Bluetooth Low Energy and IBeacons With Tessel
by SelkeyMoonbeam in Circuits > Gadgets
5220 Views, 29 Favorites, 0 Comments
Bluetooth Low Energy and IBeacons With Tessel
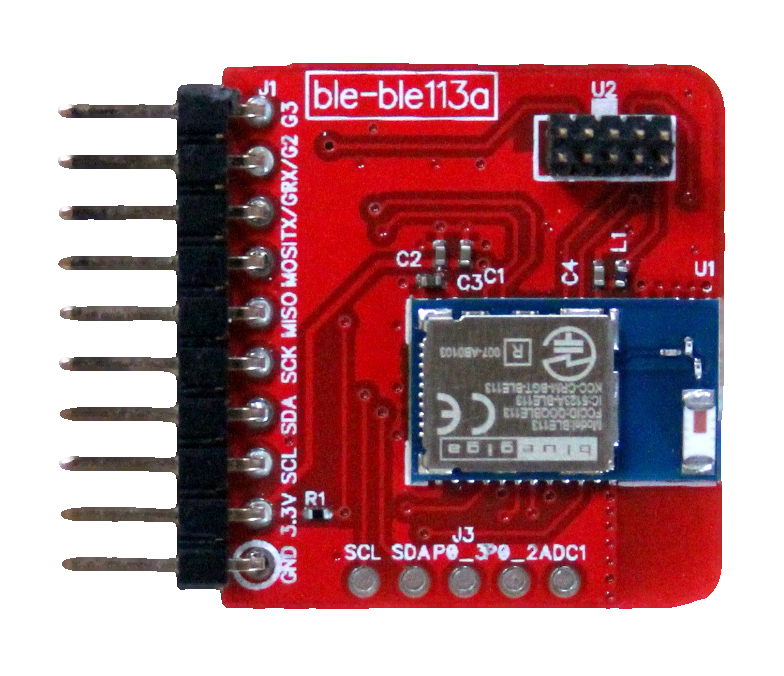
Tessel is a microcontroller you program in JavaScript. It's like Arduino, but designed to be easier to use and web-friendly.
Bluetooth Low Energy is a communication protocol that's designed to use very little energy, transferring small packets of data relatively slowly. This is good for battery-powered applications that stay in one place for a long period of time, for example emitting sensor data.
I recommend you read Evan Simpson's beginner guide to BLE if you're interested in learning more about the protocol and its uses.
In this tutorial, I'll show you how to get a Bluetooth Low Energy module up and running with a Tessel, then show you how to make it into an iBeacon.
Here's what you need:
- Tessel
- Bluetooth Low Energy module
- Another Bluetooth Low Energy device, like a newer smartphone (or another BLE module) for your Tessel to talk to
Install Tessel
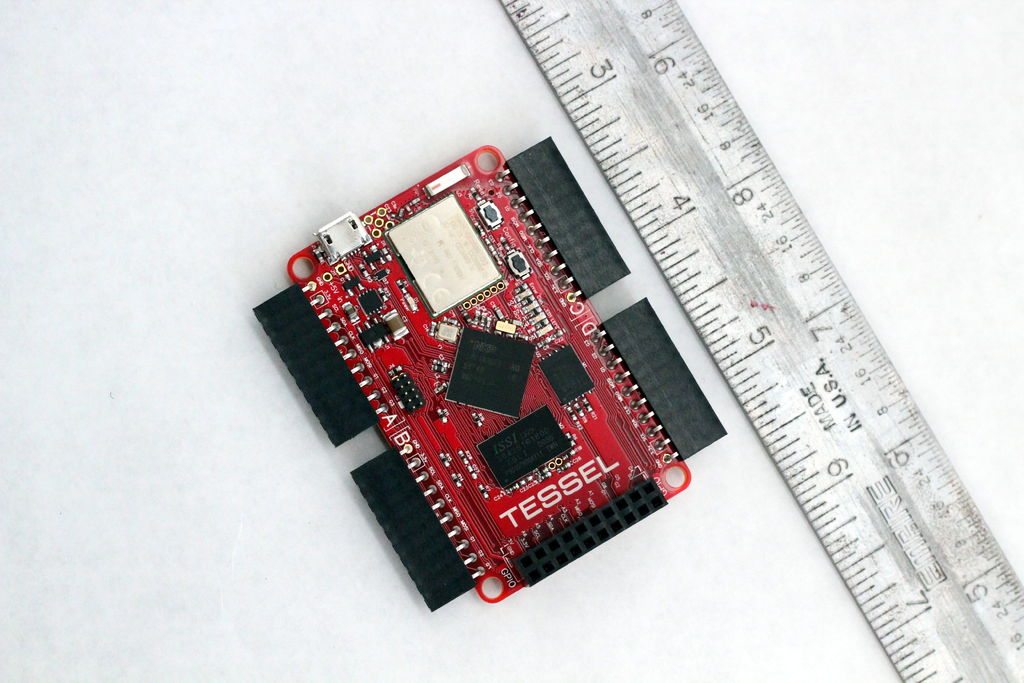
If you haven't used Tessel before, you should go install it.
Plug the Module Into Tessel's Port A
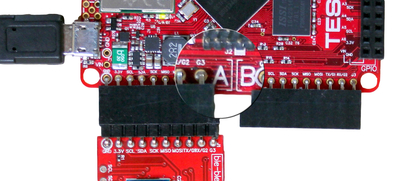
It's best to unplug Tessel from power while plugging in modules.
Plug the BLE module into Tessel port A with the hexagon/icon side down and the electrical components on the top, then plug Tessel into your computer via USB.
Install the Module
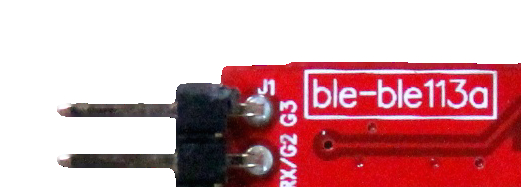
Install by typing
npm install ble-ble113a
into the command line.
Code
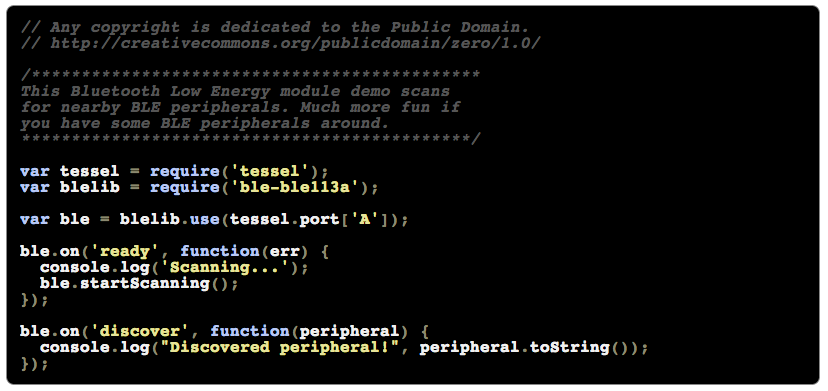
Save this code in a text file called ble.js:
// Any copyright is dedicated to the Public Domain.
// http://creativecommons.org/publicdomain/zero/1.0********************************************* This Bluetooth Low Energy module demo scans for nearby BLE peripherals. Much more fun if you have some BLE peripherals around. *********************************************/ var tessel = require('tessel'); var blelib = require('ble-ble113a'); var ble = blelib.use(tessel.port['A']); ble.on('ready', function(err) { console.log('Scanning...'); ble.startScanning(); }); ble.on('discover', function(peripheral) { console.log("Discovered peripheral!", peripheral.toString()); });
Push the Code
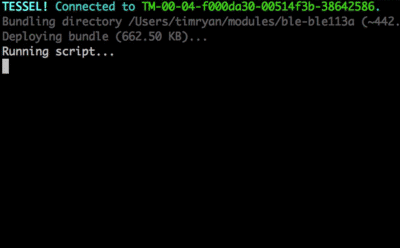
In your command line,
tessel run ble.js
Set a Bluetooth Low Energy device nearby to advertising and see if Tessel can find it!
Bonus: Change the code to print out only the IP address of discovered peripherals.
To see what else you can do with the BLE module, see the module docs here.
Make a IBeacon
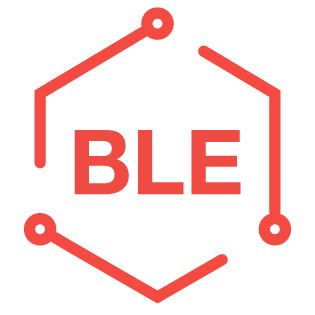
Now that you have your BLE module working, try making it emit data as an iBeacon.
An 'iBeacon' is a very specifically formatted advertising data packet. Evan Simpson wrote a very nice blog post explaining iBeacons, their merits, downfalls, and alternatives: You Probably Don't Need an iBeacon. I recommend you read it.
But in the meanwhile, here's the code to make your Tessel BLE module into an iBeacon:
var tessel = require('tessel');
var bleLib = require('ble-ble113a'); var bleadvertise = require('bleadvertise'); var uuid = 'D9B9EC1F392543D080A91E39D4CEA95C'; // Apple's example UUID var major = '01'; var minor = '10'; var iBeaconData = new Buffer(uuid+major+minor, 'hex'); // Create data Buffer var packet = { flags: [0x04], // BLE only mfrData : iBeaconData } var ad = bleadvertise.serialize(packet); var beacon = bleLib.use(tessel.port['A'], function(){ beacon.setAdvertisingData(ad, function(){ beacon.startAdvertising(); console.log('Beaconing'); }); });
Again, run with
tessel run ble.js