Breakout Game in JavaScript
.gif)
Hello, today I'm going to be teaching you how to make a simple Breakout like game using JavaScript. Just like the rotating cube there are many editable features, including amount of breakable brick rows, amount of breakable brick columns, the gab between each brick, amount of player lives, amount of levels, starting ball speed, max ball speed, paddle width and much more. I'm also going to try to explain everything as well. I learnt all this from Mt. Ford Studios and I highly recommend you check him out!!
Supplies
- A text editor of any kind
- the Developer tools for your browser
Open Your Text Editor
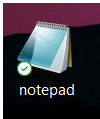.png)

The first thing you need to do is open your text editor. For this project I'm just going to be using Notepad. Before we start writing the code, we need to save the document, save it as an .html file. I called mine Breakout.html but you can call it whatever you like. Also make sure that the file is encoded in UTF-8.
Start Writing

Now we can start coding. The first thing we need to do is to write a basic html layout. What this is basically saying so far is that the language the html is using is English, it is using UCF-8 character encoding, it will contain "viewport" content, and that the title is "Breakout". Also like the rotating cube, we need to set the margins to 0, which is what is in the <style> … </style> section.
Downloads
Game Paremeters

After writing our basic html layout we can start writing the game parameters. These are the constants that we can change any time we want. Here is a list of constants that you can change:
BALL_SPD is the balls starting speed which is (BALL_SPD/1) x height of the screen per second
BALL_SPD_MAX is the max speed the ball which is itself times BALL_SPD
BRICK_COLS is the number of brick columns
BRICK_GAP is the gap between each brick which is (BRICK_GAP/1) x width of the wall (all of the bricks)
BRICK_ROWS is the starting number of brick rows
GAME_LIVES is the starting number of game lives
MAX_LEVEL is the amount of levels in the game with each level having +2 rows of brick
PADDLE_SPD is the speed of the paddle which is (PADDLE_SPD/1) x width of the screen per second
PADDLE_W is the width of the paddle which is (PADDLE_W/1) x width of the screen
Downloads
Colors

Once we finish writing the game parameters, we can write the colors. Sadly we can't really change the color of the bricks. Here is the list of constants you can change:
COLOR_BACKGROUND is the color of the background
COLOR_BALL is the color of the ball
COLOR_PADDLE is the color of the paddle
COLOR_TEXT is the color of the text
COLOR_WALL is the color of the margin
Downloads
Text

Now we can write the text. This is essentially the font of the text, all the words, the win text and the lose text. Here is a list of things you can change:
TEXT_FONT is the font of the text
TEXT_GAME_OVER is the text said when you lose
TEXT_LEVEL is the text next to the level you're at
TEXT_LIVES is the text next to your lives
TEXT_SCORE is the text next to the score
TEXT_SCORE_HIGH is the text next to your high score
TEXT_WIN is the text said when you win
Downloads
Definitions

This is just a tiny section that defines our movement with numbers 0, 1 and 2. In this case, left is defined as 0, right is defined as 1, and stop is defined as 2. Stop is just what we use to define the up key on your keyboard.
Downloads
Canvas and Context

After writing the Definitions, we will set up the canvas and context. This will be used to create the graphics of our game.
Downloads
Game Variables

Now we need to write the game variables. Our variables are going to be ball, bricks = [], paddle, gameOver, win, level, lives, score, scoreHigh, numBricks, textSize and touchX.
Downloads
Dimensions

Here we will right the variables for all of our dimensions.
Downloads
Event Listeners

An Event listener is basically a procedure that waits for an event to occur. In our game the important ones are Touchstart which will be used to reset the game when you lose, keydown which will be used to start the game by pressing space and keyup which will stop the left and right movements when you stop pressing the right or left arrow keys.
Downloads
Game Loop

The Game Loop is divided into 5 sections: Setting up the game loop, Calculate the time difference, Update, Draw, and Call the next loop.
In Setting up the game loop, var timeDelta, timeLast are the variables used to make the animation look fluid in Calculate the time difference. requestAnimationFrame(loop) is used to perform the animation.
In Calculate the time difference, timeDelta = (timeNow - timeLast) * 0.001; and timeLast = timeNow; are made so the frame is updated every 0.001 seconds.
In Update, the paddle, ball and bricks are all being told to update by timeDelta, which is 0.0001 seconds
In draw, it draws all the components of the game.
In Call the next loop, it continually executes the update, making everything animated.
Downloads
Velocities of the Ball

Now we need to update the x and y velocities of the ball. The math for the x velocity is [cos (ball speed)] and the math for the y velocity is [sin (- ball speed)].
Downloads
Functions
There are a lot of functions we need to define. So instead of making a separate text file for each function, I am going to put the text file at the end of all the functions.
Function CreateBricks

This function is used to create the brick wall, and has 3 sections which are row dimensions, column dimensions and populate the brick array.
The row dimensions section calculates the dimensions of each row and how many rows to be added for each level.
The column dimensions section calculates the dimensions of each column.
The populate the brick array section calculates how many bricks must be in each row and column and equally distributes them into the walls height and width.
Function DrawBackground

This function creates and colors the background with the color we chose at the start.
Function DrawBall

This function creates and colors the ball with the color we chose at the start.
Function DrawBricks

This function creates creates and colors the bricks white.
Function DrawPaddle

This function creates and colors the paddle with the color we chose at the start.
Function DrawText


This functions creates and colors the text with the color we chose at the start. This function then places each piece of text that we chose into its assigned area.
Function DrawWalls

This functions creates and colors the margin with the color we chose at the start.
Function KeyDown(ev)

This function controls the space bar, which is used to start the game, and the left and right arrow keys of the paddle.
Function KeyUp(ev)

this functions stops the paddle from moving until the game starts. The game starts when the space bar is pressed.
Function MovePaddle

This function moves the paddle left if the left arrow key is selected, right if the right arrow key is selected and stops if no arrow key is selected.
Function NewBall

This function tells the game to create a new ball and paddle when you lose.
Function NewGame

This function tells the game to reset the levels and reset your lives once you lose the game. It also displays your high score.
Function NewLevel

This function resets the bricks and you ball after every level.
Function OutofBounds

This function takes away a life when your ball falls out of bounds. If all of your lives are taken away, its game over.
Function Serve

This functions moves the ball in a random direction when it hits the paddle.
Function SetDimensions

This function sets the walls that the ball bounces off of.
Function SpinBall

This function sets the minimum angle the ball can move when it bounces off the paddle.
Function TouchCancel, TouchEnd, TouchMove, and TouchStart

The touchCancel, touchEnd, touchMove, and touchStart functions are all for if your using a device that is touchscreen. If your computer is touchscreen, try it out with the end result.
Function UpdateBall(Delta)

This function allows the ball to bounce back off the walls, bounce off the paddle, and move with the paddle when it is stationary. It also handles if the ball is out of bounds.
Function UpdateBricks(Delta)

This function allows each brick to detect ball collisions, bounce the ball off itself, and destroy itself if the ball hits it. It also updates the amount of brick once there is a new level.
Function UpdatePaddle(Delta)

This function allows the paddle to move and allow the ball to bounce back off it.
Function UpdateScore(brickScore)

This function records the high score in local storage so it can be displayed.
Function Ball

This function places the ball right in the middle of the paddle when the game starts or when a new level starts.
Function Brick

This function places the bricks and puts a hit box on them so they can be hit by the ball.
Function Paddle

This function creates the paddle using it's length and width we chose at the start.
Finished

Congrats! You finished the game. If you want, you can experiment with the values and make it look even better. If you don't want to go through the whole process of writing all the code, I will link the source code below.