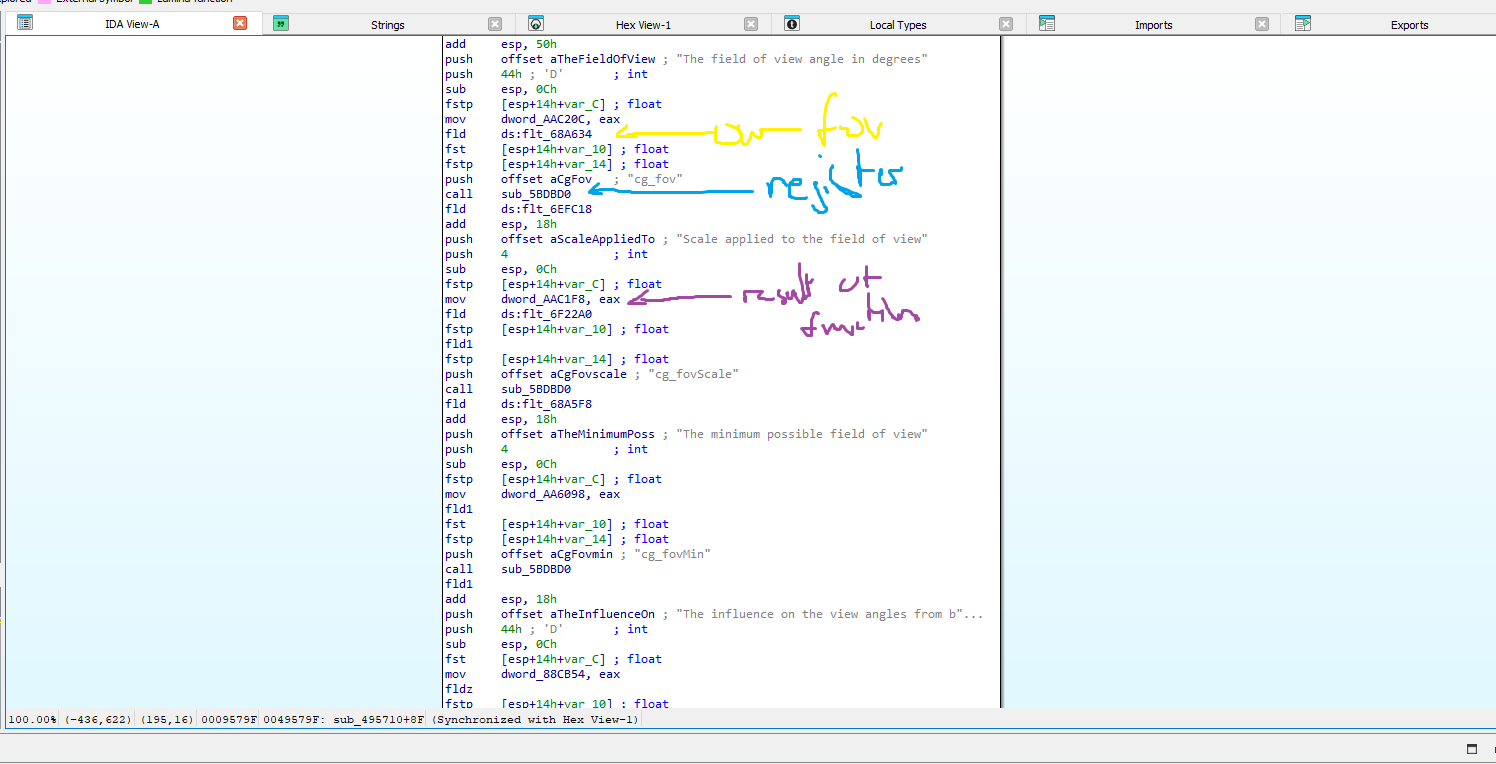
Open the subview for strings
This is all strings that IDA found in the executable.
We will search for 'cg_fov' as this is the name of the 'dvar' (diction variable, activision used a dictionary for all these kind of variables and values, things like fov, gamma, a bunch :)) you can find others by searching for cg_ or just by snooping around in the strings and functions.
once found double click the cg_fov string which will bring you to where it is being stored
double click on the function indicated by the sub... next to cg_fov.
this is the function that references this string, thus normally you would look at and see where and why it is being called. I will the reason, it is to register the dvar (this is called once at the start of every new game i believe, or it might be every time you respawn)
You'll be brought inside the function and you'll see this code
As you can see are claling the register dvar function and loading the result in the address dword_AAC1F8
Finding and Editing Fov From Dvar Address
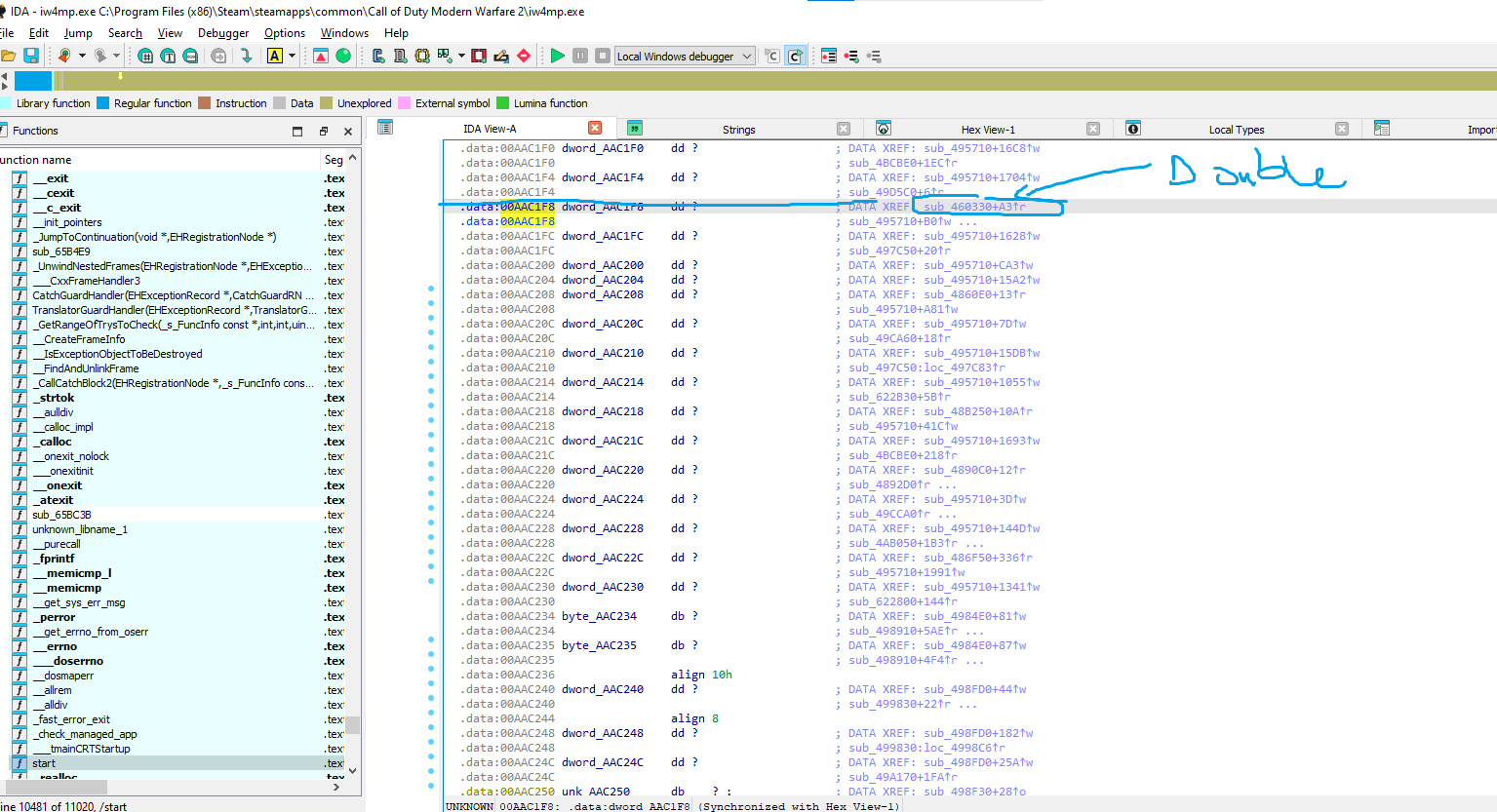
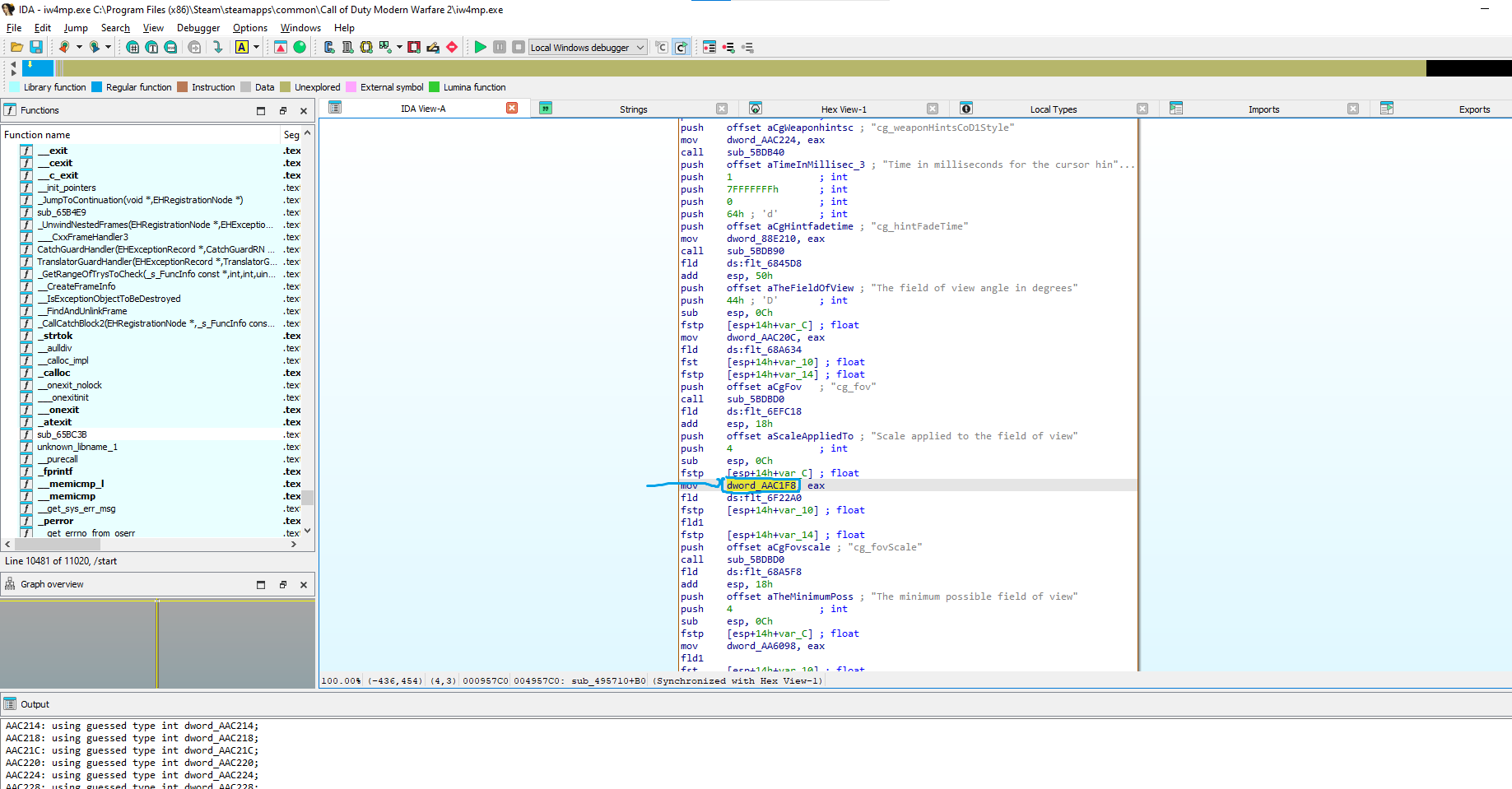
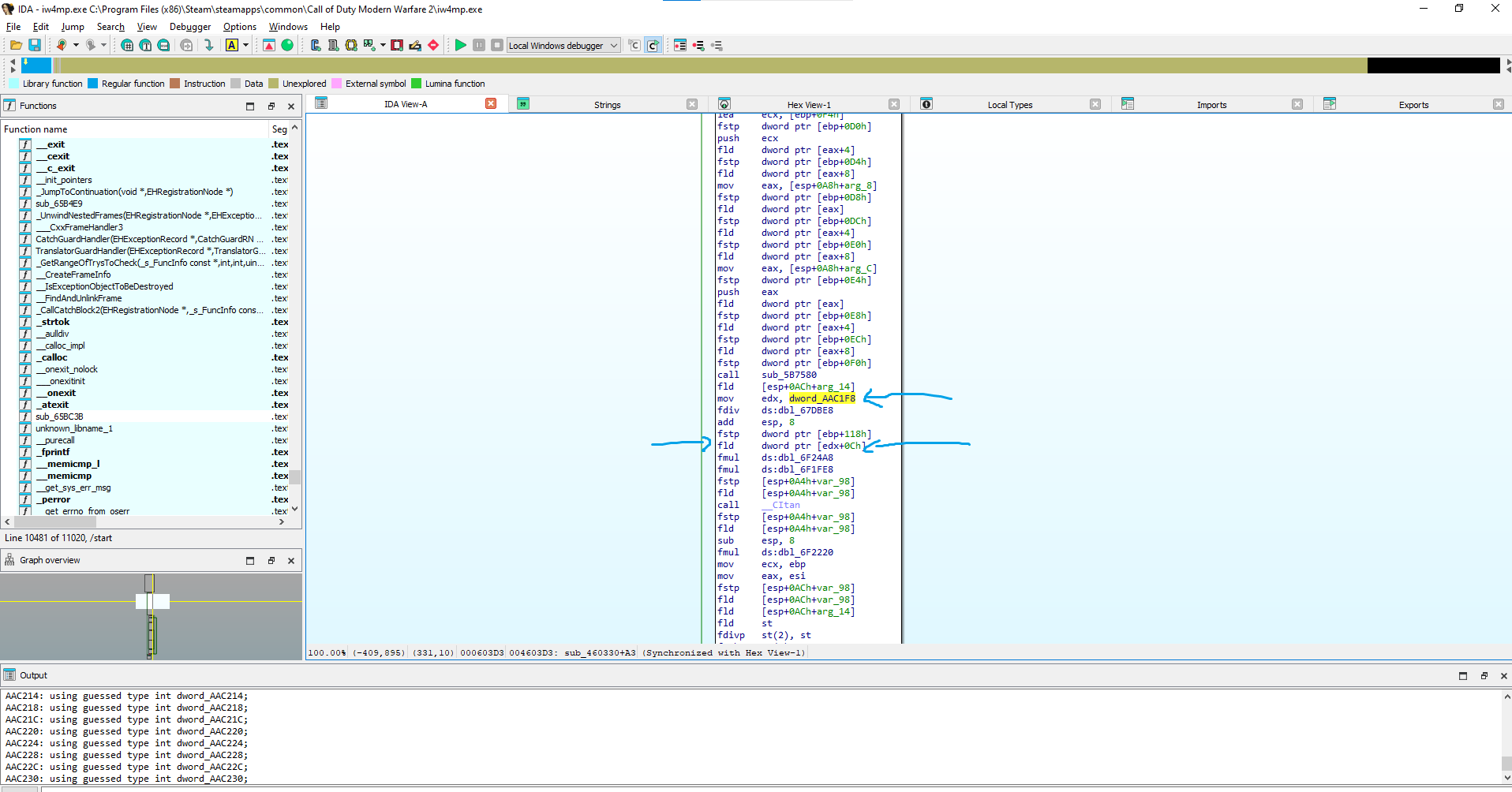
Double click the dvar address found in the previous step.
This will bring you to the address of the cg_fov dvar.
From here we can use the references to find where it is being called / used (double click first function)
Here we see the cg_fov is ebing loaded into register edx, after which 0xC is being added as an offset.
If you analyze the function from step 6 you can see that the value 65.0 (defualt value of fov) is being loaded at this position
thus all we need to do change our fov is
cg_fov dvar = readMemory(0xAAC1F8)
writeMemory(cg_fov_dvar + 0xC, <FOV> );
Hold on to that memory address and offset '0xAAC1F8', '0xC'
Writing a Program That Gets the Pid of a Program by Name
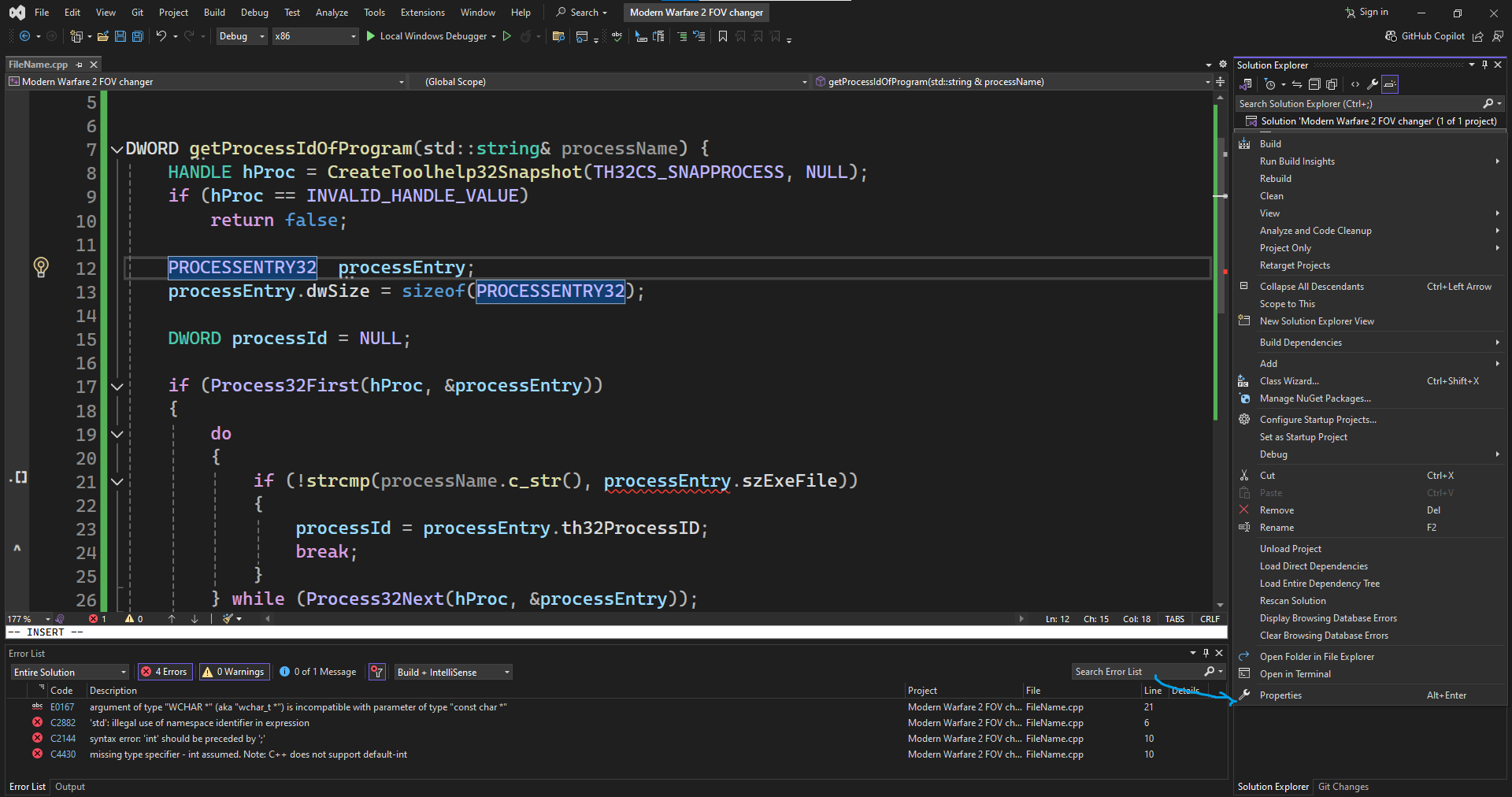
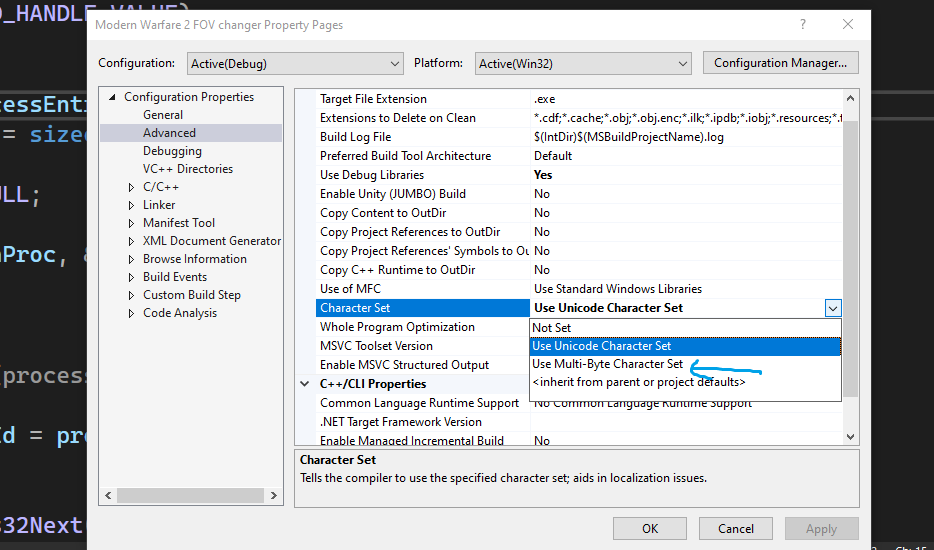
Before starting to write make sure to set your program to use the multibyte character set instead of the unicode otherwise certain functions might function differently (you can always play around and see)
This code basically iterates over all processes and if you find one that has the same name as the one we are looking for "iw4mp.exe" (modern warfare 2) it will return the pid.
Get Game Handle and Write Fov
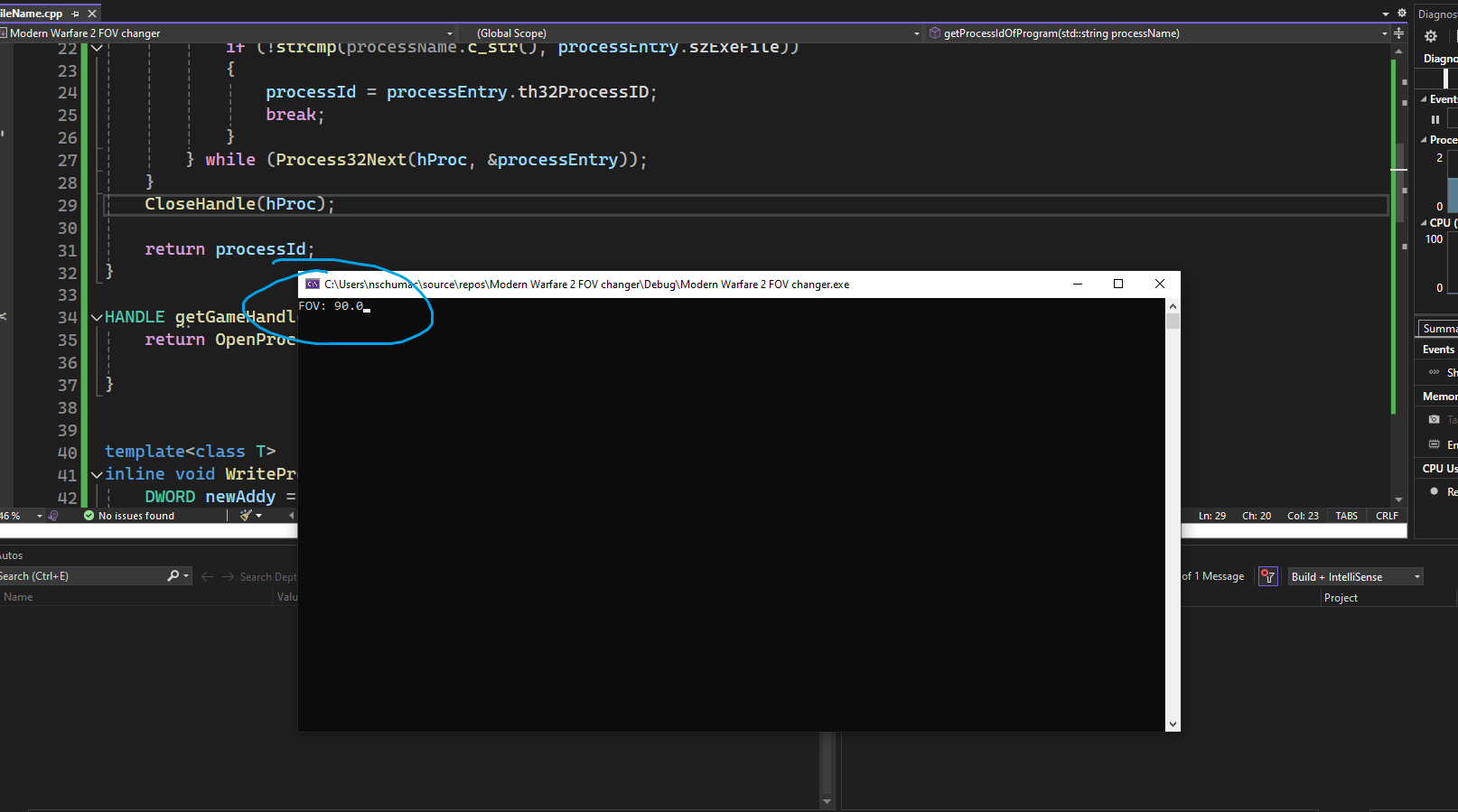
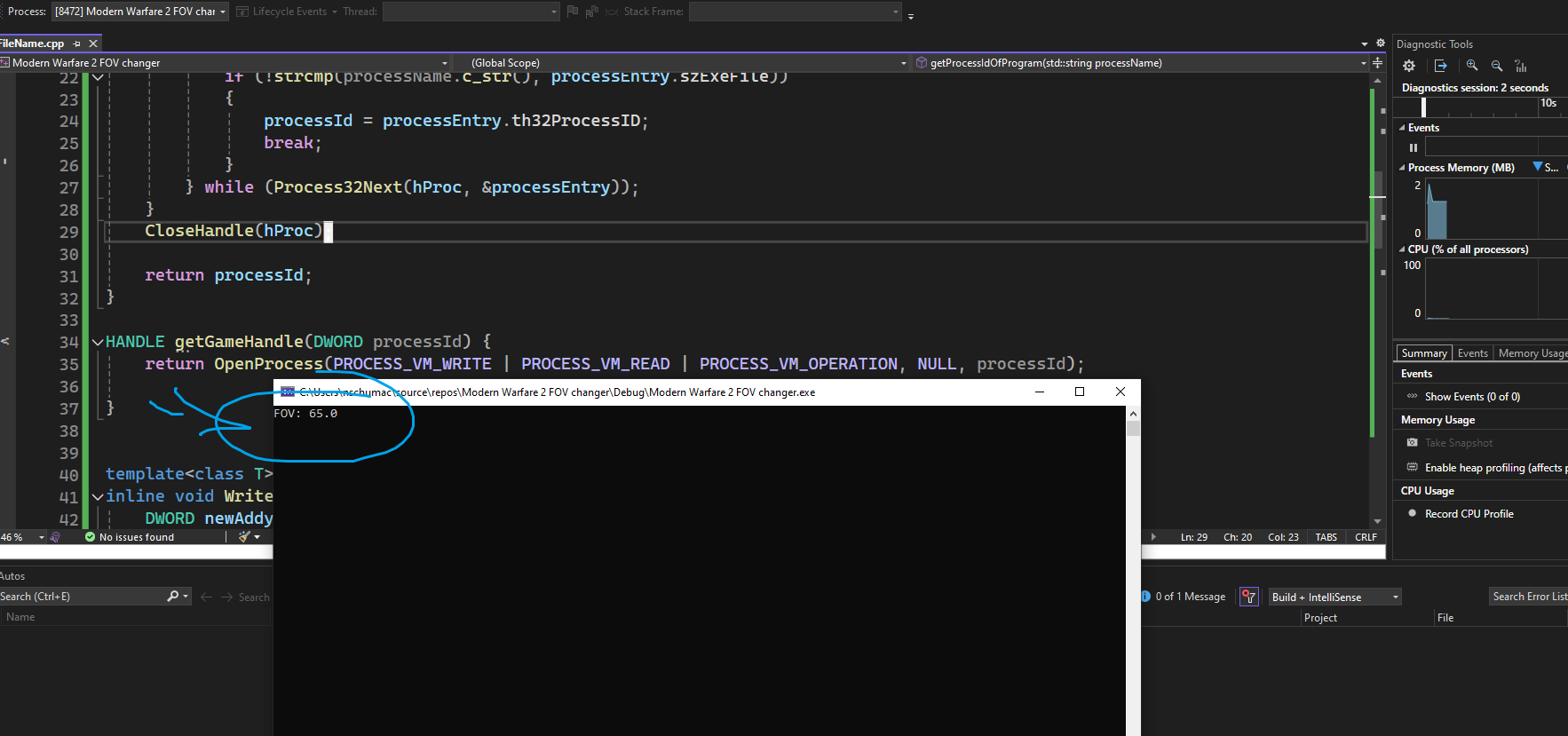
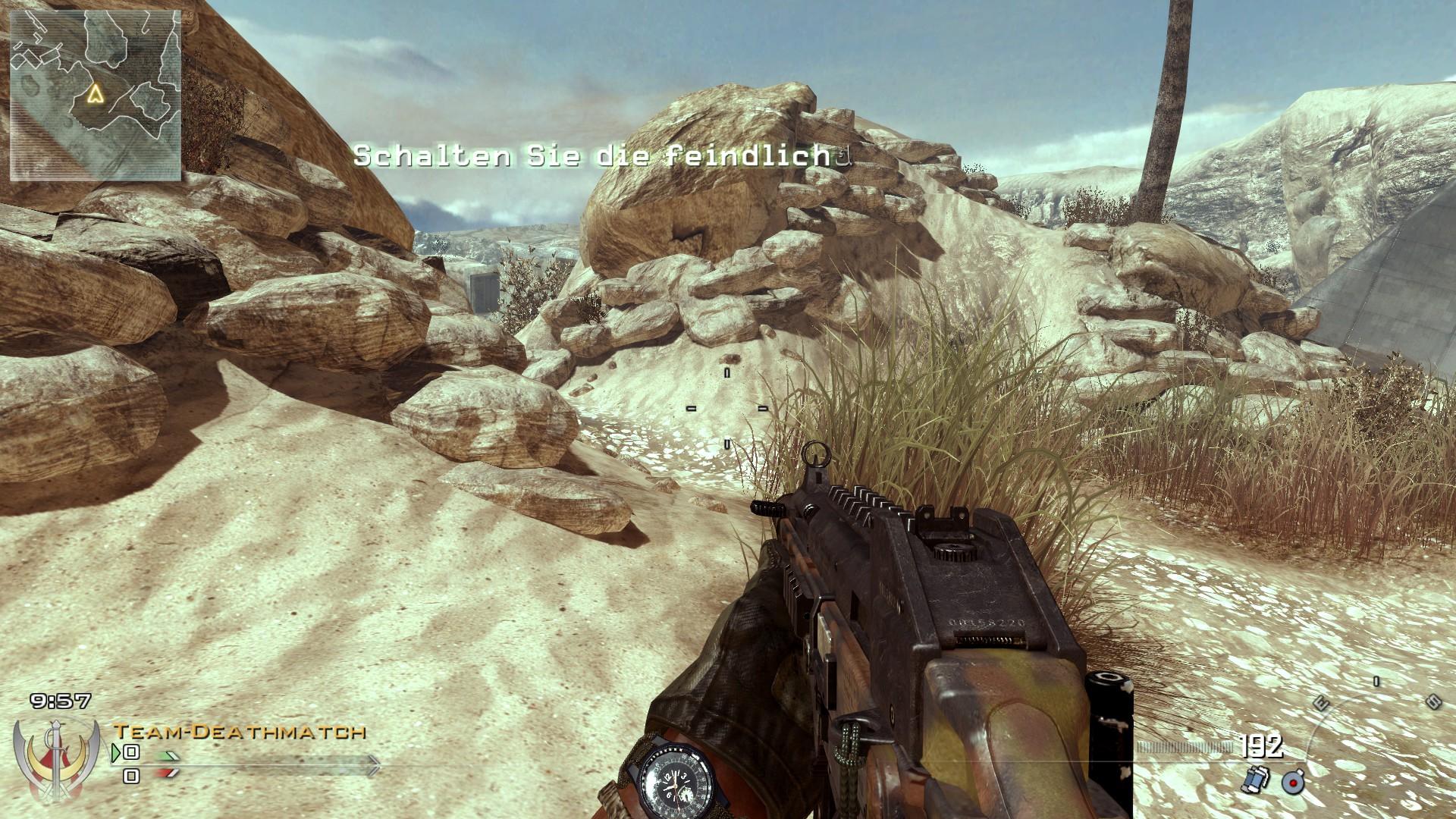
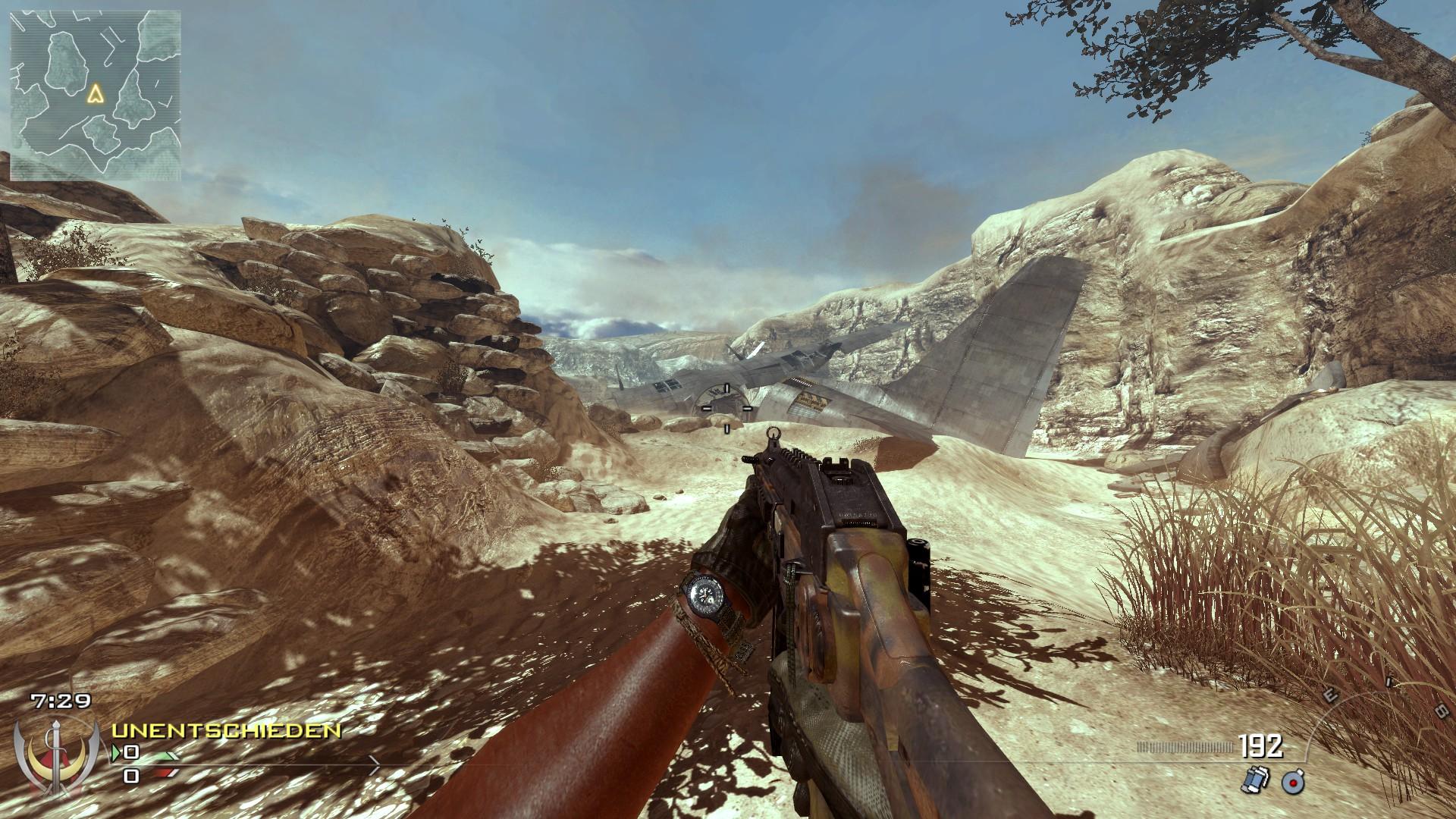
I use openprocess to get the gamehandle (access to write to the game)
I created a write process fucntion which will read the the address add the offset and write the value we want a float to fov.
I also created a small loop which listens for keystrokes
- the add button closes programm
- left and right arrow keys decrease / increase fov